In C++, a switch case can handle multiple values for a single case by using commas to separate the conditions, allowing for cleaner and more organized code. Here's an example:
#include <iostream>
using namespace std;
int main() {
int day = 3;
switch (day) {
case 1:
case 2:
cout << "It's a weekday." << endl;
break;
case 3:
case 4:
case 5:
cout << "It's a workday." << endl;
break;
case 6:
case 7:
cout << "It's the weekend!" << endl;
break;
default:
cout << "Invalid day." << endl;
}
return 0;
}
Understanding Switch-Case in C++
The switch-case statement in C++ is a powerful control structure that allows you to execute different parts of code based on the value of a variable or expression. It's particularly useful when you have multiple values to compare against a single variable. The syntax is straightforward, which enhances code readability and organization.
Here’s the basic syntax of a switch-case:
switch(expression) {
case constant1:
// code block
break;
case constant2:
// code block
break;
// more cases
default:
// default code block
}
Benefits of using switch-case include clearer intent and potentially better performance compared to a series of if-else statements, especially when dealing with numerous conditions.
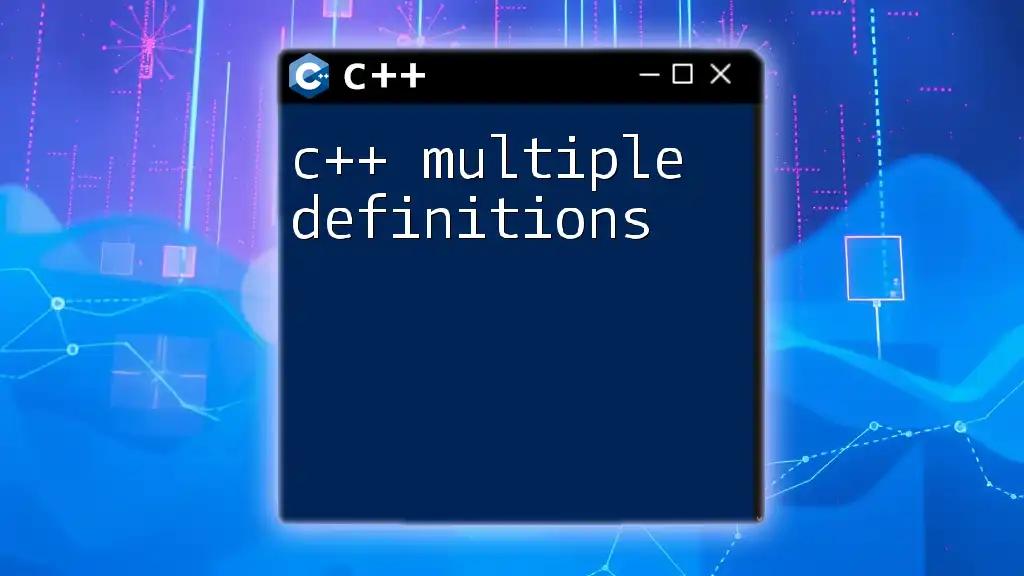
Handling Multiple Conditions in Switch-Case
One of the features of the `c++ switch case multiple conditions` is its ability to handle multiple case labels that execute the same block of code. This acts like grouping cases together without needing separate code blocks for each.
Take a look at this example:
switch(character) {
case 'a':
case 'A':
cout << "Letter A" << endl;
break;
case 'b':
case 'B':
cout << "Letter B" << endl;
break;
default:
cout << "Other Letter" << endl;
}
In this code snippet, both lower-case and upper-case letters for 'A' and 'B' yield the same output. This effectively minimizes redundancy and keeps your code concise.
Explanation of Fall-Through Behavior
The fall-through behavior is a unique characteristic of switch-case statements in C++. If you do not use a `break` statement at the end of a case block, execution will "fall through" to subsequent cases until a break is encountered or until the switch block ends.
Here's an illustration of fall-through:
switch(value) {
case 1:
cout << "First case" << endl;
case 2:
cout << "Second case" << endl;
break;
case 3:
cout << "Third case" << endl;
break;
}
Without a break after case `1`, if `value` is `1`, it will execute both case `1` and case `2`, potentially leading to unexpected results.
