An `else` statement in C++ allows you to execute a block of code when a preceding `if` condition evaluates to false.
Here’s a simple example:
#include <iostream>
int main() {
int number = 10;
if (number > 0) {
std::cout << "The number is positive." << std::endl;
} else {
std::cout << "The number is not positive." << std::endl;
}
return 0;
}
Understanding the Basics of the Else Statement
What is an Else Statement?
The else statement in C++ is a fundamental control structure that allows programmers to direct the flow of execution based on conditions. It provides an alternative path when the initial condition in an `if` statement evaluates to false. This construct is crucial for decision-making processes in programming, letting you design more sophisticated and flexible algorithms.
Syntax of Else Statement in C++
The syntax for the else statement is straightforward and can be integrated easily within your conditional logic. Here’s how it is generally structured:
if (condition) {
// code block for the if condition
} else {
// code block for else
}
In this structure:
- The `if` condition checks a specific condition. If it evaluates to `true`, the code block within the curly braces following the `if` statement runs.
- The `else` clause catches all cases where the `if` condition is `false`, allowing you to define an alternative action.
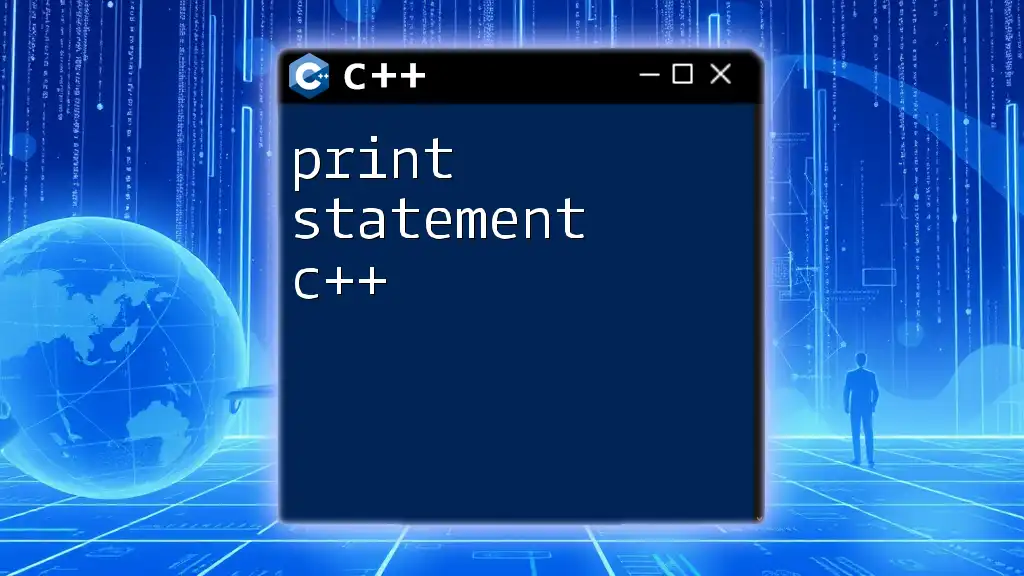
The If-Else Statement in C++
Combining If and Else Statements
In C++, the if-else statement allows you to handle binary decisions effectively. By utilizing both constructs, you can execute different blocks of code depending on the outcome of the condition evaluated in the `if`.
For instance, consider the following scenario:
int x = 20;
if (x > 10) {
std::cout << "x is greater than 10";
} else {
std::cout << "x is 10 or less";
}
In this example, if the variable `x` is indeed greater than 10, the program outputs "x is greater than 10". If not, it outputs "x is 10 or less". This illustrates the decision-making essence of the if-else structure.
Using If-Else Syntax in C++
It is crucial to adhere to proper syntax while implementing if-else statements to avoid errors that can lead to unexpected behavior. Here are a few tips:
- Ensure curly braces are used, especially with blocks containing multiple lines of code. This helps prevent logical errors.
- Always check the condition's validity to avoid infinite loops or incorrect outputs.
Best Practices for Writing Clear If-Else Statements
- Keep it Simple: Whenever possible, maintain simplicity in your conditions. Complex conditions can lead to confusion.
- Use Descriptive Conditions: Make your conditions descriptive so they are easily readable and understandable at a glance.
- Indentation: Proper indentation allows for better readability and helps avoid structural misunderstandings.
