In C++, casting a pointer to a reference can be done using the dereference operator, which allows you to access the object the pointer points to as a reference.
int main() {
int value = 42;
int* ptr = &value;
int& ref = *ptr; // Cast pointer to reference
return 0;
}
Understanding Pointers and References in C++
What is a Pointer?
A pointer is a variable that holds the memory address of another variable. It allows for direct manipulation of the memory, facilitating powerful capabilities such as dynamic memory allocation and array manipulation. The syntax for declaring a pointer is straightforward, using the asterisk (*) to denote a pointer type.
Example:
int a = 10;
int* p = &a; // Pointer to integer a
In this example, `p` is a pointer that holds the address of the variable `a`. This means that by using `p`, you can access or modify the value stored in `a`.
What is a Reference?
A reference is an alias for another variable. When you create a reference, you are not creating a new variable; rather, you are creating a new way to access an existing variable. References are initialized when they are created and cannot be changed to refer to different variables later.
Example:
int& ref = a; // Reference to integer a
In this code, `ref` becomes another name for `a`. Any changes made to `ref` will directly affect `a`, and vice versa.
Comparison Between Pointers and References
Key Differences
-
Memory allocation and access: Pointers require dereferencing to access the value they point to, using the asterisk (*). References do not require this extra step as they can be used as if they were the regular variable itself.
-
Nullability: Pointers can be assigned a `nullptr` value, indicating that they point to nothing. References, on the other hand, must always be initialized to refer to a valid object; they cannot be null.
-
Syntax differences: When using pointers, you need to use the address-of operator (&) to assign a value. References, however, use a different syntax as they are declared with the ampersand (&) in the type definition.
Example:
// Pointer can be reassigned
int* p2 = nullptr;
p2 = &a; // Reassigning pointer to point to a new variable
// Reference must be initialized once
int& ref2 = a; // Once initialized, ref2 cannot refer to a different variable
When to Use Pointers vs. References
Pointers are generally preferred in scenarios that require dynamic memory management or when you need to work with collections of items (like arrays or dynamic structures). Conversely, references are often better when you want to ensure that a variable is always valid; they provide syntactical simplicity and clarity.

