The C++ `cout` statement is used to output data to the standard output stream, typically the console, and can be used with various data types and manipulators for formatted display. Here's a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the cout Statement in C++?
The c++ cout statement is a fundamental part of the C++ programming language used primarily for outputting data to the console. Originating from the Standard Library, `cout` stands for “character output.” It allows programmers to send data to standard output, effectively allowing them to communicate results, messages, or any form of information back to the user.
As an object of the `ostream` class, the `cout` statement is equipped to handle various data types seamlessly, making it an essential tool in every C++ programmer's toolkit.
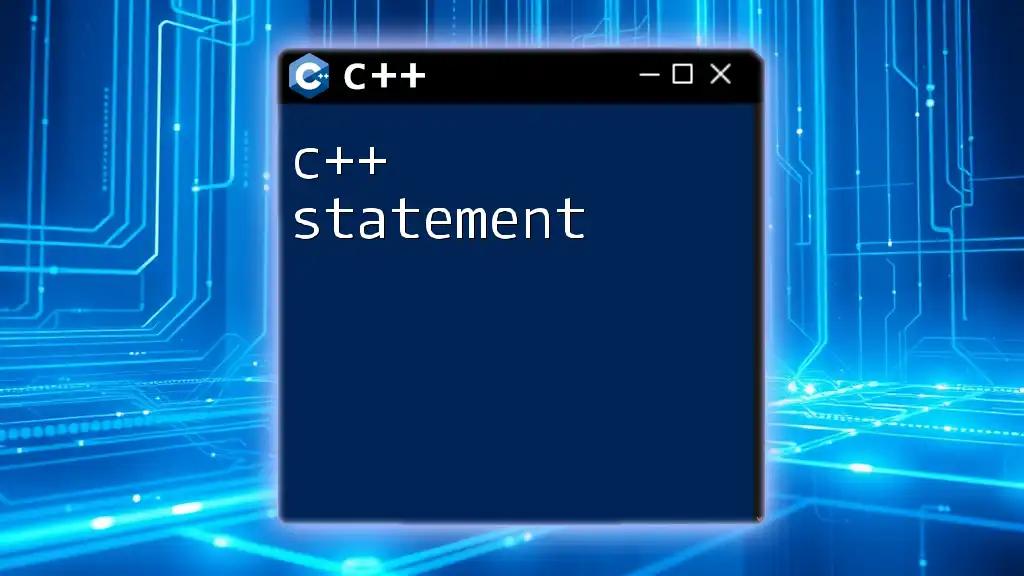
The Anatomy of a cout Statement
The basic structure of a c++ cout statement can be represented as follows:
cout << expression;
In this syntax, `cout` refers to the output stream, while the `<<` operator is called the insertion operator. It effectively tells the program to take the data following this operator and "insert" it into the output stream managed by `cout`. This simple yet powerful construct allows for flexible output formatting.
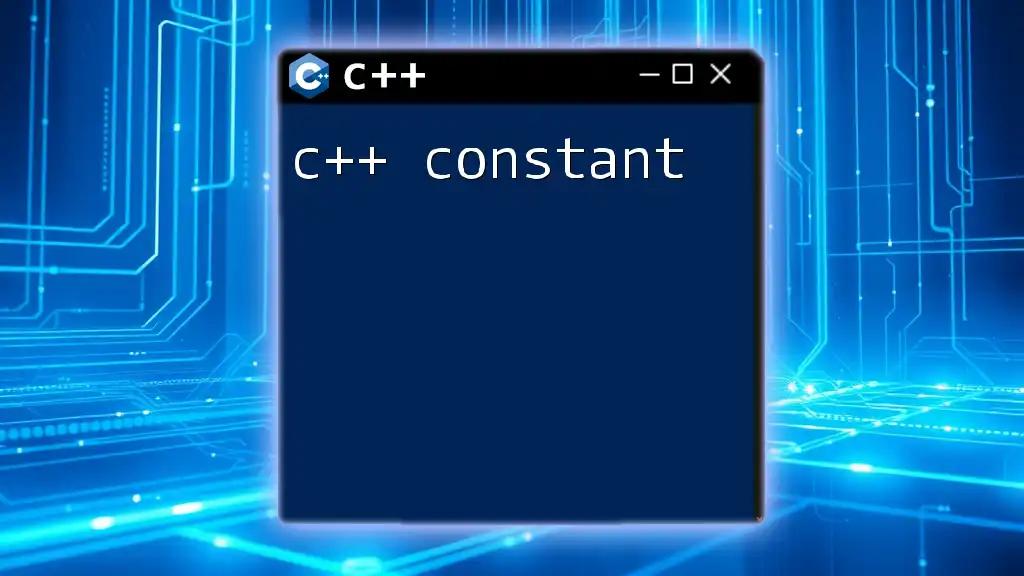
Setting Up Your Environment for C++ cout
Before diving into using `cout`, it's essential to set up an environment where you can write and execute C++ code.
