C++ reference parameters allow you to pass arguments to functions by reference, enabling the function to modify the original variable rather than a copy.
Here’s a simple example:
#include <iostream>
using namespace std;
void increment(int &number) {
number++;
}
int main() {
int value = 5;
increment(value);
cout << "Incremented value: " << value << endl; // Outputs: Incremented value: 6
return 0;
}
Understanding C++ Reference Parameters
What Are Reference Parameters?
In C++, reference parameters allow a function to access and modify the original variable passed to it. Instead of creating a copy of the variable (as happens with value parameters), a reference parameter acts as an alias, enabling the function to work directly with the original data. This capability not only enhances performance but also allows you to modify the original variable's value directly.
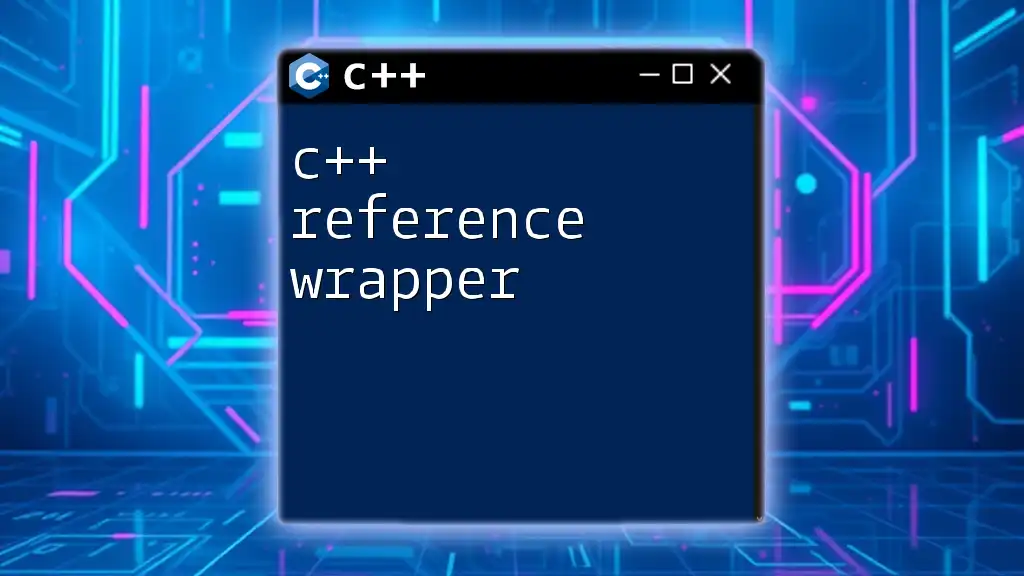
Understanding Reference Arguments in C++
What Are Reference Arguments?
Reference arguments are arguments passed to a function by reference rather than by value. This means that instead of duplicating the data of the variable, the function operates on the original piece of data, which can lead to increased efficiency, especially with large datasets or objects.
Syntax of Reference Parameters
The syntax for declaring reference parameters is straightforward. A reference parameter is denoted by an ampersand (`&`) placed after the data type in the function's parameter list. Here’s an example of the basic syntax:
void functionName(type ¶meterName) {
// function body
}
Using this syntax, you can create functions that interact seamlessly with the data passed to them.
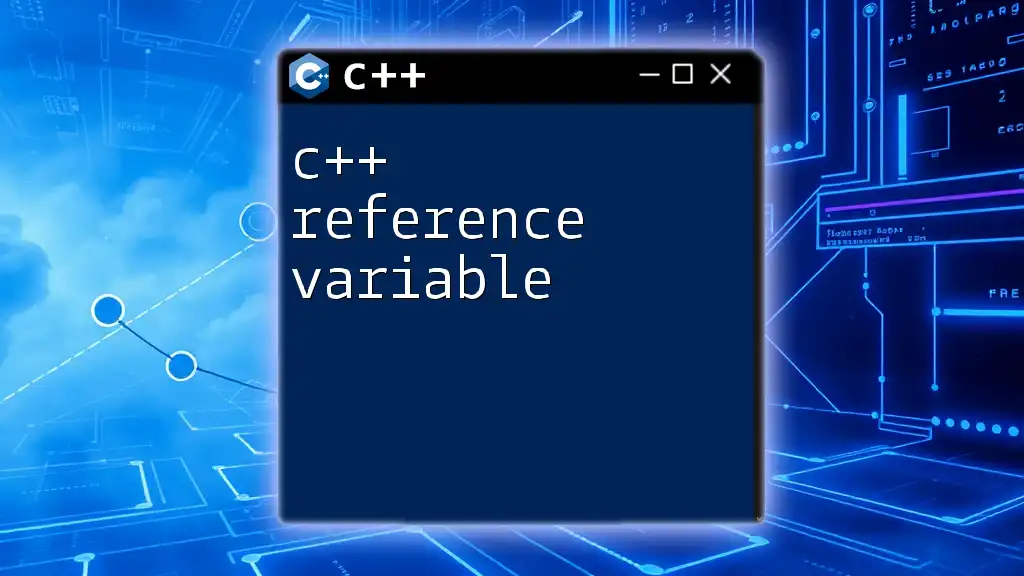
How to Pass Arguments by Reference
Declaring Functions with Reference Parameters
When you declare a function with a reference parameter, you signal that any changes made to the parameter inside the function will directly affect the argument passed. For instance, consider the following example:
void increaseValue(int &num) {
num++;
}
In this code snippet, `increaseValue` is a function that takes an integer by reference and increments its value. This means that any integer variable passed into this function will be directly modified.
