The `for` statement in C++ is used to create a loop that executes a block of code a specified number of times, allowing for controlled iteration over a sequence or collection.
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
Understanding C++ Syntax for Loop
The C++ for statement is a foundational structure in C++ programming, allowing developers to repeat a block of code a set number of times. It generally consists of three main components: initialization, condition, and increment/decrement. The syntax can be understood through the following general format:
for(initialization; condition; increment/decrement) {
/* code block */
}
Initialization
The initialization part is executed only once, at the beginning of the loop. It typically declares and initializes the loop control variable(s). This is crucial for setting the starting point of the loop.
Condition
The condition is a boolean expression that is evaluated before each iteration of the loop. If it evaluates to `true`, the loop body is executed. If it evaluates to `false`, the loop exits. This condition essentially controls how long the loop will continue to run, making it a vital part of the loop's functionality.
Increment/Decrement
After each iteration of the loop, the increment/decrement statement is executed. This updates the loop control variable(s), advancing the loop towards its termination. It is important to ensure that this statement will eventually make the condition evaluate to `false`, thereby preventing infinite loops.
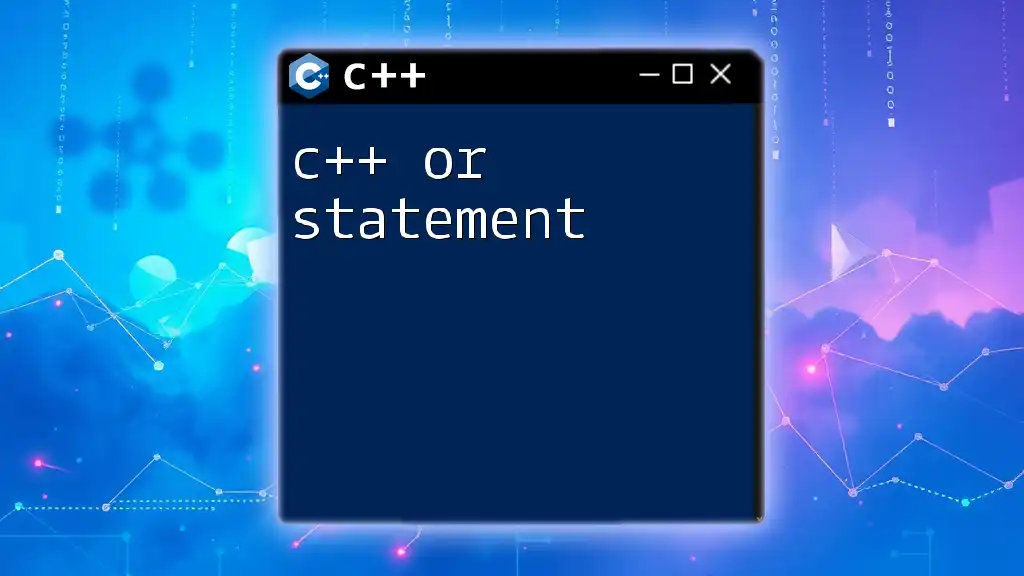
Variations of the For Statement in C++
Standard For Loop
The standard for loop is the most commonly used form. It allows for straightforward iteration, making it easy to manage the loop control variable. Here’s an example of a standard for loop that prints the numbers 0 through 9:
for(int i = 0; i < 10; i++) {
std::cout << i << " ";
}
In this example:
- `int i = 0`: initializes the variable `i` to `0`.
- `i < 10`: sets the condition that allows the loop to execute as long as `i` is less than `10`.
- `i++`: increments `i` by `1` after each iteration.
The output will be: `0 1 2 3 4 5 6 7 8 9`.
Range-Based For Loop
Introduced in C++11, the range-based for loop simplifies the iteration over collections such as arrays and vectors. It enhances code readability by removing the need for explicit indexes. Here’s how it works:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
In this example, `num` takes on the value of each element in the `numbers` vector sequentially. The output will be: `1 2 3 4 5`. This approach is particularly beneficial when dealing with complex containers, as it reduces the risk of accessing out-of-bounds elements.
Nested For Loops
Another significant variation is the nested for loop, where one loop is contained within another. This is often used when dealing with multi-dimensional data structures, such as matrices. Here’s an example:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 2; j++) {
std::cout << "i: " << i << ", j: " << j << std::endl;
}
}
This code will produce the following output:
i: 0, j: 0
i: 0, j: 1
i: 1, j: 0
i: 1, j: 1
i: 2, j: 0
i: 2, j: 1
Each iteration of the outer loop runs the inner loop completely. Nested loops are powerful for iterating over rows and columns in matrices.
