In C++, you can convert a string to its hexadecimal representation by iterating through each character and transforming it into its corresponding hexadecimal value using a stream. Here's a code snippet demonstrating this:
#include <iostream>
#include <sstream>
#include <iomanip>
std::string stringToHex(const std::string& str) {
std::ostringstream oss;
for (char c : str) {
oss << std::hex << std::setw(2) << std::setfill('0') << static_cast<int>(c);
}
return oss.str();
}
int main() {
std::string myString = "Hello";
std::string hexString = stringToHex(myString);
std::cout << "Hexadecimal: " << hexString << std::endl;
return 0;
}
Understanding Hexadecimal Representation
What is Hexadecimal?
Hexadecimal, often abbreviated as "hex," is a base-16 numbering system that uses digits 0-9 and letters A-F (or a-f) to represent values. Each hex digit corresponds to a four-bit binary value, making it highly useful in programming and computer science. For instance, the decimal number 255 is represented as FF in hexadecimal.
Why Use Hexadecimal?
Hexadecimal notation is prevalent in programming because it allows for a more human-readable representation of binary-coded values. This is particularly useful in fields such as:
- Memory address representation: Hex numbers are shorter and more readable than binary.
- Color coding in web development: Colors are often represented in hex format, like `#FFFFFF` for white.
- Data encoding and encryption: Many algorithms utilize hex values for their compactness and clarity.
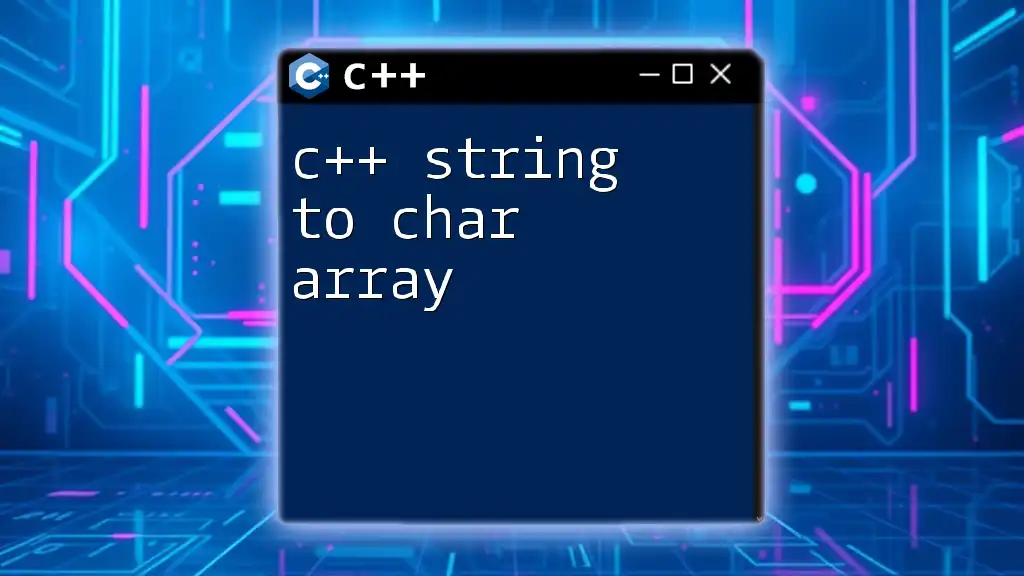
Exploring C++ Strings
What are C++ Strings?
In C++, strings are a sequence of characters used to store textual data. They are part of the Standard Library and are encapsulated in the `std::string` class. C++ strings are dynamic, meaning their size can be adjusted as the program runs, making them extremely versatile for string manipulation tasks.
String Data Types in C++
C++ supports two primary types for string data:
- `std::string`: This is the standard string class, offering methods for handling dynamic text strings efficiently.
- Character arrays (`char[]`): These are fixed-size strings and require manual management of memory and string termination.
Advantages of using `std::string` include:
- Automatic memory management
- Extensive built-in operations for search, compare, and manipulate strings, increasing productivity and reducing errors.
