In C++, you can check if a string ends with a specific suffix using the `std::string::compare()` method in combination with the `substr()` method.
Here's a code snippet to illustrate this:
#include <iostream>
#include <string>
bool endsWith(const std::string& str, const std::string& suffix) {
return (str.size() >= suffix.size()) &&
(str.compare(str.size() - suffix.size(), suffix.size(), suffix) == 0);
}
int main() {
std::string myString = "Hello, World!";
std::string suffix = "World!";
if (endsWith(myString, suffix)) {
std::cout << "The string ends with the given suffix." << std::endl;
} else {
std::cout << "The string does not end with the given suffix." << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is essentially a sequence of characters used to represent text. Unlike C-style strings, which are arrays of characters terminated by a null character (`'\0'`), C++ provides the `std::string` class as part of the Standard Template Library (STL). This class abstracts away the complexities of handling character arrays, allowing for easier and more efficient string manipulation.
Common String Operations in C++
Strings in C++ support various operations that are fundamental for text processing. Here are some common operations:
- Concatenation: You can easily combine two or more strings using the `+` operator.
- Substring: The `substr()` method allows extraction of a portion of the string.
- Length of a String: Use the `length()` or `size()` method to get the number of characters in a string.
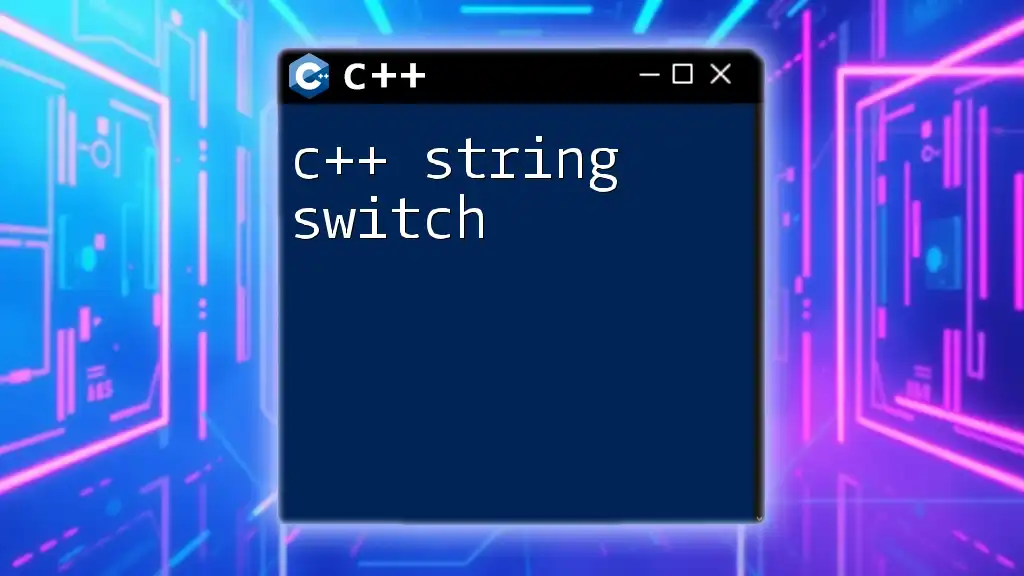
Why Check if a String Ends With a Substring?
Use Cases
Checking if a string ends with a specific substring is a common requirement in many programming scenarios. Here are some use cases where this functionality is essential:
- Validating File Extensions: When processing files, you might want to ensure that a file is of a specific type by checking its extension.
- Checking Prefixes: In web development, you may need to verify if URLs or identifiers conclude with certain markers for routing or validation purposes.
Performance Considerations
While the performance overhead for string comparisons is generally negligible for small strings, it can become a concern in scenarios involving large datasets or frequent comparisons. Knowing the most efficient methods is beneficial for optimizing performance.
