In C++, you can print content to a file using the `ofstream` class to create a file stream. Here's a code snippet demonstrating how to do this:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("output.txt");
outFile << "Hello, World!" << std::endl;
outFile.close();
return 0;
}
Understanding File Streams
What are File Streams?
In C++, file streams are abstractions that enable the program to read from and write to files. They provide a interface for I/O operations on files, allowing developers to manipulate files just as they would with standard input and output (like the console). The three primary types of file streams in C++ are:
- ifstream: Used for reading data from files.
- ofstream: Used for writing data to files.
- fstream: Combines both input and output operations in a single stream.
The fstream Library
To work with file streams in C++, you need to include the `<fstream>` header in your program. This library provides the necessary conventions and functionalities for file handling in C++. You can include it as follows:
#include <fstream>

Opening a File for Writing
Choosing a File Mode
When opening a file for writing, you must specify the appropriate file mode. The most common modes include:
- ios::out: Opens the file for output. If the file already exists, it is overwritten unless another mode is specified.
- ios::app: Opens the file in append mode. Data will be added to the end of the file without overwriting existing content.
Syntax for Opening a File
To open a file for writing, you can declare an `ofstream` object and use the following syntax:
std::ofstream outFile("example.txt", std::ios::out);
In this example, `"example.txt"` is the name of the file you want to create or open, and `std::ios::out` specifies that you intend to write to this file.
Handling File Open Errors
It’s crucial to handle potential errors when opening a file. Failing to do so can result in unexpected behaviors. You can check if the file was opened successfully with a simple conditional statement:
if (!outFile) {
std::cerr << "Error opening file!" << std::endl;
}
This snippet will print an error message to the console if the file fails to open.

Writing Data to Files
Basic Writing Techniques
Writing data to a file can be accomplished using the `<<` operator similar to how you write to the console. For instance, to write a simple string, you can use:
outFile << "Hello World" << std::endl;
The `std::endl` here not only adds a newline but also flushes the output buffer, ensuring that all data is written to the file.
Writing Different Data Types
Writing Strings
You can easily write strings to a file as follows:
std::string name = "John Doe";
outFile << name << std::endl;
This code snippet demonstrates how to output a string variable to the file.
Writing Numbers
To write numbers, simply pass numerical values in a similar manner:
int age = 30;
outFile << "Age: " << age << std::endl;
This will effectively write the age alongside a label for clarity.
Formatting Output
Effective formatting is essential for creating readable files. For numerical data, C++ provides functions like `std::setw()` to set the width and `std::setprecision()` to control the number of digits after the decimal point:
#include <iomanip>
outFile << std::setw(10) << std::setprecision(2) << std::fixed << price << std::endl;
This example will format `price` to display two decimal points with a total width of ten characters.

