In C++, you can print a double value using the `cout` stream from the `iostream` library by including the necessary header and ensuring to set the precision if needed.
Here’s an example code snippet:
#include <iostream>
#include <iomanip>
int main() {
double value = 3.14159;
std::cout << std::fixed << std::setprecision(2) << value << std::endl;
return 0;
}
Understanding Double Data Type in C++
What is a Double?
The double data type in C++ is a fundamental type that is used to represent numbers with decimal points. It provides a greater precision compared to the float data type, making it ideal for calculations that require fractional values. Specifically, a double typically uses 64 bits of memory, allowing it to store approximately 15 decimal digits.
Characteristics of Double
One of the key characteristics of double values is their precision. They can represent very large or small numbers, which is especially useful in scientific calculations. When comparing doubles to other types like `float` and `int`, it’s important to remember the following:
- Float: Typically allocates 32 bits of memory, offering less precision (around 7 decimal digits).
- Int: Used for whole numbers only, without any decimal place.
The choice between these data types depends on the specific requirements for numerical precision in your program.
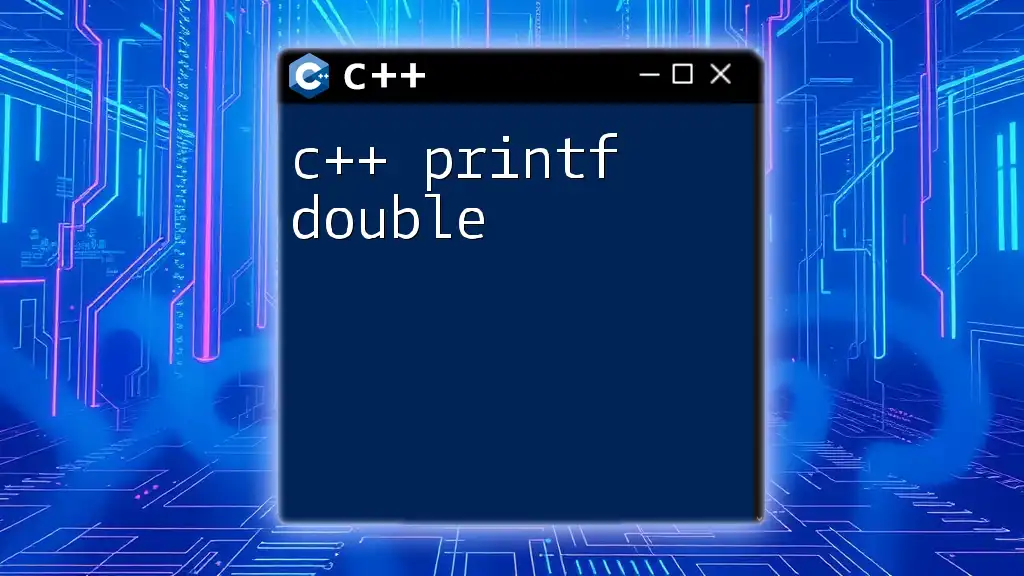
Setting Up Your C++ Environment
Required Tools
To start programming in C++, you'll need a development environment. Recommended tools include:
- Integrated Development Environments (IDEs): Such as Visual Studio, Code::Blocks, or CLion.
- Compilers: GCC (GNU Compiler Collection) or MSVC (Microsoft Visual C++).
Writing Your First C++ Program
Here’s a simple template for a C++ program that you can use as a foundation:
#include <iostream>
using namespace std;
int main() {
// Your code goes here
return 0;
}
You can neatly insert your operations within the `main` function.