Calling Functions with Reference Arguments
When you call a function that takes reference parameters, the original variable is passed to it, allowing direct manipulation. Here’s how you can call the `increaseValue` function:
int main() {
int value = 5;
increaseValue(value);
// value is now 6
return 0;
}
In this example, `value` starts at 5, but after passing it to `increaseValue`, it becomes 6. The direct modification of the original variable showcases the power of reference parameters.
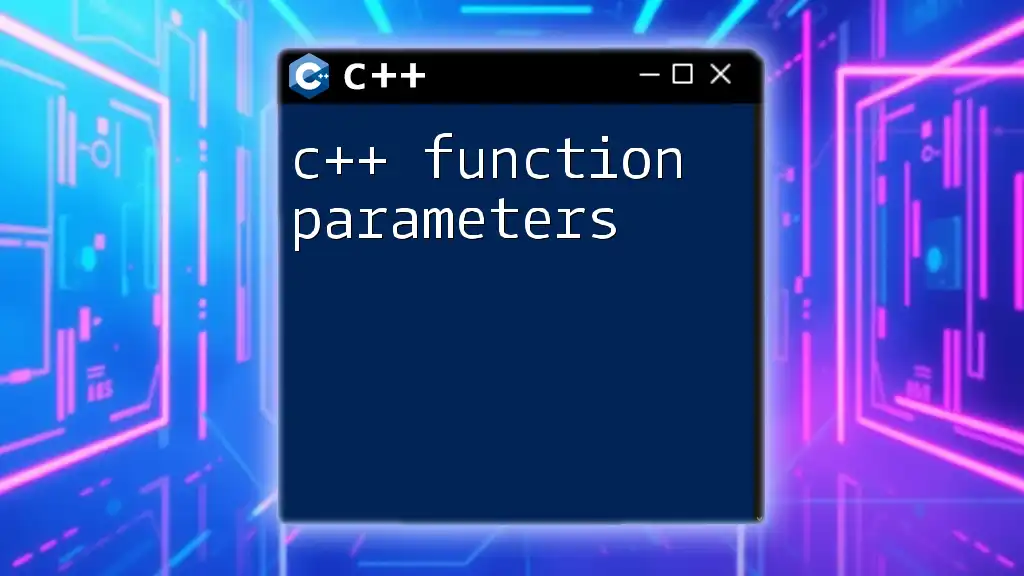
The Advantages of Using Reference Parameters
Performance Benefits
One of the most significant advantages of using reference parameters is performance. When you pass a variable by reference, you're avoiding the overhead associated with copying data. This is especially crucial for large objects, where duplicating the data can be costly in terms of both time and memory.
Memory Efficiency
Passing by reference enhances memory efficiency since only the memory address of the variable is passed to the function. This means that for large data structures, such as arrays or classes, the program does not need to allocate additional space for a copy, thus conserving memory.
Modifying Original Variables
Using reference parameters simplifies scenarios where you want to modify the state of an original variable. Instead of returning modified values, your function can directly change the input, leading to clearer and more concise code.
