In C++, a parameter is a variable in a function definition that allows you to pass data into the function when it is called.
#include <iostream>
void greet(const std::string& name) { // 'name' is a parameter
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
greet("Alice"); // Passing the argument "Alice" to the function
return 0;
}
What are Parameters?
Parameters are a fundamental concept in C++ that allow developers to pass information into functions and methods. A parameter acts as a placeholder for the value that will be provided when the function is called. By utilizing parameters, programmers can create flexible and reusable code that simplifies complex operations.
When you define a function, the parameters specify what types of arguments the function can accept, ensuring that the function behaves correctly when invoked with different inputs.
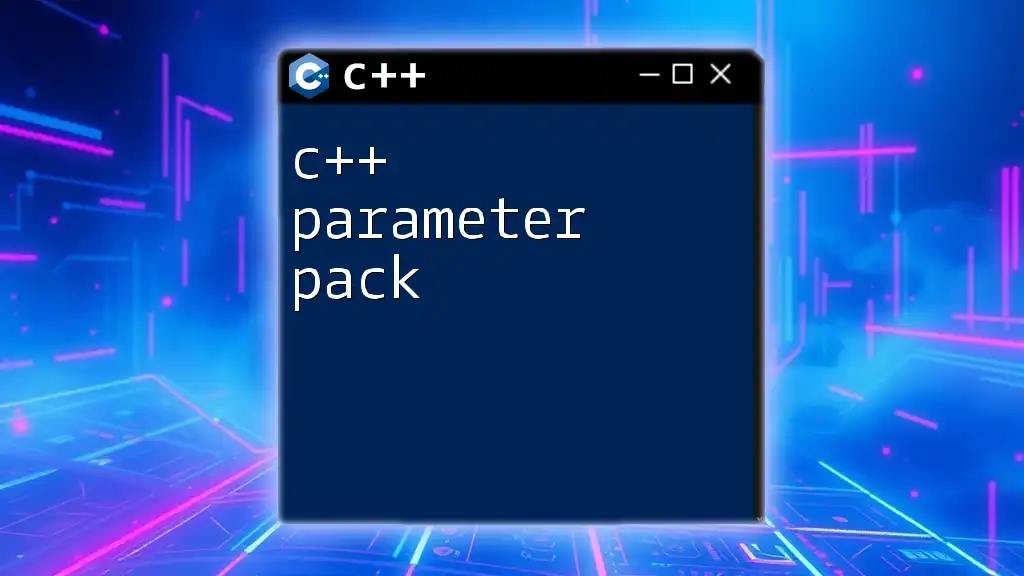
Enhancing Code Reusability
One of the key advantages of using parameters is the ability to enhance code reusability. When you define a function with parameters, you allow it to operate on different data without altering the function body. This means you can use the same function for various inputs without duplicating code, which not only makes your code cleaner but also reduces the risk of errors.
For instance, consider the function below:
void displayValue(int value) {
std::cout << "Value: " << value << std::endl;
}
This function can be called with any integer, making it highly reusable:
displayValue(5);
displayValue(10);
displayValue(-3);
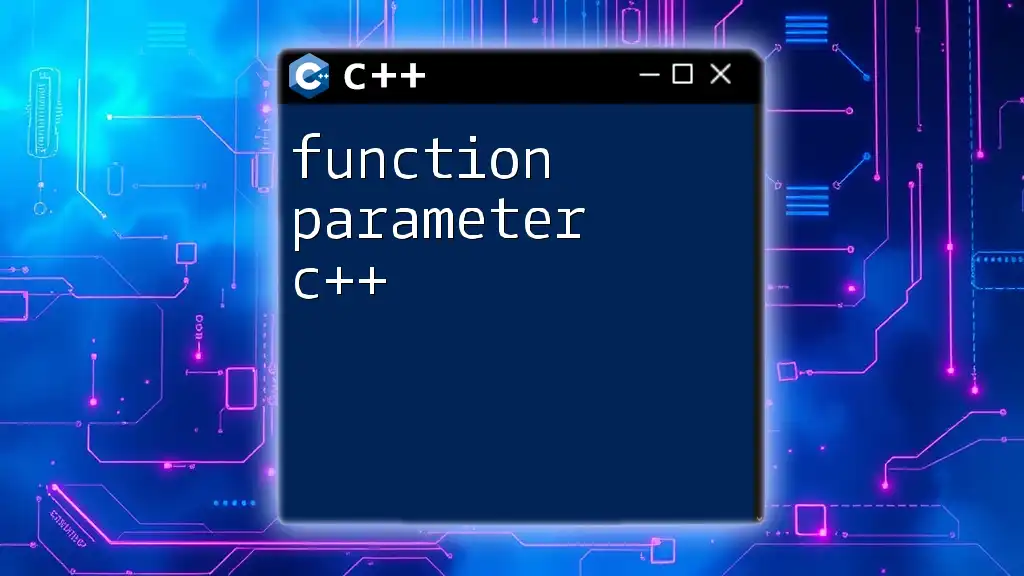
Improving Code Readability
Using descriptive and meaningful parameter names significantly improves code readability. When other developers (or even your future self) look at your code, clear parameter names help convey the purpose and expected types of inputs, making it easier to understand how functions operate.
