In C++, you can print a value in hexadecimal format using the `std::hex` manipulator along with `std::cout`. Here’s an example:
#include <iostream>
int main() {
int value = 255;
std::cout << "Hexadecimal: " << std::hex << value << std::endl;
return 0;
}
Understanding Hexadecimal Numbers
What is Hexadecimal?
Hexadecimal, or base-16, is a numeral system that uses sixteen distinct symbols: the numbers 0-9 represent values zero to nine, and the letters A-F denote values ten to fifteen. This system is a compact way to represent binary data, making it especially useful in programming, computer science, and digital electronics. In contrast to decimal (base-10) and binary (base-2), hexadecimal simplifies the representation of large binary values, as each hexadecimal digit corresponds to four binary bits.
Why Use Hexadecimal in C++?
Hexadecimal is commonly used in programming for tasks such as:
- Representing memory addresses, which are often displayed in hexadecimal for clarity.
- Dealing with color codes in graphic programming, where colors are expressed in hex format (e.g., #FF5733).
- Working with binary data representation, as it offers a simpler way to visualize data compared to binary directly.

Using cout to Print Hexadecimal in C++
Introduction to cout
In C++, `cout` is an output stream that allows programmers to send data to the standard output screen. To use `cout`, you need to include the `<iostream>` header. Here's a basic example of how `cout` operates:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This snippet simply prints "Hello, World!" to the console.
Basic Syntax for cout
The basic structure for using `cout` involves the insertion operator `<<`, which directs output to the console. Below is a simple example followed by a slightly extended usage illustration:
int num = 42;
std::cout << "The number is: " << num << std::endl;
The above code outputs "The number is: 42".
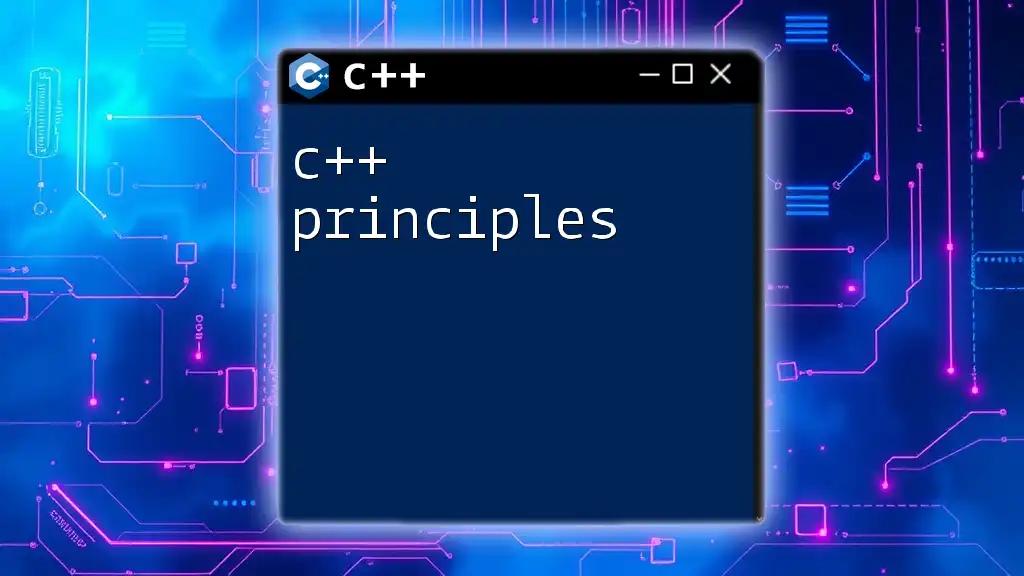
Printing Hexadecimal with cout
Setting Output Format to Hex
To display numbers in hexadecimal format, you utilize the `std::hex` manipulator, which modifies the stream so that any subsequent integer output will be represented as a hexadecimal value.
For instance, consider the following code:
#include <iostream>
int main() {
int num = 255;
std::cout << "Decimal: " << num << "\n";
std::cout << "Hexadecimal: " << std::hex << num << "\n";
return 0;
}
In this example, the output would be:
Decimal: 255
Hexadecimal: ff
Resetting the Output Format
After printing a number in hexadecimal, you may want to reset the output format back to decimal. This can be done using the `std::dec` manipulator. Here’s how it works:
std::cout << std::dec << num; // Resetting to decimal
Using `std::dec` ensures that any following numbers are printed back in their standard decimal format.

