In C++, to print the value of a pointer (which represents a memory address), you can use the `cout` stream along with the dereference operator `*` to access the value being pointed to.
#include <iostream>
int main() {
int value = 42;
int* ptr = &value;
std::cout << "Value pointed to by ptr: " << *ptr << std::endl; // Prints: Value pointed to by ptr: 42
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. This reference to a memory location allows for dynamic memory allocation, management of arrays and data structures, and the powerful ability to directly manipulate the contents of a memory location. To declare a pointer, you use the asterisk (*) symbol alongside the variable’s type. For instance:
int* ptr; // Declares a pointer to an integer
How Pointers Work
Pointers are crucial in C++ as they facilitate efficient memory management. Each variable is allocated a certain amount of memory based on its type, and pointers enable programmers to directly access and manipulate that memory. Unlike regular variables, pointers embrace the concept of memory addresses, providing control over both storage and retrieval of data.
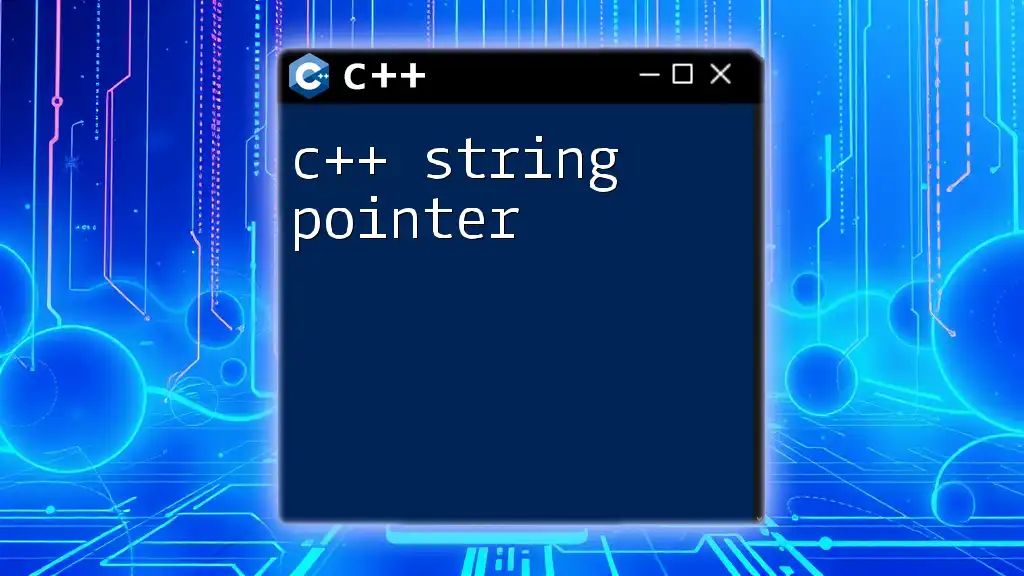
The Basics of Printing Pointers
Using `cout` to Print Pointers
In C++, the `cout` object from the iostream library is commonly used for outputting data to the console. To print the address contained in a pointer, simply use the pointer variable directly with `cout`.
int var = 5;
int* ptr = &var;
std::cout << ptr; // Prints the memory address of var
The output will be the hexadecimal memory address where `var` is stored.
Format Specifiers for Pointer Types
When printing pointers, format specifiers can modify how the addresses are displayed. You can utilize `std::hex`, `std::dec`, and `std::oct` to print the address in different base systems.
std::cout << std::hex << ptr; // Prints pointer in hexadecimal format
std::cout << std::dec << ptr; // Prints pointer in decimal format
std::cout << std::oct << ptr; // Prints pointer in octal format
These format specifiers enhance readability and usability, especially during debugging.