Closing the File
Why It's Important to Close Files
It is essential to close files after you finish writing to them. This action ensures that all buffered data is flushed to the file, preventing data loss and freeing up system resources. Always use:
outFile.close();
to close the file.
Checking if a File is Open
Before closing the file, it's a good practice to check whether it is open. This can help you avoid potential errors in your program:
if (outFile.is_open()) {
outFile.close();
}
This simple check guarantees that you are only attempting to close an active file.
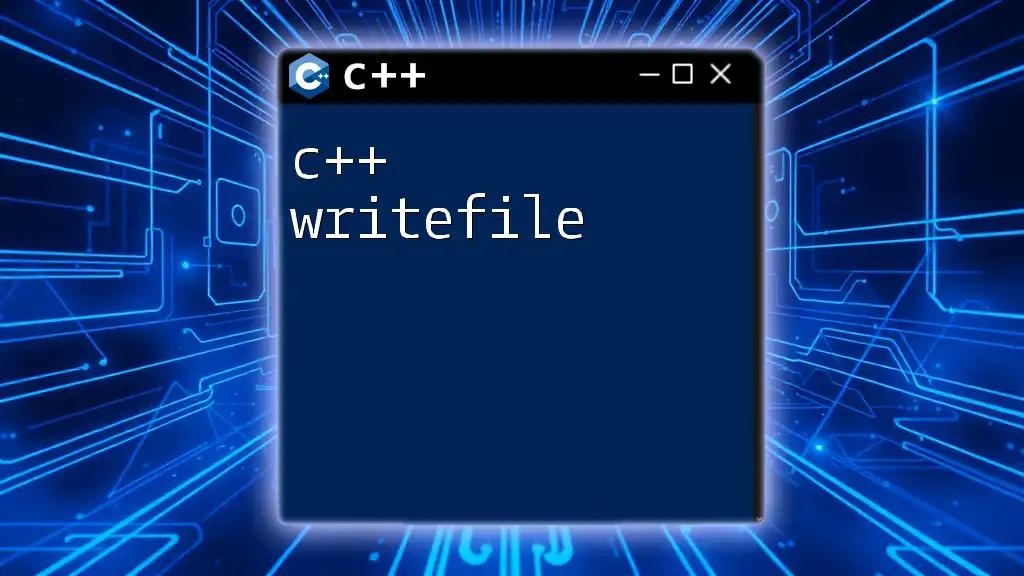
Advanced Writing Techniques
Writing Arrays and Vectors
C++ also allows you to write collections like arrays and vectors to a file. Here’s an example of how to write a vector of integers:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
outFile << num << " ";
}
This loop outputs each number in the vector, separated by spaces.
Serializing Objects to a File
Serialization is crucial when you want to write complex data types or objects to a file. You can implement a custom function to write class members to a file, allowing for easy reconstitution of the object later on.
For example, consider a class `Person`:
class Person {
public:
std::string name;
int age;
void serialize(std::ofstream& outFile) {
outFile << name << std::endl;
outFile << age << std::endl;
}
};
// When writing an object:
Person p;
p.name = "Jane Doe";
p.age = 25;
p.serialize(outFile);
This method allows you to systematically write object data to a file.
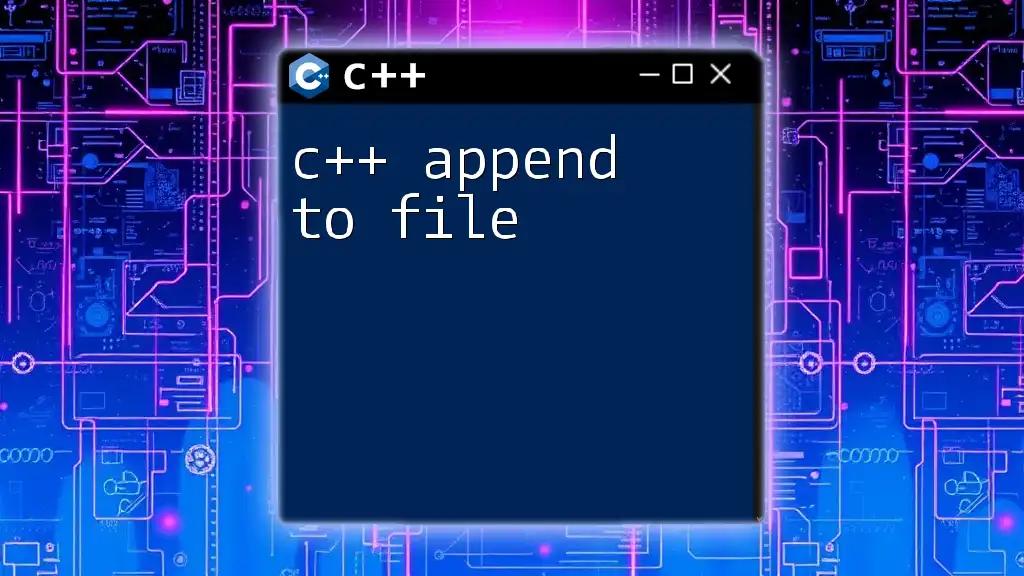
Common Errors and Debugging Tips
Not Opening the File
One common mistake is neglecting to open the file before writing to it. Always ensure you have opened the file correctly and that it’s in the expected mode.
Incorrect File Paths
Another frequent issue arises from incorrect file paths—ensure you're using the right path and permissions. Different operating systems handle paths differently, so be mindful when specifying them.

Conclusion
By mastering the techniques outlined above, you can effectively print data to files in C++. From understanding the fundamental principles of file streams to handling more complex data structures, this guide equips you with essential knowledge to manage file I/O competently. As you advance in your coding journey, practice these concepts with real-world examples to solidify your understanding.

Additional Resources
For further exploration, consider visiting online C++ documentation and joining programming communities where you can seek assistance and share knowledge. Engaging with others can significantly enhance your learning experience.