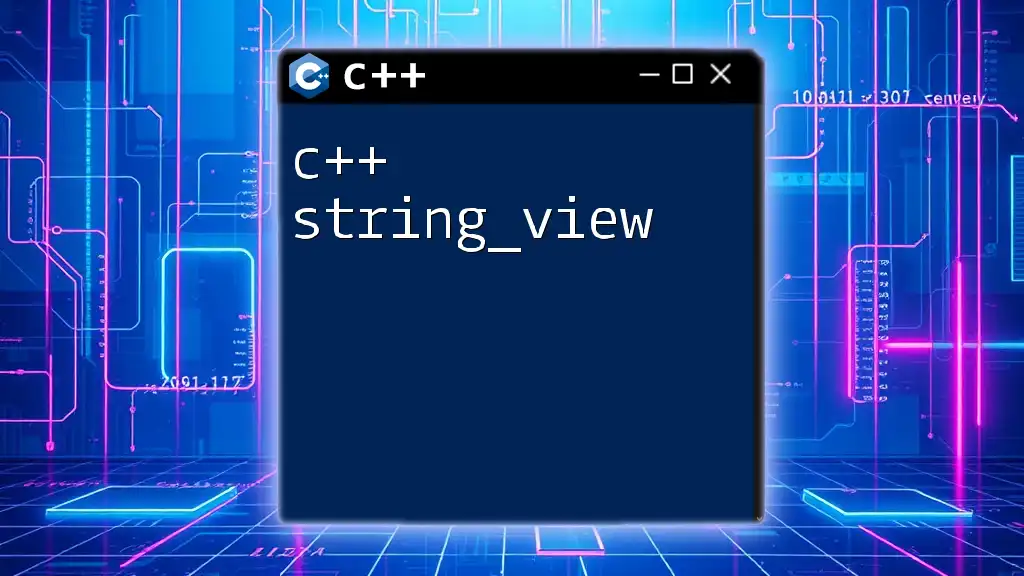
Converting C++ Strings to Hex
Basic Concept of String to Hex Conversion
When converting a C++ string to hex, each character in the string is represented by its ASCII value, which is then converted into its corresponding hex representation. Each byte (8 bits) maps to two hexadecimal digits.
C++ Code Snippet: Basic Conversion
Here’s a straightforward implementation of a function to convert a string to its hexadecimal representation:
#include <iostream>
#include <sstream>
#include <iomanip>
std::string stringToHex(const std::string& input) {
std::ostringstream oss;
for (char c : input) {
// Convert each character to hex
oss << std::hex << std::setw(2) << std::setfill('0') << (int)c;
}
return oss.str();
}
In this code:
- `std::ostringstream` is used for building the output string.
- `std::hex`, along with `std::setw()` and `std::setfill()`, ensures that each character is represented by two hexadecimal digits, properly formatted to avoid loss of leading zeros.
Example of Using the Basic Conversion Function
Here’s how you can use the `stringToHex` function in a C++ program:
int main() {
std::string myString = "Hello";
std::string hexString = stringToHex(myString);
std::cout << "Hexadecimal: " << hexString << std::endl;
return 0;
}
When executed, this program will output:
Hexadecimal: 48656c6c6f
This result, "48656c6c6f," is the hex representation of the string "Hello."
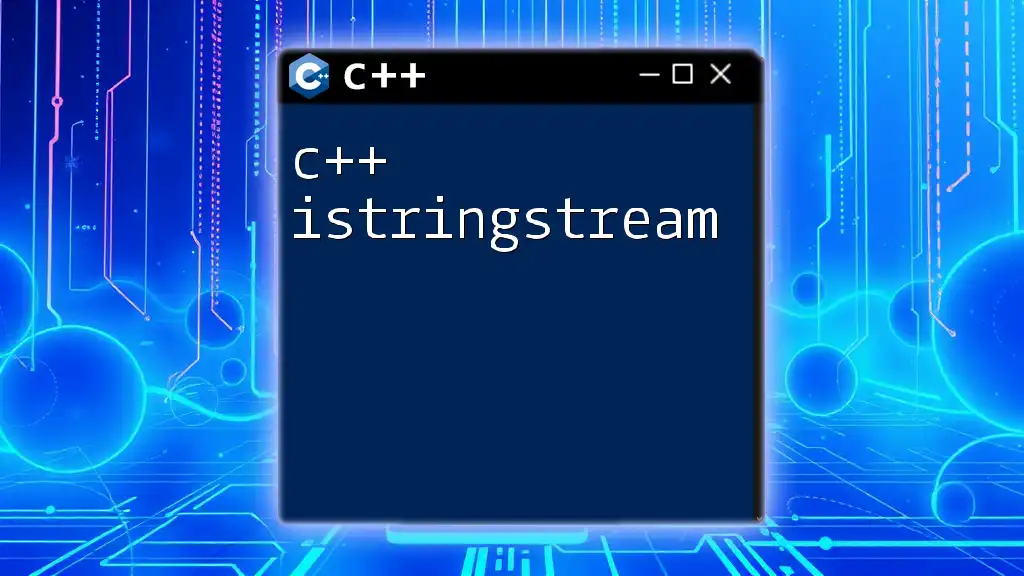
Handling Different Character Encodings
Understanding Character Encodings
Character encoding refers to the way characters are stored and represented in memory, with common encodings including ASCII and UTF-8. ASCII can represent 128 characters, while UTF-8 can represent every character in the Unicode standard, allowing for a much broader set of symbols, including multi-byte characters.