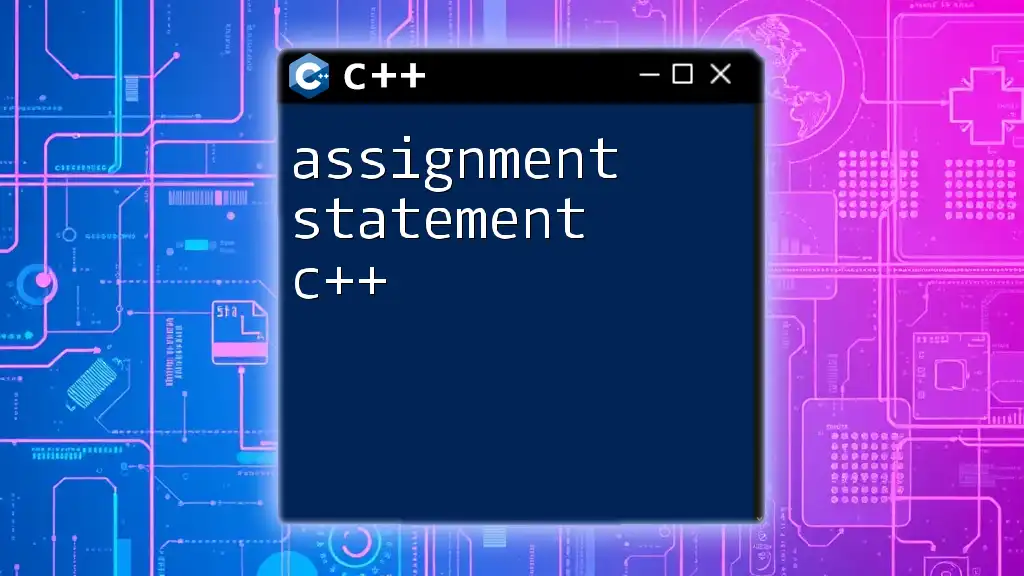
The Else If Statement in C++
Understanding Else If Statement
The else if statement provides a way to evaluate additional conditions after an initial `if` condition. This is valuable when you need to check multiple conditions sequentially.
Syntax of Else If Statement
The syntax for the else if structure is as follows:
if (condition1) {
// code block for condition1
} else if (condition2) {
// code block for condition2
} else {
// code block if none of the above conditions are true
}
This structure allows for a clearer representation of multiple conditions. Each `else if` checks an additional condition if the previous `if` or `else if` conditions evaluated to false.
Practical Example of Else If Statement
To demonstrate, let’s consider we want to assign letter grades based on a score. Here’s how the code might look:
int score = 85;
if (score >= 90) {
std::cout << "Grade: A";
} else if (score >= 80) {
std::cout << "Grade: B";
} else if (score >= 70) {
std::cout << "Grade: C";
} else {
std::cout << "Grade: F";
}
In this example, the program evaluates the `score` variable step-by-step. It assigns a letter grade accordingly based on the predefined ranges. If the score falls within the range for a specific letter grade, that particular code block executes, ensuring accurate results.
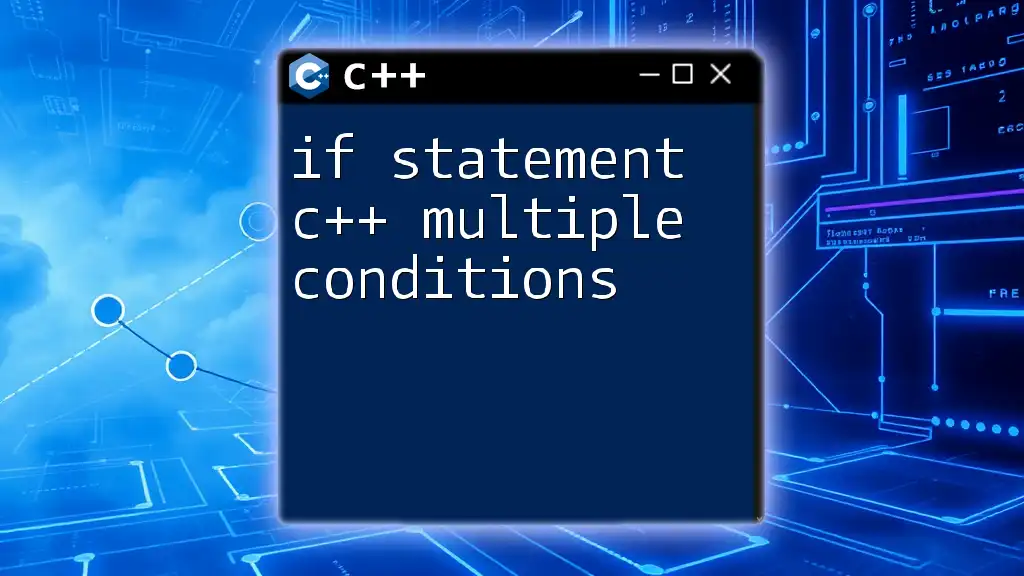
The Complete Picture: If and Else Statements
Understanding how if and else statements work together enhances your ability to create complex decision-making structures. You can nest these statements within each other, allowing for even greater flexibility.