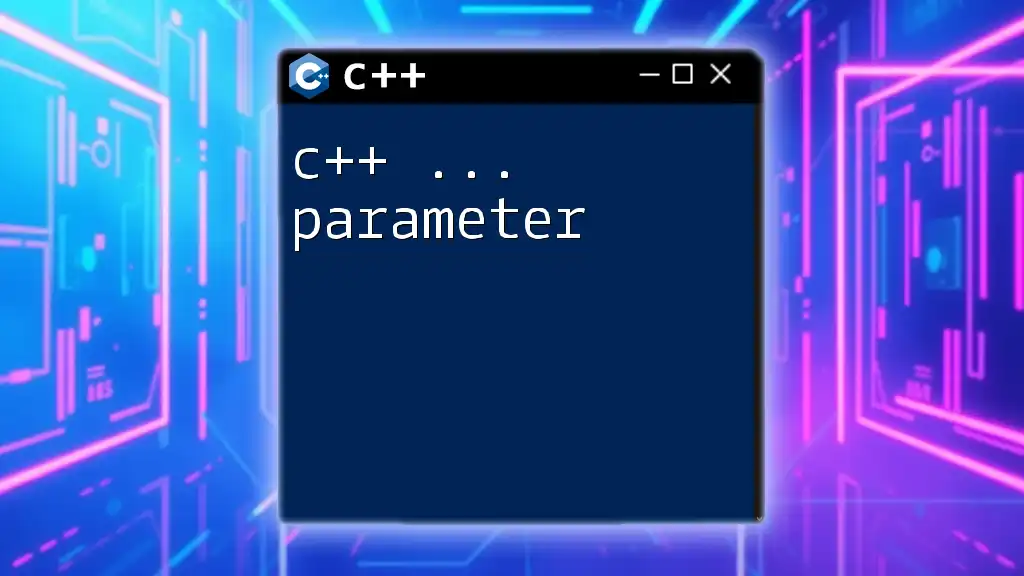
Limitations and Considerations
Potential Pitfalls of Reference Parameters
While reference parameters offer many benefits, they also come with potential risks. One major pitfall is the risk of unintended modifications to variables. If a variable should remain unchanged, passing it by reference can lead to accidental alterations.
When to Use Reference Parameters
It’s advisable to use reference parameters in situations where:
- You need to modify the input variable.
- The data type is large or complex, warranting the performance benefits of avoiding copies.
- You want to improve code clarity by operating on the original data.
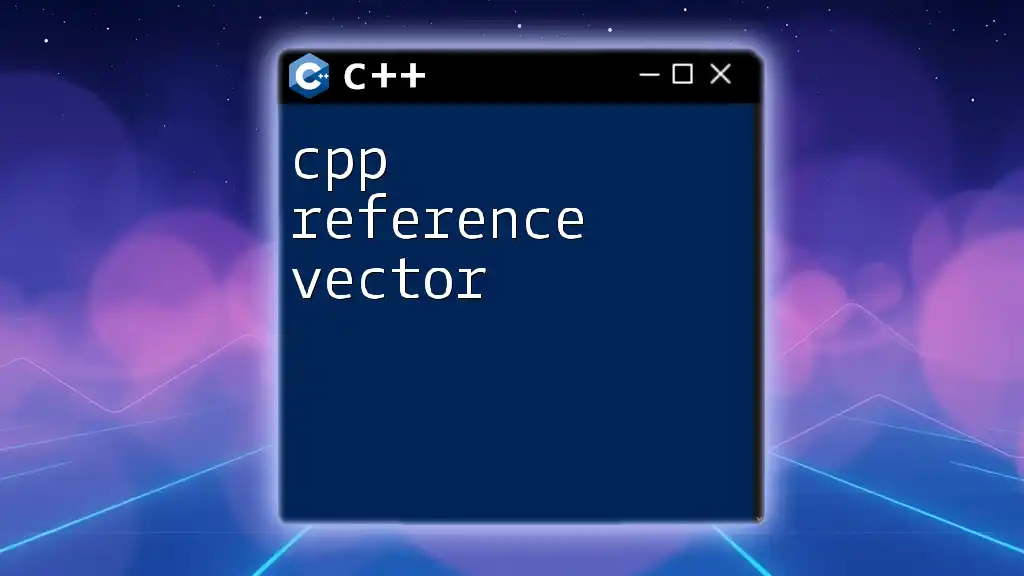
Advanced Concepts in Reference Parameters
Const Reference Parameters
Const reference parameters prevent modification of the original variable while still benefiting from the performance of passing by reference. By using the `const` keyword, you protect the parameter from being altered:
void printValue(const int &num) {
std::cout << num << std::endl;
}
In this example, the `printValue` function can read the value of `num` without the risk of modifying it, making it safe for cases where function input should remain unchanged.
Returning Reference Parameters
Another advanced feature of reference parameters is the ability to return references from functions. This practice can be incredibly useful but should be approached with caution to avoid dangling references. Here’s an example of returning a reference:
int& getMaxValue(int &a, int &b) {
return (a > b) ? a : b;
}
In this function, the larger of two integers is returned by reference. Care must be taken to ensure that the referenced variable remains in scope to avoid undefined behavior.
