In C++, a vector reference allows you to create an alias for an existing vector, enabling you to manipulate the original vector's data directly without making a copy.
#include <iostream>
#include <vector>
void modifyVector(std::vector<int>& vec) {
vec[0] = 100; // Modify the first element
}
int main() {
std::vector<int> myVector = {1, 2, 3};
modifyVector(myVector);
std::cout << myVector[0]; // Outputs: 100
return 0;
}
Understanding C++ Vectors
What are Vectors in C++?
C++ vectors are a part of the Standard Template Library (STL) that serve as dynamic arrays. Unlike arrays, vectors can automatically resize themselves during runtime, making them extremely versatile. This flexibility allows developers to easily manage collections of elements without worrying about preallocating a fixed size.
Using vectors offers several benefits over traditional arrays:
- Dynamic resizing: You can add or remove elements at runtime.
- Memory management: Vectors manage their own memory, reducing the need for manual allocation and deallocation.
- Rich features: Vectors come with numerous built-in functions for searching, sorting, and modifying their elements.
Basic Operations on Vectors
Understanding basic operations is crucial for leveraging the power of vectors. Here are some fundamental operations:
Creating a vector: To create a vector, simply include the relevant library and declare a vector with a specific data type.
#include <vector>
std::vector<int> vec;
Adding elements: You can add elements using the `push_back()` method, which appends an element to the end of the vector.
vec.push_back(10); // Adds 10 to the vector
Accessing elements: You can access elements using the indexing operator. Keep in mind that vectors use zero-based indexing.
int firstElement = vec[0]; // Retrieves the first element (10 in this case)
Removing elements: To remove the last element from the vector, you can use the `pop_back()` method.
vec.pop_back(); // Removes the last element (10)
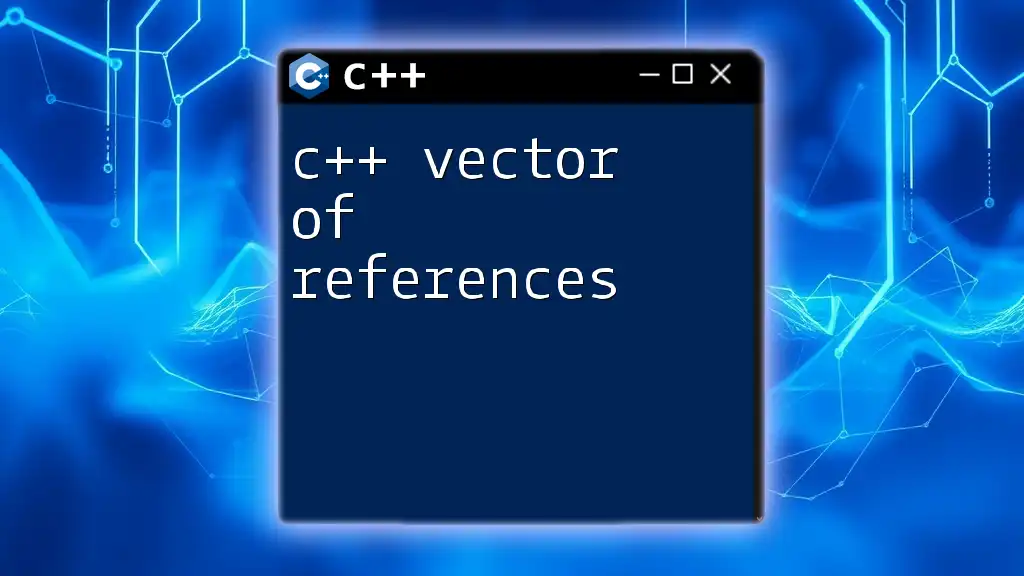
What is a Reference in C++?
Definition of References
In C++, a reference is an alias for another variable. Unlike pointers, which can point to various memory locations, references must be initialized to refer to a particular variable and cannot be changed thereafter. This makes them straightforward and more intuitive to use.
Benefits of Using References
Using references in C++ comes with several benefits, particularly in terms of efficiency and code clarity:
- Avoiding unnecessary copies: When you pass large data structures like vectors to functions, using a reference prevents the creation of a copy. This results in better performance.
- Ease of use: References are syntactically simpler than pointers, enhancing code readability and maintainability.
