In C++, the `...` syntax (known as variadic arguments) allows functions to accept an indefinite number of arguments, enabling more flexible function signatures. Here’s a simple example:
#include <iostream>
#include <cstdarg>
void printArgs(int count, ...) {
va_list args;
va_start(args, count);
for(int i = 0; i < count; ++i) {
int arg = va_arg(args, int);
std::cout << arg << " ";
}
va_end(args);
std::cout << std::endl;
}
int main() {
printArgs(3, 10, 20, 30); // Output: 10 20 30
return 0;
}
What are args in C++?
In C++, args refer to command-line arguments that provide a way to pass information to your program when it is executed. Understanding and effectively using these arguments is crucial, especially for applications that require user input right at the start.
`argc` (Argument Count) and `argv` (Argument Vector) are the two primary components that help manage command-line arguments. `argc` counts how many arguments are passed, including the program name itself. `argv` is an array of character pointers (strings) that hold the actual arguments.
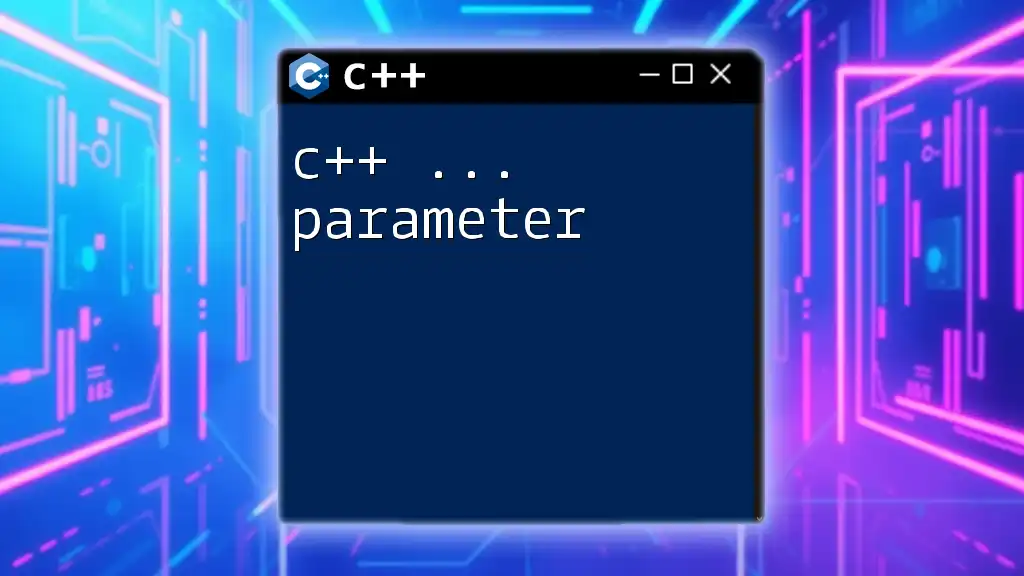
How Command-Line Arguments Work
The command-line arguments allow users to interact with your program by passing inputs at runtime. The central idea is that you run a program in the console, typing out `./program arg1 arg2 ...`, where `arg1`, `arg2`, etc., are the arguments your program can manipulate.
For instance, executing a program like this:
./myprogram Hello World
passes "Hello" as `argv[1]` and "World" as `argv[2]`.
Basic Syntax and Structure
To access command-line arguments in C++, you typically define the `main` function as follows:
int main(int argc, char *argv[]) {
// Code here
}
- argc: An integer representing the number of command-line arguments.
- argv: An array of C-style strings (char arrays) containing the actual arguments.
Here is a simple example illustrating how to retrieve and print all the arguments:
#include <iostream>
int main(int argc, char *argv[]) {
for (int i = 0; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
This snippet prints all the provided command-line arguments, enabling you to see what has been passed when you execute the program.
