In C++, a vector of references can be implemented using `std::reference_wrapper`, allowing you to store references in a container like `std::vector`, which typically requires storing values.
Here's a code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <functional> // for std::reference_wrapper
int main() {
int a = 10, b = 20, c = 30;
std::vector<std::reference_wrapper<int>> vec = {std::ref(a), std::ref(b), std::ref(c)};
for (auto& ref : vec) {
std::cout << ref.get() << " "; // Accessing the values through references
}
return 0;
}
What is a Vector in C++?
Definition of a Vector
In C++, a vector is a dynamic array that can resize itself automatically when elements are added or removed. Unlike traditional arrays that have a fixed size, vectors provide more flexibility in managing data storage.
Creating Vectors
To declare a vector, you can use the following syntax:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
return 0;
}
In the example above, we have created a vector named `numbers` that contains five integers.
Common Operations on Vectors
Vectors support a variety of operations, some of the most common include:
-
Adding elements: The `push_back()` method appends an element to the end of the vector.
numbers.push_back(6); // Appending 6 to the vector
-
Accessing elements: You can access elements using the index operator `[]`.
std::cout << numbers[0]; // Outputs the first element (1)
-
Iterating through vectors: Vectors can be traversed using loops.
for (int num : numbers) { std::cout << num << " "; }
These operations demonstrate the versatility and ease of use of vectors in C++.
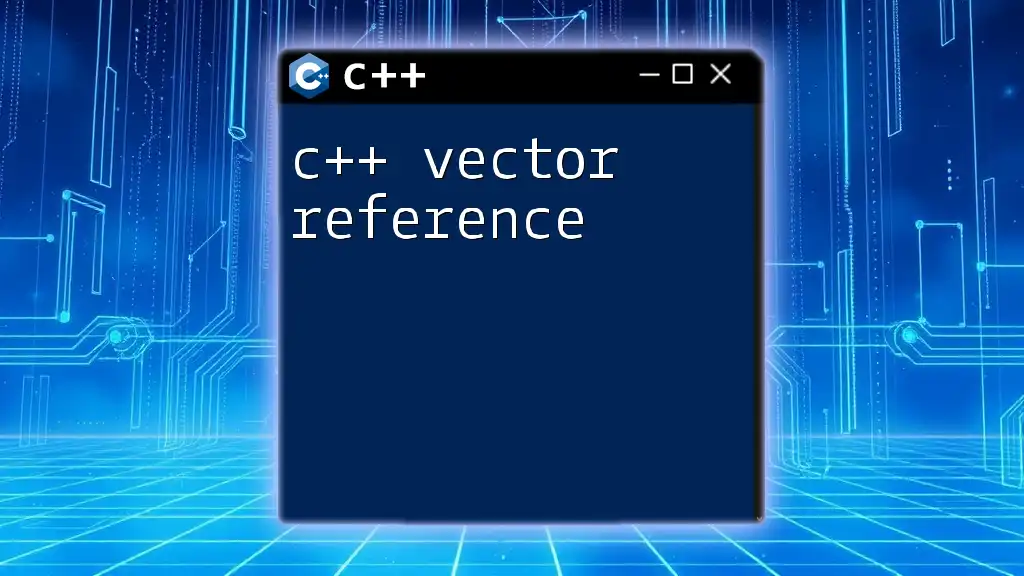
Understanding References in C++
What is a Reference?
A reference in C++ is essentially an alias for another variable. Once a reference is established, it acts as an alternative name for the original variable, allowing you to modify the variable through the reference itself.
How References Work
The lifetime of a reference is bound to the lifetime of the variable it refers to. If the original variable goes out of scope, the reference becomes invalid. For example:
void increment(int &num) {
num++;
}
In this case, `num` is a reference to an integer. Calling the `increment` function will increase the value of the original variable passed to it.
Benefits of Using References
Using references can lead to:
- Reduced memory usage, as no additional variable or pointer memory is needed.
- Simplified code, making it easy to read and maintain.
For example, instead of passing a large object to a function, you can pass a reference, which can enhance performance significantly.