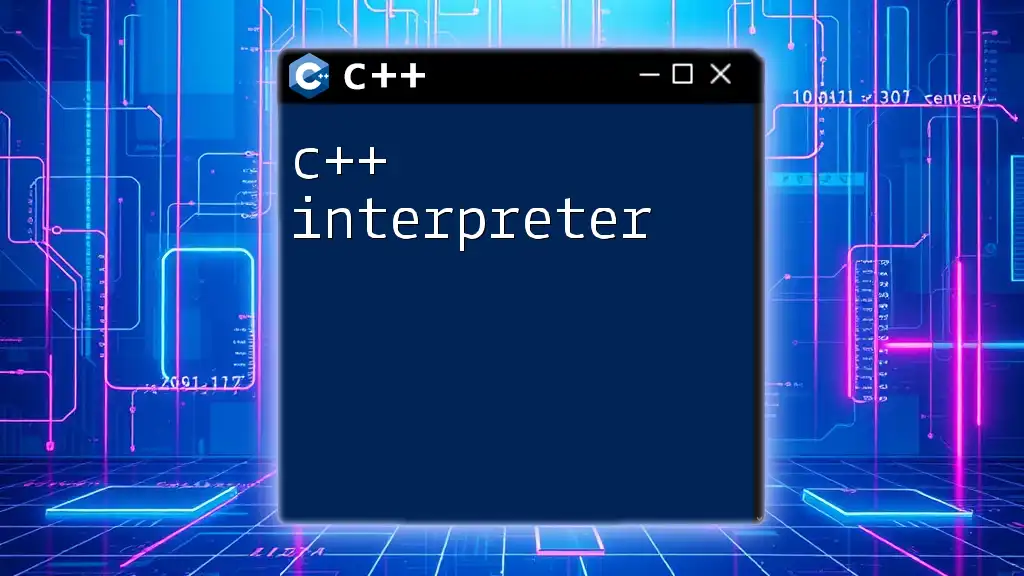
Best Practices for Using Reference Parameters in C++
Tips for Effective Use
To maximize the benefits of reference parameters, consider the following best practices:
- Ensure clarity: Code using reference parameters should be easy to understand. Comments can be helpful.
- Use const references when the function does not modify the input. This makes it clear that the input is purely for reading.
- Avoid using references for small, simple data types where the overhead of copying is minimal. In such cases, value parameters may be easier to understand.
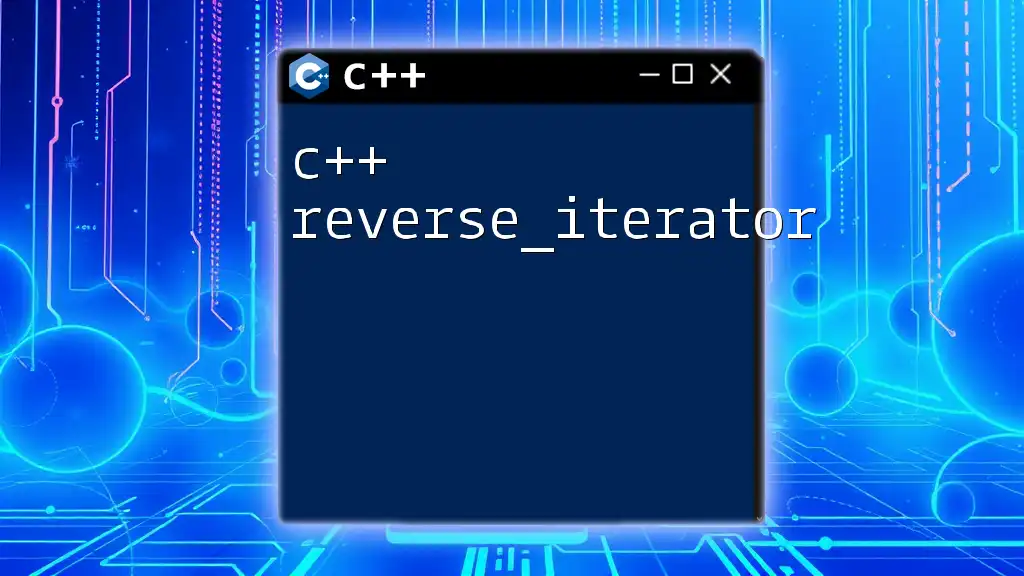
Conclusion
C++ reference parameters open up a powerful toolset for writing efficient and clear code. By allowing functions to manipulate original data directly, they provide both performance benefits and the ability to modify inputs seamlessly. However, caution is necessary to avoid unintended modifications and ensure that the use of references is appropriate for the scenario.
