In C++, you can use an `if` statement with multiple conditions by employing logical operators such as `&&` (and) and `||` (or) to evaluate more than one condition at once.
Here’s an example code snippet:
#include <iostream>
using namespace std;
int main() {
int age = 25;
bool hasPermission = true;
if (age >= 18 && hasPermission) {
cout << "You are allowed to enter." << endl;
} else {
cout << "Access denied." << endl;
}
return 0;
}
Understanding the If Statement in C++
The if statement is a fundamental control structure in C++, allowing programmers to execute specific blocks of code based on certain conditions. It enables decision-making in programs, making it crucial for effective logic implementation.
Basic Structure and Syntax of the If Statement
The typical structure of an if statement in C++ follows this syntax:
if (condition) {
// code to execute if condition is true
}
Here, the `condition` is an expression that evaluates to either true or false. If it evaluates to true, the code block within the curly braces executes; if false, the program proceeds to the next block of code outside the if statement.
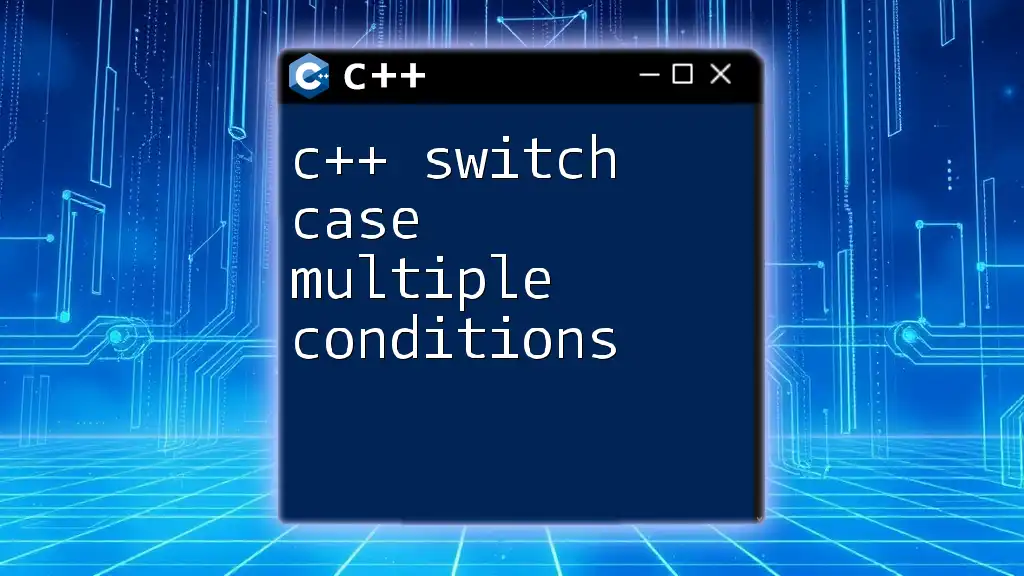
Working with Multiple Conditions
Combining Conditions with Logical Operators
When working with the if statement in C++ with multiple conditions, you often need to combine different expressions to refine your logic. This is where logical operators come into play.
-
AND (`&&`): This operator allows you to check if multiple conditions are true simultaneously. If all conditions return true, the entire expression evaluates to true.
- Example Code:
if (condition1 && condition2) { // code if both conditions are true }
-
OR (`||`): This operator is useful when you want to execute code if at least one of several conditions is true. The expression evaluates to true if any single condition returns true.
- Example Code:
if (condition1 || condition2) { // code if at least one condition is true }
-
NOT (`!`): This operator negates the boolean value of a condition, allowing you to check if a condition is false.
- Example Usage:
if (!condition1) { // code if condition1 is false }
Nested If Statements
Nested if statements occur when an if statement is placed inside another if statement. They are beneficial when needing to evaluate multiple layers of conditions.
Consider the following scenario: You want to check whether a user is eligible for a discount based on multiple conditions, such as membership status and the purchase amount.
Example Code:
if (isMember) {
if (purchaseAmount > 100) {
// code for members with purchase over $100
}
}
While nested ifs are powerful, excessive nesting can make code harder to read. Using clear and logical conditions helps maintain code readability.