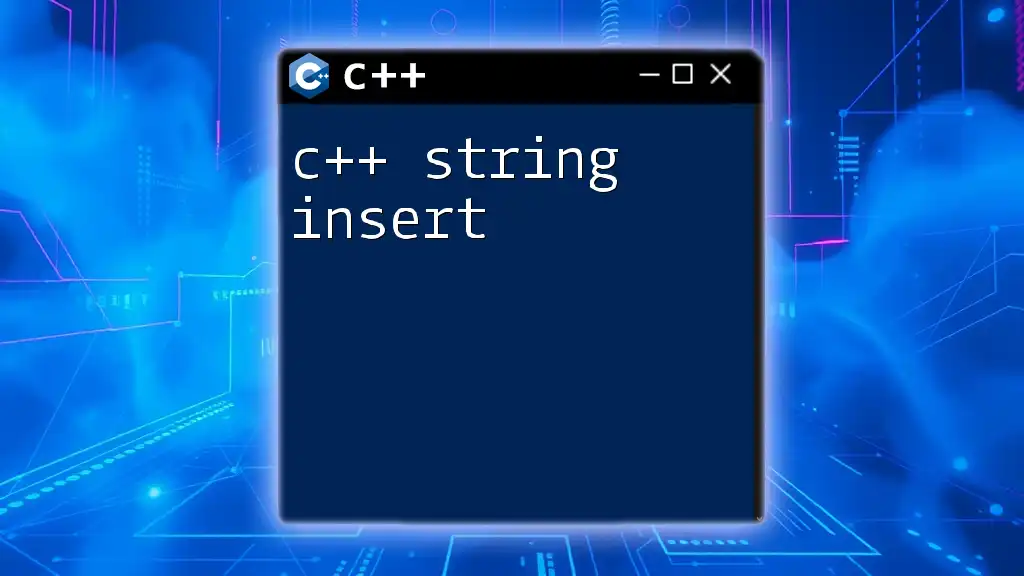
Techniques to Check if a String Ends With a Substring
Traditional Approach Using STL Functions
One common method for checking if a C++ string ends with a specific substring involves using the `std::string::substr()` method. Here's an example implementation:
#include <iostream>
#include <string>
bool endsWith(const std::string &str, const std::string &suffix) {
if (str.length() >= suffix.length())
return (str.compare(str.length() - suffix.length(), suffix.length(), suffix) == 0);
else
return false;
}
int main() {
std::string myString = "example.txt";
std::cout << std::boolalpha << endsWith(myString, ".txt") << std::endl; // Outputs: true
}
In this code, the `endsWith` function checks if the string `str` ends with the substring `suffix`. It first checks if the length of `str` is greater than or equal to the length of `suffix`. If so, it performs a comparison on the relevant portion of `str`.
Using `std::string::compare()`
Another approach is to utilize the `std::string::compare()` method directly, allowing for a more concise implementation. The following example demonstrates this technique:
bool endsWithCompare(const std::string &str, const std::string &suffix) {
return str.size() >= suffix.size() &&
str.compare(str.size() - suffix.size(), suffix.size(), suffix) == 0;
}
This function carries out a very similar operation but in a more streamlined manner.
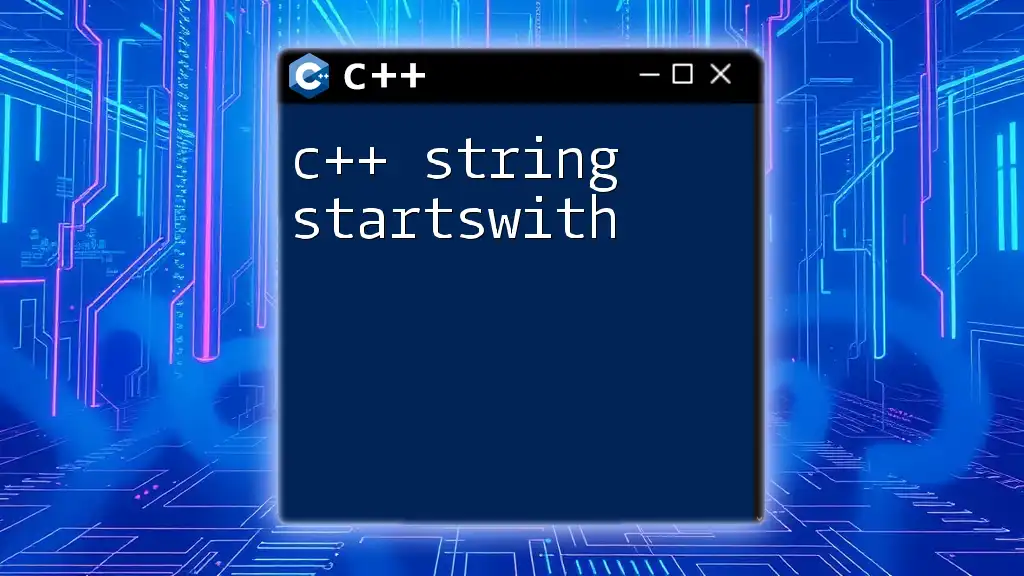
Using C++17 std::string Features
With the introduction of C++17, the `std::string` class gained a new member function: `ends_with()`. This method provides a clear and efficient way to determine if a string ends with a specified substring:
#include <iostream>
#include <string>
int main() {
std::string myString = "test.pdf";
if (myString.ends_with(".pdf")) {
std::cout << "The string ends with '.pdf'" << std::endl;
}
}
This new functionality simplifies syntax and enhances readability, making it the preferred method in modern C++ programming.