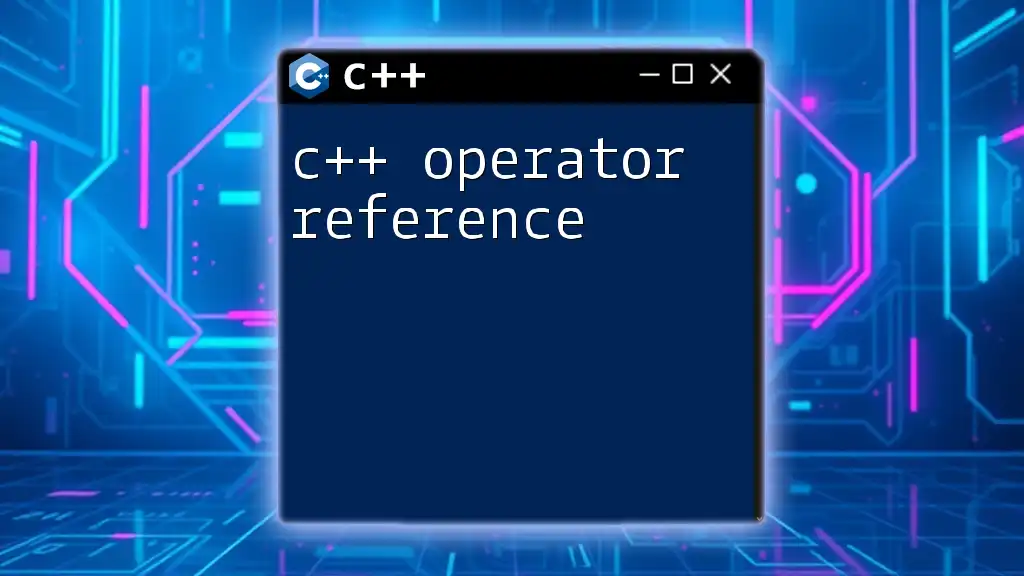
C++ Vector Reference: Detailed Explanation
Declaring a Vector Reference
To declare a reference to a vector, use the `&` symbol along with the vector type. This allows you to work directly with the initial vector without copying its contents.
std::vector<int>& ref = vec; // ref is now a reference to vec
This reference can be particularly useful when you want to manipulate the original vector within a function.
Modifying a Vector via Reference
One of the powerful features of using a vector reference is the ability to modify its contents directly. For example, you can define a function that takes a vector as a reference and adds an element to it.
void modifyVector(std::vector<int>& vecRef) {
vecRef.push_back(20); // Adds 20 to the vector referenced
}
To see this in action, consider the following example:
std::vector<int> myVector = {1, 2, 3};
modifyVector(myVector); // myVector is now {1, 2, 3, 20}
In this case, the original `myVector` is modified since `vecRef` references it directly.
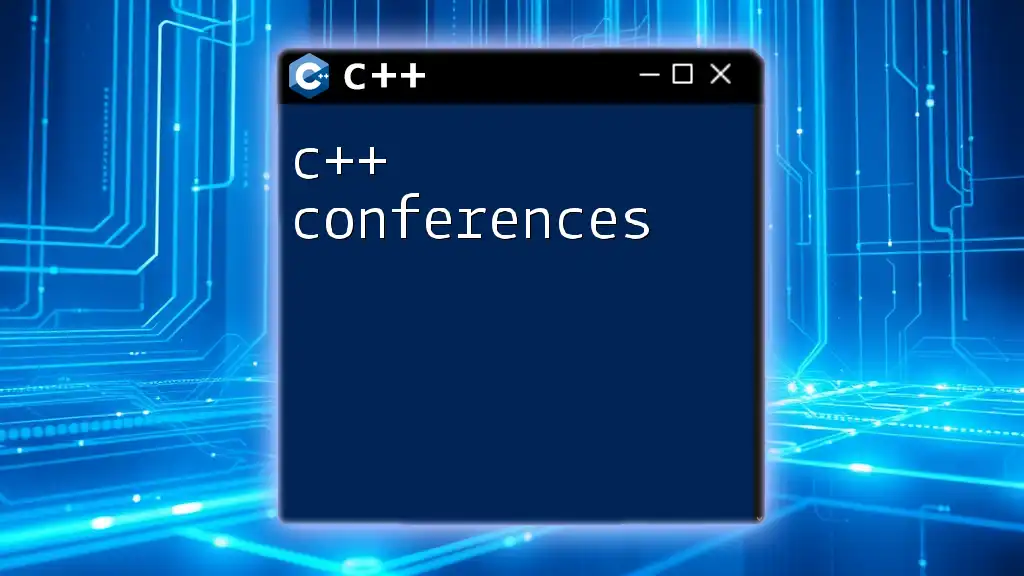
Advanced Concepts of Vector References
Const References
Const references are references that cannot modify the value of the object they refer to. This is useful when you want to pass a vector to a function without the intent of modifying it.
void printVector(const std::vector<int>& vecRef) {
for (const auto& elem : vecRef) {
std::cout << elem << " "; // Outputs each element in the vector
}
}
Using `const` ensures that the function cannot alter the contents of the vector, promoting safer and clearer code practices.
Using References in Standard Library Functions
Many functions in the Standard Library are designed to accept vector references. For example, `std::sort` allows you to sort a vector without copying it:
#include <algorithm>
std::sort(vec.begin(), vec.end()); // Sorts the vector in ascending order
In this case, `std::sort` operates directly on the original vector, maintaining efficiency.