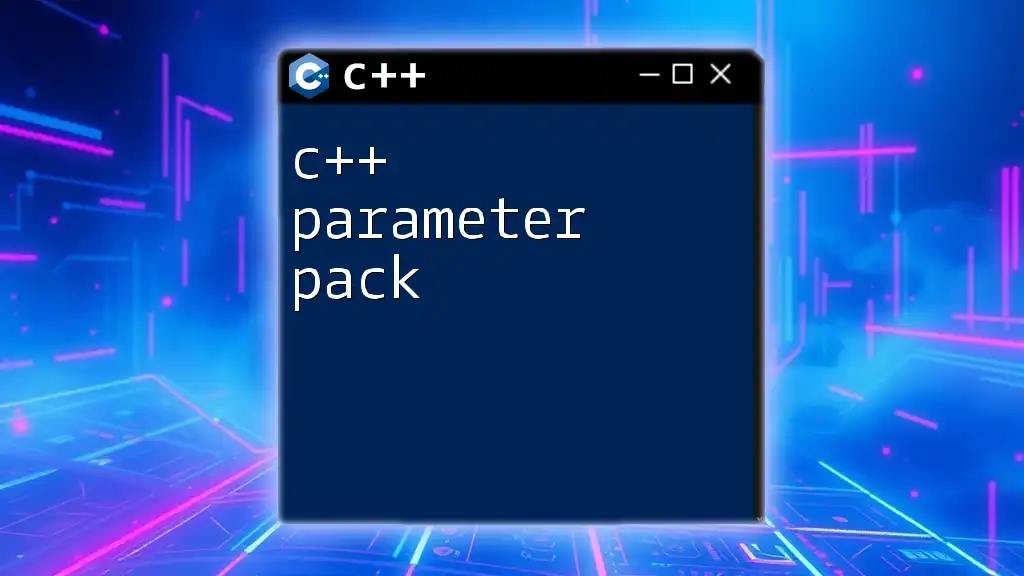
Additional Resources
For those eager to delve deeper into C++ and the intricacies of reference parameters, consider exploring resources such as programming textbooks, online courses, and interactive coding platforms that offer practice opportunities.
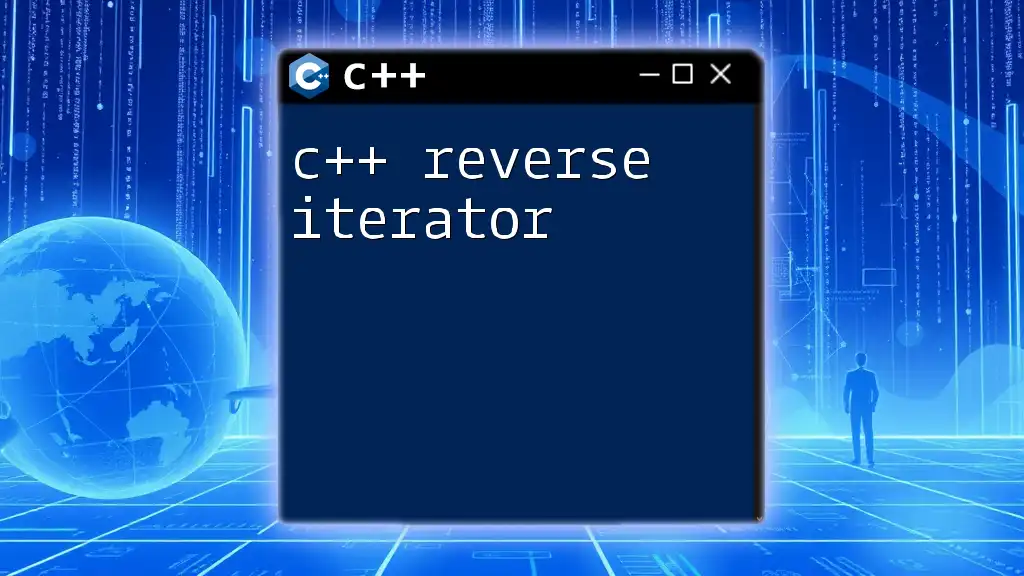
Call to Action
Join us to enhance your C++ programming skills! Explore our community forums, sign up for hands-on courses, and be part of a network that thrives on knowledge and skill-sharing. Your journey into the world of efficient coding begins now!