Converting UTF-8 Strings to Hex
For characters represented in UTF-8, the conversion process must be more thoughtful as characters can take up more than one byte. Here’s a code snippet addressing this scenario:
#include <iostream>
#include <sstream>
#include <iomanip>
#include <string>
std::string utf8StringToHex(const std::string& utf8String) {
std::ostringstream hexStringStream;
for (unsigned char c : utf8String) {
hexStringStream << std::hex << std::setw(2) << std::setfill('0') << (int) c;
}
return hexStringStream.str();
}
Example of UTF-8 Conversion
In this example, if you want to convert a UTF-8 encoded string like "Café" to hex, you would do it as follows:
int main() {
std::string utf8String = "Café";
std::string hexOutput = utf8StringToHex(utf8String);
std::cout << "UTF-8 Hexadecimal: " << hexOutput << std::endl;
return 0;
}
Running this code will produce:
UTF-8 Hexadecimal: 436166c3a920
Here, the additional bytes for the character "é" are correctly accounted for in the hex output.
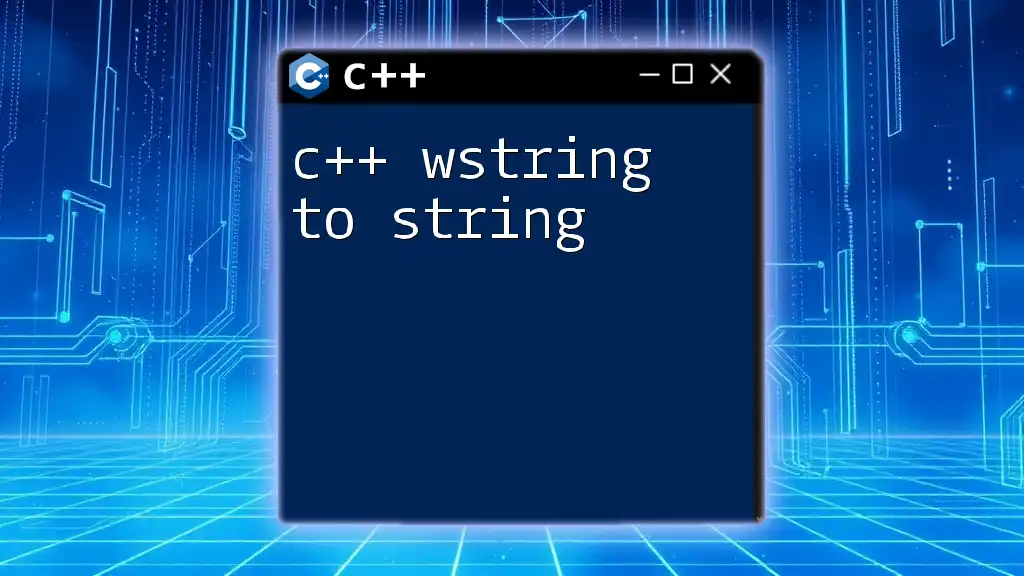
Common Pitfalls and Troubleshooting
Handling Non-Printable Characters
When working with string conversions, you might encounter non-printable characters that can introduce errors or unexpected results. It is good practice to filter these characters before conversion. For instance, you might want to skip characters with ASCII values under 32.
Performance Considerations
Efficiency is a crucial aspect when converting large strings. The use of `std::ostringstream` is preferred for performance. However, if you're working with extremely large data sets, consider reducing memory allocations by reserving space in your output string stream beforehand.