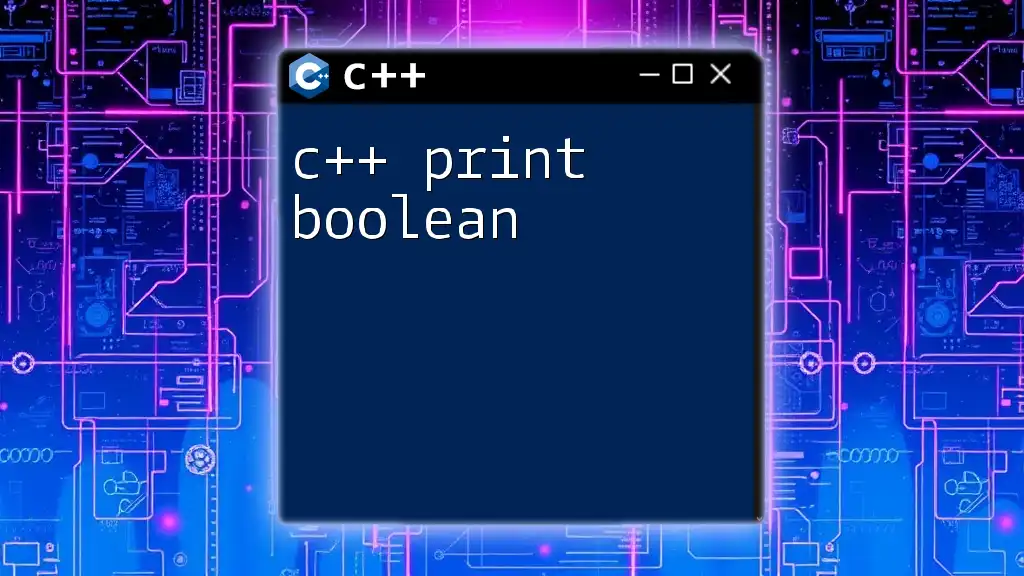
Printing Double in C++
Basic Syntax for C++ Output
In C++, the `cout` statement is used to print output to the console. Its basic syntax is as follows:
cout << "Your message";
This operator (`<<`) directs the output to the standard output stream.
Printing Double Values
To print a double value using `cout`, you simply include the double variable in the statement. Here’s a straightforward example:
#include <iostream>
using namespace std;
int main() {
double value = 10.5;
cout << "The value of the double is: " << value << endl;
return 0;
}
In this example, the program initializes a double variable `value` and prints it with a message. The `endl` is used to insert a new line.
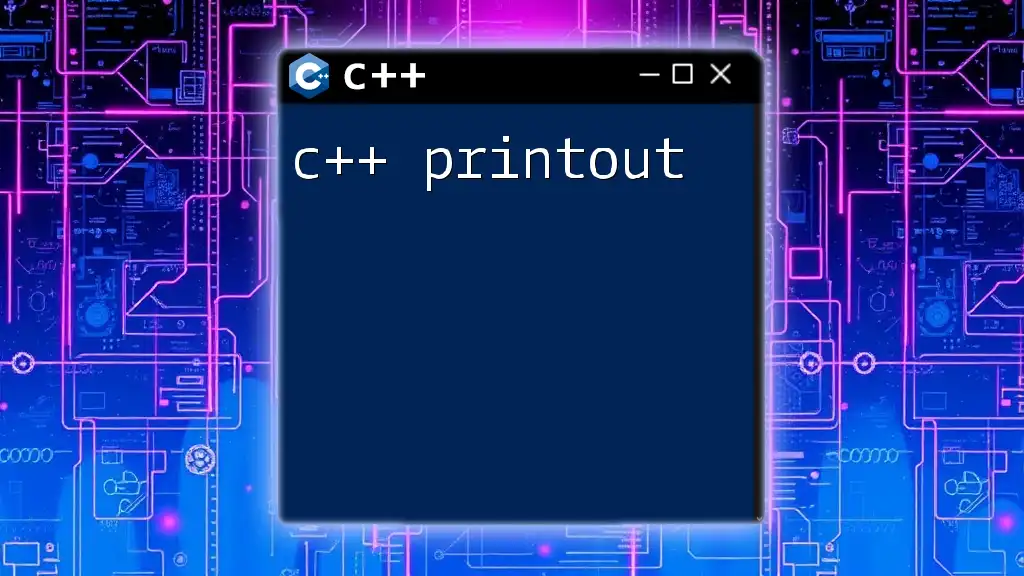
Formatting Output for Double Values
Default Formatting
By default, C++ outputs double values in a format that may not always meet the specific precision needs of your program. Thus, you may want to customize the output format.
