In C++, the `switch` statement can only evaluate integral types, so to use it with strings, you typically need to use a series of `if-else` statements instead; however, here's an example of using a `switch` statement with an integer that represents the desired string case.
#include <iostream>
#include <string>
int main() {
std::string input = "apple";
int caseIndex;
// Mapping strings to integers
if (input == "apple") caseIndex = 1;
else if (input == "banana") caseIndex = 2;
else if (input == "cherry") caseIndex = 3;
else caseIndex = 0;
switch (caseIndex) {
case 1: std::cout << "You selected an apple!" << std::endl; break;
case 2: std::cout << "You selected a banana!" << std::endl; break;
case 3: std::cout << "You selected a cherry!" << std::endl; break;
default: std::cout << "Unknown selection!" << std::endl; break;
}
return 0;
}
Understanding the Switch Statement in C++
What is a Switch Statement?
A switch statement is a control structure in C++ that allows you to execute different parts of code based on the value of an expression. It provides a cleaner and more readable way to dispatch execution to different blocks of code compared to using multiple `if-else` statements.
The basic structure of a switch statement looks like this:
switch (expression) {
case value1:
// code block
break;
// other cases
default:
// code block
}
In this structure, the `expression` is evaluated once, and its value is compared with each `case`. If a match is found, the associated code block executes until a `break` statement is encountered. Without `break`, execution continues into the next case, which might lead to unintended behavior.
Benefits of Using Switch Statements
Switch statements offer several advantages:
-
Improved Readability: They allow better visual organization of code when handling a multiple-choice scenario. This is particularly useful when you have numerous possible values for a single variable.
-
Enhanced Performance: For larger numbers of cases, switch statements can perform more efficiently than multiple `if-else` conditions, mainly because they often compile to jump tables.
-
Clear Organization: By categorizing similar logic under case labels, it makes the code easier to follow, especially when compared to nested or chain `if-else` statements.
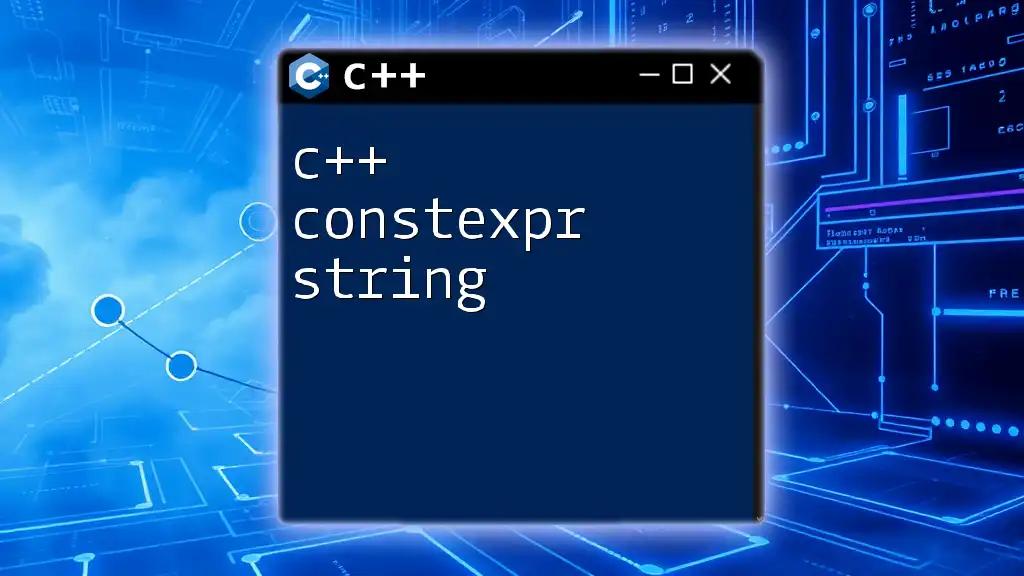
Using Strings with Switch Statements
The Challenge of Strings in Switch Statements
While the switch statement is powerful for many data types, it comes with limitations. In C++, you can only use integral types (like `int`, `char`, or enumerations) in switch statements. Unfortunately, strings cannot be directly used with a switch statement. Hence, understanding this limitation is crucial when working with conditions that involve string comparisons.
Workaround: Using `if-else` Statements
Since you cannot utilize strings in switch cases, a common alternative is to use the `if-else` construct. This allows for straightforward string comparison, as demonstrated below:
std::string command = "start";
if (command == "start") {
// Execute start command
} else if (command == "stop") {
// Execute stop command
} else {
// Handle unknown command
}
This code snippet illustrates how you can check the value of a string against various possibilities using simple equality checks. However, this method can lead to lengthy blocks of code if many conditions are required.
