In C++, you can use the `printf` function to output boolean values by converting them to integers, where `true` is displayed as `1` and `false` as `0`. Here's an example:
#include <cstdio>
int main() {
bool myBool = true;
printf("The value of myBool is: %d\n", myBool);
return 0;
}
Understanding Boolean in C++
Boolean values in C++ represent two states: `true` and `false`. These are fundamental in programming, influencing control flow (such as loops and conditionals), function return types, and logical operations. In C++, the `bool` data type is specifically used to hold these boolean values, which are internally represented as integers: 1 for `true` and 0 for `false`.
Boolean Data Types in C++
The built-in `bool` data type in C++ is capable of storing one of the two boolean values: `true` or `false`. Each boolean value consumes one byte of memory, which allows for efficient representation and manipulation in various programming contexts.
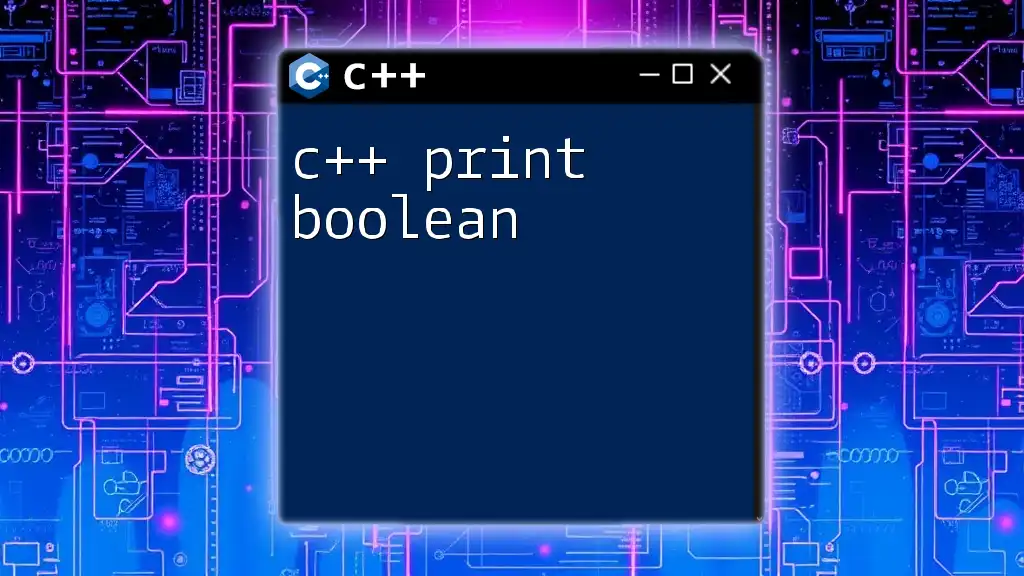
The C++ printf Function
Introduction to printf
The `printf` function is a standard library function in C and C++ that allows formatted output to be sent to the standard output, typically the console. It provides more control over output formatting than the C++ output streams (`cout`). While `printf` is influenced by C conventions, its flexibility in formatting strings and numbers makes it a practical choice for many C++ programmers, especially when dealing with legacy code or system-level programming.
Format Specifiers in printf
With `printf`, a variety of format specifiers can be used:
- `%d` for integers
- `%f` for floating-point numbers
- `%s` for strings
When it comes to printing boolean values, you will primarily be using the `%d` format specifier, since C++ does not have a dedicated format specifier for booleans.