For example, instead of using a generic name like `x` for a parameter, using `temperatureCelsius` is far more informative.
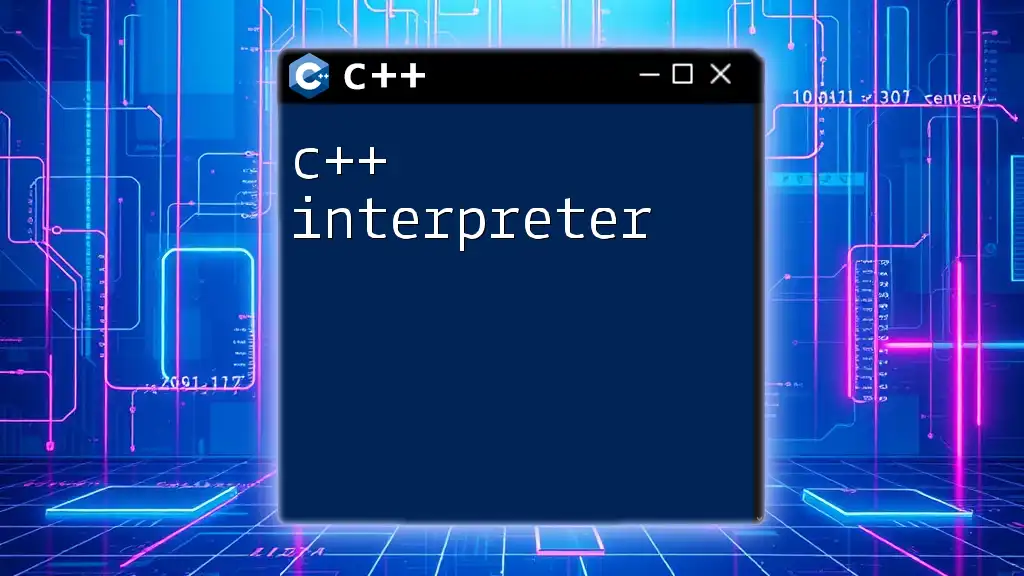
Types of Parameters in C++
Value Parameters
Value parameters are the simplest type of parameters. When you pass a variable to a function as a value parameter, the function receives a copy of the variable. Any modifications made to the parameter within the function do not affect the original variable.
void increment(int value) {
value++; // This modifies the copy of the variable, not the original
}
Reference Parameters
Reference parameters allow functions to operate directly on the original variables rather than on copies. They are defined using the ampersand (`&`) symbol. This enables the function to modify the passed variable.
void increment(int &number) {
number++; // This modifies the original variable
}
int main() {
int n = 10;
increment(n);
std::cout << "Incremented value: " << n << std::endl; // Outputs 11
}
Using reference parameters can be particularly beneficial when working with large data structures because it avoids the overhead of copying.
Pointer Parameters
Pointer parameters introduce another level of flexibility. When a function accepts a pointer parameter, it can manipulate the memory address of the original variable.
void setValue(int *ptr) {
*ptr = 20; // Modify the value at the memory address pointed by ptr
}
int main() {
int number = 10;
setValue(&number); // Passing the memory address of number
std::cout << "Set value: " << number << std::endl; // Outputs 20
}
Pointer parameters are often used in data structures and algorithms requiring dynamic memory management.