Using `std::unordered_map` as a Switch Alternative
To avoid the complexity of long `if-else` chains, you can leverage `std::unordered_map` from the C++ Standard Library. This allows you to store string keys with associated actions, enabling a switch-like approach with strings.
Example Code with Unordered Map
Here’s how you can implement command execution using `std::unordered_map`:
#include <iostream>
#include <unordered_map>
#include <string>
#include <functional>
void executeCommand(const std::string &command) {
std::unordered_map<std::string, std::function<void()>> commandMap = {
{"start", []() { std::cout << "Starting...\n"; }},
{"stop", []() { std::cout << "Stopping...\n"; }},
{"pause", []() { std::cout << "Pausing...\n"; }}
};
auto it = commandMap.find(command);
if (it != commandMap.end()) {
it->second(); // Execute the corresponding function
} else {
std::cout << "Unknown command!\n";
}
}
In this example, `commandMap` links string commands with corresponding lambda functions that execute the related operations. When `executeCommand` is called, it searches for the `command` within the map, and if found, invokes the associated function. If you input a command that's not mapped, a default message indicates an unknown command.
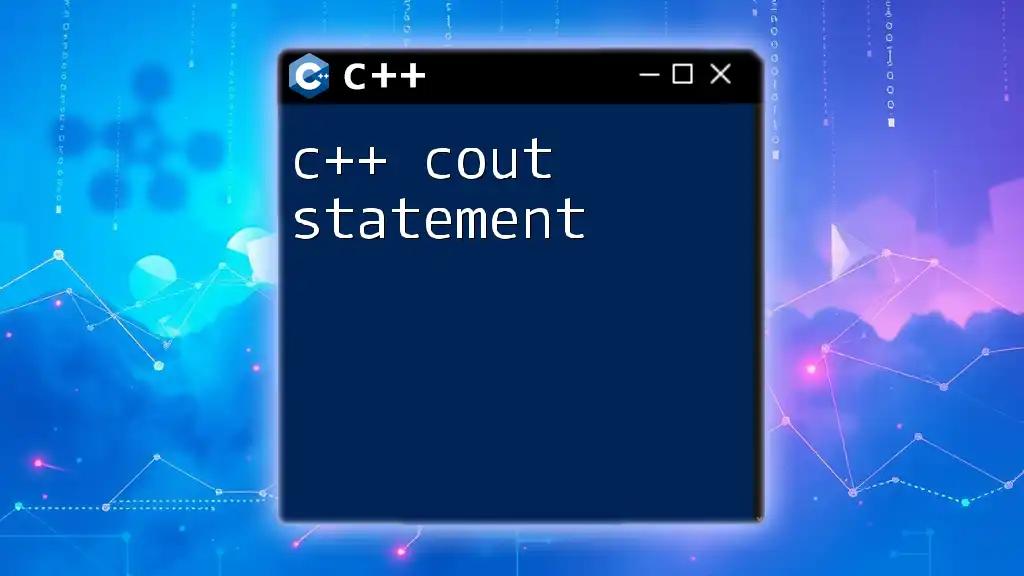
Comparing Approaches: Switch vs. If-Else vs. Unordered Map
Pros and Cons of Each Approach
-
Switch Statement:
- Pros: Efficient for integral types and improves code organization.
- Cons: Doesn’t support strings, making it impractical in this context.
-
If-Else Statements:
- Pros: Directly compares strings; simple to implement.
- Cons: Can become verbose with many conditions; less organized and harder to maintain.
-
Unordered Map:
- Pros: Flexible and maintains a clear association between commands and actions; scales well with added commands; enhances readability.
- Cons: Slightly more overhead in setup.