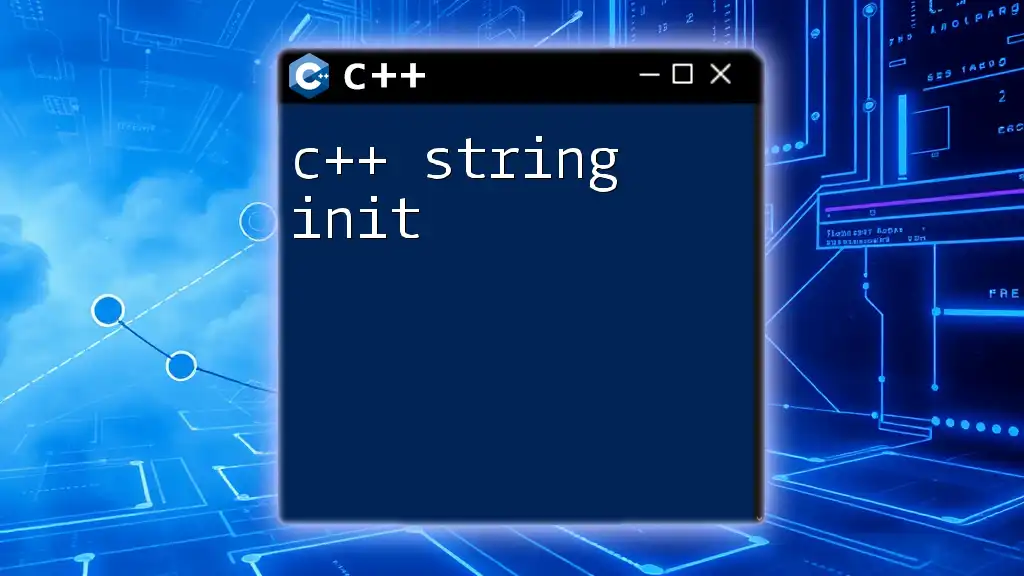
Edge Cases
Empty Strings and Suffixes
When working with strings, handling edge cases such as empty strings is crucial. The following behaviors are important to note:
- An empty string should be considered to end with another empty string (`""`).
- Any string should end with an empty suffix.
- However, an empty string should not end with a non-empty suffix.
Here’s how these conditions can be observed in code:
std::cout << endsWith("", "") << std::endl; // true
std::cout << endsWith("test", "") << std::endl; // true
std::cout << endsWith("", "test") << std::endl; // false
Case Sensitivity
By default, string comparisons in C++ are case-sensitive. This means that comparing `"Hello"` and `"hello"` will yield `false`. Developers often need to handle case sensitivity explicitly if required. One option is converting both strings to the same case before performing the comparison:
#include <algorithm>
bool endsWithCaseInsensitive(const std::string &str, const std::string &suffix) {
std::string strLower = str, suffixLower = suffix;
std::transform(strLower.begin(), strLower.end(), strLower.begin(), ::tolower);
std::transform(suffixLower.begin(), suffixLower.end(), suffixLower.begin(), ::tolower);