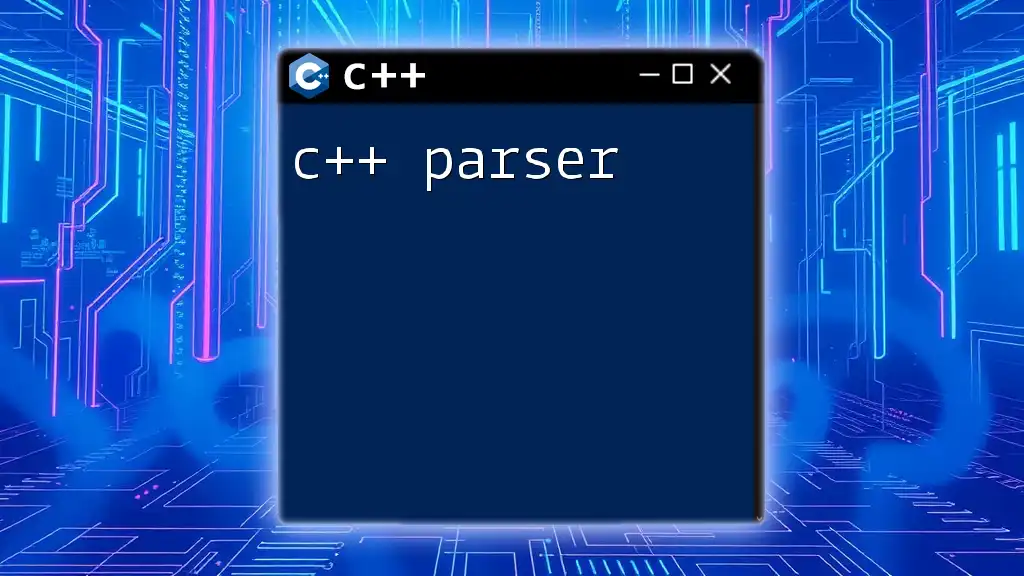
Default Parameters
Defining Default Parameters
C++ supports default parameters, which allow developers to define a default value for a parameter. When the function is called without an argument, the default value is used.
void printMessage(std::string message = "Hello, World!") {
std::cout << message << std::endl;
}
This means you can call the function with or without a parameter:
printMessage("Custom Message"); // Outputs: Custom Message
printMessage(); // Outputs: Hello, World!
When to Use Default Parameters
Using default parameters is beneficial when you want to provide commonly used values while still allowing for customization. They can simplify function calls and make your code less verbose.
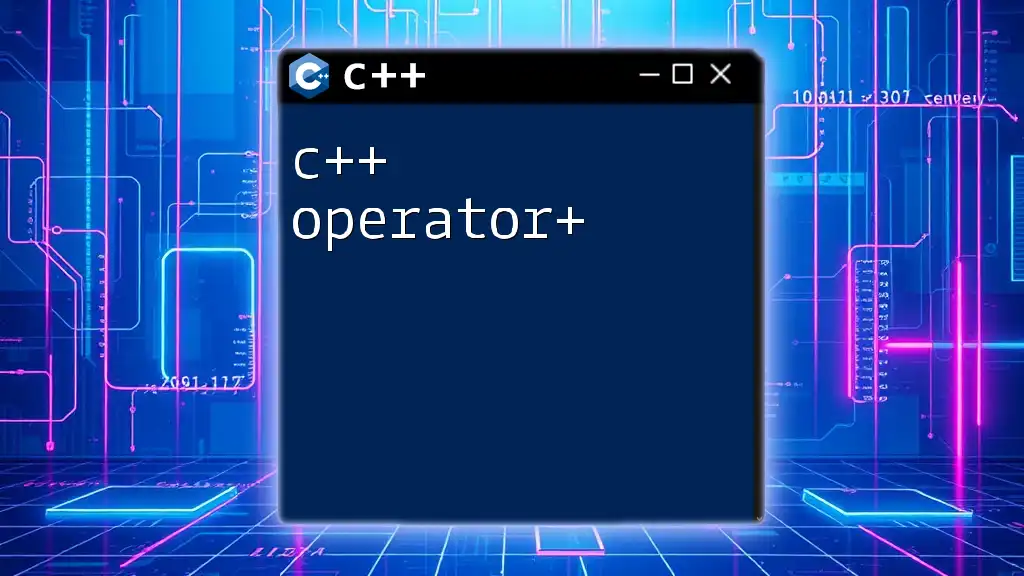
Parameter Passing Techniques
Pass by Value
When you pass a parameter by value, only a copy of the variable is made, which can be quickly processed. While this method ensures the original variable remains unchanged, it can lead to performance inefficiencies if the data structure is large.
Pass by Reference
Passing by reference retains the efficiency of accessing the original variable while allowing modifications directly to that variable. This is particularly useful in cases where you need to return multiple values from a function or modify a large struct or class.
Pass by Pointer
Passing by pointer provides even more control over memory and data manipulation. It is crucial in situations where dynamic memory management or data structures like linked lists are utilized. Understanding when to use pointers versus references is essential for effective C++ programming.