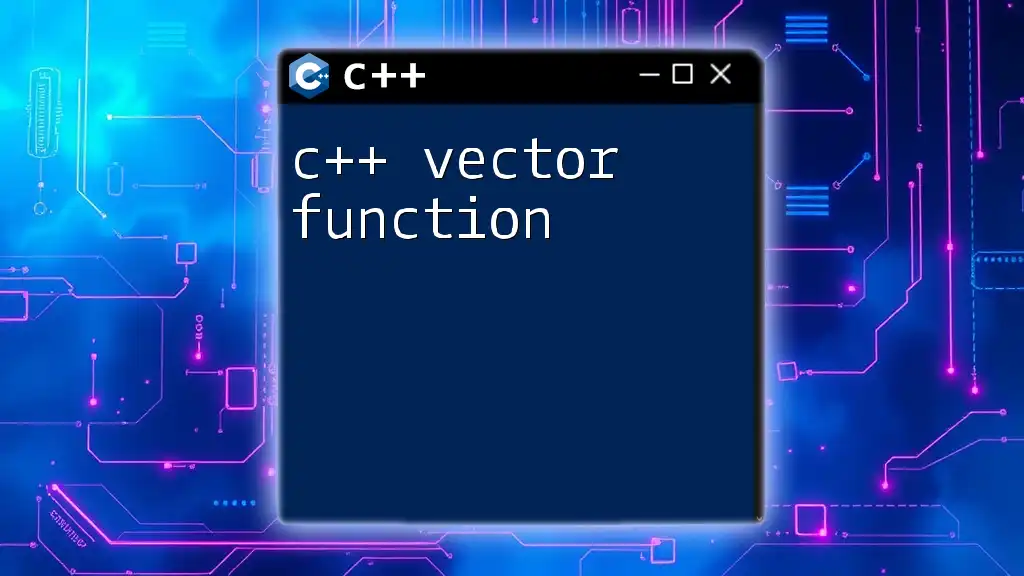
Best Practices for Managing C++ Vector References
Avoiding Dangling References
A dangling reference occurs when a reference points to an object that has gone out of scope. To avoid this, always ensure that the referenced object remains valid for the lifetime of the reference. For example, never return a reference to a local object from a function, as it will be destroyed when the function exits.
Ensuring Safe Access
When working with vectors via references, it’s essential to ensure safe modifications. Performing bounds checking before accessing elements can prevent runtime errors. Always consider whether it’s best to work with a copy or a reference based on your specific use case.
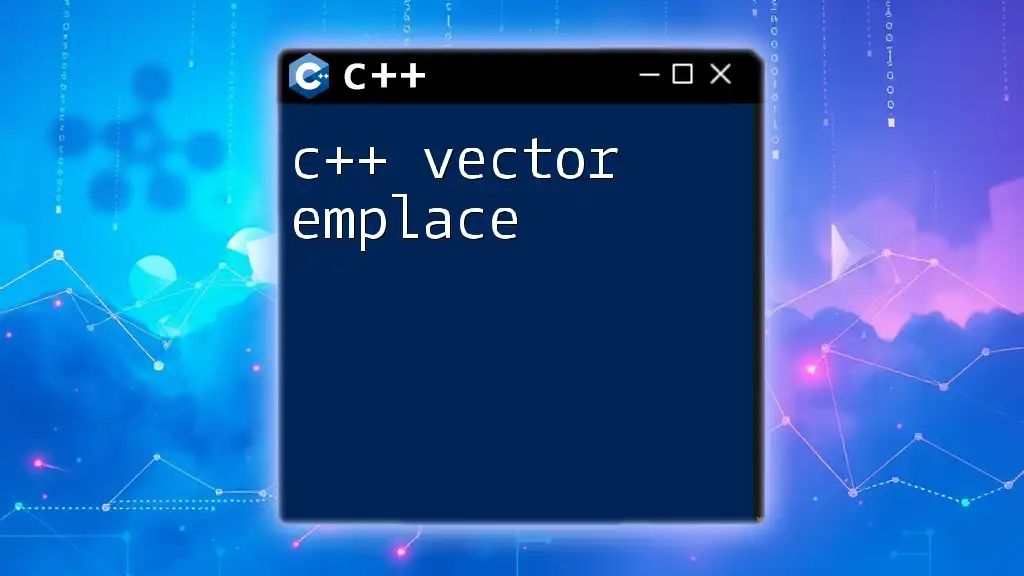
Conclusion
Understanding `c++ vector reference` is crucial for effectively managing data in C++. References allow you to manipulate vectors with increased efficiency and safety, ultimately enhancing your coding practices.