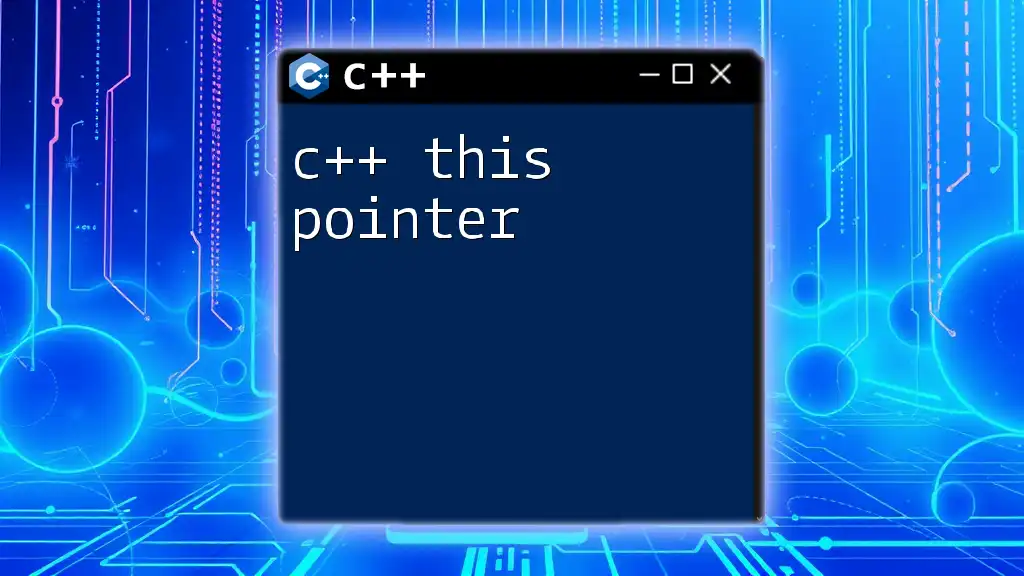
Advanced Techniques for Printing Pointers
Printing Pointer Values Meaningfully
To get the value a pointer points to, you can dereference the pointer using the asterisk (*) operator. This yields the actual value stored at that memory address.
std::cout << *ptr; // Prints the value stored at the address in ptr
This line will output `5`, which is the value stored in the variable `var`.
Printing Pointers in Arrays
Pointers can also be very useful when working with arrays. By leveraging pointer arithmetic, you can easily traverse and print the contents of an array.
int arr[] = {1, 2, 3, 4};
int* ptr = arr; // Points to the first element of the array
for (int i = 0; i < 4; i++) {
std::cout << *(ptr + i) << " "; // Prints each element of the array
}
In this example, the output will be `1 2 3 4`. The technique showcases how pointers can simplify operations on arrays.
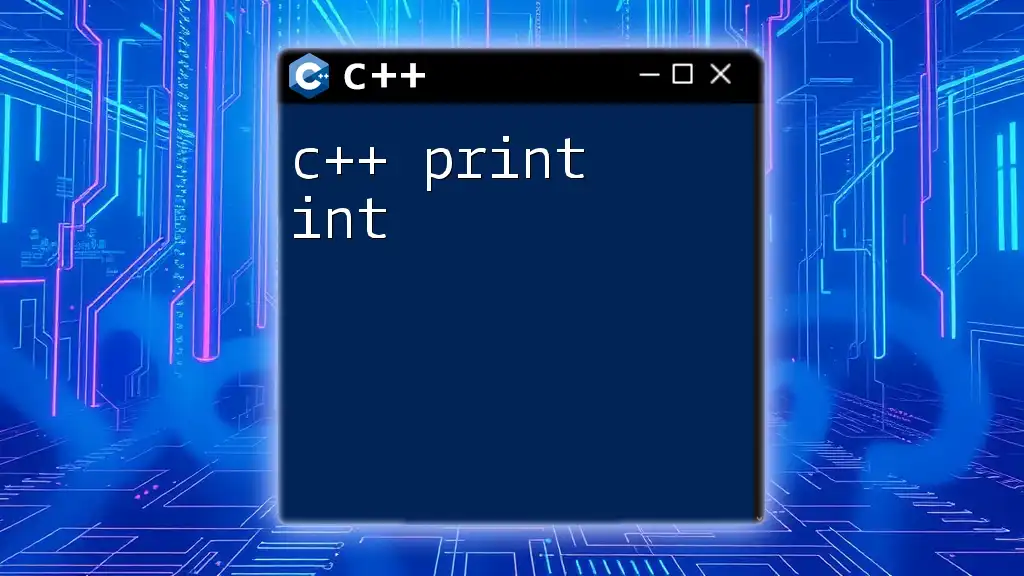
Common Mistakes When Printing Pointers
Dereferencing Null or Uninitialized Pointers
One of the most common pitfalls in pointer usage is dereferencing null or uninitialized pointers. Accessing memory that hasn’t been allocated can lead to crashes and unpredictable behavior in your program.