Using `setprecision` for Specific Precision
To control the number of decimal places displayed, you can use the `setprecision` manipulator included in the `iomanip` header. Here's how it works:
#include <iostream>
#include <iomanip> // Required for setprecision
using namespace std;
int main() {
double value = 10.123456;
cout << fixed; // Use fixed-point notation
cout << "Value with default precision: " << value << endl;
cout << "Value with two decimal precision: " << setprecision(2) << value << endl;
return 0;
}
In this code, setting `fixed` modifies the output format to fixed-point notation, while `setprecision(2)` displays the value with two decimal points.
Formatting with Fixed and Scientific Notation
It’s essential to decide when to use fixed or scientific notation for double values. Use fixed notation for everyday decimal numbers and scientific notation for very large or very small numbers.
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
double largeNumber = 123456789.0;
double smallNumber = 0.000000123456;
cout << fixed << largeNumber << endl; // Outputs in fixed-point format
cout << scientific << smallNumber << endl; // Outputs in scientific format
return 0;
}
This demonstrates how C++ handles output for large and small numbers differently, enhancing the readability of your results.
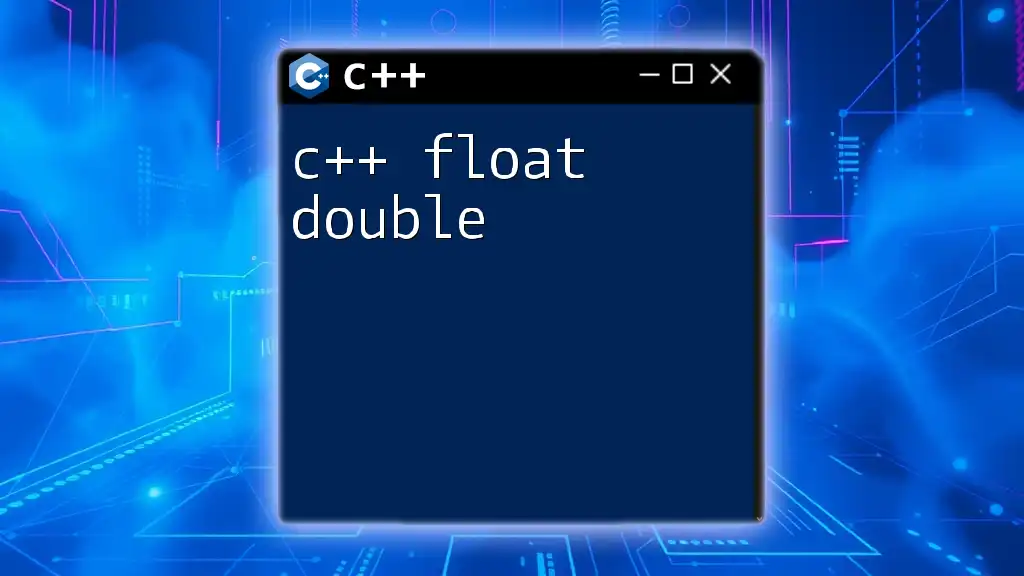
Handling Special Cases
Printing Negative and Positive Doubles
C++ naturally recognizes positive and negative double values, and it prints them accordingly. For instance:
double positiveValue = 5.0;
double negativeValue = -5.0;
cout << "Positive value: " << positiveValue << endl;
cout << "Negative value: " << negativeValue << endl;
Edge Cases: NaN and Infinity
In C++, special values like NaN (Not a Number) and Infinity are handled efficiently. They can arise in certain calculations, such as divisions by zero. Here’s how to display these values:
#include <iostream>
#include <cmath> // For NaN and Infinity
using namespace std;
int main() {
double NaNValue = NAN;
double InfinityValue = INFINITY;
cout << "NaN Value: " << NaNValue << endl; // Outputs NaN
cout << "Infinity Value: " << InfinityValue << endl; // Outputs Infinity
return 0;
}
This example demonstrates how you can print these special cases, allowing you to handle edge cases effectively in your programs.