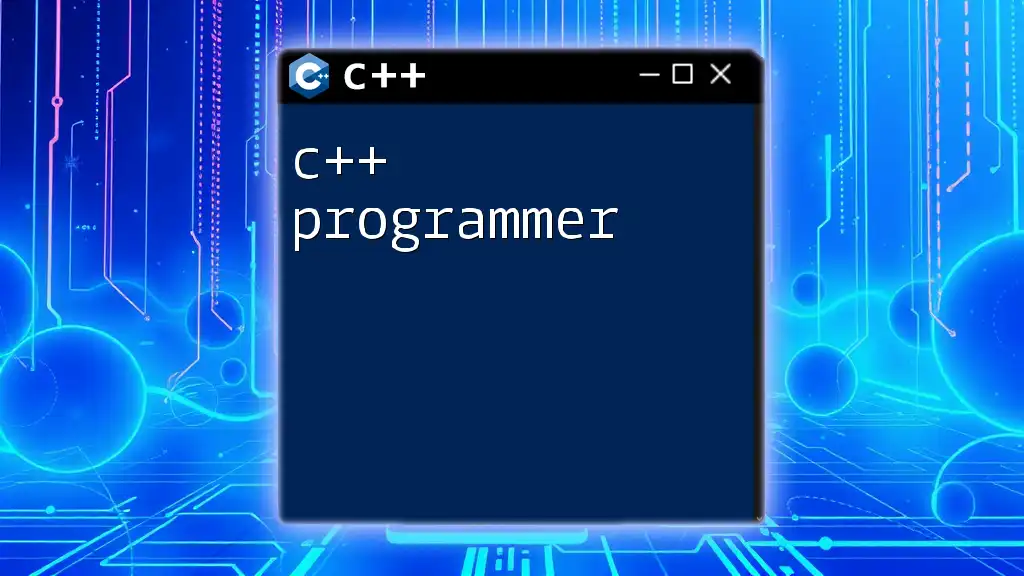
Common Mistakes with Parameters
Misunderstanding Value vs Reference
One common pitfall for beginners involves confusing value and reference parameters. Remember, changes made to value parameters will not affect the original variable, while changes made to reference parameters will. This can lead to unintended consequences in your code if not properly understood.
Dangling Pointers
Another critical issue is dealing with dangling pointers, which occur when a pointer references a memory location that has been freed or deallocated. This can lead to undefined behavior in your program. Always ensure that your pointers are valid before dereferencing them or make use of smart pointers for safer memory management.
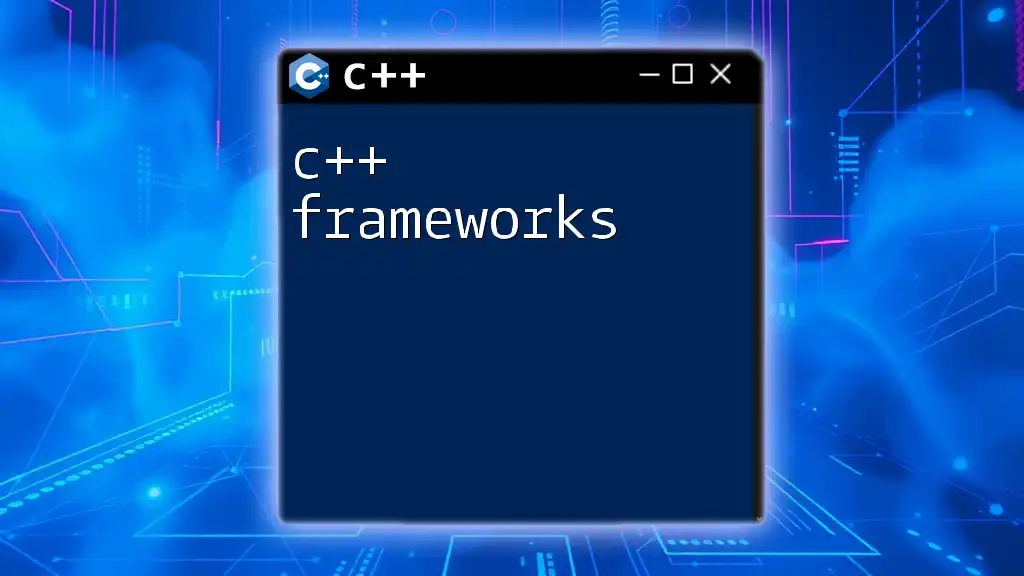
Function Overloading with Parameters
Function overloading enables multiple functions to share the same name if their parameter lists differ in the number or type of parameters. This is an effective way to provide different functionalities based on the types of inputs.
void calculate(int a, int b) {
std::cout << "Sum: " << a + b << std::endl;
}
void calculate(double a, double b) {
std::cout << "Product: " << a * b << std::endl;
}
By using function overloading, C++ allows for intuitive code while maintaining distinct functionality for different input types.