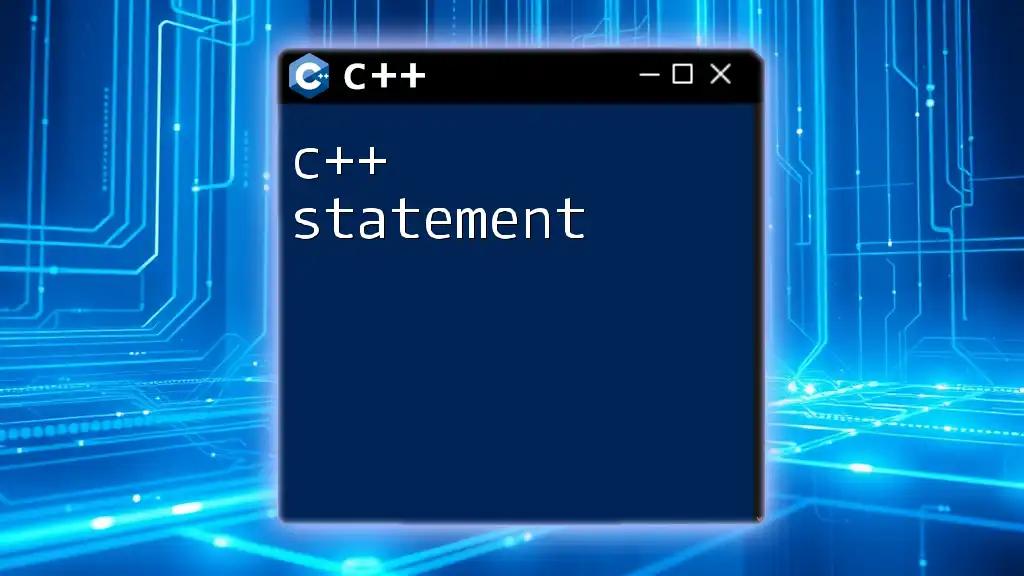
Best Practices When Using Conditional Statements
Choosing the Right Control Structure
Selecting the appropriate control structure depends on multiple factors, such as the context of the problem, the number of conditions, and readability. Generally, you should prefer:
- Switch statements for operations on integral values where clarity is vital.
- If-else chains for simple decisions and fewer conditions.
- Unordered maps when dealing with strings to keep the code modular and comprehensible.
Structuring Your Code for Clarity
When facing complex logic with multiple conditions, it’s best to maintain a structured format. Avoid nesting too deeply, as this can complicate flow and readability. Instead, consider writing smaller functions or using lookup structures like unordered maps to handle behavior, which keeps your main logic concise and clear.
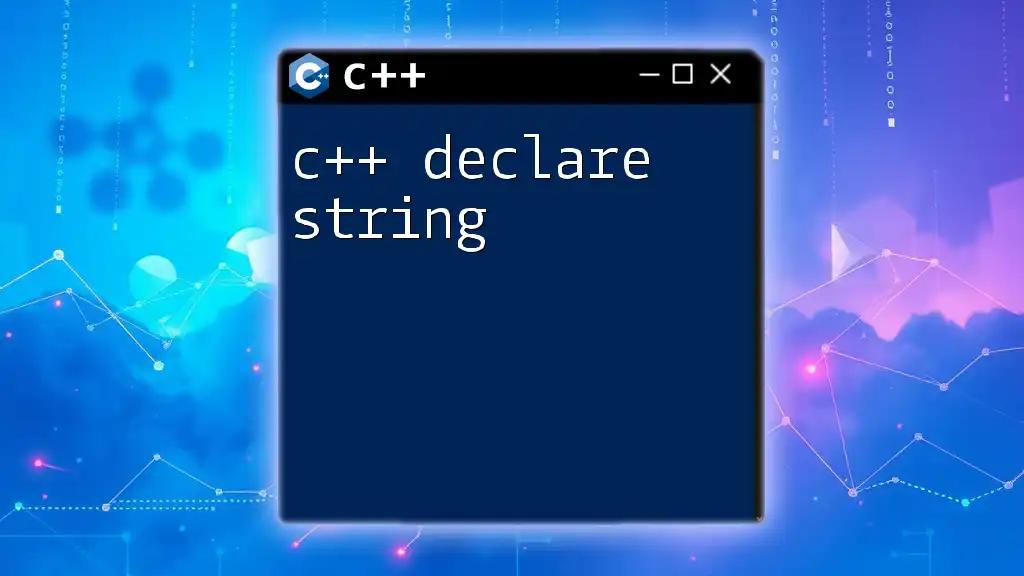
Conclusion
Using the C++ switch statement string is restricted due to type limitations, which necessitates exploring alternatives such as `if-else` constructs or `std::unordered_map`. Understanding these alternatives not only helps in crafting effective control structures but also enhances the maintainability of your code. By embracing clean structures, you prepare your code for scalability and readability, making it much easier to manage as your project grows.
Take the time to experiment with these concepts, and explore your options for mastering C++ control flow. For further tips, tutorials, and concise guides on C++, visit our company’s resources to elevate your learning experience!