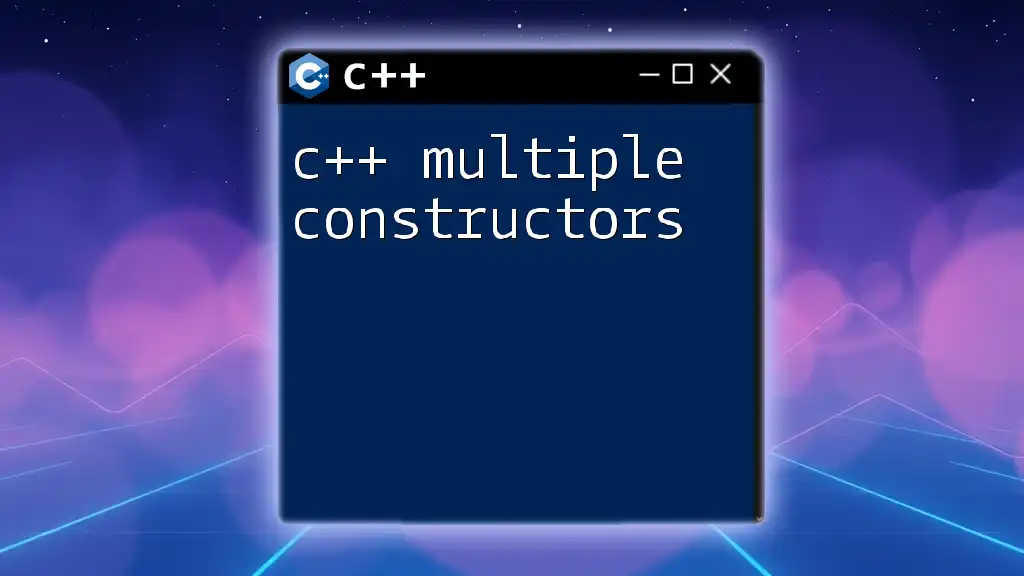
Practical Examples of If Statements with Multiple Conditions
Real-world Scenario 1: Grading System
Implementing a grading system is one common application of if statements. Here’s an example where different score ranges determine the grades:
int score = 85;
if (score >= 90) {
// A grade
} else if (score >= 80) {
// B grade
} else if (score >= 70) {
// C grade
} else {
// F grade
}
In this example, the program evaluates the `score` variable against multiple conditions, each represented by an if or else-if statement. It provides a clear and straightforward method to determine the user's grade.
Real-world Scenario 2: User Access Levels
Here, let's consider user roles in an application to illustrate another usage of the if statement c++ multiple conditions. Different access levels are given based on user roles:
int userRole = 2; // 0: Guest, 1: User, 2: Admin
if (userRole == 2) {
// Grant access to admin features
} else if (userRole == 1) {
// Grant access to user features
} else {
// Guest access
}
This structure efficiently checks the role of the user and executes the appropriate block of code, ensuring that users have access only to the features that their role allows.
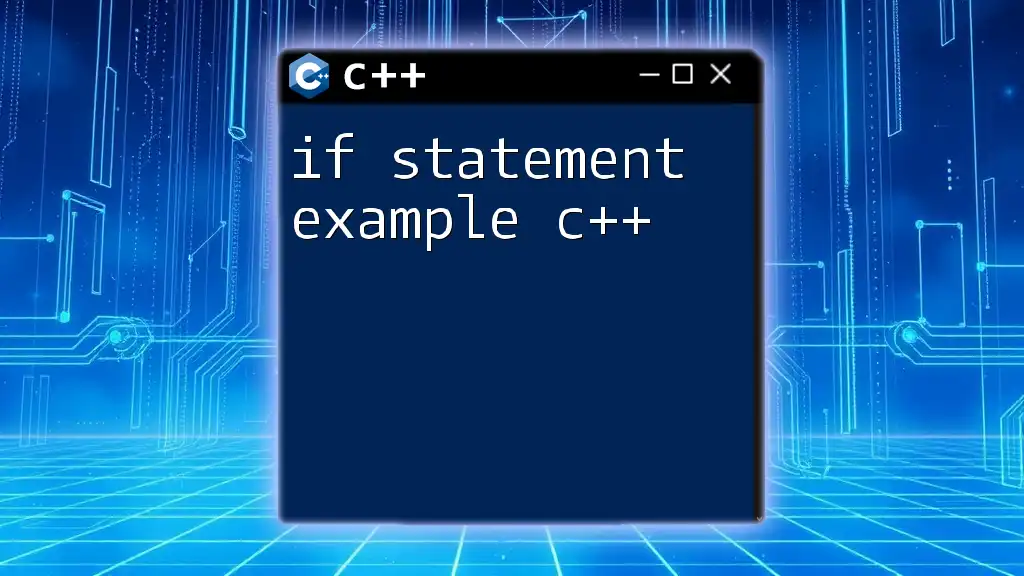
Best Practices for Using If Statements with Multiple Conditions
Keeping Conditions Clear and Readable
Clarity is key in programming, especially when dealing with multiple conditions. Aim for concise and straightforward conditions that accurately represent the logic you intend to implement. Techniques for enhancing readability include:
- Using descriptive variable names that convey meaning.
- Breaking complex conditions into smaller, named functions when necessary.