int* nullPtr = nullptr;
// std::cout << *nullPtr; // This is unsafe!
Before dereferencing a pointer, it’s crucial to ensure it points to valid memory. Always check for null values to prevent runtime errors.
Misinterpretation of Output
It's essential to understand what pointer addresses represent. The output of printing a pointer is simply the address in memory, which means different runs of the program may yield differing results.
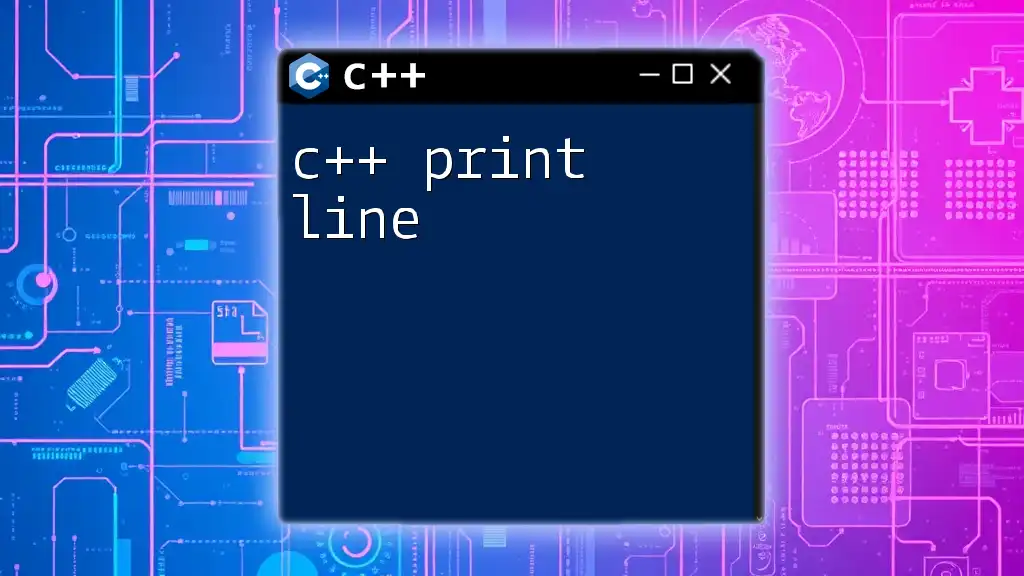
Debugging Tips for Pointer Printing
Using `std::cout` vs. Other Debugging Tools
While `cout` is straightforward and beneficial for quick debugging, more complex issues may require you to use dedicated debugging tools such as GDB. These tools provide in-depth insights into memory usage and pointer states.
Understanding Compiler Warnings and Errors
Compilers often provide warnings related to the mismanagement of pointers. These warnings serve as an invaluable resource for optimizing your code. Pay close attention to them, and ensure proper pointer initialization and management to avert issues down the line.