Customizing Hexadecimal Output
Specifying Number Width
When printing hexadecimal values, you may want to ensure a consistent width or padding for alignment purposes. You can achieve this with `std::setw` from the `<iomanip>` header:
#include <iomanip>
int main() {
int num = 30;
std::cout << "Hexadecimal (padded): "
<< std::setw(4) << std::setfill('0') << std::hex << num << "\n";
return 0;
}
In the output, even if the hexadecimal equivalent of 30 is "1e", it would display as "001e" due to the specified width and padding.
Uppercase Hexadecimal
To print the letters in uppercase (A-F) rather than lowercase (a-f), use the `std::uppercase` manipulator. Here’s a simple illustration:
#include <iostream>
#include <iomanip>
int main() {
int num = 255;
std::cout << "Uppercase Hexadecimal: " << std::uppercase << std::hex << num << "\n";
return 0;
}
The output of this code will be "FF" instead of "ff".

Practical Examples
Printing Hexadecimal Values of Various Data Types
C++ allows for printing hexadecimal values from different data types seamlessly. Here’s an example that demonstrates this:
int a = 30;
float b = 20.5f;
char c = 'A';
std::cout << "Hex of int: " << std::hex << a << "\n";
std::cout << "Hex of float: " << std::hexfloat << b << "\n"; // (Note this is for specialized output)
std::cout << "Hex of char: " << std::hex << static_cast<int>(c) << "\n"; // Cast char to int
In this snippet, float output in hexadecimal form requires specific use of manipulators, while the `char` must be cast to an integer to display its numeric representation.
Reading Hexadecimal Input from User
C++ also allows users to enter hexadecimal numbers through standard input. By setting the stream to `std::hex`, you can read and process input in hexadecimal form effortlessly. For example:
int userInput;
std::cout << "Enter a number in hex: ";
std::cin >> std::hex >> userInput;
std::cout << "You entered: " << std::dec << userInput << "\n"; // Display in decimal
In this instance, if a user inputs "A5", the output would reflect the user-entered value converted to decimal.

Common Mistakes and Troubleshooting
Common Errors When Printing Hexadecimal
New programmers may encounter pitfalls while working with hexadecimal output in C++. A common error is misunderstanding how stream manipulators work. Failing to reset the format after a `.hex` output can lead to confusion in subsequent outputs.
Debugging Output Issues
When you find that your hexadecimal output is incorrect or not displaying properly, consider checking:
- Whether you have applied `std::hex`, `std::dec`, or any other relevant manipulators appropriately.
- Ensure you have included necessary headers like `<iomanip>` for advanced formatting options.
- Confirm the type of the variable you are trying to print; casting may be needed with types like `char` or when handling floating-point numbers.

Conclusion
Printing hexadecimal values in C++ using `cout` not only enhances your programming skills but also equips you with essential knowledge for performing advanced data manipulation. By understanding hexadecimal representation, utilizing format manipulators, and avoiding common pitfalls, you can efficiently handle various programming scenarios.
Embark on experimenting with these concepts to solidify your understanding and leverage the power of hexadecimal representation in your C++ projects!
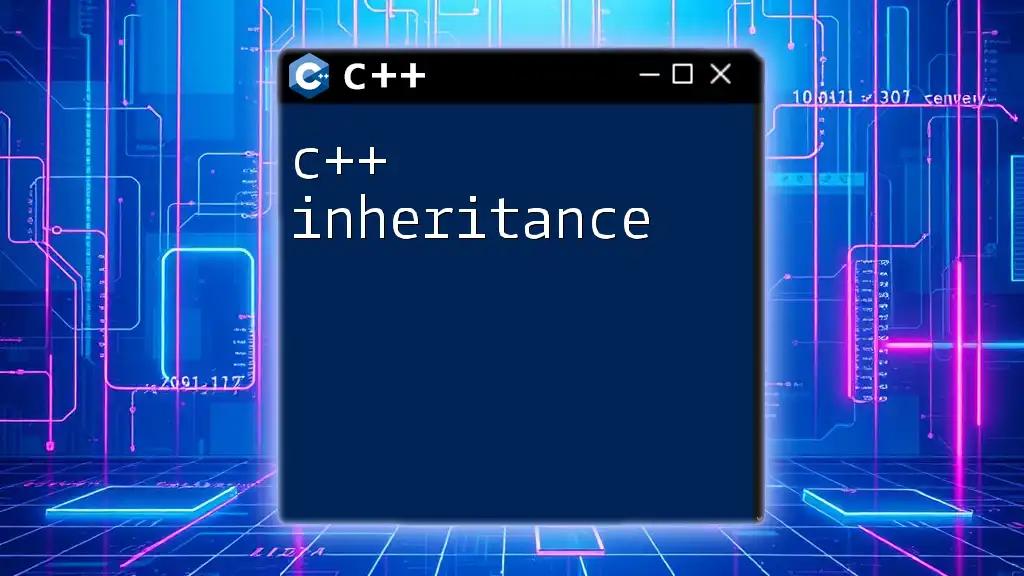
Additional Resources
For further exploration of C++ and mastering advanced output techniques, refer to the official C++ documentation, or consider recommended books and online tutorials for comprehensive learning materials.