An if statement in C++ allows you to execute a block of code based on whether a specified condition evaluates to true or false.
Here's a simple example demonstrating its usage:
#include <iostream>
using namespace std;
int main() {
int number = 10;
if (number > 5) {
cout << "The number is greater than 5." << endl;
}
return 0;
}
Understanding the If Statement in C++
The if statement is one of the fundamental control structures in C++. It allows your program to make decisions based on certain conditions, influencing the flow of execution. Mastering the if statement example in C++ is crucial for any budding programmer, as it lays the groundwork for more complex logic and algorithms.
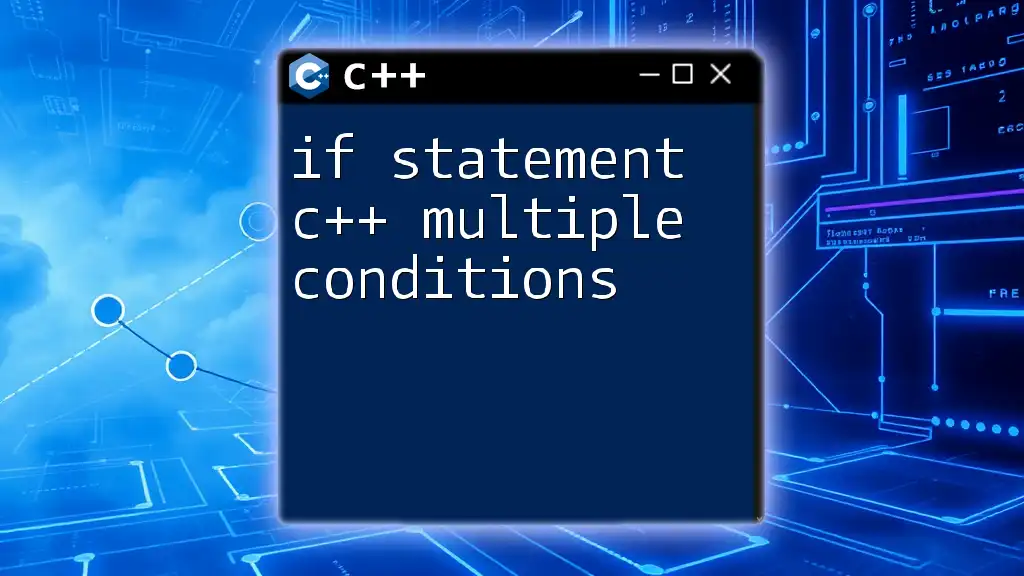
Basic Syntax of the If Statement
To effectively use the if statement, it is essential to understand its syntax:
if (condition) {
// code to execute if condition is true
}
Explanation of Each Component
-
Condition: The condition is an expression that evaluates to `true` or `false`. It can contain comparison operators (like `==`, `!=`, `>`, `<`) and logical operators (like `&&`, `||`).
-
Code Block: The code inside the braces `{}` will only execute if the condition evaluates to `true`. If it's `false`, the program will skip this block.
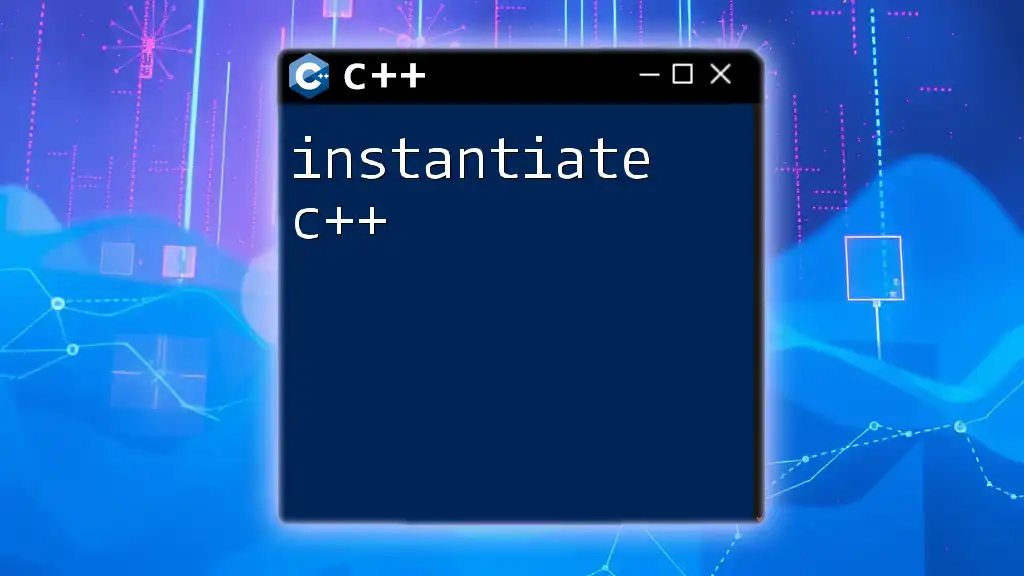
Using If Statements with Examples
Simple If Example
Consider the following simple example that checks if a person is an adult based on their age:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
}
In this example, when the variable age is greater than or equal to 18, the message "You are an adult." is printed. If the condition evaluates to `false`, nothing happens, demonstrating how the execution flow changes based on this decision point.