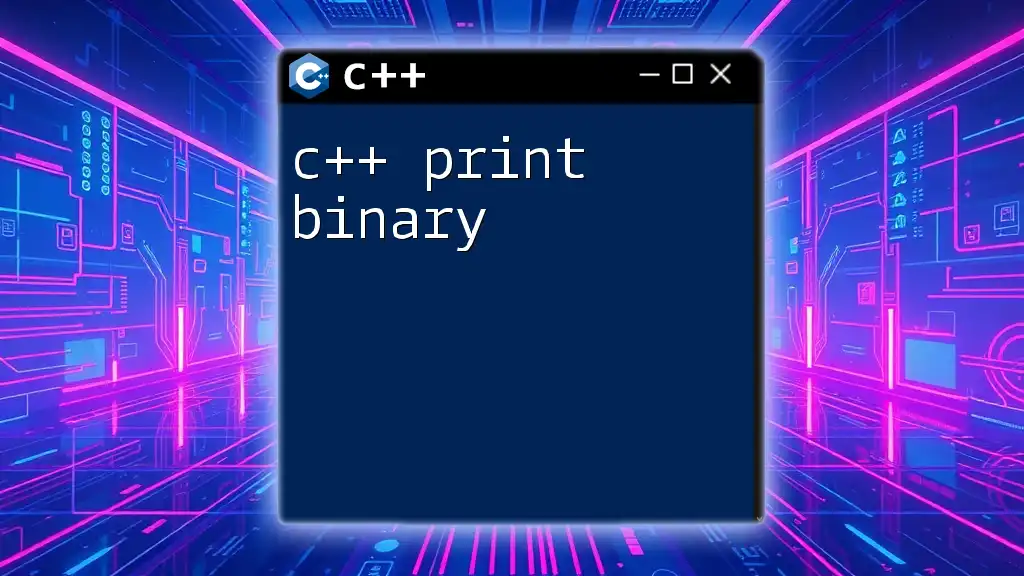
Conclusion
Understanding how to effectively c++ print pointer is vital for both novice and seasoned programmers. Pointers constitute a powerful element within C++, enabling complex programming maneuvers when wielded with care. By mastering the processes associated with pointer declaration, manipulation, and output through various methods, programmers can refine their coding practices. Engaging further with pointers through more advanced techniques will only enrich your programming repertoire.
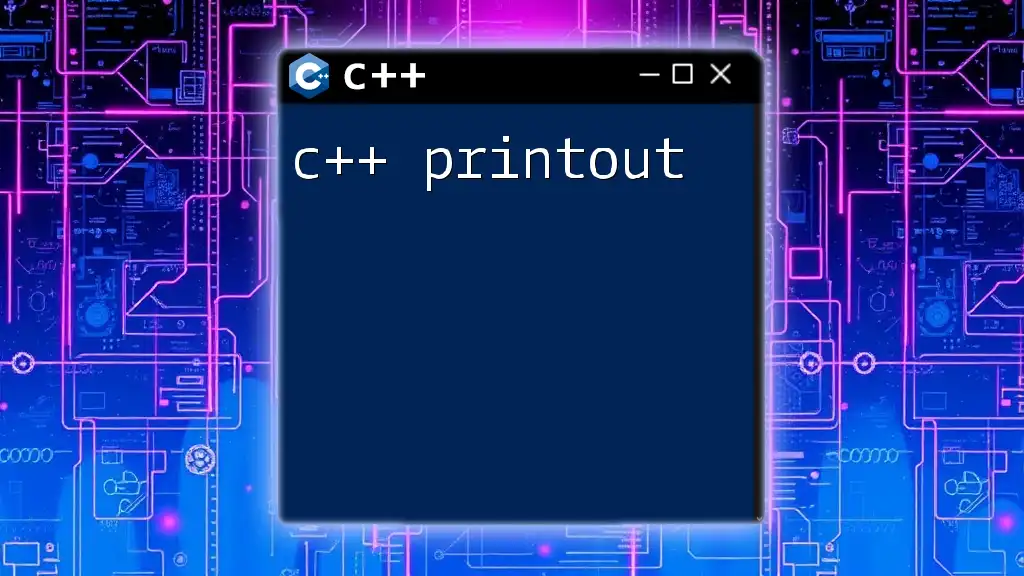
Additional Resources
For more detailed understanding and exploration of pointers in C++, refer to the [official C++ documentation](https://en.cppreference.com/w/cpp/language/pointer) or consider delving into specialized books and online courses dedicated to C++ programming.