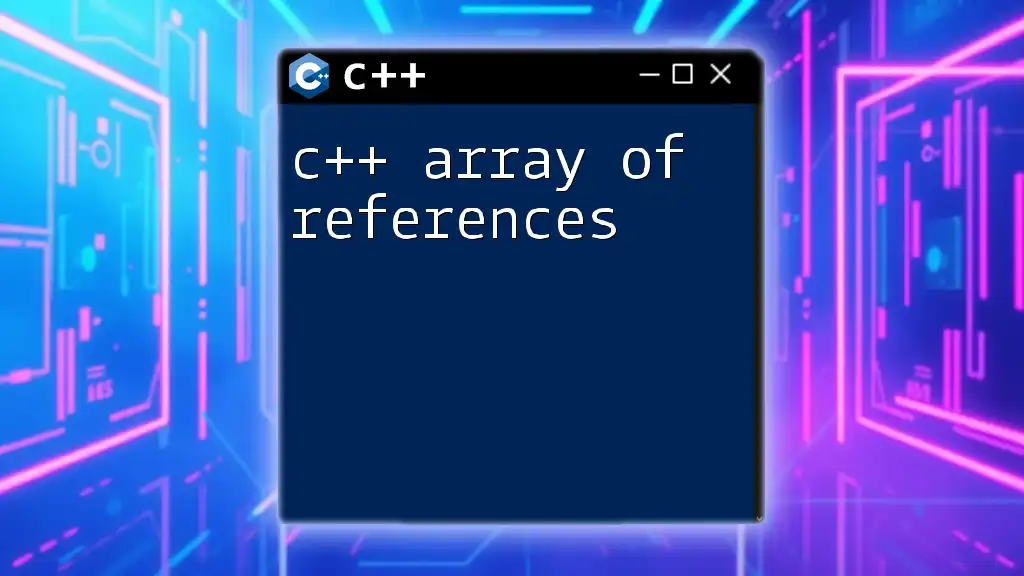
C++ Vector of References
What is a Vector of References?
A C++ vector of references is a vector that holds references instead of the values directly. This means that the vector will reference existing variables, allowing changes made to the referenced variable to reflect in the vector itself.
Syntax and Declaration
To declare a vector of references, use the following syntax:
std::vector<int&> refVector;
Here, `refVector` can hold references to integer variables.
Use Cases for a Vector of References
Vectors of references can be advantageous in scenarios where:
- You want to maintain a dynamic list of objects or variables without copying them.
- Performance is critical, especially when dealing with large data structures.
As an example, consider managing a collection of counters, where modifying one count should reflect in all its references.
Accessing Elements in a Vector of References
To access and modify elements in a vector of references, you can simply use index notation. Here’s an example:
int a = 10, b = 20;
std::vector<int&> refVector = {a, b};
refVector[0] = 30; // Now a becomes 30
In this code, changing `refVector[0]` directly modifies `a`, demonstrating how references within a vector can lead to real-time updates on the original variables.
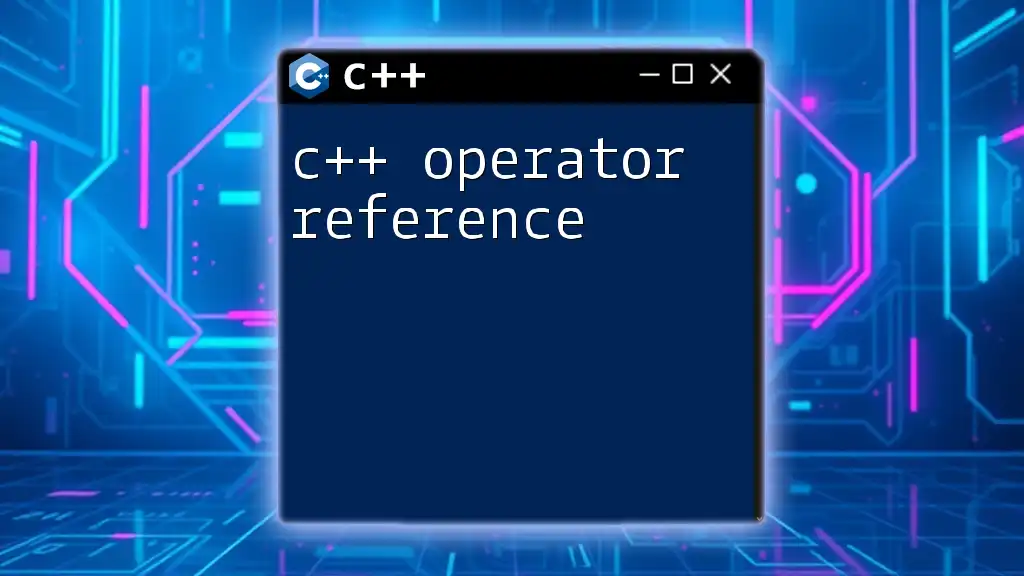
Common Mistakes When Using Vectors of References
Lifetimes of Referenced Variables
One of the most crucial aspects to validate when using vectors of references is ensuring that the referenced variables remain in scope. A frequent mistake is to create a reference to a local variable which subsequently goes out of scope:
int* p = nullptr;
{
int x = 10;
std::vector<int&> refVector = {x}; // okay
} // x goes out of scope
// refVector now holds a reference to an invalid location
When the block ends, `x` is destroyed, leaving `refVector` with a dangling reference.