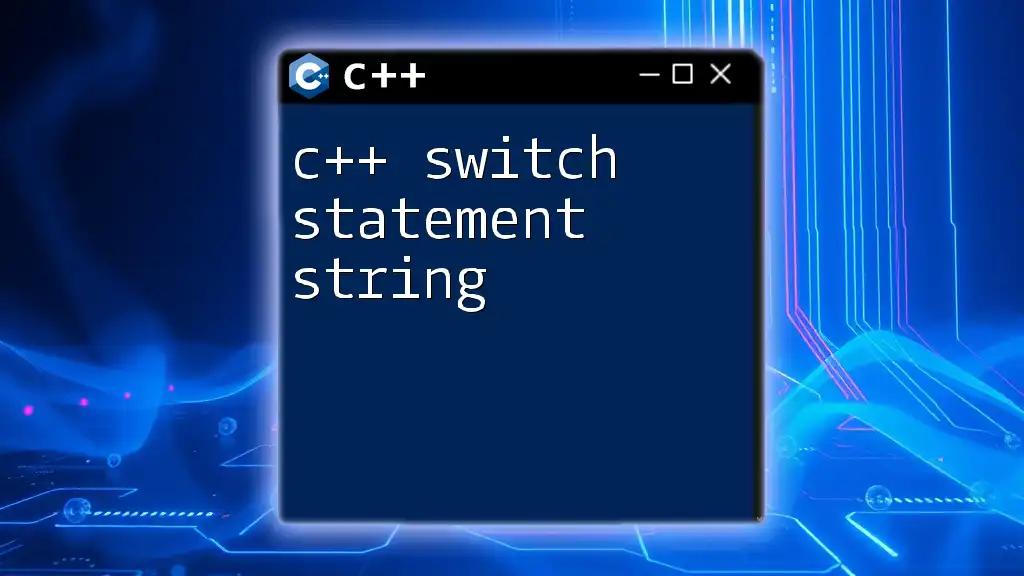
Advantages and Disadvantages
Advantages of Switch-Case with Multiple Conditions
-
Readability and Organization: Using switch-case can significantly improve the readability of your code, especially when dealing with numerous conditions.
-
Performance Considerations: For a large set of conditions, switch-case statements can be more efficient than if-else chains, particularly as the number of conditions increases.
Disadvantages of Switch-Case
-
Type Limitations: The switch-case statement is limited to integral types (i.e., integers, characters, and enums). This can be restrictive compared to the flexibility of if-else statements which work with any expressions.
-
Fall-Through Risks: While fall-through can be useful, it can also introduce bugs if not managed correctly, causing unintended code execution.
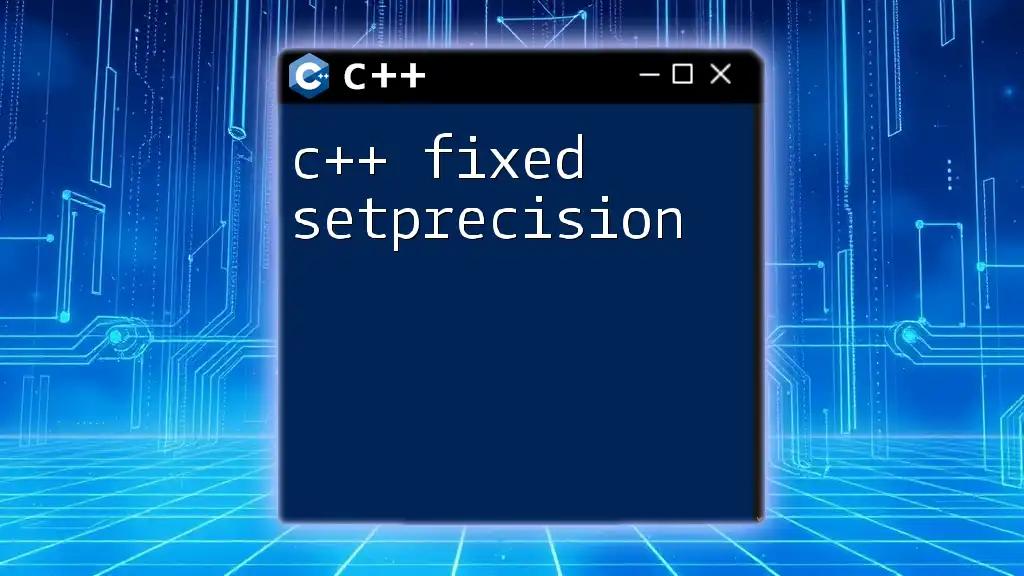
Best Practices for Using Switch-Case
To ensure effective use of `c++ switch case multiple conditions`, consider the following best practices:
-
Clarity and Conciseness: Always aim for clear and brief code. Avoid unnecessary cases that complicate maintenance.