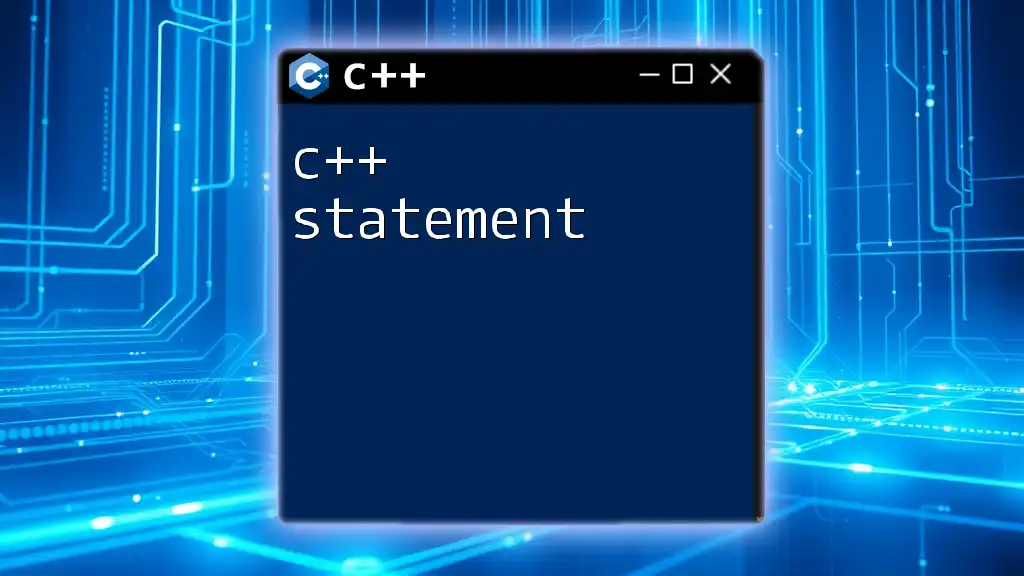
Common Mistakes When Using For Loops
Navigating the intricacies of the C++ for statement can lead to several common errors.
Off-by-One Errors
These errors occur when the loop executes one too many or one too few times. This often results from miscalculating the loop boundary. For instance, when iterating from `0` to `10`, using `i <= 10` instead of `i < 10` will lead to an extra iteration.
Infinite Loops
Developers sometimes inadvertently create infinite loops by not updating the loop control variable properly, or by having an incorrect termination condition. For example:
for(int i = 0; i < 10; ) {
std::cout << i << " "; // Missing i++
}
This code will result in an infinite output of `0` because `i` never increments.
Variable Scope
It’s essential to be cautious about variable scope within for loops. Declaring a loop control variable inside the loop limits its scope to the loop itself, which can lead to errors if you try to access it outside the loop.
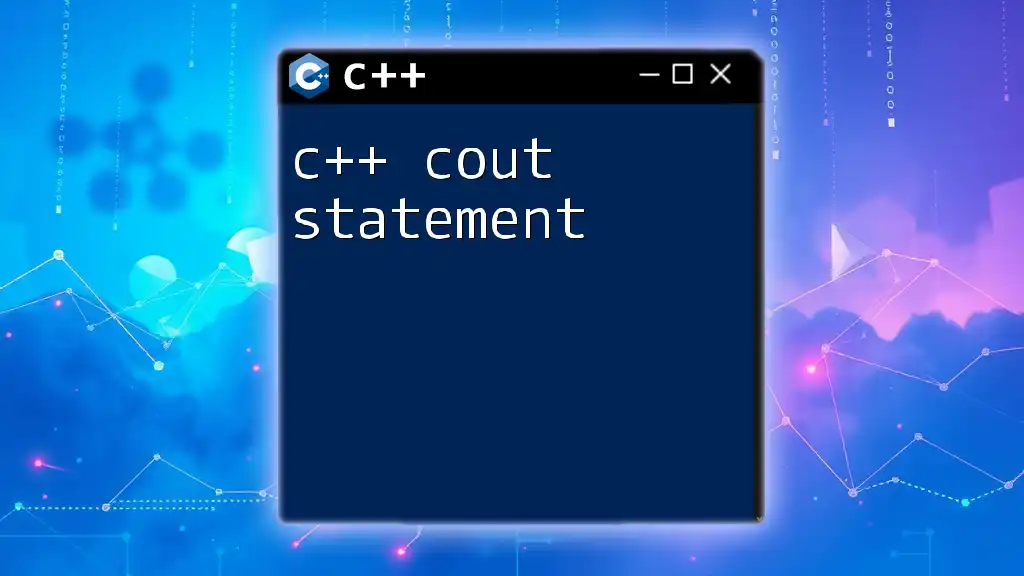
Best Practices for Using For Loops in C++
To ensure effective use of the C++ for statement, consider the following best practices:
- Keep the loop body clear and concise: A well-structured loop enhances readability and reduces the likelihood of errors.
