In C++, you can print a boolean value using the `std::cout` stream, which outputs `true` or `false` based on the boolean variable's value. Here's a code snippet demonstrating this:
#include <iostream>
int main() {
bool isTrue = true;
std::cout << std::boolalpha << isTrue << std::endl; // Outputs: true
return 0;
}
Understanding Boolean Values in C++
The Boolean Data Type
In C++, the boolean data type is used to represent truth values. A boolean can only store one of two possible states: `true` or `false`. This is defined using the keyword `bool`.
Syntax Example:
bool isReady = true;
Here, `isReady` is a boolean variable initialized to `true`.
Common Boolean Values
The essence of boolean logic revolves around its two values: `true` and `false`. In programming, these values serve as the foundation for decision-making and control flow.
For example:
bool isFinished = false;
if (isFinished) {
// do something
}
In this instance, the `if` condition will not execute because `isFinished` is `false`.
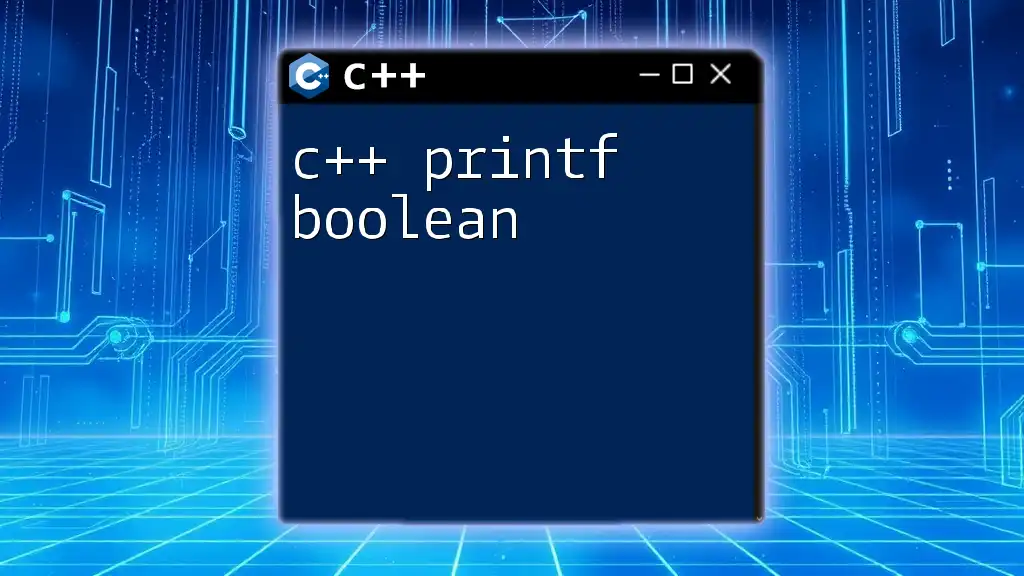
Printing Boolean Values in C++
Using `std::cout` to Print Booleans
To display boolean values in C++, you can utilize `std::cout` from the `<iostream>` library. The default behavior of `std::cout` represents `true` as `1` and `false` as `0`.
Consider the following example:
#include <iostream>
using namespace std;
int main() {
bool myBool = true;
cout << myBool; // Outputs: 1
return 0;
}
The line `cout << myBool;` prints `1` to the console since `myBool` is `true`.
The Output of Boolean Values
When printing boolean variables, it’s vital to understand how these values are portrayed. The number `1` signifies `true`, while `0` denotes `false`.
Here's how to demonstrate this:
bool flag1 = true;
bool flag2 = false;
cout << "Flag1 is: " << flag1 << endl; // Prints: Flag1 is: 1
cout << "Flag2 is: " << flag2 << endl; // Prints: Flag2 is: 0
As shown, `flag1` results in `1` and `flag2` results in `0` when printed.