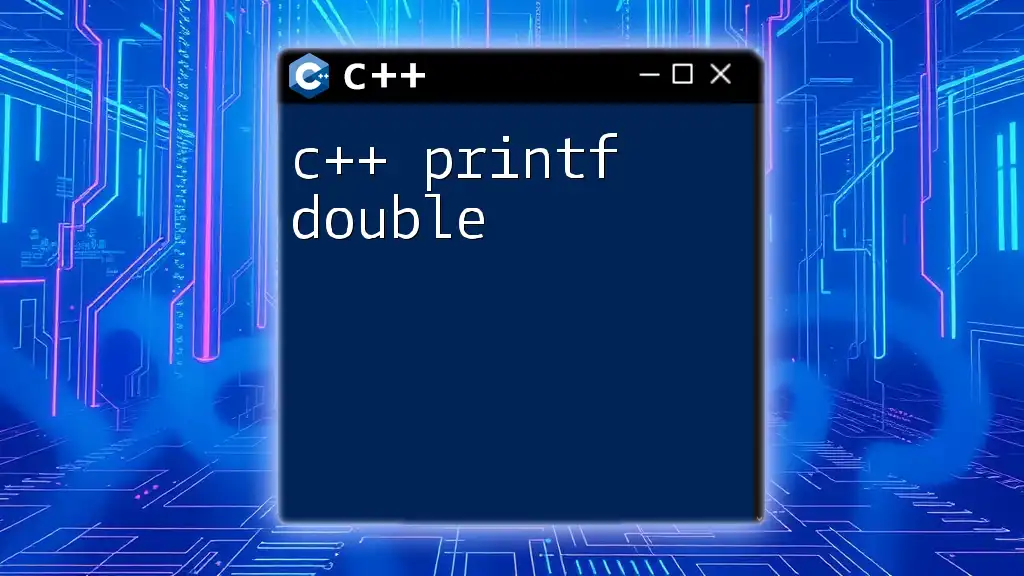
Printing Boolean Values with printf
Understanding Boolean to Integer Conversion
In C++, boolean values convert to integers surprisingly easily. When using `printf`, the boolean value `true` is converted to 1 and `false` to 0. This behavior is foundational and allows developers to smoothly integrate boolean checks within formatted print statements.
Consider the following example:
bool flagTrue = true;
bool flagFalse = false;
printf("flagTrue: %d\n", flagTrue); // Outputs: flagTrue: 1
printf("flagFalse: %d\n", flagFalse); // Outputs: flagFalse: 0
Here, `flagTrue` outputs 1, while `flagFalse` outputs 0, illustrating the direct relationship between boolean and integer values.
Printf with Booleans Directly
When working with `printf`, you can express boolean values directly by utilizing the `%d` format. This is a straightforward way to output the status of boolean flags. For instance:
#include <cstdio>
int main() {
bool isAvailable = true;
printf("Is Available: %d\n", isAvailable); // Outputs: Is Available: 1
return 0;
}
In this code, `isAvailable` being `true` translates to an output of 1.
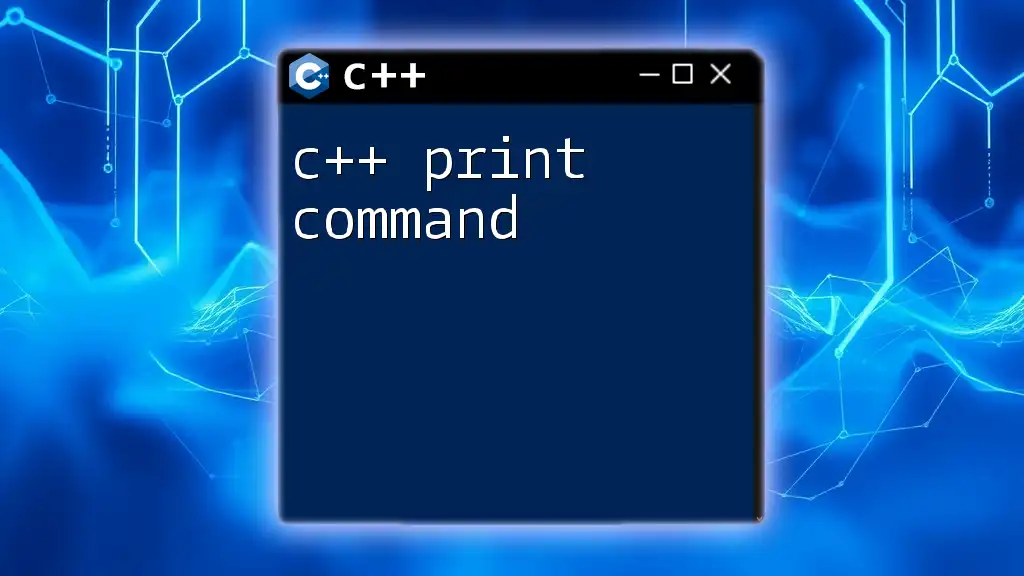
Advanced Boolean Output Formatting
Customizing Boolean Output
For more user-friendly output, you can implement conditional expressions to provide more meaningful messages, enhancing the readability of your program's output. The following example demonstrates how to achieve this:
bool isOnline = false;
printf("User is %s\n", isOnline ? "Online" : "Offline"); // Outputs: User is Offline
In this code snippet, we use a ternary operator to determine the output string based on the boolean value. This approach clarifies the meaning of the output rather than just displaying 1 or 0.
