C++ flags are compiler options that modify the behavior of the compilation process, allowing developers to optimize code, enable warnings, or define preprocessor directives.
g++ -O2 -Wall -std=c++11 my_program.cpp -o my_program
Understanding Compiler Options
What are Compiler Options?
Compiler options are command-line arguments that you can pass to a C++ compiler to change its behavior. They allow developers to enable certain features, modify the optimization process, specify required standards, and manage warnings or debugging information. C++ flags are specific types of compiler options that provide fine-grained control over the compilation of your code.
Common Compiler Options in C++
Different C++ compilers come with various flags and options. Among the most popular compilers are GCC (GNU Compiler Collection), Clang, and MSVC (Microsoft Visual C++). While many flags are universal, some might differ between these compilers, so it’s essential to refer to the documentation specific to the one you are using.

Types of C++ Flags
Optimization Flags
Optimization flags dictate how the compiler optimizes the generated output for performance. They can significantly impact your program's speed and size.
For example, using the following command enables level 2 optimization, which balances performance with reasonable compilation time:
g++ -O2 myfile.cpp -o myfile
The optimization levels include:
- O0: No optimization (default)
- O1: Minimal optimization that does not impede compilation time significantly.
- O2: Recommended level that increases the speed of your program without significantly increasing compilation time.
- O3: Aggressive optimization, which may include more complex techniques, potentially leading to longer compile times.
- Os: Optimize for size, reducing the size of the generated binary.
- Ofast: Enable optimizations that are not strictly compliant with the C++ standard.
Warning Flags
Warnings are crucial during development because they help catch potential issues in your code. Using warning flags allows you to receive feedback about your code quality and adherence to best practices.
For instance, the command below activates a comprehensive set of warning messages:
g++ -Wall -Wextra myfile.cpp -o myfile
- -Wall: Enables most warning messages.
- -Wextra: Activates additional warnings that are not included in `-Wall`.
- -Werror: Treats warnings as errors, forcing your code to be warning-free before it compiles.
Debugging Flags
Debugging flags are essential for creating executables that contain information useful for debugging. When you need to troubleshoot issues in your application, adding debugging information can save valuable time.
To include debugging information, you can use:
g++ -g myfile.cpp -o myfile
This flag generates a binary that contains symbols for the debugger, allowing you to inspect variable values and control execution flow during runtime.
Standard Flags
C++ has evolved through various standards, and using the correct standard flag is essential to ensure your code is compatible with your compiler. The command below instructs the compiler to adhere to the C++17 standard:
g++ -std=c++17 myfile.cpp -o myfile
Understanding the available standards is critical, as different features have been introduced in various C++ versions. These include C++11, C++14, C++17, and C++20, each offering new capabilities and optimizations.
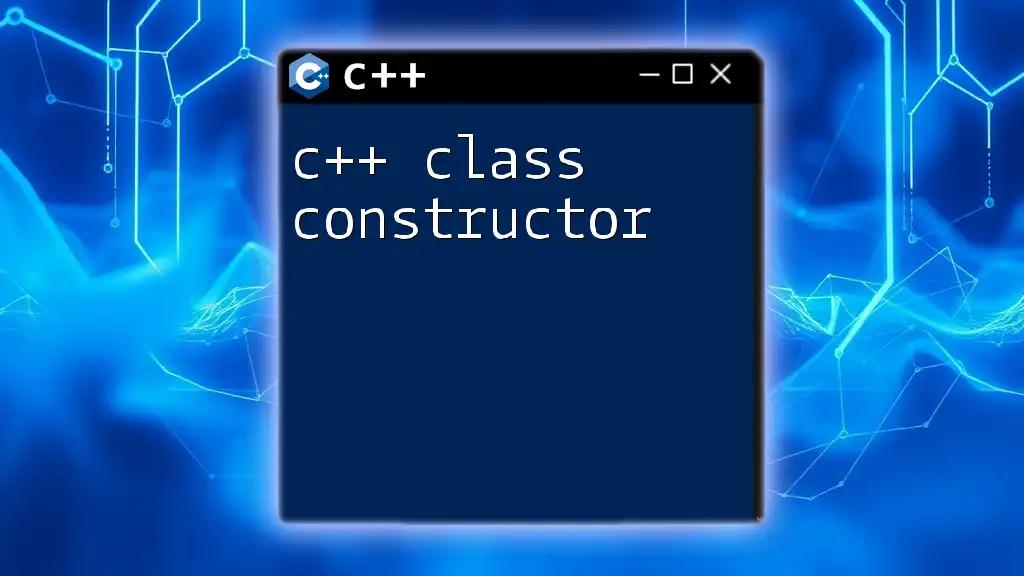
Using C++ Flags Effectively
Best Practices for Using Flags
When working with C++ flags, it's important to choose wisely. Start with a base set of flags and gradually add more as needed. Balancing optimization, compilation speed, and flag complexity is vital. Often, using a combination of flags makes for an effective development process. For example, you might combine optimization with debugging:
g++ -O2 -g -std=c++17 myfile.cpp -o myfile
This command compiles your code with optimization, includes debugging information, and adheres to the C++17 standard.
Creating a Makefile with C++ Flags
Makefiles simplify the build process by allowing a single command to compile your entire project. By specifying the flags you commonly use, you can streamline your workflow.
Here's a basic example of a Makefile that incorporates relevant flags:
CXX = g++
CXXFLAGS = -Wall -O2 -g
all: myapp
myapp: main.o utils.o
$(CXX) $(CXXFLAGS) -o myapp main.o utils.o
clean:
rm -f *.o myapp
In this Makefile, you set the compiler (CXX) and the flags (CXXFLAGS). It specifies how to create the final executable from object files and includes a clean target to remove generated files.