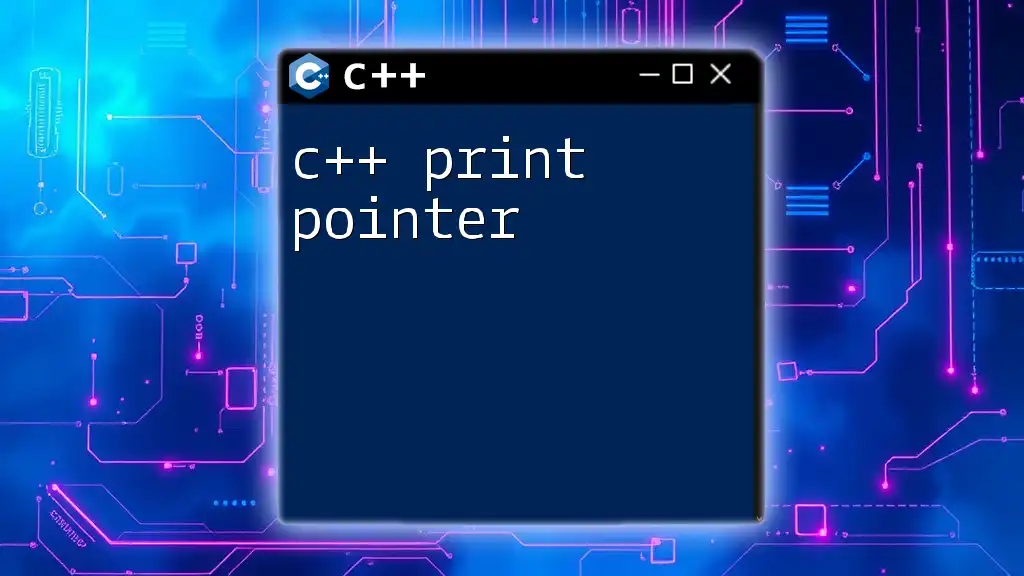
Conclusion
Throughout this guide, we explored c++ print double commands, focusing on various methods for outputting double values with proper formatting. Understanding how to manipulate double output ensures clarity and precision in your programming endeavors. With these techniques at your disposal, you can enhance your skills in C++ programming. Happy coding!
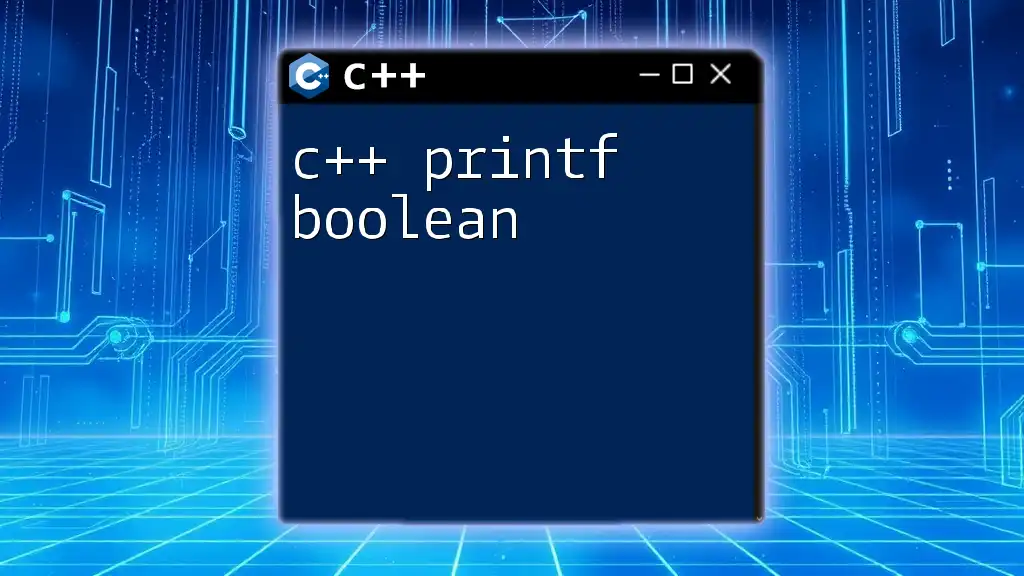
Supplementary Resources
For further learning, consider checking out recommended programming books and online courses, or engage with community forums where you can discuss C++ programming topics and gain additional insights.