Using std::boolalpha with C++
To print boolean values in a more understandable format using C++, you can utilize `std::boolalpha` in conjunction with output streams like `cout`. While `printf` does not support `std::boolalpha`, this feature offers a straightforward way to print boolean values as `true` or `false`. Consider the following example:
#include <iostream>
#include <cstdio>
int main() {
bool isActive = true;
printf("Is Active: %d\n", isActive); // Outputs: Is Active: 1
// Using cout
std::cout << std::boolalpha;
std::cout << "Is Active: " << isActive << std::endl; // Outputs: Is Active: true
return 0;
}
In this example, we first use `printf` to output the integer representation of the boolean, and then we switch to `cout` to display it as a more readable true or false.
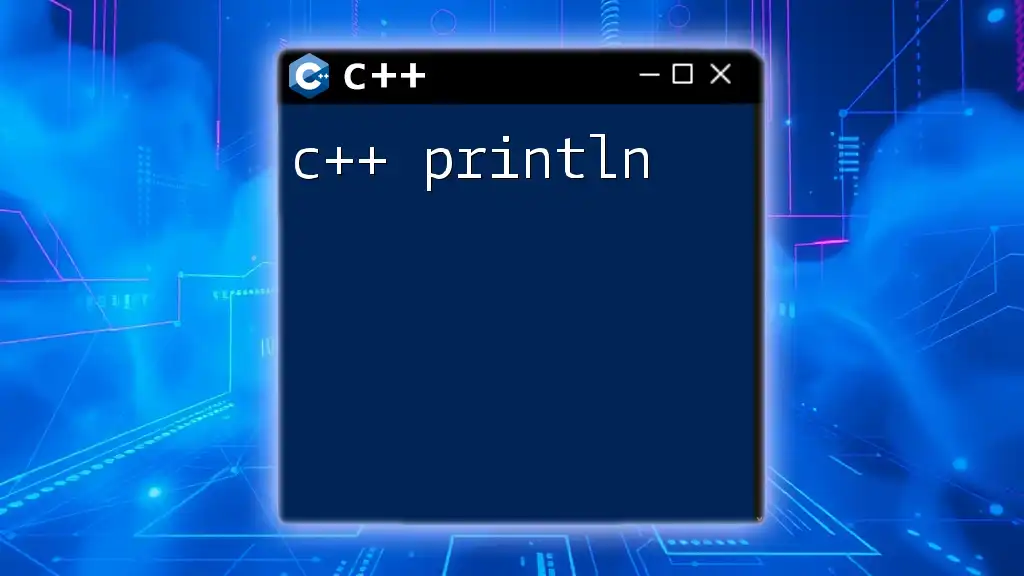
Best Practices When Using printf with Booleans
When to Use printf vs cout
Choosing between `printf` and `cout` often depends on the context of your program. Use `printf` for scenarios where precise formatting and legacy support are required. On the other hand, C++ streams (`cout`) are often preferred in modern C++ code for their type safety, easier syntax, and native support for boolean representation.
Avoiding Common Pitfalls
When utilizing `printf` with boolean values, one common pitfall is the improper use of format specifiers. Ensure that you always use `%d` for boolean values; incorrectly using `%s` or other specifiers might lead to unexpected behaviors or runtime errors.
