A C++ flat_set is a sorted, unique collection of elements that maintains its elements in a contiguous memory structure, providing fast access and minimal memory overhead.
Here's a code snippet demonstrating the use of a flat_set:
#include <iostream>
#include <boost/container/flat_set.hpp>
int main() {
boost::container::flat_set<int> flatSet;
// Inserting elements
flatSet.insert(3);
flatSet.insert(1);
flatSet.insert(2);
// Displaying elements
for (const auto& element : flatSet) {
std::cout << element << " ";
}
return 0;
}
What is a Flat Set?
A C++ flat set is a type of container that implements a set structure but stores its elements in a sorted sequence, primarily utilizing a contiguous memory structure, typically a vector. Its characteristics include ordered, unique elements, and efficient search capabilities, making it distinct from other associative containers in C++ like `std::set` or `std::unordered_set`.
The flat set is often preferred in scenarios where the set needs to support frequent read operations. The sorted nature allows for efficient binary searches, while the contiguous storage offers benefits like improved cache locality.
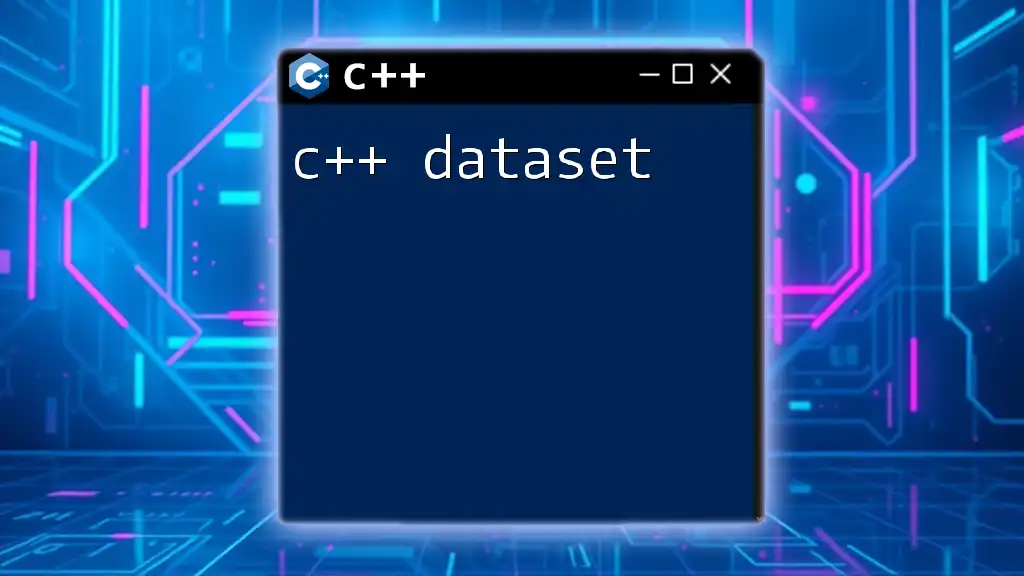
Importance of Using Flat Sets in C++
Utilizing a C++ flat set can lead to significant performance benefits in specific applications. The contiguous memory model means that when elements are stored linearly, accessing them leverages CPU caching more efficiently, reducing access time.
Flat sets are particularly useful in applications where it is essential to maintain a unique collection of items that often require sorting, such as in algorithms that require frequent searching, merging, or deductive operations.
