In C++, passing by reference allows a function to modify the original variable passed to it, rather than a copy, thereby improving performance and enabling direct manipulation of the variable's value.
Here’s a simple code snippet demonstrating passing by reference:
#include <iostream>
void increment(int& value) {
value++;
}
int main() {
int num = 5;
increment(num);
std::cout << "Incremented value: " << num << std::endl; // Output: Incremented value: 6
return 0;
}
What Does Passing by Reference Mean?
Passing by reference in C++ refers to the method of providing a function with references to variables rather than copies of their values. This allows the function to directly manipulate the original data without creating additional copies, optimizing both memory usage and processing time.
Difference Between Pass by Value and Pass by Reference
When you pass by value, a copy of the variable is made. Modifications within the function have no effect on the original variable. In contrast, when you pass by reference, you provide a reference (essentially an alias) to the original variable, meaning any changes made within the function will affect the actual variable.
To visualize this, if you have a variable `x`, passing `x` by value creates a new variable with its own memory space, while passing `x` by reference directs the function to use the same memory location.
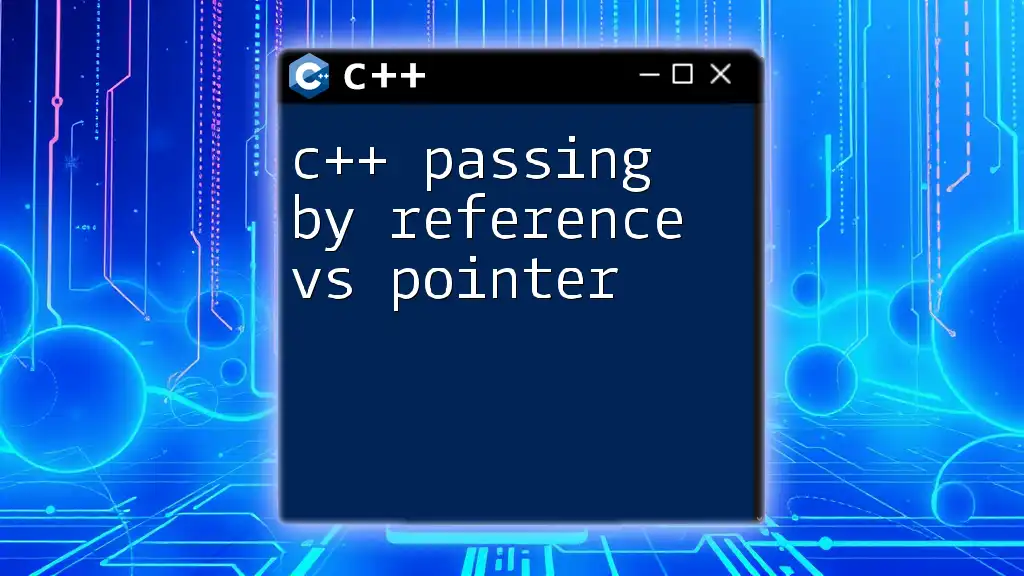
Advantages of Passing by Reference in C++
-
Efficiency: Passing large data structures (like arrays or objects) by reference can significantly reduce the overhead associated with copying their contents. This not only saves memory usage but also improves performance, especially in applications demanding efficiency.
-
Ability to modify the original variable: Functions can modify the passed argument directly. This can lead to clearer, more intuitive code when the intent is to manipulate the original data.