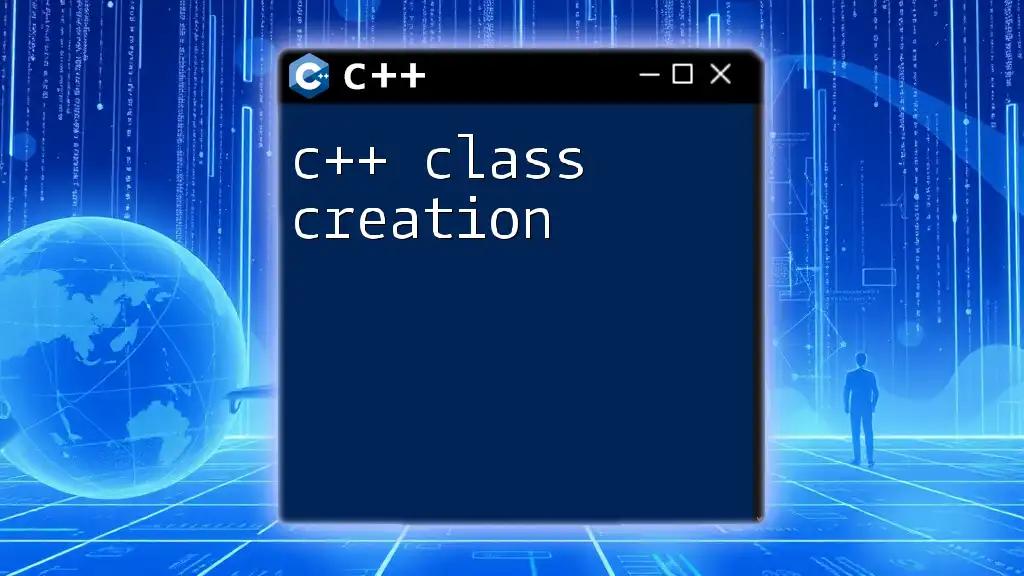
Advanced Flag Usage
Combining Multiple Flags
It's often necessary to combine multiple flags to achieve the desired behavior from your compiler. This can enhance performance, improve debugging, and maintain standards compliance all at once.
An example command that combines various flags is:
g++ -Wall -O2 -g -std=c++17 myfile.cpp -o myfile
Here, several critical flags work together for a comprehensive outcome.
Platform-Specific Flags
Using platform-specific flags ensures that your application runs smoothly on different environments. For example, some flags can help define preprocessor directives that adjust code based on the compilation context.
An example command that uses a preprocessor directive for debugging is:
g++ -D_DEBUG myfile.cpp -o myfile
This directive instructs the compiler to include debugging code within your application, facilitating easier troubleshooting on specific platforms.

Conclusion and Next Steps
Understanding and utilizing C++ flags is vital for optimizing your software development process. By effectively applying these flags, you not only enhance performance but also maintain code purity and improve your debugging efforts.
Experiment with various combinations of flags in your projects, and consider joining advanced C++ courses or resources to deepen your knowledge. The right use of flags can significantly elevate your coding capabilities.
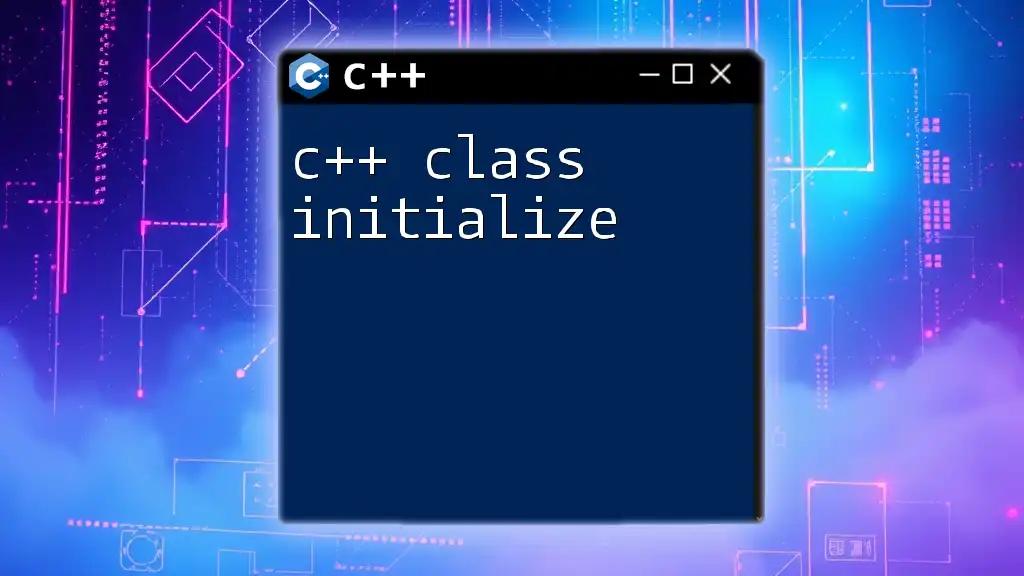
Additional Resources
For those looking to expand their understanding further, several resources are available:
- Official documentation for respective C++ compilers
- Online coding forums and communities dedicated to C++
- Books focusing on advanced C++ programming techniques
By continually learning about C++ flags and their applications, you can refine your skills and write more efficient, reliable code.