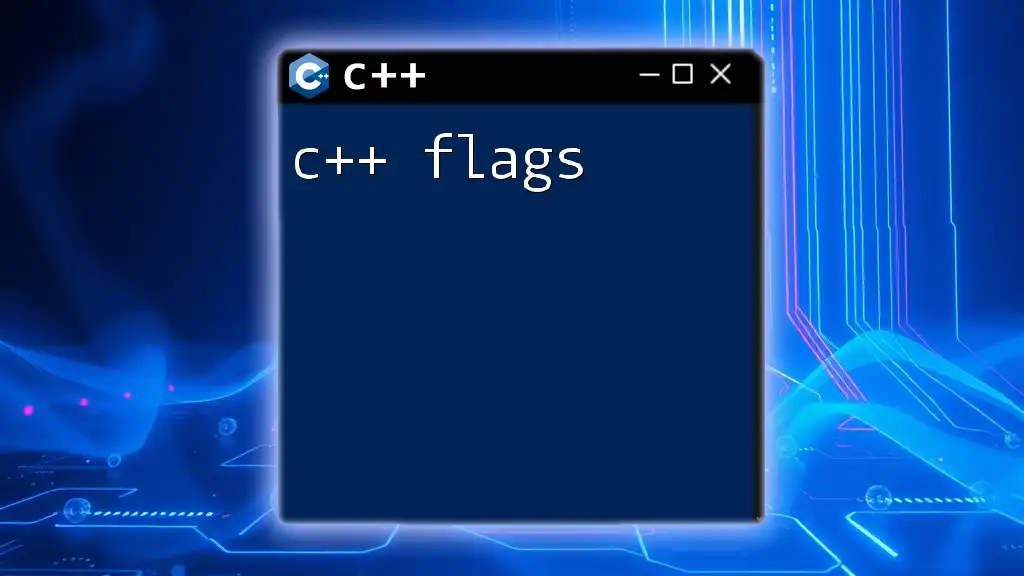
Key Features of C++ Flat Set
Contiguous Storage
The main feature of a C++ flat set is its use of contiguous storage through STL vectors. Contiguous storage offers both memory efficiency and performance benefits, particularly in scenarios involving large data sets. This structure minimizes memory fragmentation and allows for efficient use of CPU cache, resulting in faster access times as nearby data elements can be fetched with fewer cache misses.
Sorted Elements
Maintaining order is essential in a flat set. Elements in a flat set are maintained in a sorted state, which justifies the need for sorting operations during insertion and merging. This ordered nature not only accelerates search operations using binary search algorithms, which have a time complexity of O(log n), but also ensures predictable iteration operations, as you'll always traverse elements in a specific order, improving overall code reliability.
Unique Elements
As with any set, a C++ flat set enforces the uniqueness of its elements. This aspect is managed during insertion by checking if an element already exists before adding it to the set. Consequently, duplicate elements are naturally filtered out, ensuring the integrity of data.
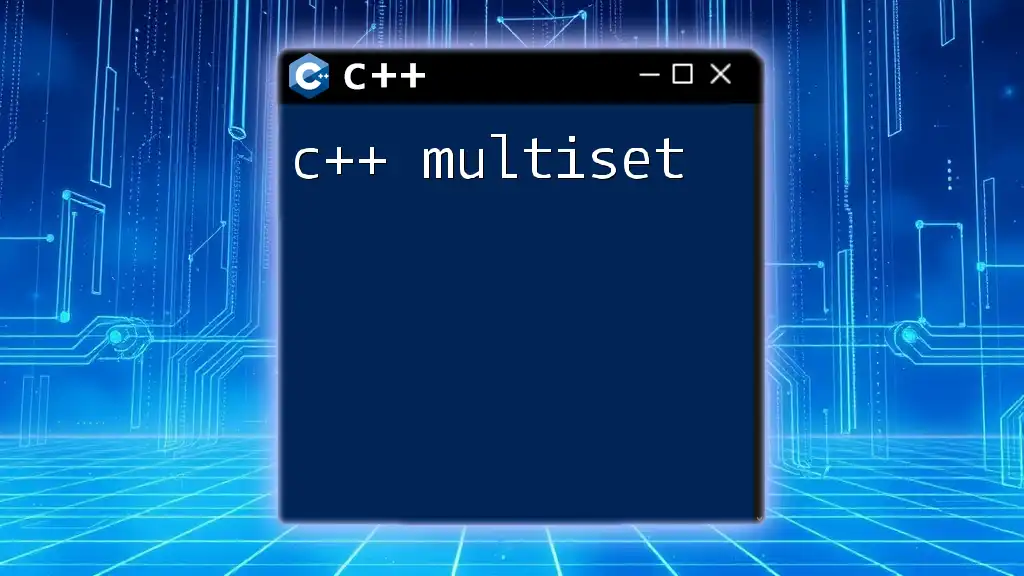
How to Implement C++ Flat Set
Including Required Headers
To get started with a C++ flat set, you will need to include the required headers:
#include <vector>
#include <algorithm>
#include <iostream> // For standard input/output operations
Basic Structure of a Flat Set
You can represent a flat set using a vector:
std::vector<int> flat_set; // Example of a flat set stored in a vector
Adding Elements to a Flat Set
Adding elements to the flat set involves checking for uniqueness and maintaining sorted order. Here’s an example function:
void addElement(std::vector<int>& flat_set, int element) {
if (std::find(flat_set.begin(), flat_set.end(), element) == flat_set.end()) {
flat_set.push_back(element);
std::sort(flat_set.begin(), flat_set.end()); // Keep it sorted
}
}
This method not only checks if the item already exists, but it also sorts the vector after each insertion, ensuring that the elements remain in order.