To start, you need a compiler that supports C++. Popular options include:
- GCC: The GNU Compiler Collection, widely used in various operating systems.
- Visual Studio: A robust IDE by Microsoft, offering powerful tools for C++ development.
- Code::Blocks: An open-source IDE that supports multiple compilers.
Once you have your environment set up, you can write a simple program to demonstrate the `cout` statement:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this example, the program includes the `iostream` library necessary for input/output operations. Within the `main` function, `cout` outputs the string "Hello, World!" to the console. This is the simplest form of using `cout`, showcasing its core functionality.
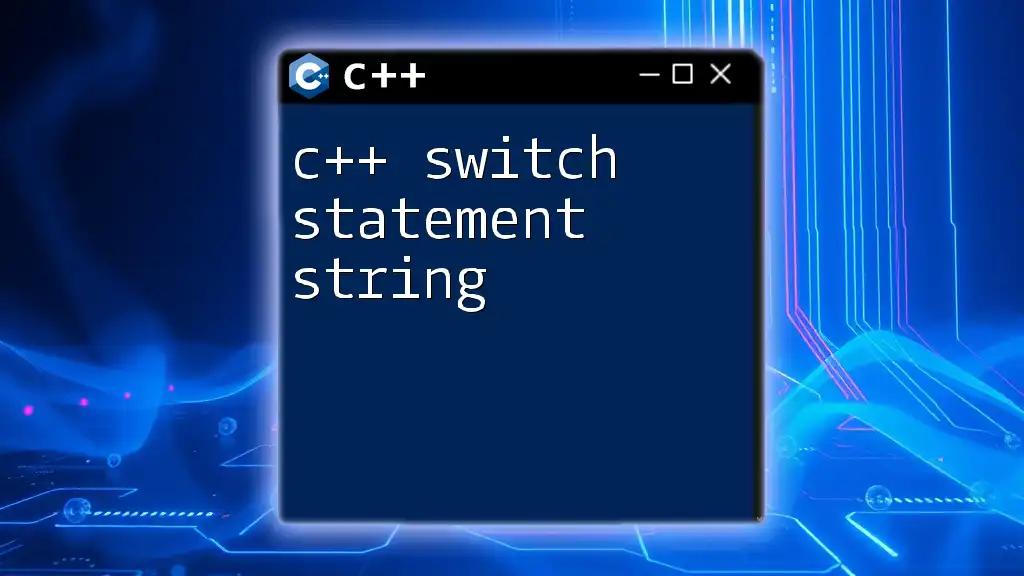
Utilizing cout for Different Data Types
One of the strengths of the c++ cout statement is its ability to handle various data types effortlessly.
Outputting Basic Data Types
Integer
To output an integer, you can write:
int age = 25;
std::cout << "Age: " << age << std::endl;
In this example, the program outputs the message "Age: 25". The `std::endl` statement not only adds a newline but also ensures that the output is flushed, meaning it is immediately shown on the screen.
Floating-Point Number
Outputting floating-point numbers is equally straightforward:
float height = 5.9;
std::cout << "Height: " << height << std::endl;
In this case, the program outputs "Height: 5.9".
Characters and Strings
You can also output characters and strings with `cout`:
char initial = 'A';
std::string name = "Alice";
std::cout << "Initial: " << initial << ", Name: " << name << std::endl;
Here, `cout` will display "Initial: A, Name: Alice", demonstrating its versatility in handling different data types.