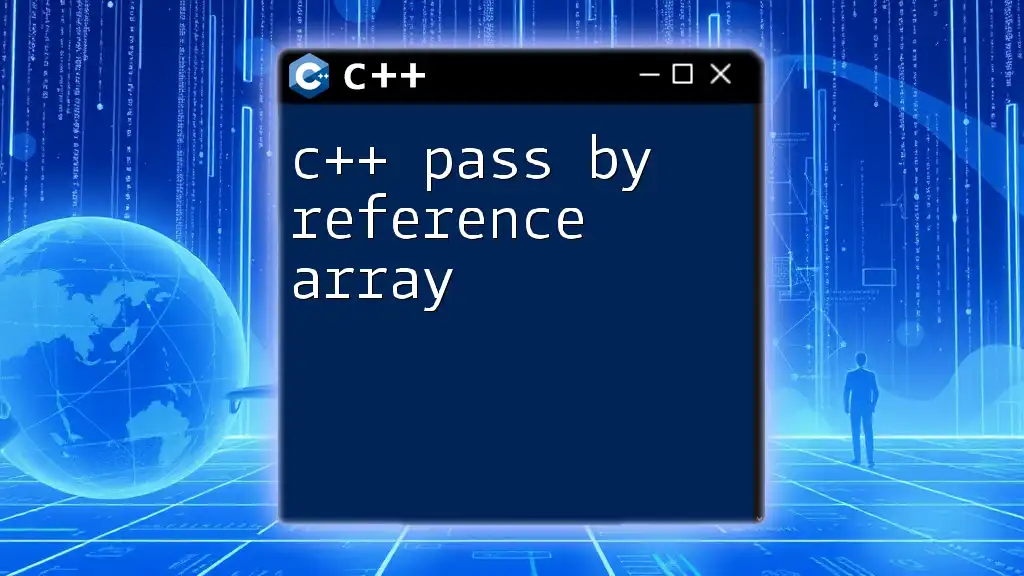
How to Pass by Reference in C++
The syntax for passing by reference is straightforward. You simply prepend the variable type with an `&` symbol in the function declaration. This informs the compiler that the function will receive a reference to the variable, not a separate copy.
Here’s a basic code snippet that illustrates how to pass a variable by reference:
void updateValue(int &value) {
value = 100; // Directly modifies the original variable
}
In this example, when you call `updateValue(x)`, `x` will be set to `100` directly in the calling scope.
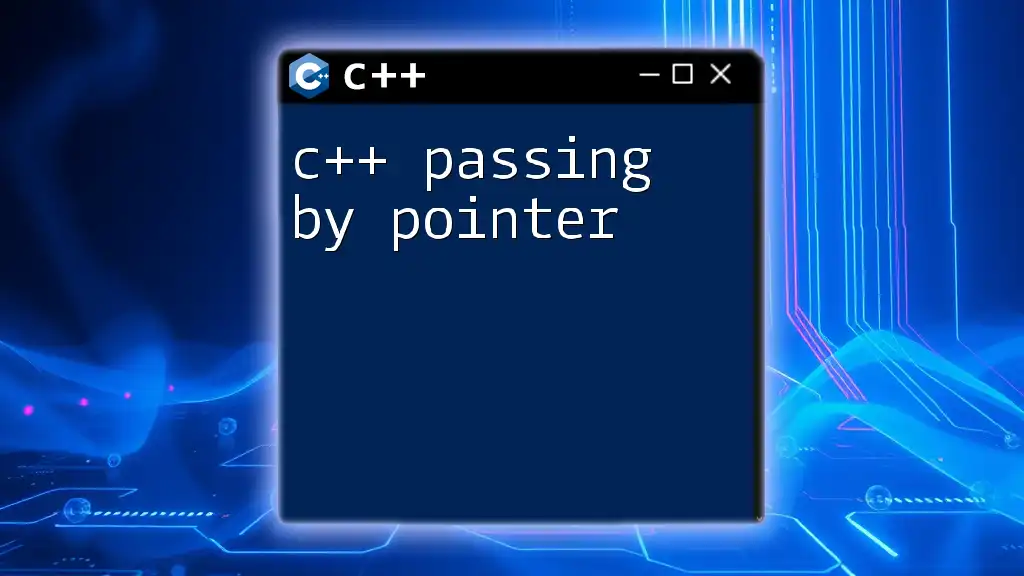
Examples: C++ Pass by Reference in Action
Example 1: Swapping Values
Passing by reference is particularly useful when swapping two values. Here’s a function that swaps two integers:
void swap(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
When you call this function with:
int x = 5, y = 10;
swap(x, y);
After execution, `x` will be `10` and `y` will be `5`. The function successfully modifies both variables in the calling scope.