If-Else Statement
The if-else structure allows you to provide an alternative execution path if the condition is not met:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
Here, if the age is less than 18, the message "You are a minor." will display. This structure effectively offers clarity in the decision-making process, providing feedback for both outcomes.
Else If Ladder
Sometimes, you need to evaluate multiple conditions. For that, you can structure your code using an else if ladder:
if (grade >= 90) {
std::cout << "Grade: A" << std::endl;
} else if (grade >= 80) {
std::cout << "Grade: B" << std::endl;
} else if (grade >= 70) {
std::cout << "Grade: C" << std::endl;
} else {
std::cout << "Grade: F" << std::endl;
}
In this case, the program will check each condition in order. For example, if grade is 85, it would print "Grade: B". This allows for granular control over numerous scenarios, enhancing flexibility in your C++ programs.
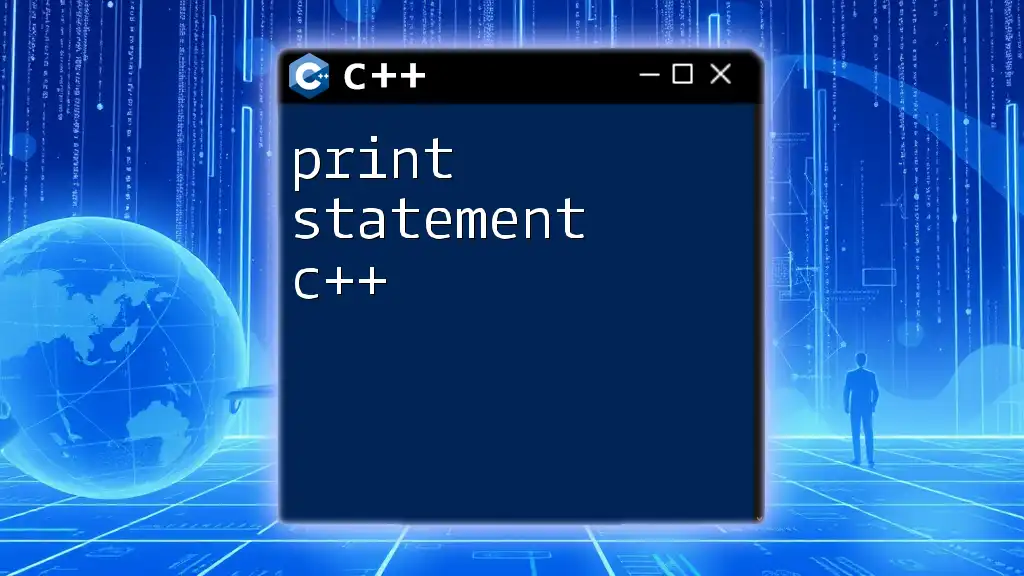
Nested If Statements
Definition and Use Cases
Nested if statements refer to placing an if statement inside another if statement. This can be valuable for more complex conditions where multiple criteria must be satisfied.
Example of Nested If
Here's a practical example:
if (x > 0) {
if (x % 2 == 0) {
std::cout << "Positive Even Number" << std::endl;
} else {
std::cout << "Positive Odd Number" << std::endl;
}
}
In this code, the program first checks if x is greater than 0. If so, it further evaluates whether x is even or odd. This clear layering of conditions is a powerful feature, enabling sophisticated logic to be implemented in a structured manner.