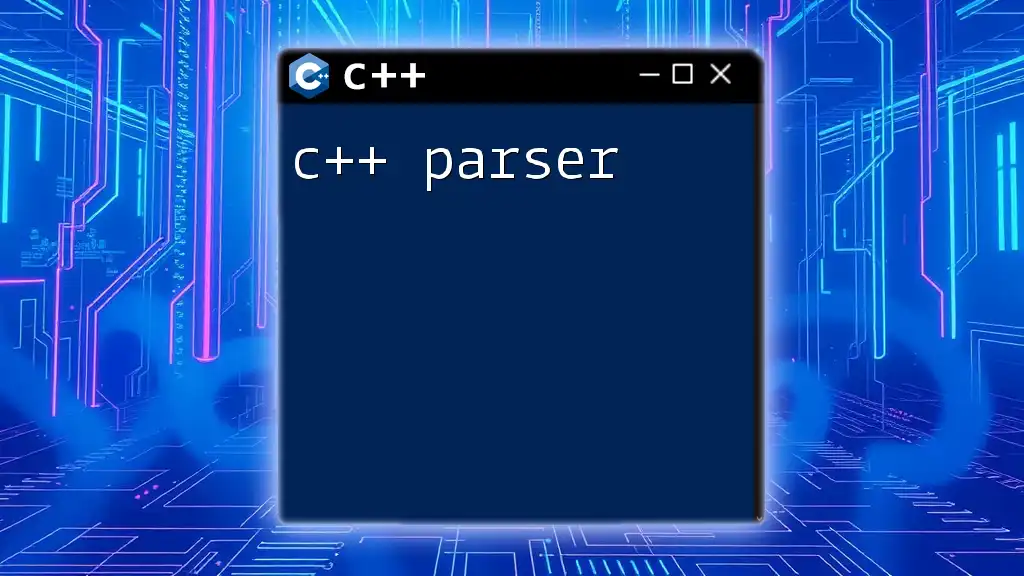
Using Args in C++
Accessing Command-Line Arguments
To use command-line arguments effectively, you need to know how to access them within your program. Using the `argv` array, you can access any argument by its index.
If you want to print only the first argument (after the program name), you can use the following code:
#include <iostream>
int main(int argc, char *argv[]) {
if (argc > 1) {
std::cout << "First argument: " << argv[1] << std::endl;
} else {
std::cout << "No arguments provided." << std::endl;
}
return 0;
}
Data Types and Conversion
It’s common to need numeric values from command-line arguments. Since arguments are received as strings, conversion is required. C++ offers several functions for this, such as `std::stoi` (for integers) and `std::stof` (for floats).
Here's an example that demonstrates converting a string argument to an integer:
#include <iostream>
int main(int argc, char *argv[]) {
if (argc > 1) {
try {
int num = std::stoi(argv[1]); // Converts first argument to integer
std::cout << "You entered the number: " << num << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument: Please provide a valid number." << std::endl;
return 1;
}
}
return 0;
}
In this example, we gracefully handle the possibility of invalid input by utilizing a `try-catch` block.