Removing Elements from a Flat Set
To support dynamic operations on the flat set, elements can be removed. Here's how you can do that while ensuring the integrity of the set:
void removeElement(std::vector<int>& flat_set, int element) {
flat_set.erase(std::remove(flat_set.begin(), flat_set.end(), element), flat_set.end());
}
This method searches for the element and removes it without leaving invalid entries behind.
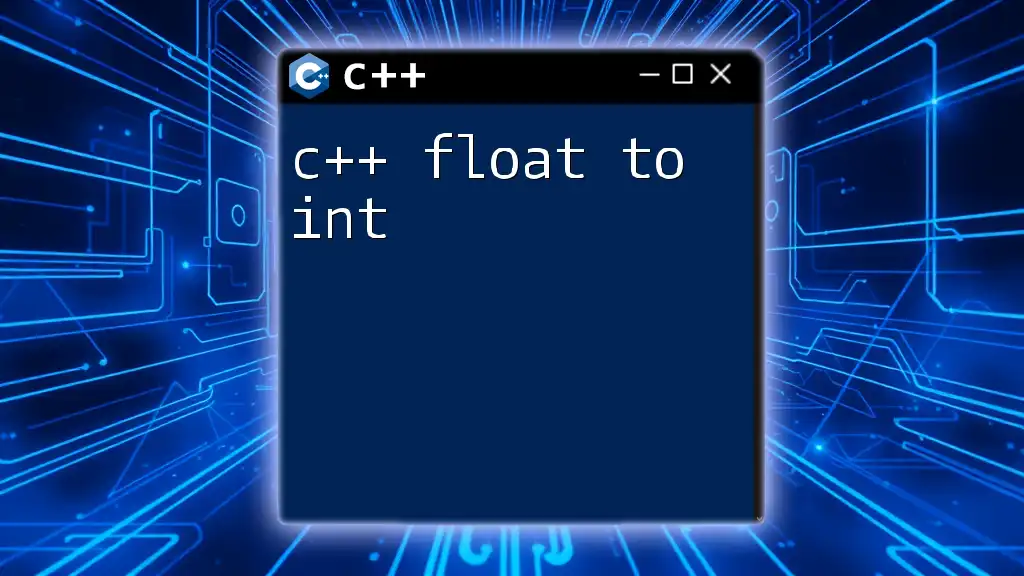
Operations on C++ Flat Set
Searching for Elements
Searching within a flat set can be optimized using binary search, thanks to the sorted nature of the elements:
bool contains(const std::vector<int>& flat_set, int element) {
return std::binary_search(flat_set.begin(), flat_set.end(), element);
}
This function provides an efficient way to check if an element exists in the flat set, leveraging O(log n) complexity due to binary search.
Iteration through a Flat Set
Iterating over elements in a C++ flat set is straightforward:
for (int elem : flat_set) {
std::cout << elem << ' ';
}
This loop will print each element in order, highlighting the predictability afforded by the sorted data structure.
Merging Two Flat Sets
Merging two flat sets while keeping the uniqueness and order intact can be achieved as follows:
std::vector<int> mergeFlatSets(const std::vector<int>& set1, const std::vector<int>& set2) {
std::vector<int> merged = set1;
merged.insert(merged.end(), set2.begin(), set2.end());
std::sort(merged.begin(), merged.end());
auto last = std::unique(merged.begin(), merged.end());
merged.erase(last, merged.end());
return merged;
}
This function combines the contents of both sets, sorts the merged set, and subsequently removes any duplicates.