return endsWith(strLower, suffixLower);
}
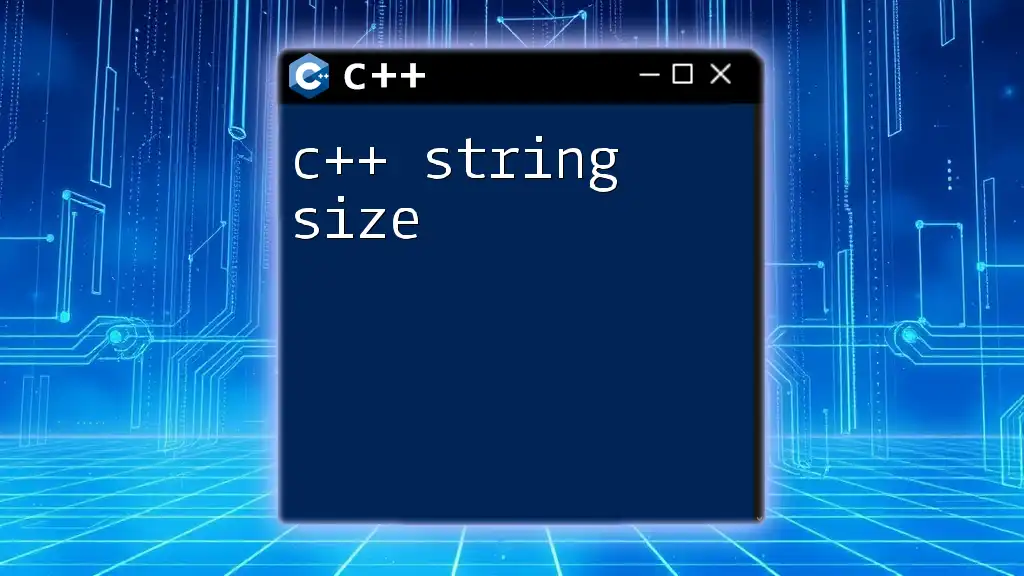
Conclusion
In this article, we explored various methods for determining if a C++ string ends with a specific substring, focusing on the powerful `std::string` class. From traditional approaches using STL functions to the elegant simplicity of the new `ends_with()` feature in C++17, developers now have several effective tools at their disposal.
We encourage you to implement these techniques in your programming endeavors and experiment with different string manipulations. Whether you are validating file types, ensuring URL integrity, or simply learning more about C++ string handling, mastering the concept of string endings can significantly improve your coding proficiency.
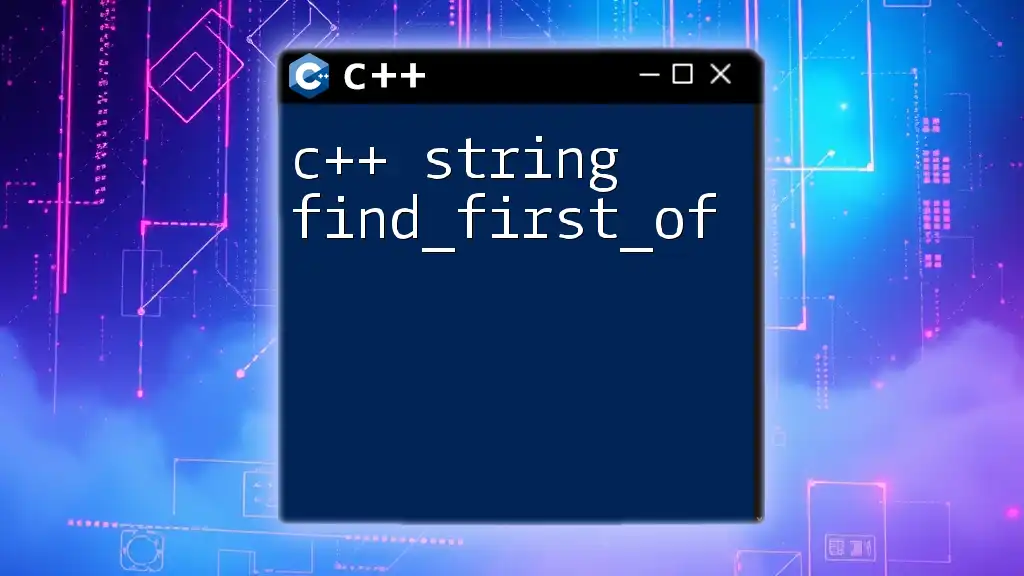
Additional Resources
To further enhance your understanding of string handling in C++, consider checking out the following resources:
- [C++ Standard Documentation](https://en.cppreference.com/w/cpp/string/basic_string)
- [String Manipulation Tutorials](https://www.learncpp.com/cpp-tutorial/strings-in-c/)
We would love to hear your thoughts! Feel free to share your own string manipulation techniques or engage in discussions about different approaches in the comments below.