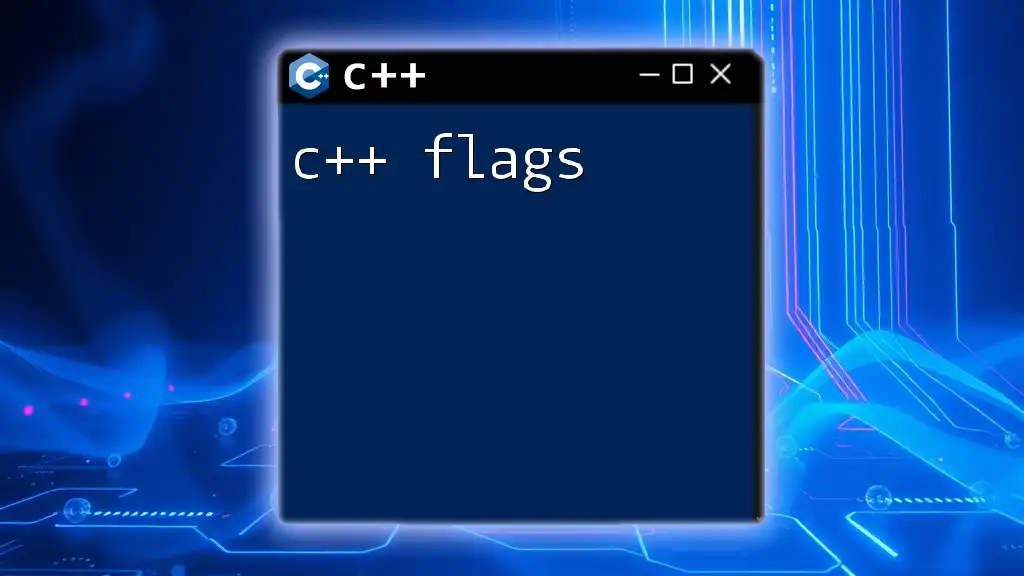
Advanced Usage of Args
Handling Multiple Arguments
C++ allows you to pass multiple arguments, and managing them can enhance program interactivity. A practical example is a simple calculator that performs different operations based on user-supplied arguments.
For instance, if the arguments provided are `add 2 3`, you can parse these to perform the specified operation.
#include <iostream>
#include <string>
int main(int argc, char *argv[]) {
if (argc != 4) {
std::cerr << "Usage: " << argv[0] << " <operation> <num1> <num2>" << std::endl;
return 1;
}
std::string operation = argv[1];
int num1 = std::stoi(argv[2]);
int num2 = std::stoi(argv[3]);
if (operation == "add") {
std::cout << "Result: " << (num1 + num2) << std::endl;
}
// Additional operations can be handled similarly
return 0;
}
Tips for Effective Argument Parsing
To manage and parse command-line options more efficiently, consider using libraries such as `getopt` or `argparse`. These tools simplify the process of defining and handling command-line options, making your code cleaner and more user-friendly.
Another effective tactic is to organize complex arguments using data structures, such as maps. This approach fosters flexibility in handling a variety of commands and options.
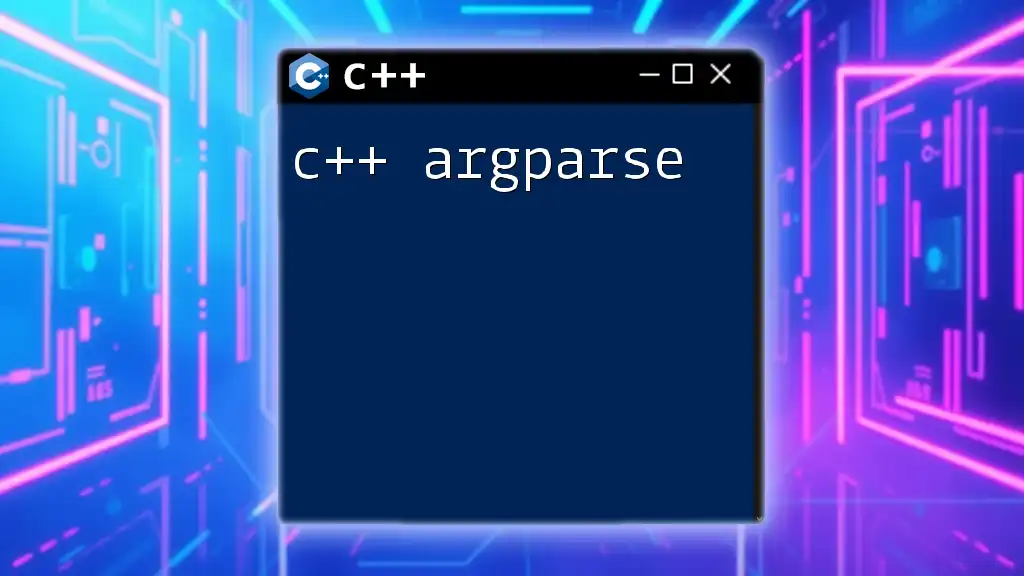
Common Pitfalls with Command-Line Args
Errors and Exception Handling
When dealing with command-line arguments, robust error handling is essential. Users may input unexpected data that can cause your program to crash. Therefore, always validate and sanitize inputs before processing them.