Using Vectors of References with Non-Copyable Types
Be cautious when using vectors of references with objects that cannot be copied (like `std::unique_ptr` or other non-copyable classes). For instance, if you're attempting to store vectors of references to non-copyable objects, you might end up running into compile-time errors or unpredictable behavior.
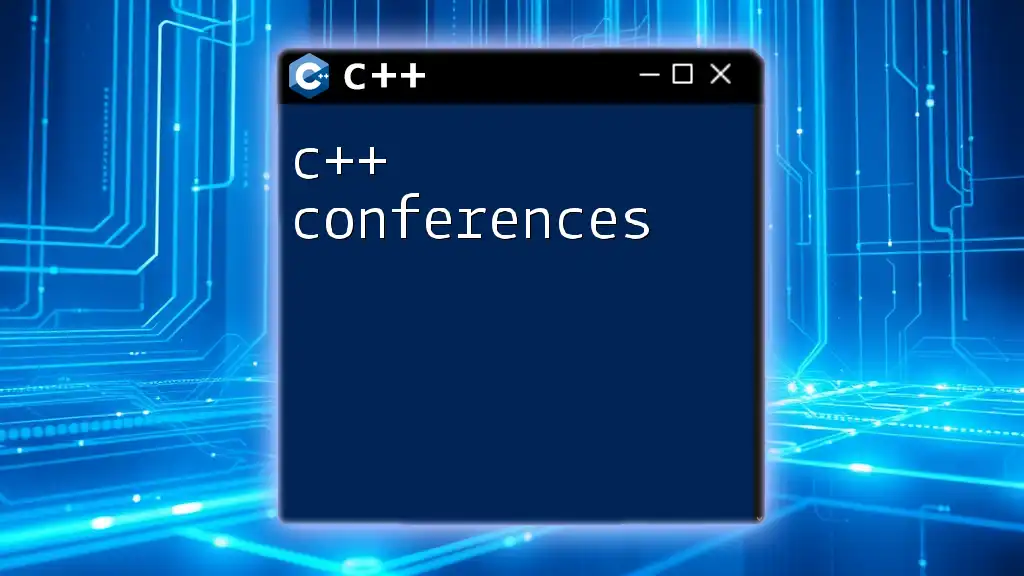
Conclusion
Understanding the C++ vector of references is crucial for efficient memory management and performance optimization. By leveraging this feature, developers can manage collections of variables effectively while avoiding unnecessary copies. The potential pitfalls, including lifetime concerns and non-copyable objects, highlight the necessity of meticulous coding practices. Equipped with this knowledge, you can confidently implement vectors of references in your C++ applications to streamline operations and enhance efficiency.