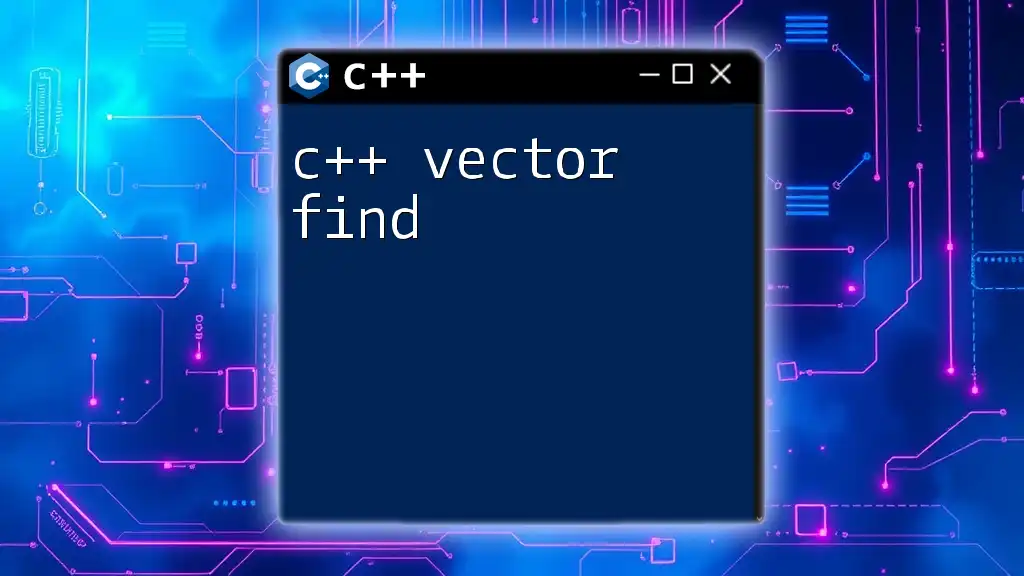
Additional Resources
For those looking to expand their knowledge, consider exploring textbooks on C++, reputable online resources, and community forums where advanced topics and best practices are discussed.
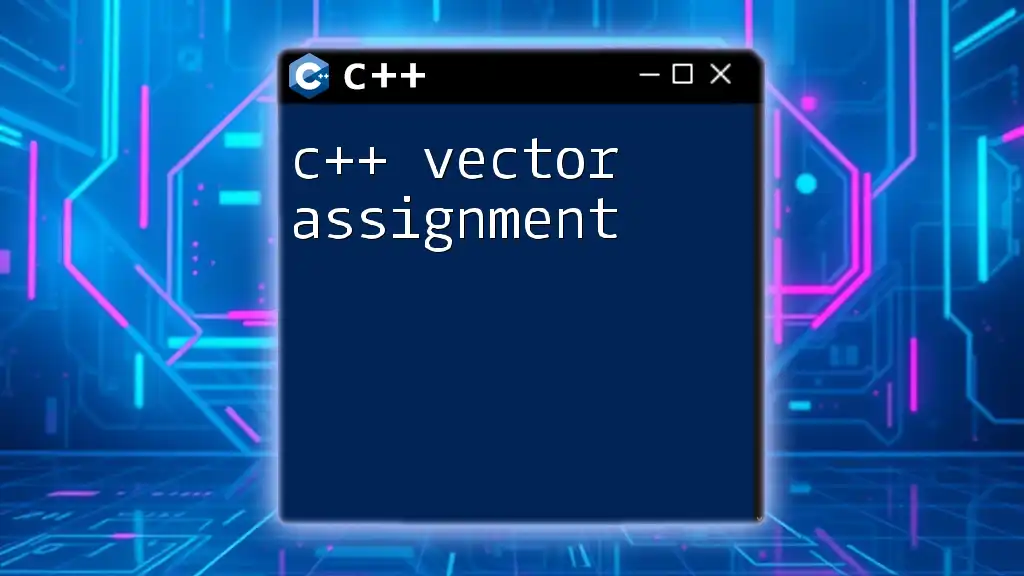
FAQs about C++ Vector References
What is the difference between a vector and a vector reference?
A vector is an object that stores a dynamic array of elements, while a vector reference is an alias for an existing vector, allowing it to be accessed or modified without copying.
Can a vector reference be null?
No, a vector reference must always refer to a valid vector object. You cannot declare a null reference.
How do I pass a vector to a function without a reference?
You can pass a vector to a function by value, but this will create a copy of the vector, which may incur additional overhead.
What happens to a vector reference when the vector goes out of scope?
If a vector goes out of scope, the reference to it becomes dangling, which can lead to undefined behavior if it is accessed afterwards.
Final Thoughts
Understanding and using `c++ vector reference` effectively can transform the way you handle data in your applications. I encourage you to experiment with these concepts, and feel free to share your insights or ask questions about vector references in C++.