For instance:
int age = 18;
if (age < 13) {
std::cout << "You are a child.";
} else if (age < 20) {
std::cout << "You are a teenager.";
} else {
std::cout << "You are an adult.";
}
In this scenario, multiple age categories are evaluated. If `age` is less than 13, the program states that the individual is a child. If not, it checks if the age is less than 20 to categorize them as a teenager, and if neither condition is true, it concludes that the individual is an adult. This hierarchy showcases how you can structure multiple levels of decision-making efficiently.
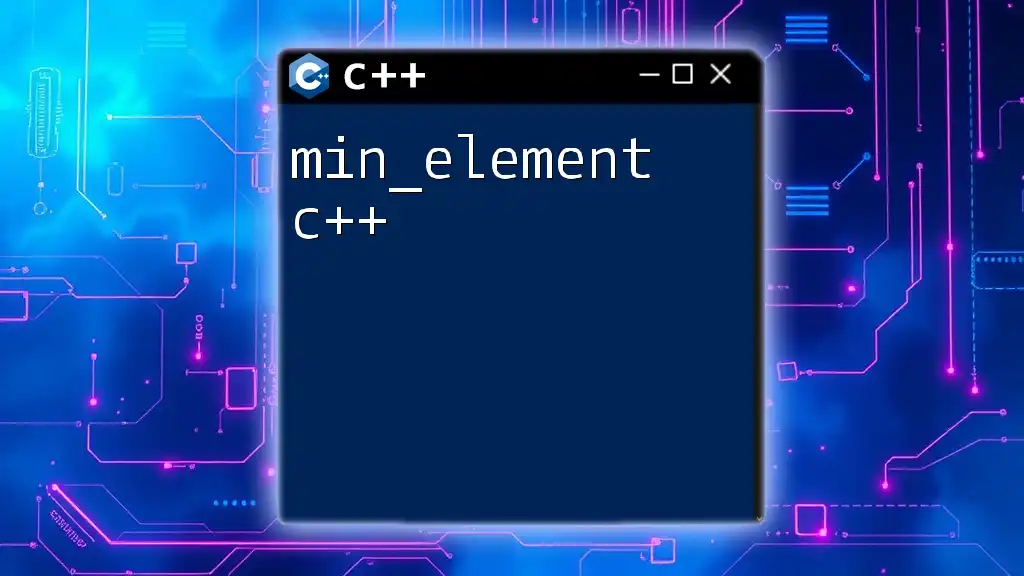
Common Errors in Using Else Statements
Mistakes to Avoid
Even though the else statement seems straightforward, there are common pitfalls programmers encounter:
- Forgetting curly braces: When the block under an `if` statement only contains one line, it's easy to forget to encapsulate multiple lines later.
- Missing conditions: Ensure that all possible conditions are covered.
- Improper indentation: Neglecting to maintain clean coding practices can lead to misinterpretation of the code logic.
Debugging Tips
When debugging if-else structures, utilize print statements to inspect which part of your decision flow is being executed. Additionally, leverage IDE tools that highlight syntax errors, allowing you to pinpoint problems more easily.
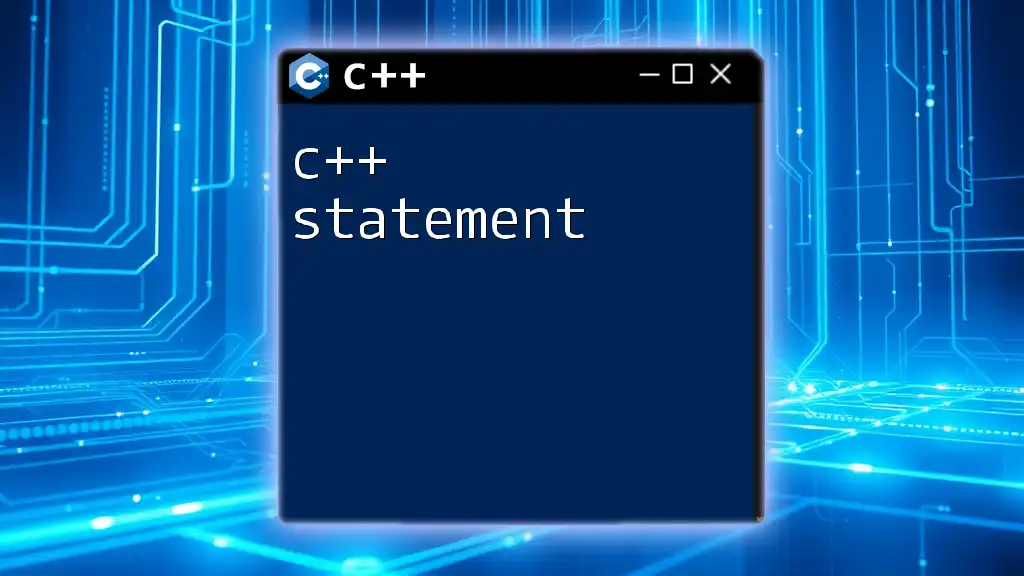
Conclusion
Mastering the else statement in C++ is essential for building robust, decision-based logic in your code. By understanding the principles behind if-else structures and practicing with various examples, you can enhance your problem-solving skills and create more effective applications.