-
Structured Cases: Group related cases together logically to maintain an organized structure.
-
Utilize Break Statements: To avoid fall-through errors, ensure you consistently use break statements unless there’s an intentional reason for fall-through behavior.
-
Handle Default Cases: Always include a default case to catch unexpected values and prevent logical errors in your application.

Alternatives to Switch-Case for Multiple Conditions
While switch-case is useful, `if-else` statements serve as a valid alternative, especially when conditions are complex or when you're dealing with types other than integral types.
For example:
if (character == 'a' || character == 'A') {
cout << "Letter A" << endl;
} else if (character == 'b' || character == 'B') {
cout << "Letter B" << endl;
} else {
cout << "Other Letter" << endl;
}
The above code achieves the same result but without the limitations of a switch-case structure.

Real-World Examples
Practical application of `c++ switch case multiple conditions` can be seen in various software scenarios. For example, consider a shopping cart application where items are categorized by types:
switch(itemType) {
case 'E':
// Handle electronics
break;
case 'C':
// Handle clothing
break;
case 'H':
// Handle household
break;
default:
// Handle unknown items
}
This scenario highlights how switch-case allows for clear handling of items based on their type, allowing for easy extensions and changes down the road.

Conclusion
In conclusion, mastering the use of `c++ switch case multiple conditions` can lead to cleaner, more efficient code. By understanding the syntax and behavior of switch-case statements and considering when to use them versus alternatives, you can enhance both your coding skills and the quality of your programs. Practice using switch-case in different scenarios, and share your coding experiences with the community for feedback and growth.

Additional Resources
For those looking to dive deeper into C++, consider exploring additional articles related to C++ programming, as well as recommended books and online courses that provide comprehensive insights into advanced C++ topics.
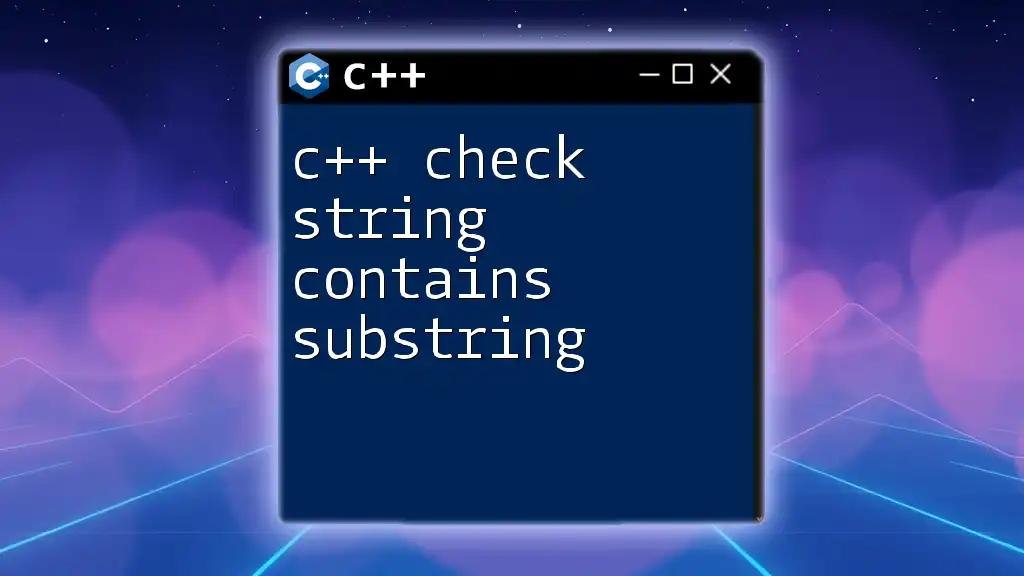
FAQs
Q: Can switch-case handle types other than integral types? A: No, switch-case is limited to integral types like integers and characters. For other types, use if-else statements.
Q: What should I do if I have many conditions? A: For extensive conditions, a switch-case statement is often more readable and manageable than an if-else chain.