Example 2: Modifying a Class Member
You can also use passing by reference to modify class members directly. Consider a class `Counter`:
class Counter {
public:
int count;
Counter() : count(0) {}
};
void increment(Counter &c) {
c.count++; // Directly modifies the count member
}
When you call `increment(counterObj)`, the `count` value of `counterObj` will be increased by one without creating additional copies of the `Counter` object itself.
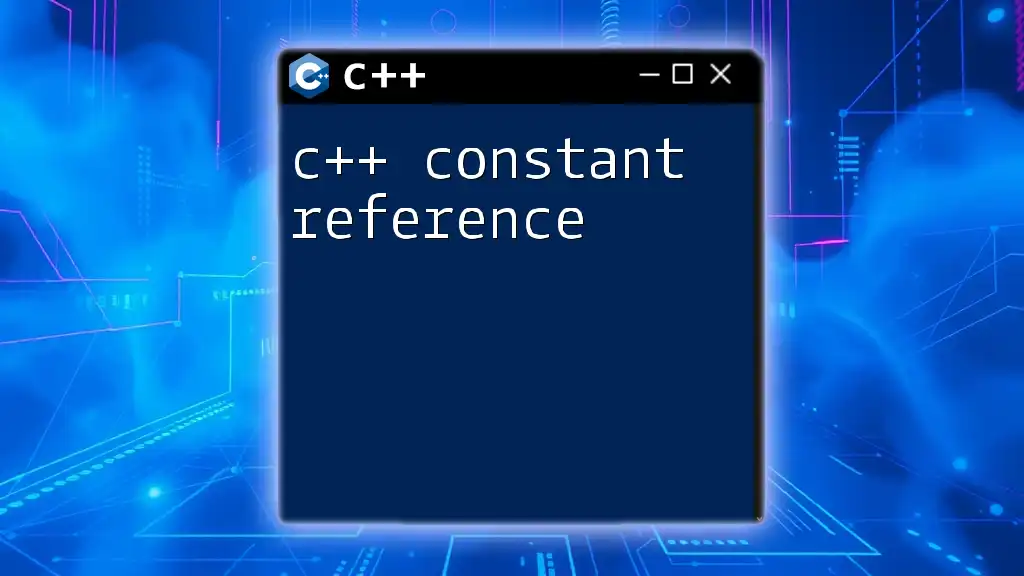
Common Pitfalls and Mistakes with Passing by Reference
While passing by reference has many advantages, there are pitfalls to be aware of:
-
Forgetting to use the reference operator `&`: Omitting this can lead to unexpected behavior where you inadvertently pass by value.
-
Changes to pointers vs. references: Understanding the distinction is vital. A pointer can be reassigned, while a reference cannot. Using a pointer might prevent unintended modifications.
-
Risk of dangling references: If a reference points to a variable that goes out of scope, using that reference can lead to undefined behavior. To avoid this, ensure that references remain valid as long as they are in use.
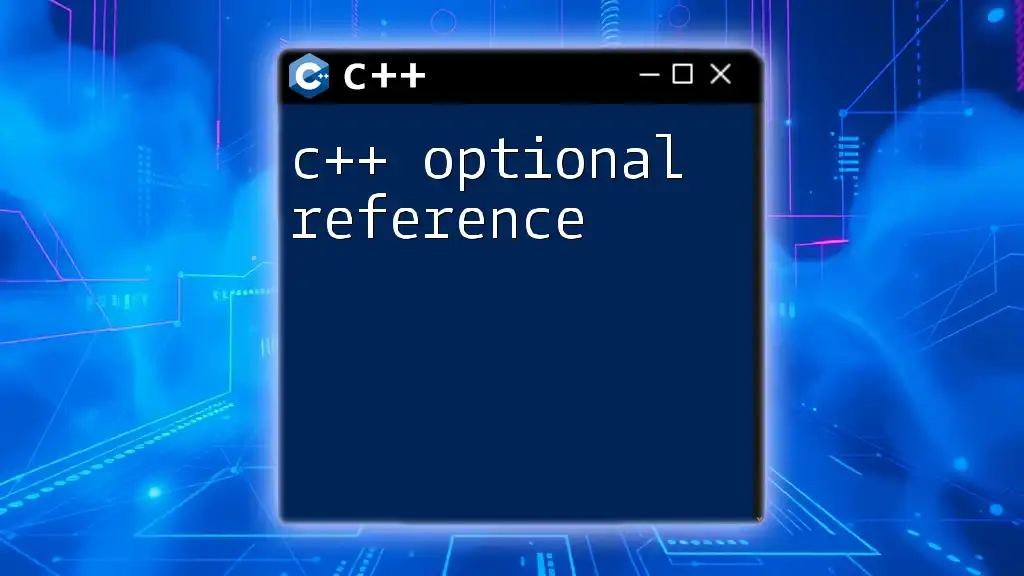
How to Pass by Reference in C++ with Const
Using `const` with references allows the function to read the variable without modifying it. This method is essential when you want to ensure the function does not alter the provided argument. Here’s how it's done:
void display(const int &value) {
std::cout << value << std::endl; // Safe read, no modifications allowed
}
Using `const` prevents any changes to `value`, promoting safer code practices while still benefiting from the efficiency of passing by reference.