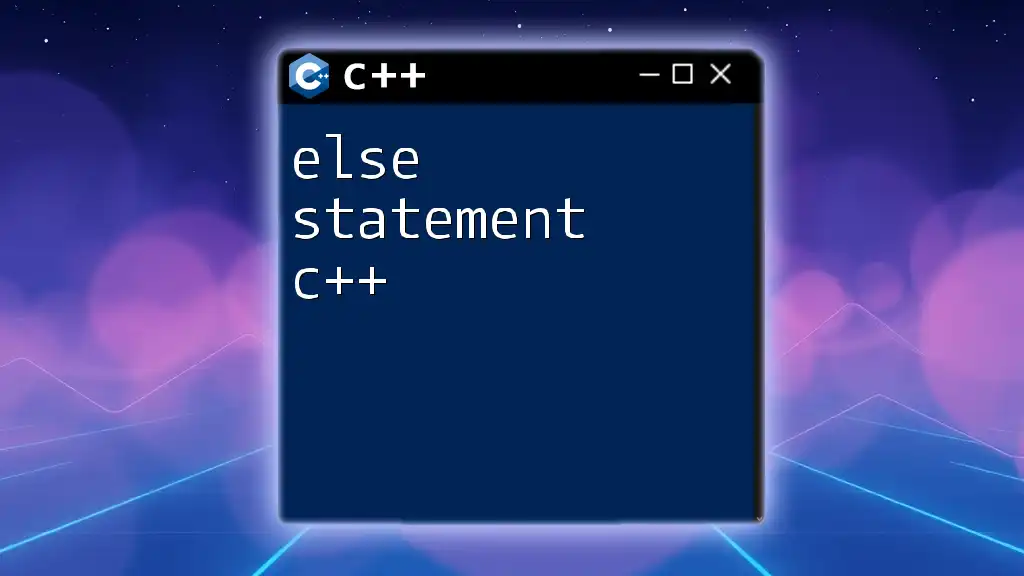
Best Practices for Using If Statements in C++
To achieve optimal clarity and maintainability when using if statements, consider the following best practices:
-
Clarity: Always strive for clarity in your code. Use meaningful variable names that describe their purpose, making it easier for yourself and others to understand.
-
Limit Nesting: While nested if statements can be useful, limit their levels to maintain readability. Overly complex nesting can confuse readers and complicate debugging.
-
Consistent Formatting: Use consistent indentation and spacing in your code blocks, which enhances readability. This can prevent logical errors and help others follow your logic effortlessly.
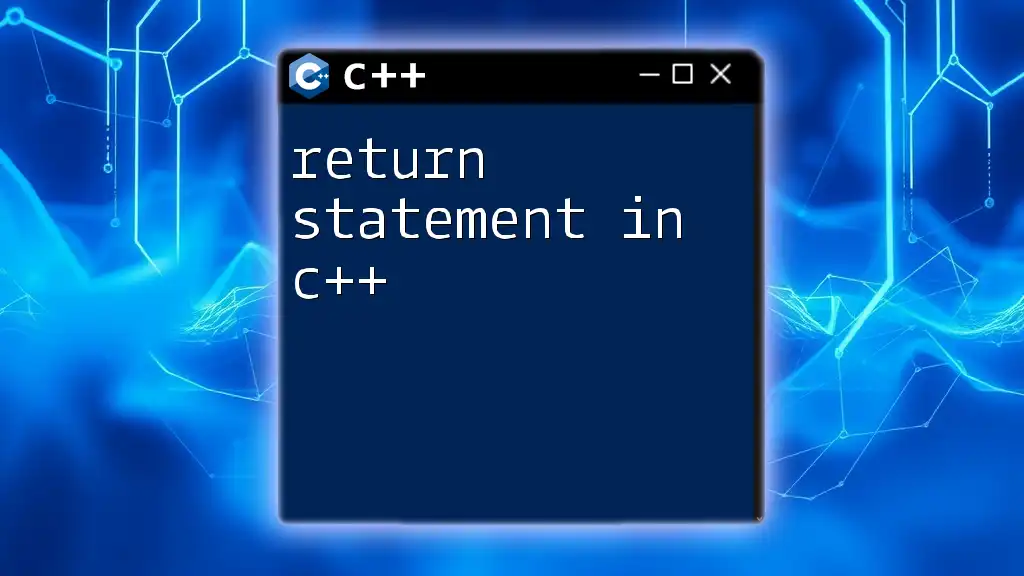
Common Mistakes with If Statements
Even experienced programmers can make mistakes with if statements. Here are a few common pitfalls to avoid:
-
Forgetting Braces: Omitting braces can lead to errors. Always use them for clarity, even in cases with a single line of code.
-
Misusing Comparison Operators: Confusing assignment (`=`) with equality (`==`) can introduce bugs. Always double-check your operators when writing conditions.