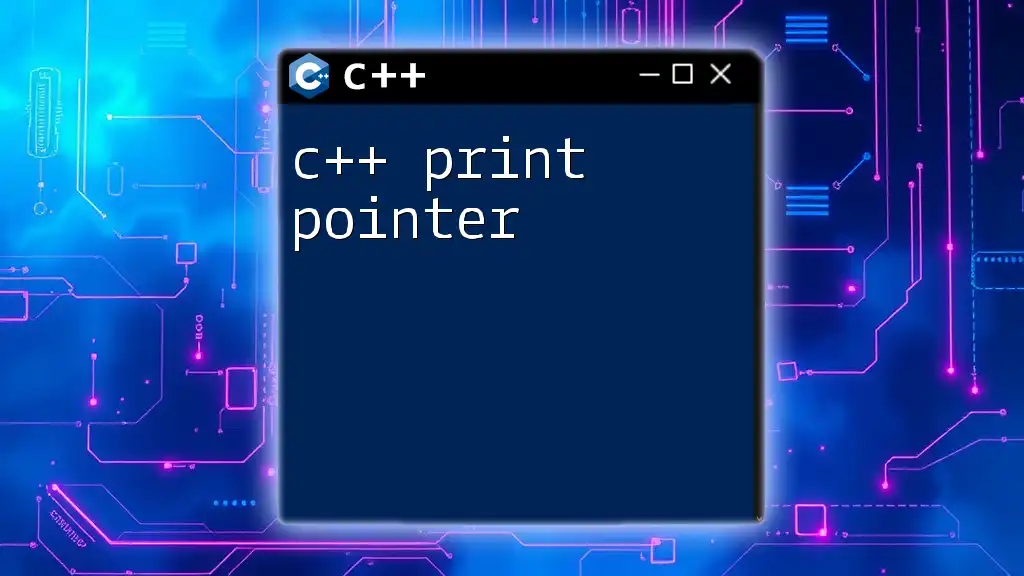
Conclusion
Understanding how to use `printf` with boolean values is essential for effective C++ programming. This knowledge not only enhances debugging and output readability but also provides a solid foundation for working with control flows and conditional operations. By mastering `printf` and its boolean output capabilities, programmers equip themselves with a versatile tool for crafting clear and informative console applications.
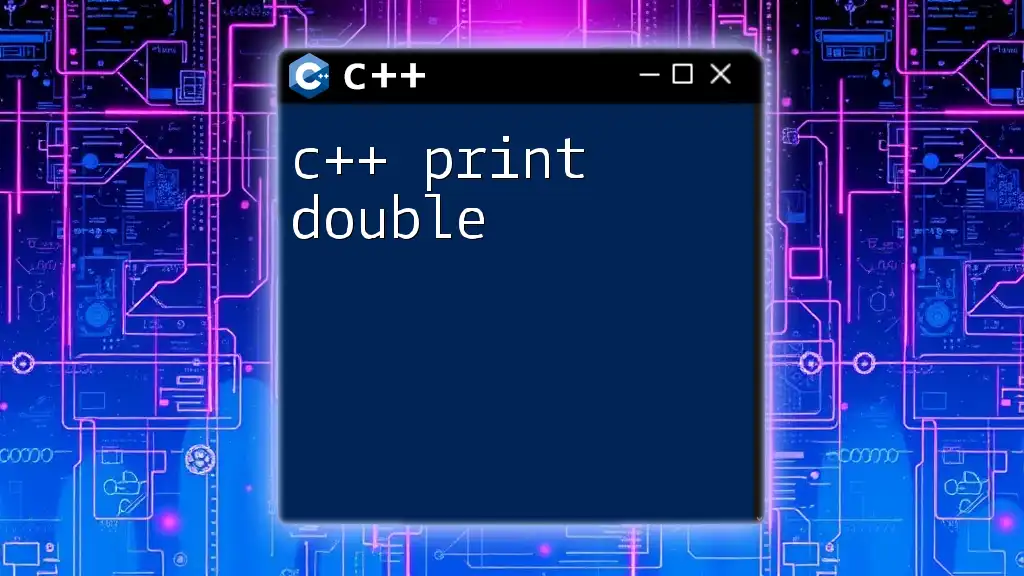
Additional Resources
To deepen your understanding of `printf`, boolean types, and formatting in C++, consider exploring additional C++ documentation, online coding tutorials, and resources that focus on advanced C++ programming techniques. Familiarizing yourself with these materials can further empower you to write efficient and expressive C++ code.