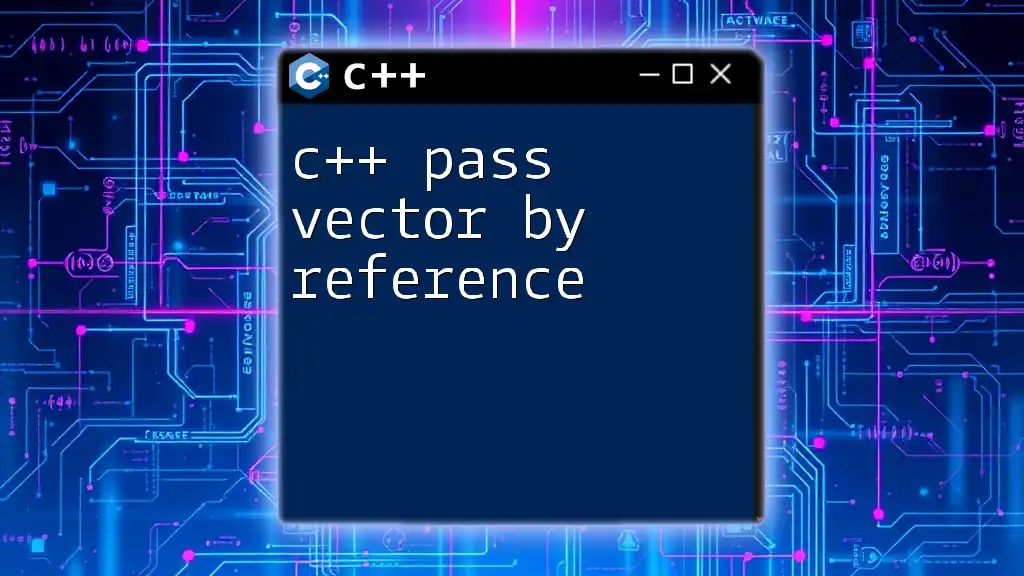
C++ Pass by Reference vs. Pointer
While both passing by reference and pointers allow functions to manipulate the original data, they have key differences:
-
Syntax: Pointers require explicit dereferencing and have to be declared with `*`, while references use `&` and omit dereferencing, leading to cleaner syntax.
-
Reassignment: Pointers can be reassigned to point to different variables, whereas references cannot change which variable they refer to after initialization.
Understanding these distinctions is crucial in scenarios where one might be preferred over the other, such as when dealing with dynamic memory or when needing to represent an optional object.