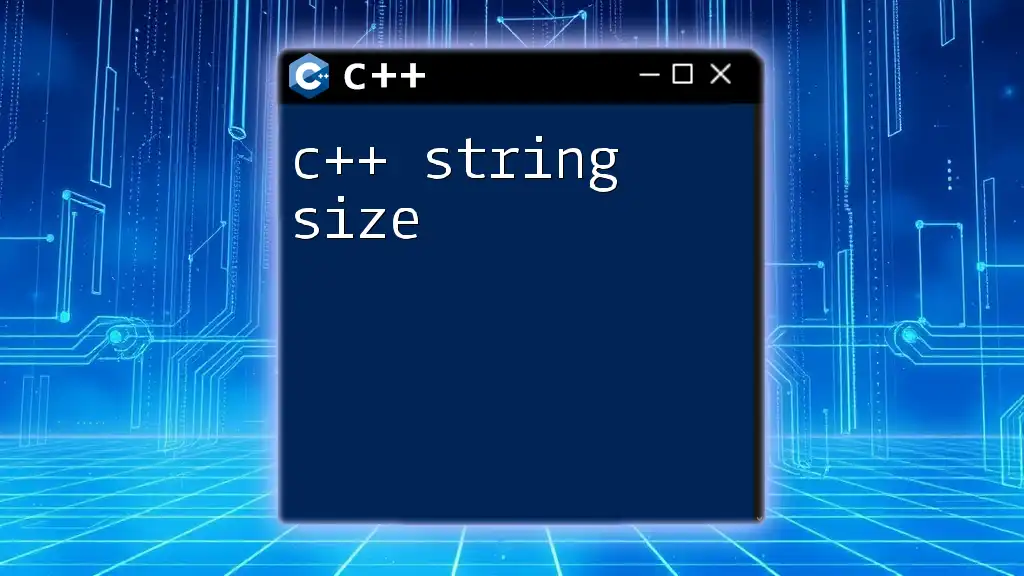
Advanced Topics
String to Hex using Template Functions
C++ template functions allow for creating generic solutions, enabling you to convert various data types to hex format. Here’s an example function:
template <typename T>
std::string toHex(const T& input) {
std::ostringstream oss;
const char* data = reinterpret_cast<const char*>(&input);
for (size_t i = 0; i < sizeof(T); ++i) {
oss << std::hex << std::setw(2) << std::setfill('0') << static_cast<int>(data[i]);
}
return oss.str();
}
Using templates, you can easily extend the conversion capability to numbers, structs, or any type defining operator overloading for the output stream.
Using Standard Libraries for Conversion
For more complex applications, leveraging libraries like Boost or OpenSSL can simplify conversion tasks. These libraries have built-in functionalities for secure hexadecimal conversion and data management. Look into the specific library documentation for usage examples tailored to your needs.
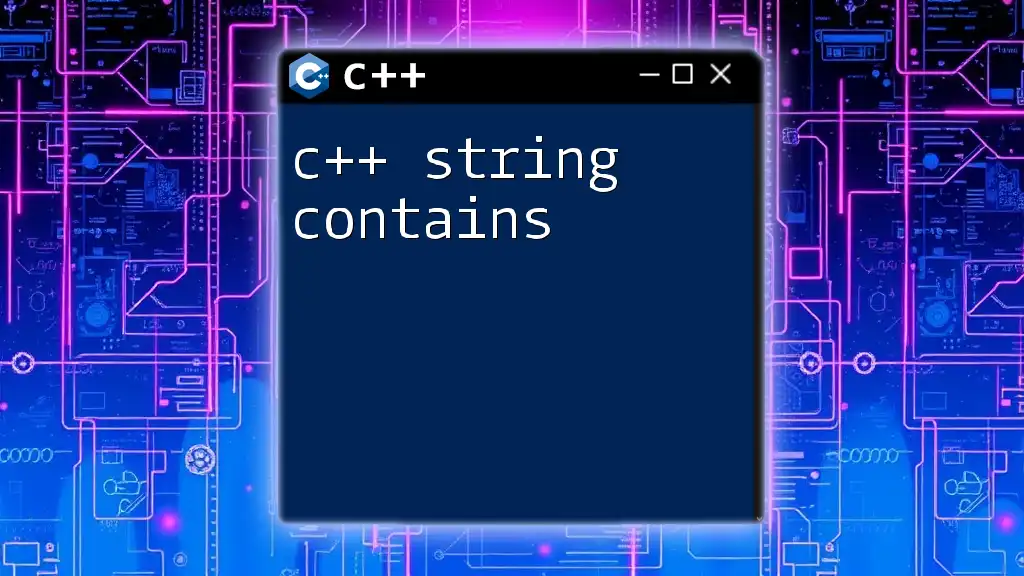
Conclusion
Converting C++ strings to hexadecimal is a foundational task that supports a variety of applications, from memory management to data transmission. Whether working with basic ASCII strings or handling UTF-8 encoding, having a strong grasp of these techniques enhances your programming repertoire and enables clear data representation.
The ability to convert strings to hex is not just a technical requirement but a powerful tool that can be applied in many domains. Engaging in practice and experimentation with the provided code snippets will help you become proficient in this skill. Happy coding!