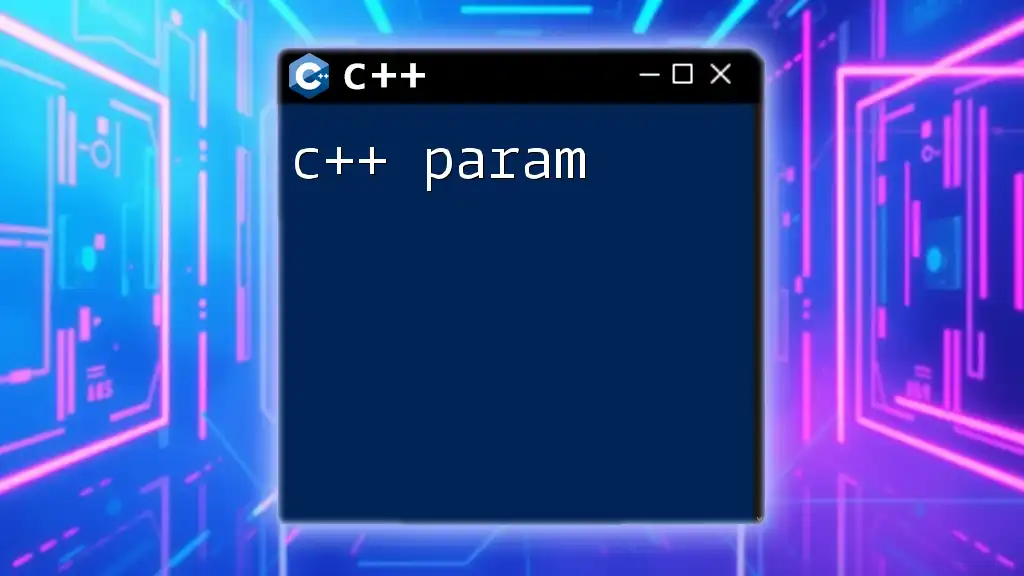
Final Thoughts on C++ Parameters
In summary, understanding how C++ parameters work and how to use them effectively is essential for any programmer looking to master C++. They enhance code reusability, readability, and flexibility, making it easier to write efficient and maintainable code.
Encouragement to Practice
Take the time to practice using different types of parameters in your functions. Experiment with default parameters, understand the differences between passing by value, reference, and pointer, and always keep an eye out for common pitfalls along the way.
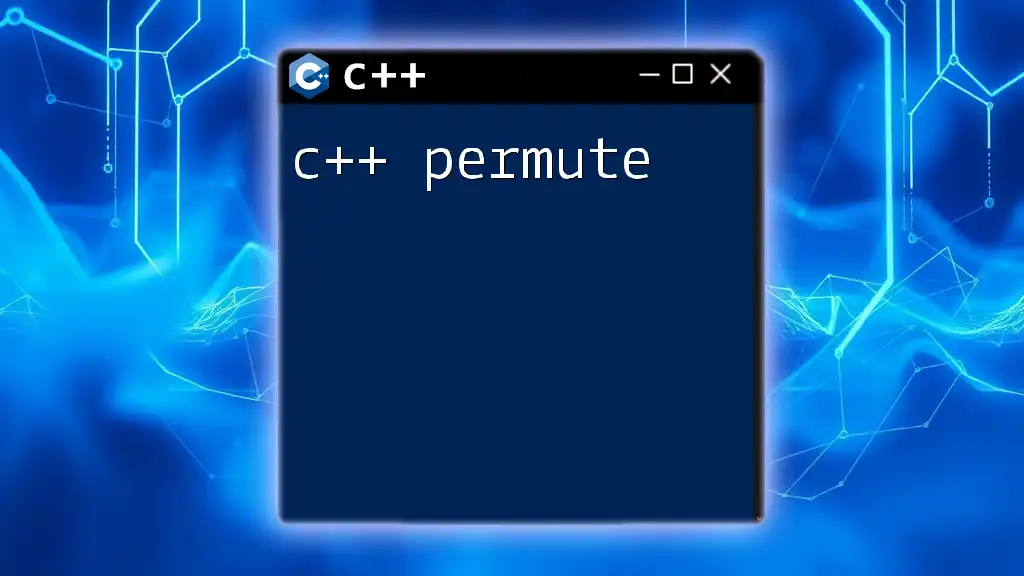
Additional Resources
If you're eager to deepen your understanding of C++ parameters, consider exploring books and online courses tailored specifically to C++ programming. Engaging with community forums can also provide support and opportunities to ask questions as you continue your coding journey.