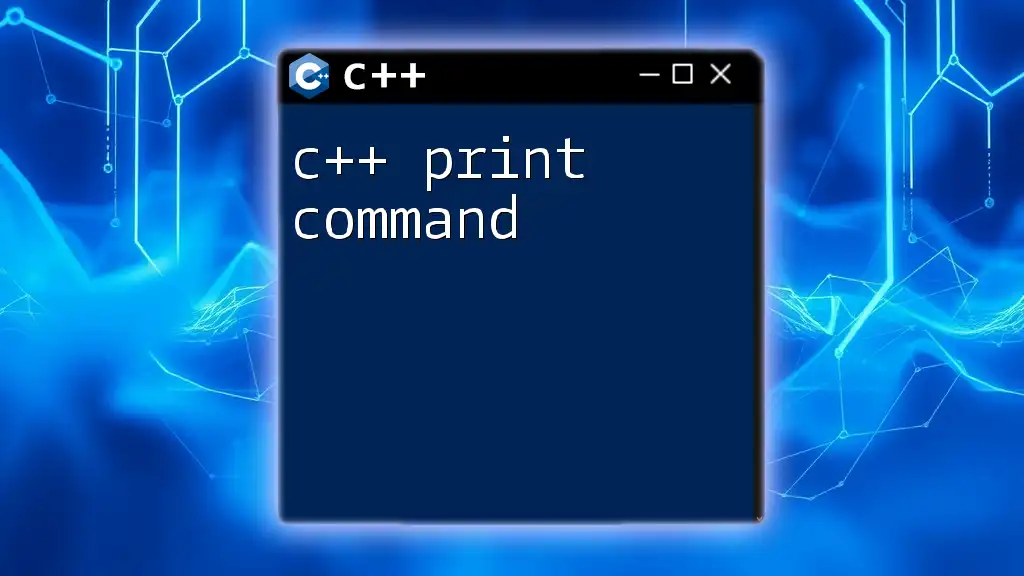
Formatting Boolean Output
Customizing Boolean Print Representation
Although the default output may serve basic needs, it often lacks clarity. To enhance readability, you can create custom functions to display boolean values more meaningfully.
Here’s an illustrative example:
void printBool(bool value) {
cout << (value ? "True" : "False") << endl;
}
This function allows us to output "True" or "False" instead of `1` or `0`.
Example Usage of Custom Print Function
Implementing the custom function enhances the output representation significantly:
int main() {
bool testValue = false;
printBool(testValue); // Outputs: False
return 0;
}
When calling `printBool(testValue);`, the console outputs "False", providing greater clarity than the numerical representation.
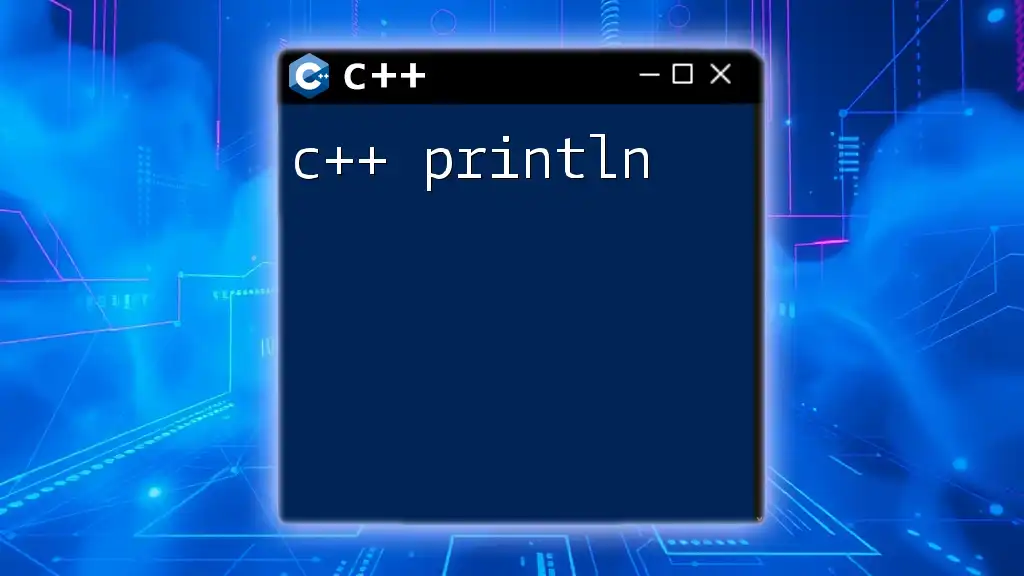
When and Why to Print Boolean Values
Debugging and Tracking Logic Flow
Printing boolean values is incredibly valuable for debugging. During program execution, you can track variable states to verify that your logic is working as intended.
Consider a situation where we check a condition:
bool isAvailable = true;
if (isAvailable) {
cout << "Item is available." << endl;
} else {
cout << "Item is not available." << endl;
}
In this scenario, if `isAvailable` is `true`, the output will confirm that the item is available, aiding in just-in-time debugging.
Practical Applications in Real-World Programs
Boolean values play a critical role in various real-world applications. They often dictate the flow of control statements, guiding the program to make decisions based on different conditions.
For instance:
bool isOnline = false;
bool isRegistered = true;
if (isOnline && isRegistered) {
cout << "User is online and registered." << endl;
}
Here, the combination of boolean values determines whether the user meets both conditions to reach a specific outcome. If either condition is false, the message will not be displayed.