- Use meaningful variable names: Naming loop control variables based on their purpose helps improve code clarity.
- Consider performance: When dealing with large containers, using references (e.g., `for (const auto& num : vector)`) is often more efficient, avoiding unnecessary copies of objects.
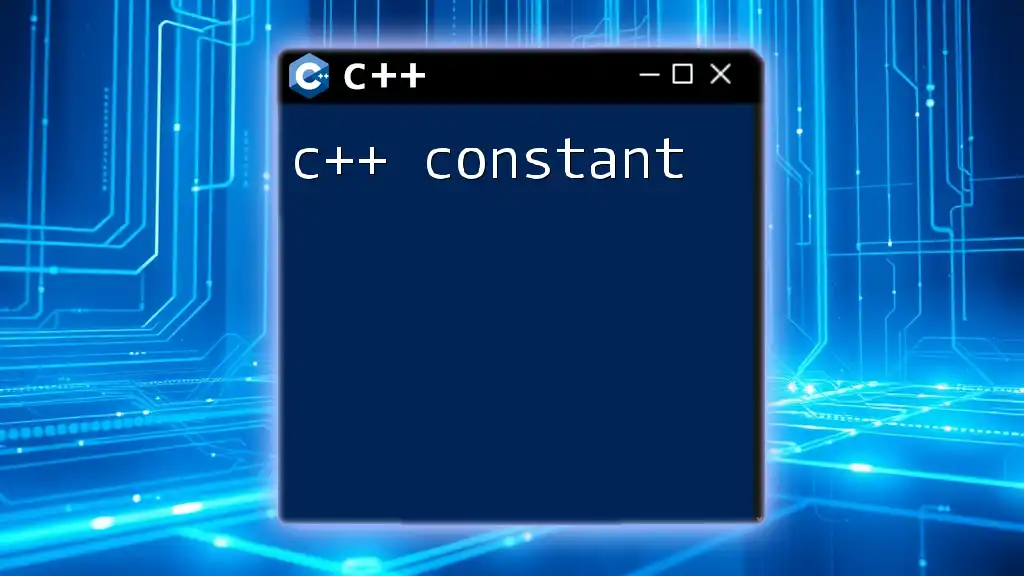
Conclusion
The C++ for statement is an essential tool for any programmer, providing flexibility and efficiency in code execution. Mastering its various forms and understanding common pitfalls will significantly enhance your programming skills. As you practice implementing for loops in your projects, you'll unlock a deeper understanding of C++ programming as a whole.
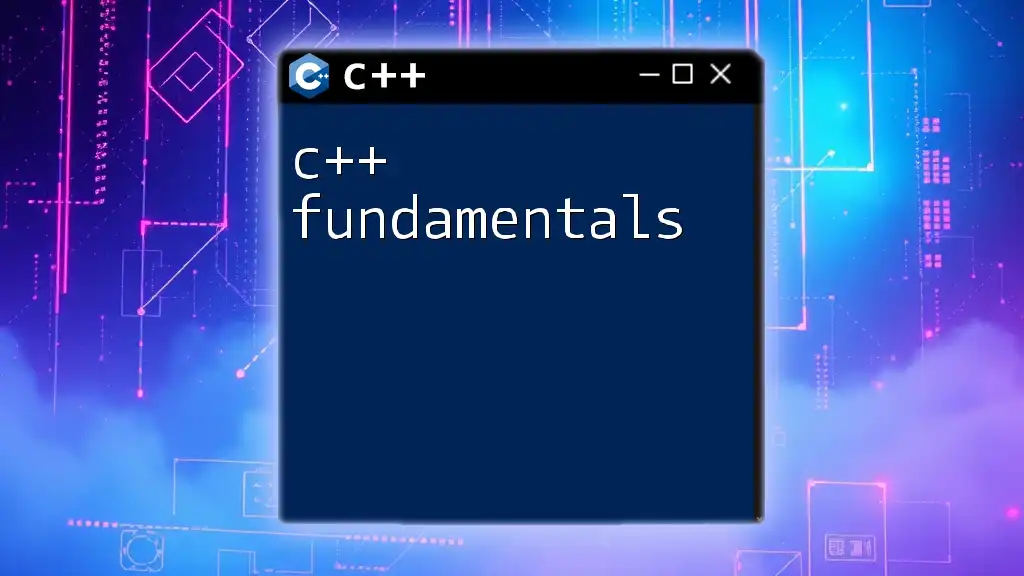
Additional Resources
For those wishing to further their knowledge on the C++ for statement, consider exploring online documentation, coding platforms, and recommended textbooks related to C++ programming. With practice, the nuances of looping and control structures will soon become second nature.