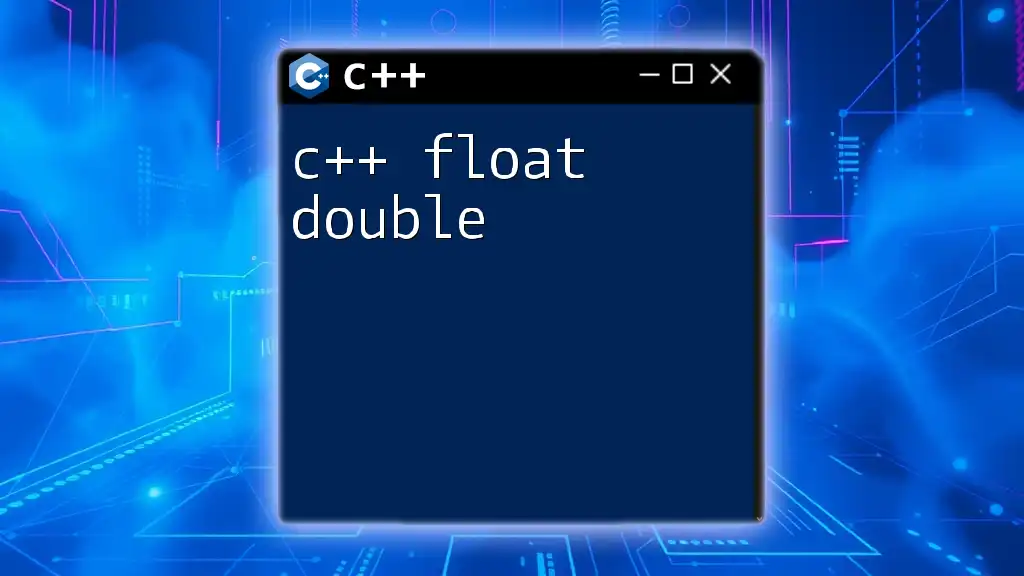
Performance Analysis of C++ Flat Set
Time Complexity of Operations
The time complexity of operations in a C++ flat set is vital to understand.
- Insertion: O(n log n) due to the need for sorting after each addition.
- Deletion: O(n) for searching and removing an element.
- Searching: O(log n) thanks to binary search.
When compared to other data structures like `std::set`, which offer logarithmic complexity for insertion and deletion, flat sets can be less efficient in scenarios requiring frequent write operations.
Space Complexity Considerations
The space complexity of a flat set emerges primarily from the vector that holds the elements. Because it uses contiguous storage, there is potential for greater efficiency, but one must also account for the overhead of memory allocation.
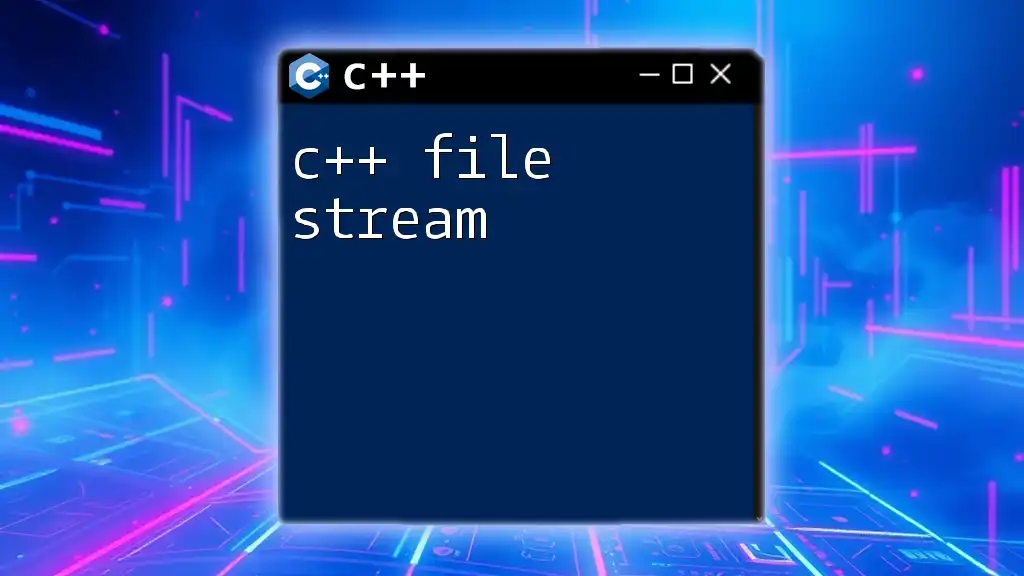
Best Practices for Using Flat Sets
Choosing the Right Use Case
Flat sets shine in applications focused on retrieval rather than modification. Use them in scenarios where:
- You expect more read operations than writes.
- You need sorted data.
- Unique elements are critical for logic or algorithms, such as in search algorithms or data deduplication processes.
Maintaining Performance
To ensure your C++ flat set maintains performance:
- Batch insertions can be performed before sorting when possible, reducing the frequency of costly sort operations.
- Avoid excessive deletions; consider using a persistent data structure if frequent removals are needed.