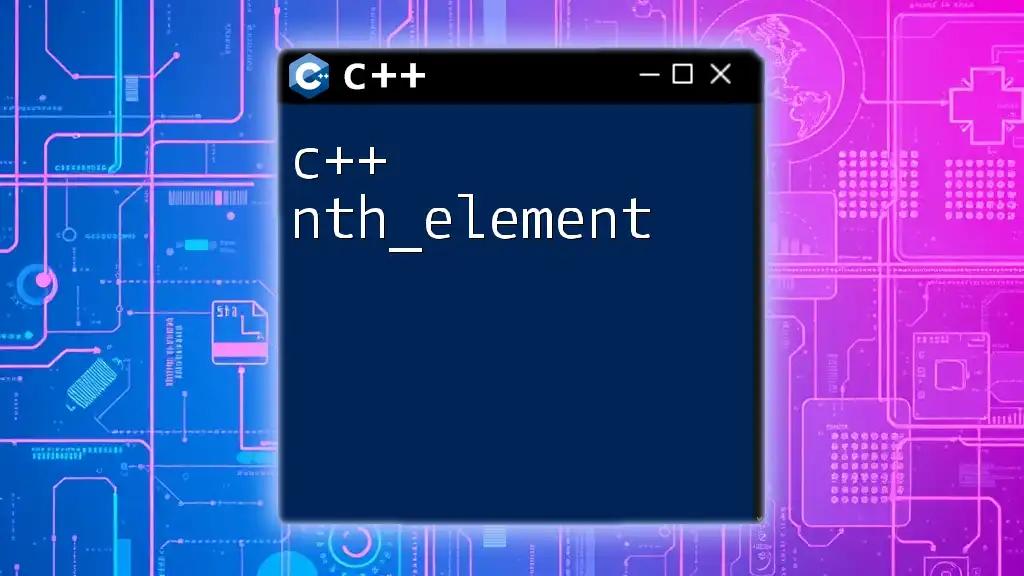
Formatted Output with cout
Sometimes, straightforward output isn't enough, and you may want to format your data for better readability.
Using std::endl
One way to manage output formatting is by using `std::endl`. This not only adds a new line but also flushes the output buffer:
std::cout << "Welcome to C++ programming!" << std::endl;
Adding Formatting
You can use manipulator functions such as `std::setw` and `std::setprecision` for more complex formatting:
#include <iomanip>
float pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << "Pi: " << pi << std::endl;
This code sets the number of decimal places shown to two, resulting in "Pi: 3.14". `std::fixed` ensures that the floating-point number is displayed in fixed-point notation rather than scientific notation.

Chaining cout Statements
A powerful feature of the c++ cout statement is the ability to chain multiple outputs together into a single statement. This helps keep your code concise while enhancing readability.
For instance, you might want to print multiple variables at once:
int a = 5, b = 10;
std::cout << "a: " << a << ", b: " << b << std::endl;
This statement outputs "a: 5, b: 10", demonstrating how easy it is to combine different pieces of information into one line.
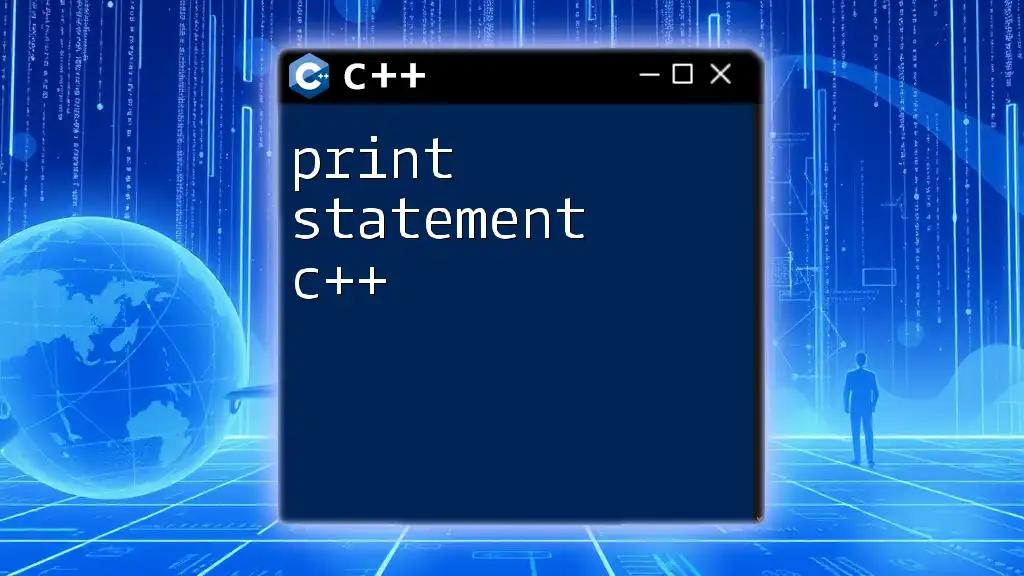
Error Handling with cout
Good programming practices include handling potential errors, and managing output is no different. You can check if the output operation succeeded with:
if (std::cout.good()) {
std::cout << "Output successful!" << std::endl;
} else {
std::cerr << "Output failed!" << std::endl;
}
This code uses `std::cerr` to print an error message to the standard error stream, helping you identify issues when output fails.