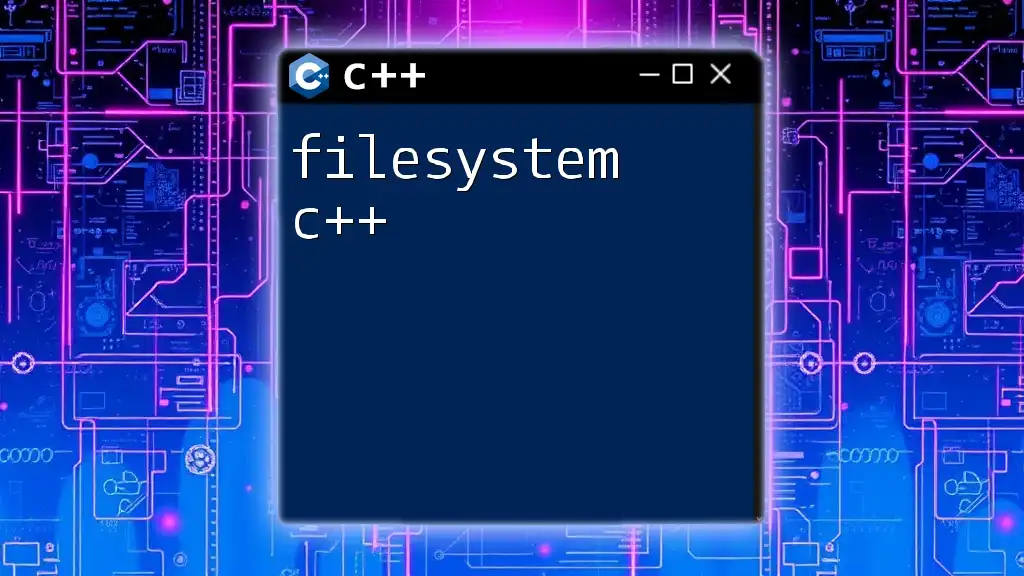
Resources for Further Learning
For deeper understanding and further learning opportunities about C++ programming, consider exploring recommended books or enrolling in online courses. These resources can provide more comprehensive insights into programming concepts and best practices.
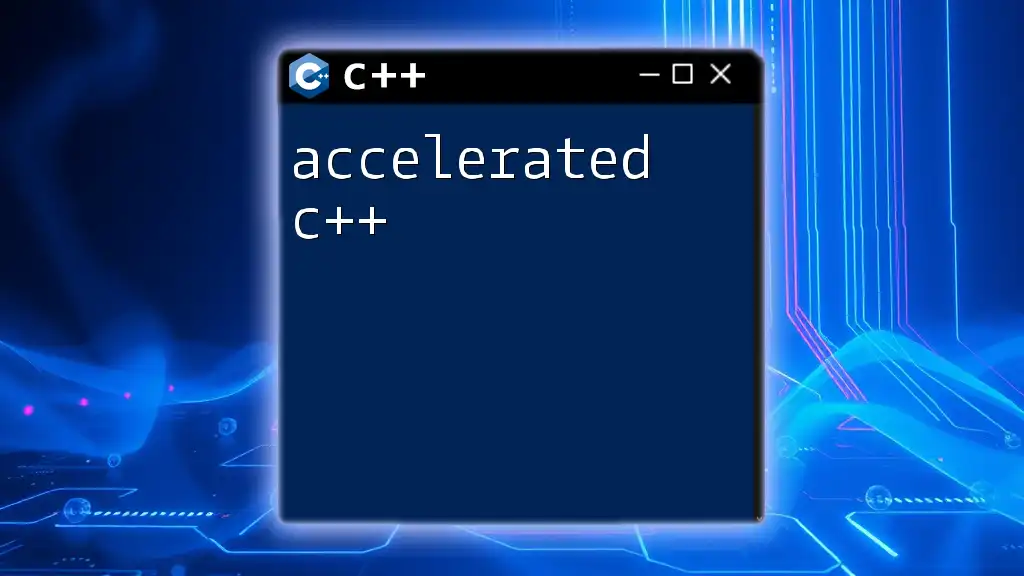
FAQs About Else Statements in C++
Frequently Asked Questions
-
What is the difference between if and else? The `if` statement checks a condition and executes its block if true, while the `else` block provides an alternative when the `if` condition is false.
-
Can else statements exist without if? No, else statements must always be used after an `if` statement. They are inherently linked.
-
How many else if statements can I use? There’s no strict limit; however, keep your conditions clear and concise to maintain readability. Using too many can lead to confusion.