-
Logical Fallacies: Ensure that your conditions accurately reflect what you intend to check. Logical errors can result in unexpected behavior in your programs.
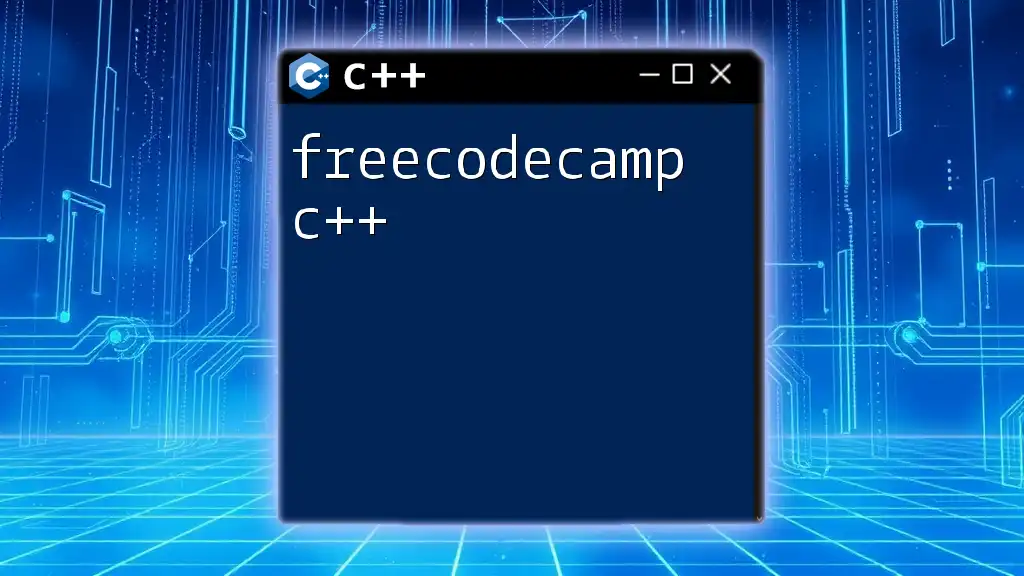
Conclusion
The if statement is a fundamental building block of programming in C++. By mastering the examples and concepts outlined here, you establish a strong foundation for making informed decisions within your code. Remember to practice various scenarios to reinforce these principles, as they pave the way for more advanced control structures.
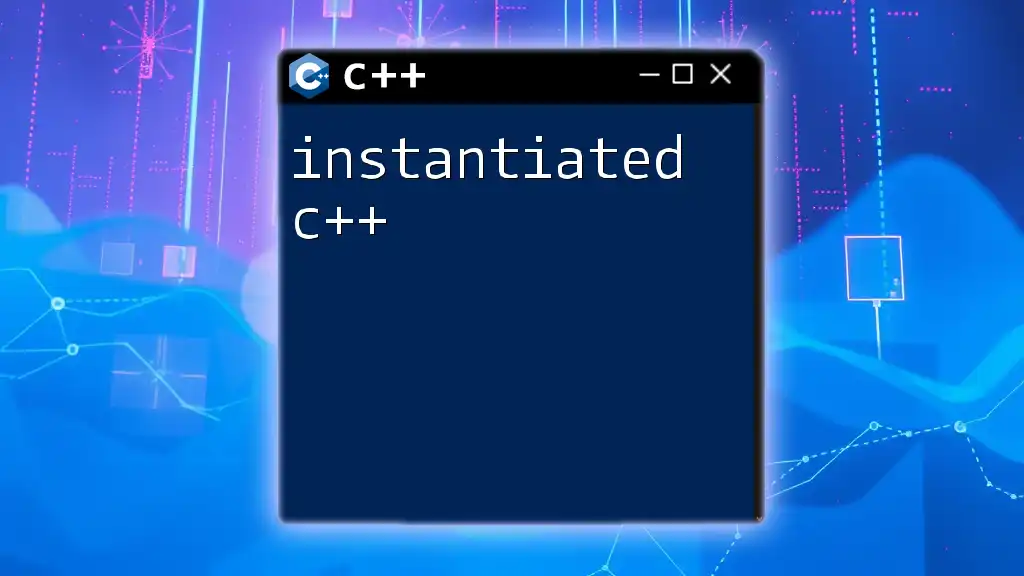
Call to Action
If you find this guide helpful, consider signing up for our courses to gain deeper insights into C++ programming. Share this article with fellow aspiring programmers to encourage their journey in understanding the intricacies of the if statement example in C++!