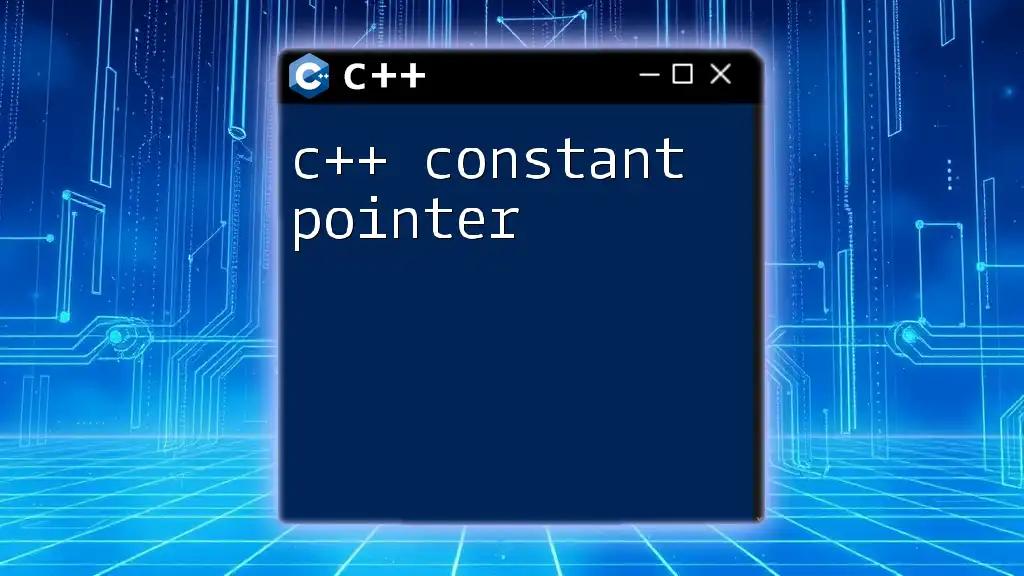
Best Practices for Using cout in C++
To make the most of the c++ cout statement, consider these best practices:
-
Clarity and Readability: Always aim for concise and clear output. Use full sentences and understandable messages to help users understand the information being provided.
-
Consistent Formatting: Strive for uniformity in how you output data. If you're frequently displaying numbers, choose a format and stick with it throughout your application to avoid confusion.

Conclusion
The c++ cout statement is a powerful and essential element of the C++ language, enabling smooth, flexible output of varying data types. Through mastering its usage—from basic commands to formatted output and good practices—you can effectively enhance your programming skills and provide clear communication to users within your applications.
As you become more comfortable using `cout`, challenge yourself to create increasingly complex and informative outputs as you code. The more you practice, the more proficient you'll become in leveraging this vital tool in your programming arsenal.
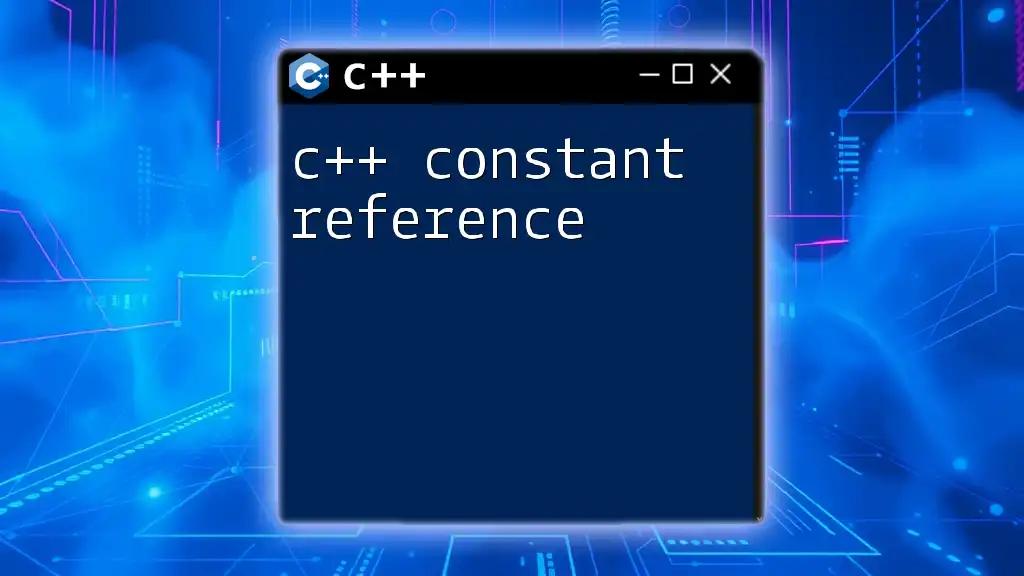
Additional Resources
For those looking to deepen their understanding of the c++ cout statement and related topics, consider referring to official C++ documentation, and various online tutorials, or diving into programming books dedicated to C++. These resources will help broaden your knowledge and facilitate your journey in mastering C++.