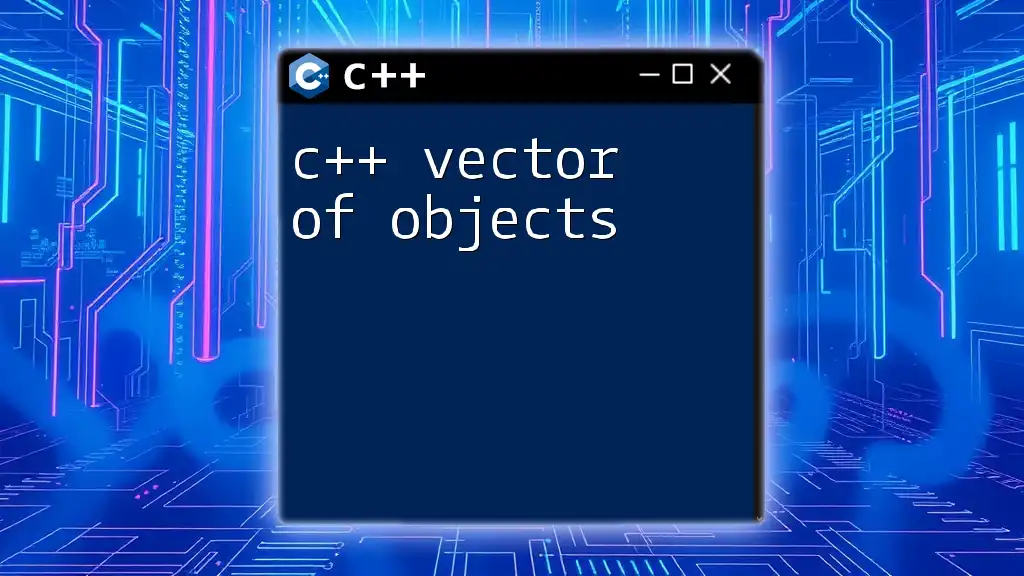
Additional Resources
For further learning, consider exploring additional resources like:
- C++ official documentation
- Online tutorials on C++ STL (Standard Template Library)
- Books on advanced C++ topics focusing on memory management and performance
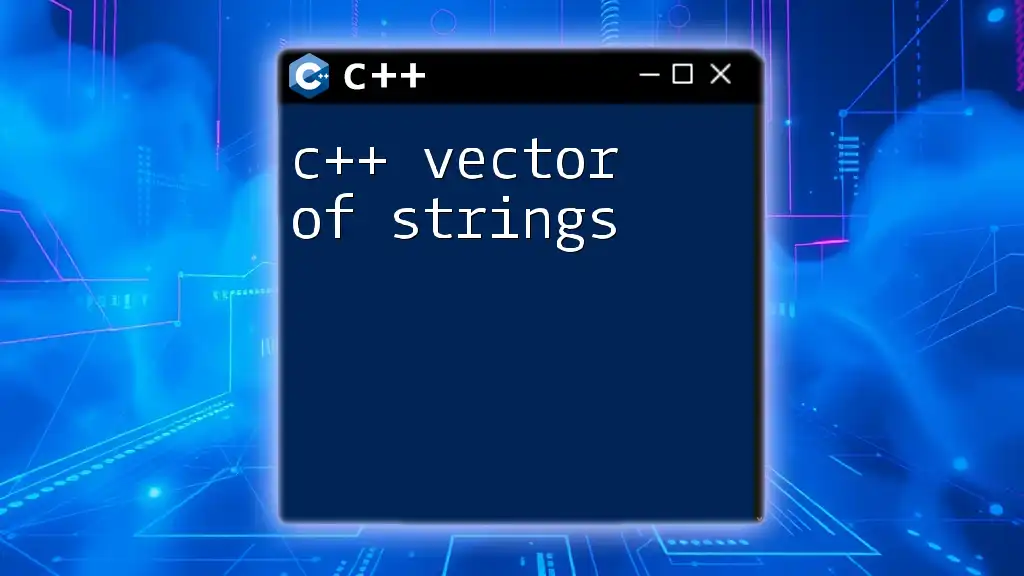
FAQs
Can you put references to local variables in a vector?
No—doing so can lead to dangling references as local variables go out of scope once they fall out of the block where they were declared.
What happens when a reference inside a vector is modified?
Modifying a reference within a vector will also change the original variable it points to, since a reference is essentially an alias.
Are there alternatives to using a vector of references?
Yes, alternatives include using pointers or smart pointers like `std::shared_ptr` or `std::unique_ptr`, which provide more flexibility in managing memory and object lifetimes.