The Concept of Casting in C++
What is Casting?
Casting is the process of converting a variable from one type to another. In C++, there are several types of casting available: `static_cast`, `dynamic_cast`, `const_cast`, and `reinterpret_cast`. Each serves a different purpose, allowing you to control how variables are interpreted and manipulated.
Importance of Casting
Casting is essential for various reasons. It allows for type conversion when you are working with different data types, ensuring that your code runs smoothly without type mismatches. For example, when you want to upcast or downcast an object in class hierarchies, casting is crucial.
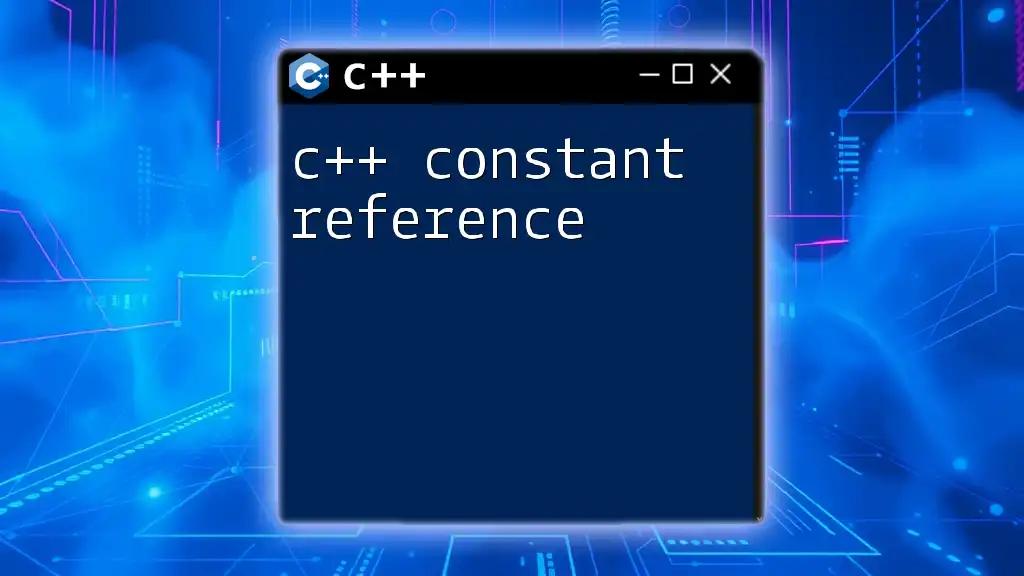
Casting from Pointer to Reference
How to Cast Pointer to Reference
To cast a pointer to a reference, you dereference the pointer, essentially accessing the value it points to. The syntax simply involves using the dereference operator (*) on the pointer.
Example:
int* ptr = &a;
int& ref = *ptr; // Casting pointer to reference
Here, `ref` becomes a reference to the integer value pointed to by `ptr`. Changes made through the reference will affect the original variable.
Safety and Error Handling
It's important to ensure that the pointer is valid (i.e., not null) before casting it to a reference. Dereferencing a null pointer can lead to undefined behavior, causing your program to crash or behave unpredictably.
Example of safe casting:
if (ptr != nullptr) {
int& ref_safe = *ptr; // Safe casting
// Now you can use ref_safe safely
} else {
// Handle error, e.g., print an error message or throw an exception
}
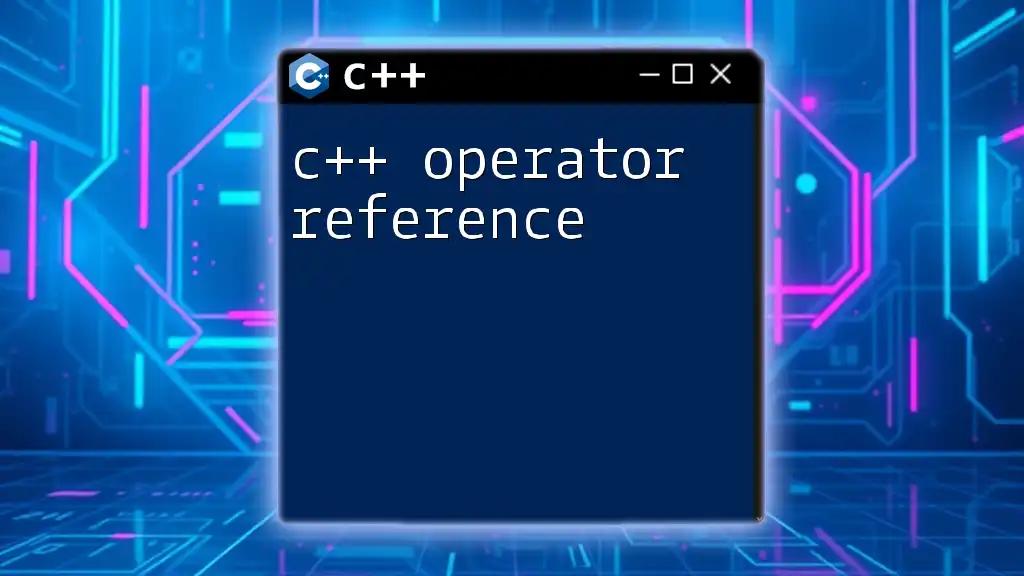
Practical Examples
Example 1: Basic Pointer to Reference Casting
Casting a pointer to a reference can be particularly useful for modifying data without needing to use the pointer everywhere in your code. Consider this method that updates the value of an integer by using a pointer:
void updateValue(int* ptr) {
if (ptr != nullptr) {
int& ref = *ptr;
ref += 5; // Increments the original variable by 5
}
}
In this function, if the pointer is not null, we cast it to a reference and modify the original variable directly.
Example 2: Using Casting for Function Parameters
Casting is also helpful when dealing with function parameters. For instance, passing a pointer to a function that expects a reference can help in simplifying code, particularly for operations on larger types.
void process(int* ptr) {
if (ptr != nullptr) {
int& ref = *ptr; // Casting pointer to reference for safer code
ref *= 2; // Double the original value
}
}
This technique enables you to maintain the integrity of the variable while making the function easier to work with.

Performance Considerations
In most cases, the performance difference between using pointers and references is negligible. However, pointers may introduce some overhead when involved in complex data structures due to the dereferencing operation. References generally offer a clearer, more concise syntax, often proving beneficial in terms of code maintenance.

Common Mistakes and How to Avoid Them
Mistakes When Casting Pointers to References
One common mistake programmers make is failing to check whether a pointer is null before casting it to a reference. It’s essential to ensure that the pointer is valid. Another mistake is attempting to use references assuming they can be reassigned like pointers, which can lead to confusion.
To avoid these pitfalls:
- Always check for null pointers before dereferencing.
- Remember that once a reference is set, it cannot be changed to reference another variable.

Conclusion
Understanding how to cast pointer to reference in C++ is pivotal for effective memory management and code clarity. By recognizing the differences between pointers and references, learning the casting process, and adhering to best practices, you can enhance your programming skills significantly. Bridging the gap between pointers and references opens up a world of possibilities in C++, making you a more proficient and efficient programmer. Don't hesitate to practice these concepts and seek additional resources to deepen your understanding of C++.