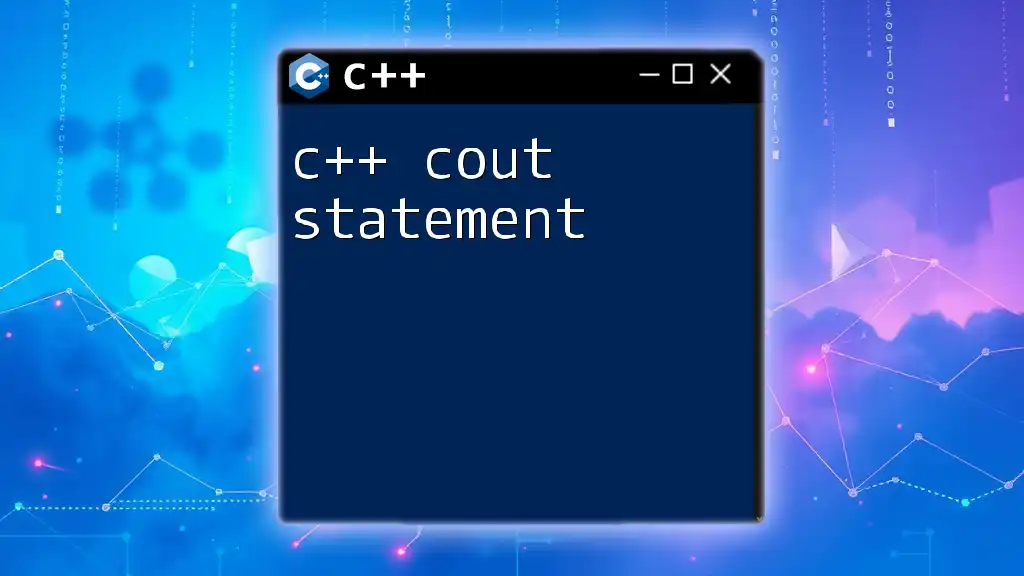
Conclusion
In summary, a C++ flat set provides a compelling balance of memory efficiency, search performance, and data integrity. Its implementation through vectors is straightforward and offers considerable advantages in specific contexts. Understanding when to use this structure can significantly enhance your program’s efficiency and performance.
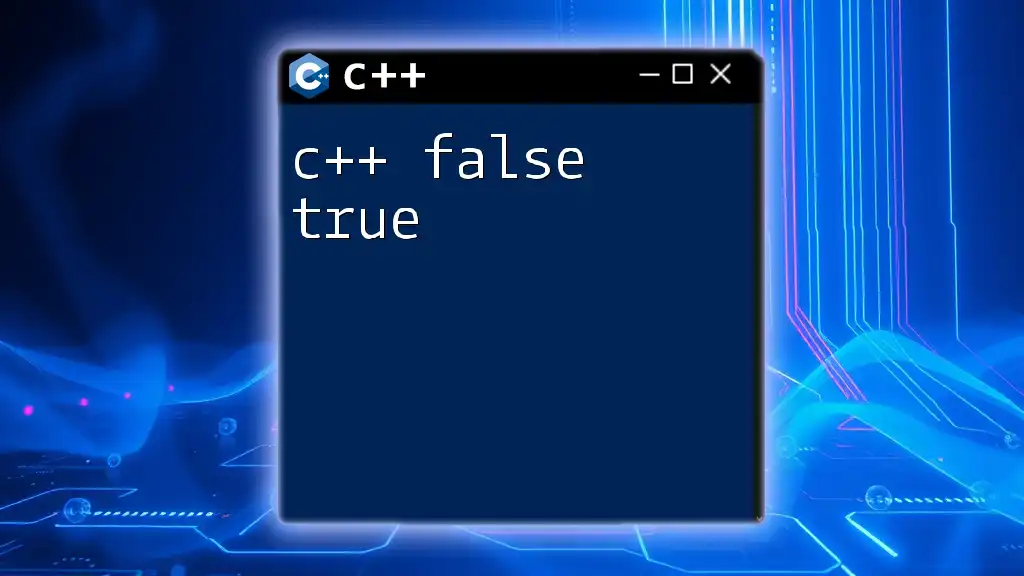
Additional Resources
For a deeper understanding of implementing C++ flat sets and improving your C++ skills, consider exploring various programming communities, textbooks, and online tutorials. Engaging with experts and enthusiasts can provide additional insights and foster a better learning environment.