Here's how you could incorporate error-checking:
try {
int num = std::stoi(argv[1]);
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument: Please provide a valid number." << std::endl;
return 1;
}
Overcoming Limitations
C++ interacts with the operating system, which imposes limits, such as the maximum number of allowed arguments. Keeping track of system specifications can help you design better, user-friendly applications.
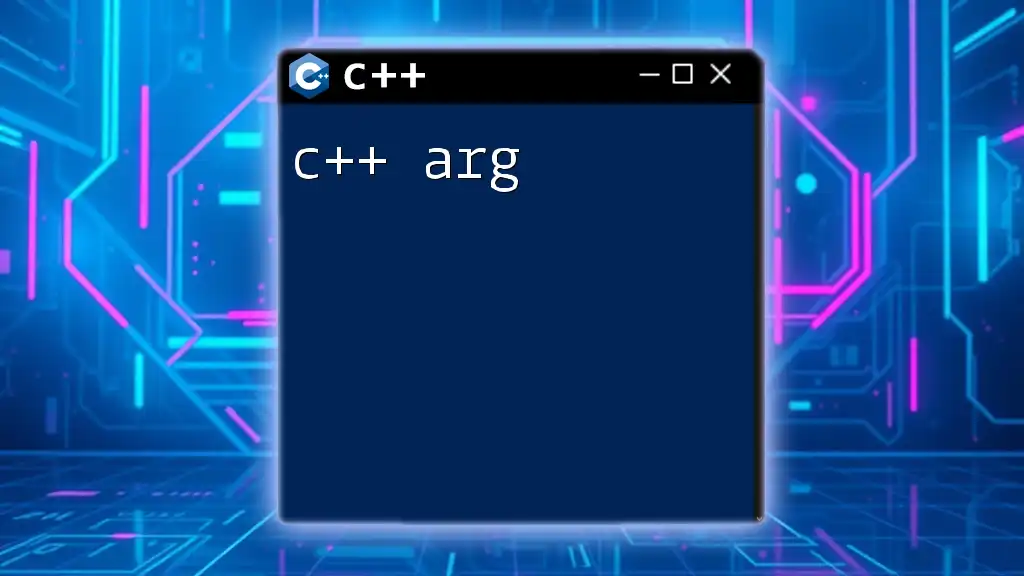
Real-World Applications of Args in C++
Using Args in File Processing
A practical application of command-line arguments might involve processing files. By passing a filename as an argument, you can control which file your program reads or modifies:
#include <iostream>
#include <fstream>
int main(int argc, char *argv[]) {
if (argc < 2) {
std::cerr << "Usage: " << argv[0] << " <filename>" << std::endl;
return 1;
}
std::ifstream file(argv[1]);
if (!file) {
std::cerr << "Could not open the file: " << argv[1] << std::endl;
return 1;
}
// File processing logic here
return 0;
}
Creating Flexible CLI Applications
Command-line interfaces (CLI) are a powerful way to enable dynamic and flexible applications. By leveraging command-line arguments, you can create programs that are not only user-interactive but also capable of executing a wide variety of tasks based on user input. This approach encourages versatility and could lead to more efficient usage of your program.