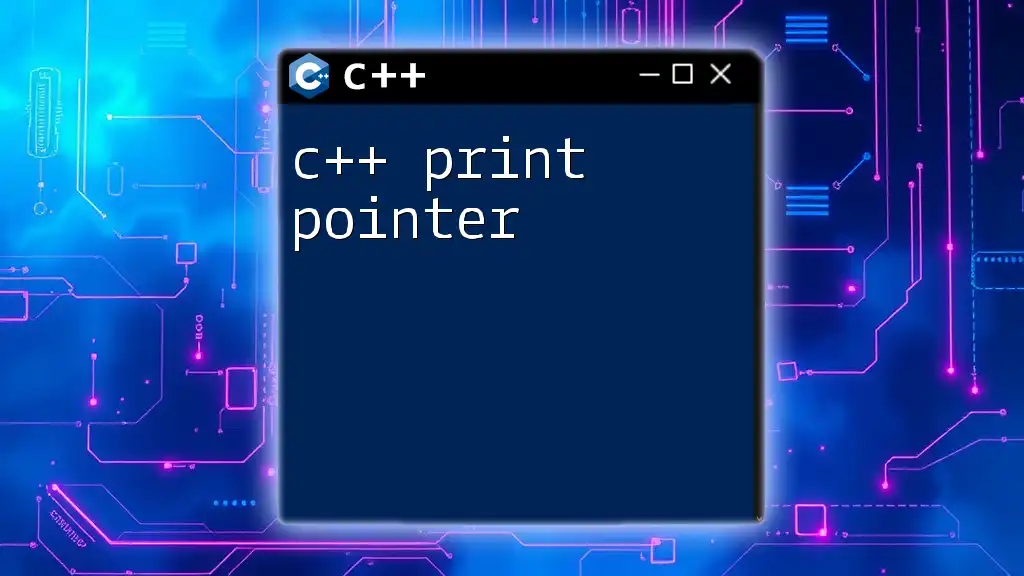
Conclusion
In summary, understanding how to c++ print boolean values is essential for effective coding and debugging. From the basics of using `std::cout` to customizing output formats, mastering these concepts can greatly enhance your programming skills. Experimenting with printing boolean values will not only equip you with debugging tools but will also aid in structuring your C++ programs effectively.
Take the time to practice and explore more of C++ features as you continue to learn and grow in your programming journey!
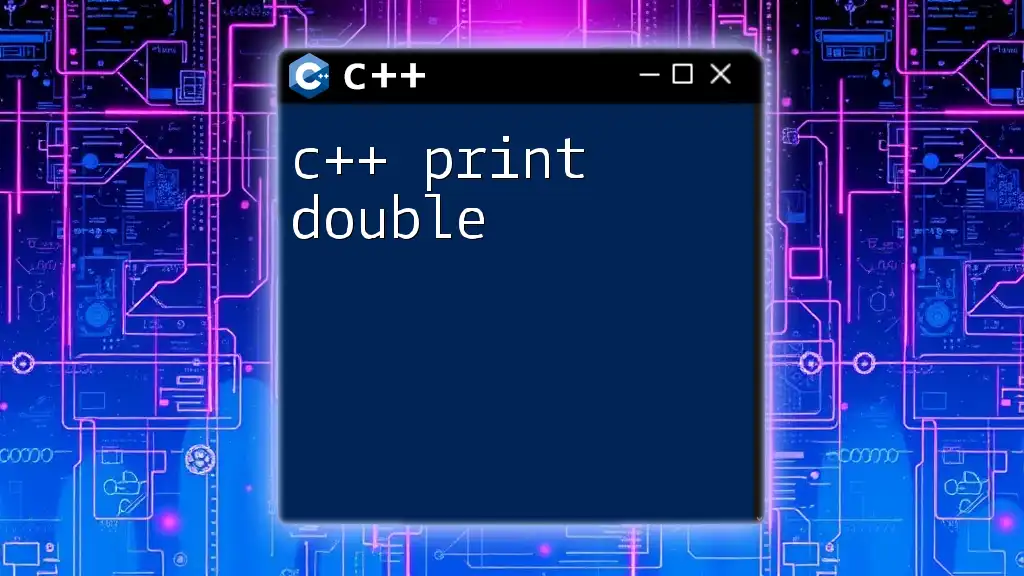
Additional Resources
Related Articles and Tutorials
To further enrich your learning experience, consider exploring articles on advanced C++ topics, best practices for debugging, and more tutorials that cover function usage.
What’s Next?
Stay tuned for more insights into C++ programming, where we will dive deeper into advanced concepts, tips, and tricks to help elevate your coding skills! Engage with our community and share your queries or feedback!