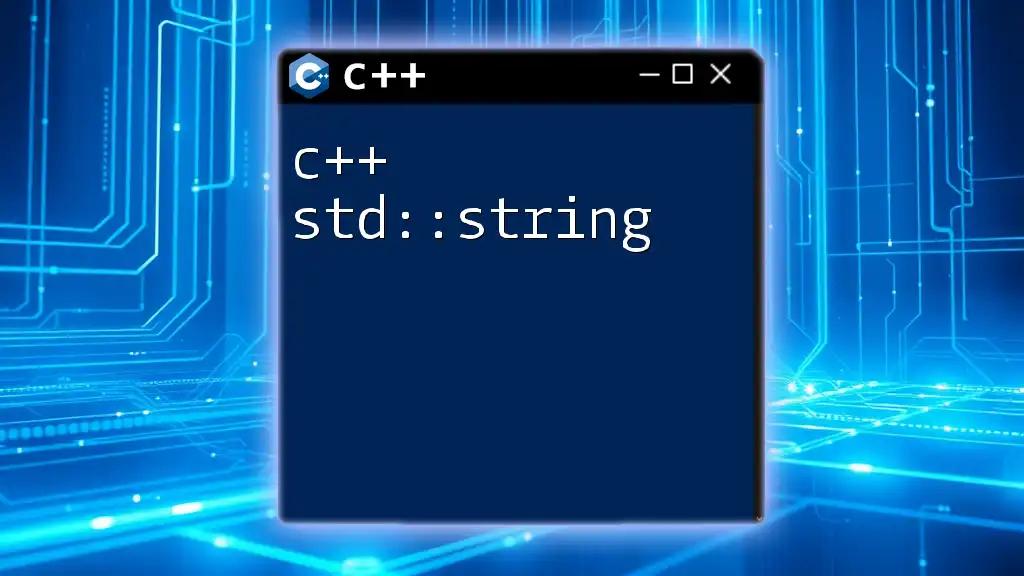
Additional Resources
For those looking to delve deeper, refer to the C++ documentation on switch statements and unordered maps for official guidelines, or check out recommended books and online resources for extensive learning. Feel free to reach out for inquiries or further assistance in your C++ journey!
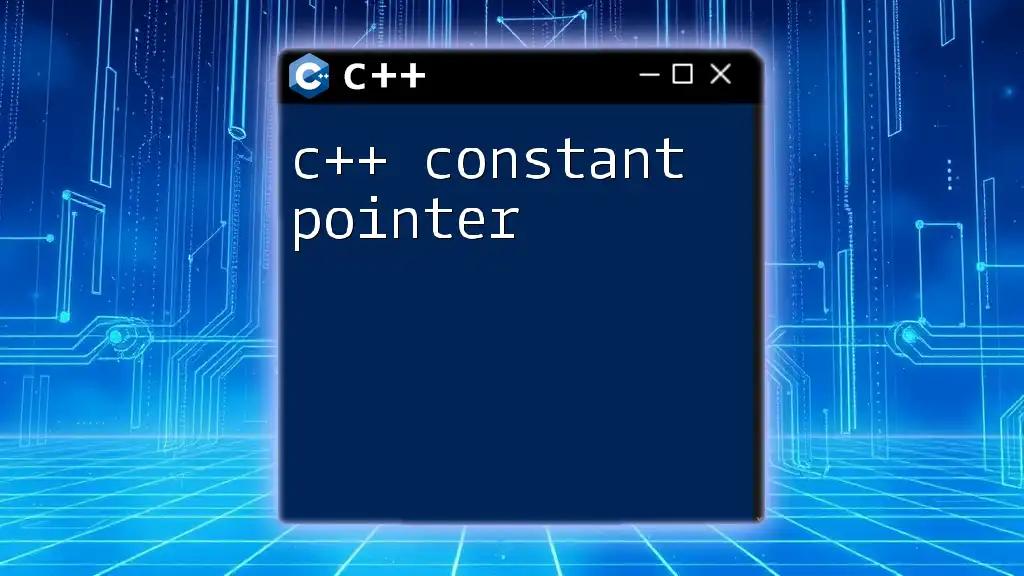
FAQs
Can I use switch statements to compare strings in C++?
Unfortunately, no. C++ switch statements only support integral types, meaning strings cannot be used directly. Instead, consider utilizing `if-else` constructs or `std::unordered_map`.
What is a more efficient way to handle multiple string comparisons in C++?
Using an `std::unordered_map` is generally more efficient and cleaner, allowing for a more modular approach to string-based commands. Alternatively, simple `if-else` statements can work well with a limited number of conditions.
Are there scenarios where using if-else is better than a switch statement?
Yes, if-else statements are advantageous when dealing with string comparisons or when you have a small number of conditions. While switch statements are excellent for clarity and performance with integral types, they’re not always the best fit for strings.