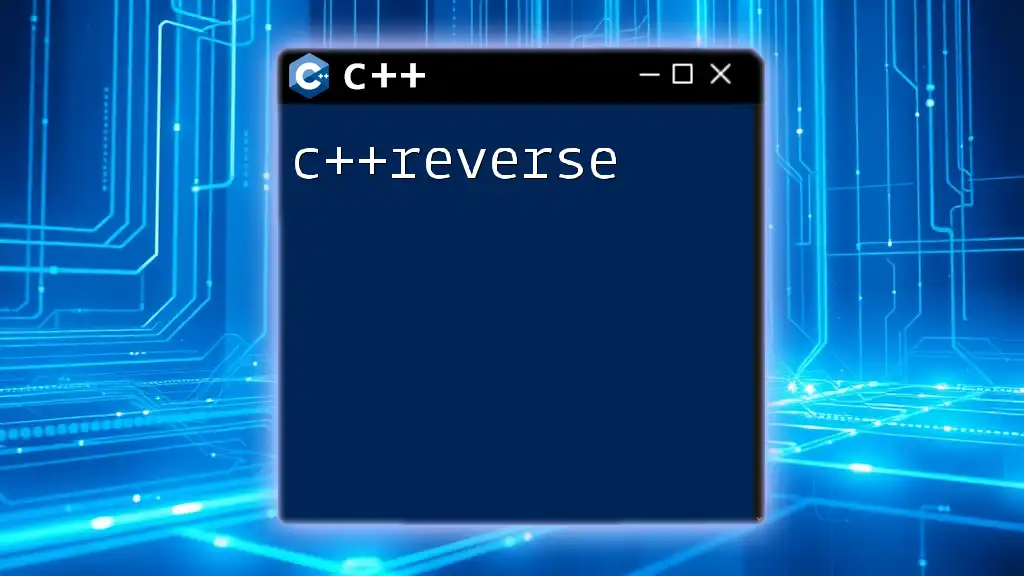
Conclusion
Understanding how to work with args in C++ opens up a world of possibilities for creating complex applications that require user input from the outset. By utilizing `argc` and `argv`, converting argument types, handling errors, and applying these concepts to real-world applications, you can significantly enhance the functionality and user experience of your programs.
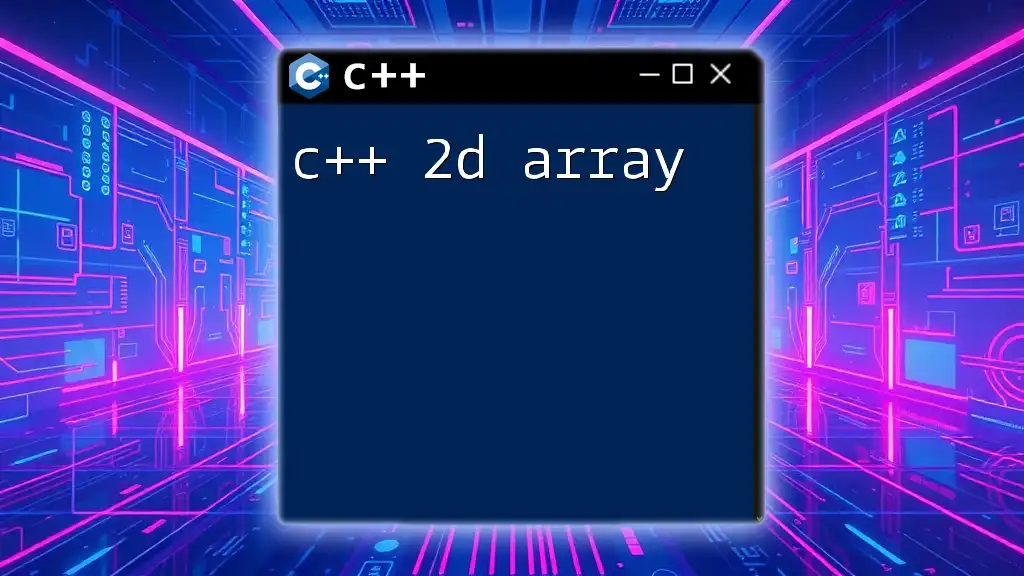
Additional Resources
Engaging with additional reading materials, online courses, and community discussions can further your understanding of using command-line arguments in C++. Aspiring developers are encouraged to practice by crafting small projects that utilize command-line arguments effectively.