Avoiding Deep Nesting
While nested if statements are useful, they can quickly complicate code, making it difficult to follow. Deep nesting can lead to confusion and increased chances of errors. Instead, consider restructuring your code by:
- Restructuring conditions to lessen nesting and improve clarity.
- Using a `switch` statement when appropriate for multiple discrete conditions.
- Leveraging polymorphism in object-oriented programming to handle complexity.
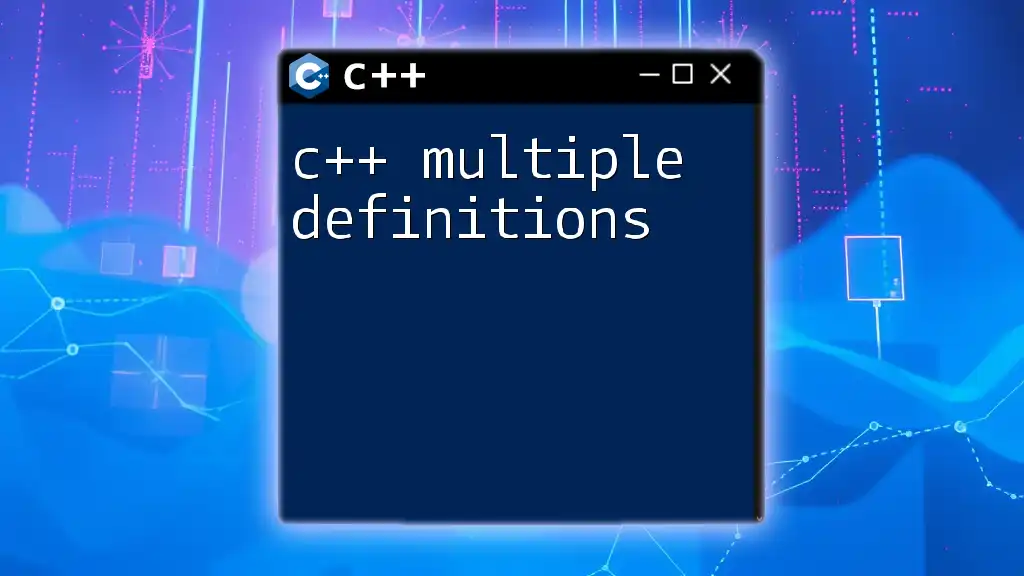
Conclusion
Understanding how to use if statements in C++ with multiple conditions is essential for developing robust applications. It allows you to craft more complex logical flows that can adapt to various scenarios encountered in programming.
Regular practice with different conditions will enhance your coding skills and deepen your grasp of C++ programming logic. Remember, clarity and simplicity are vital; aim for readable and maintainable code in all your projects.
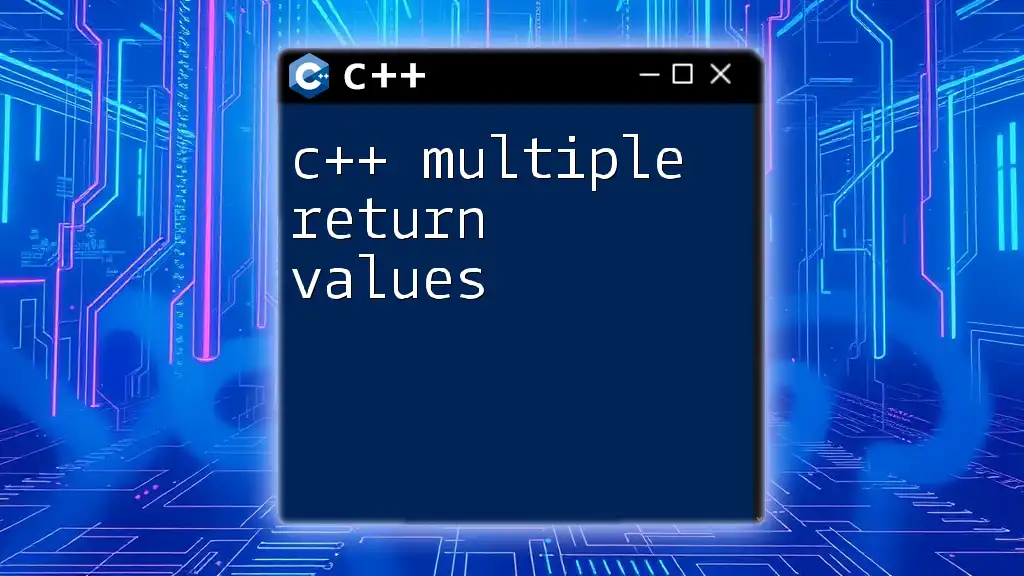
Additional Resources
To further master C++, consider exploring online resources that offer tutorials, coding challenges, and community support. Continuous learning and practice are the best strategies for improving your programming capabilities.