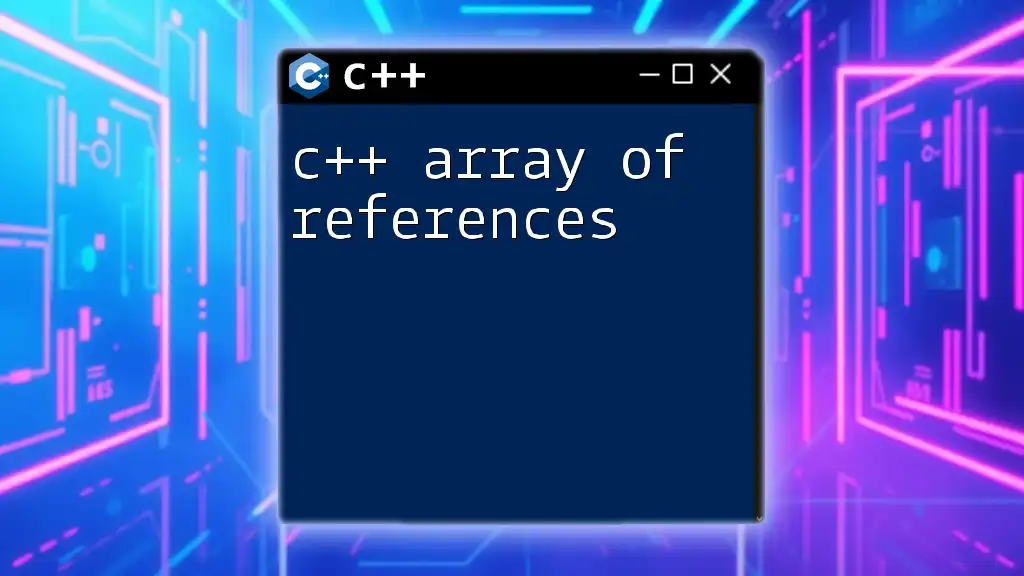
Conclusion
Understanding C++ passing by reference is essential for writing efficient and readable code. It allows functions to manipulate original variables without unnecessary copying, enhancing performance and expressing intent clearly. As you practice with more complex examples, the benefits of passing by reference will become increasingly apparent in your coding journey. Don't hesitate to explore further and ask questions. Happy coding!
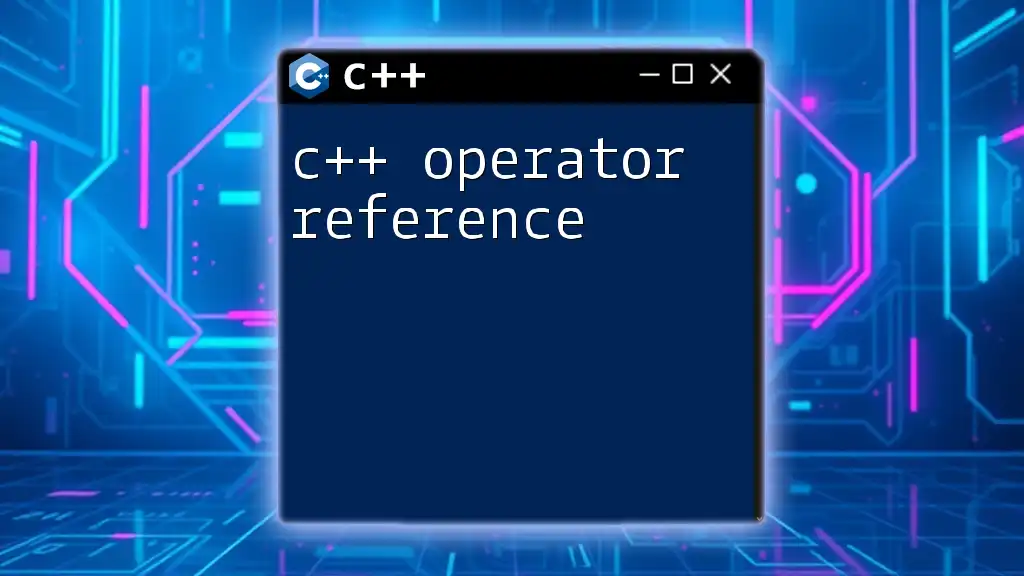
References and Further Reading
For those eager to dive deeper into C++ programming, numerous resources provide comprehensive insights:
- [C++ Reference Documentation](https://en.cppreference.com/)
- [The C++ Programming Language by Bjarne Stroustrup](https://www.stroustrup.com/4th.html)
- [Effective C++ by Scott Meyers](https://effectivecpp.com/)
These references will strengthen your understanding and mastery of C++ concepts, including passing parameters efficiently.