The C++ new placement operator allows you to construct an object at a specific memory address by combining the `new` keyword with a pointer to that address, allowing for more control over memory management.
#include <new> // Needed for placement new
#include <iostream>
struct MyObject {
MyObject() { std::cout << "MyObject constructed!\n"; }
~MyObject() { std::cout << "MyObject destructed!\n"; }
};
int main() {
char buffer[sizeof(MyObject)]; // Allocate raw memory
MyObject* obj = new (buffer) MyObject(); // Use placement new
obj->~MyObject(); // Manually call the destructor
return 0;
}
What is Placement New?
Placement new in C++ is a specialized version of the new operator that allows you to specify the memory location where an object should be constructed. Unlike the traditional new operator, which allocates memory and constructs the object in one go, placement new utilizes a pre-allocated buffer. This method grants the developer fine control over memory management and is particularly useful in performance-critical applications.
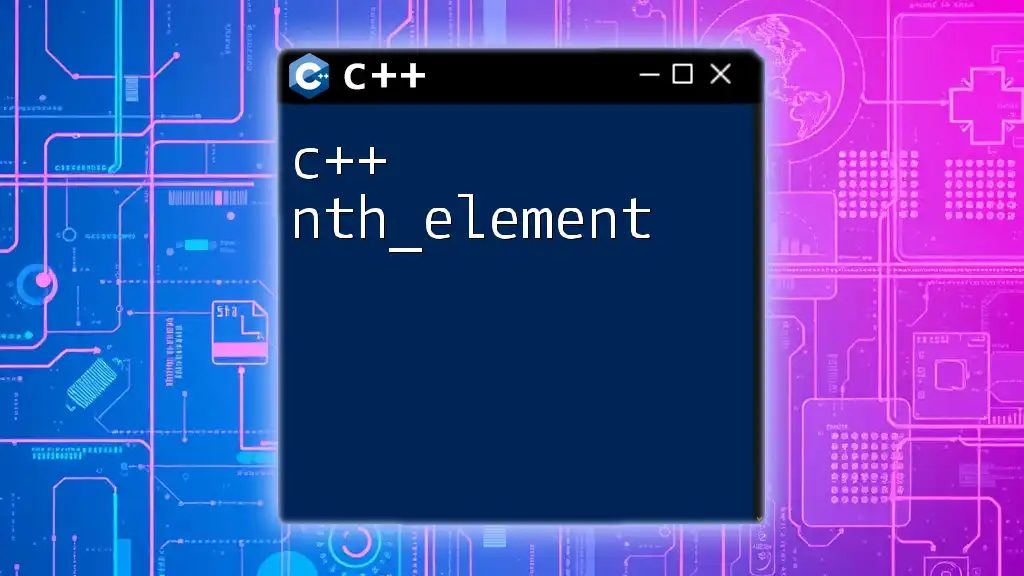
Why Use Placement New?
The primary reasons for using placement new include improved memory management and enhanced performance. By allowing direct control over where an object is instantiated, it helps to minimize memory fragmentation and optimize the allocation process. Moreover, it enables meticulous control over the lifetime of objects, making it especially handy in scenarios where performance is paramount, such as real-time systems and game development.
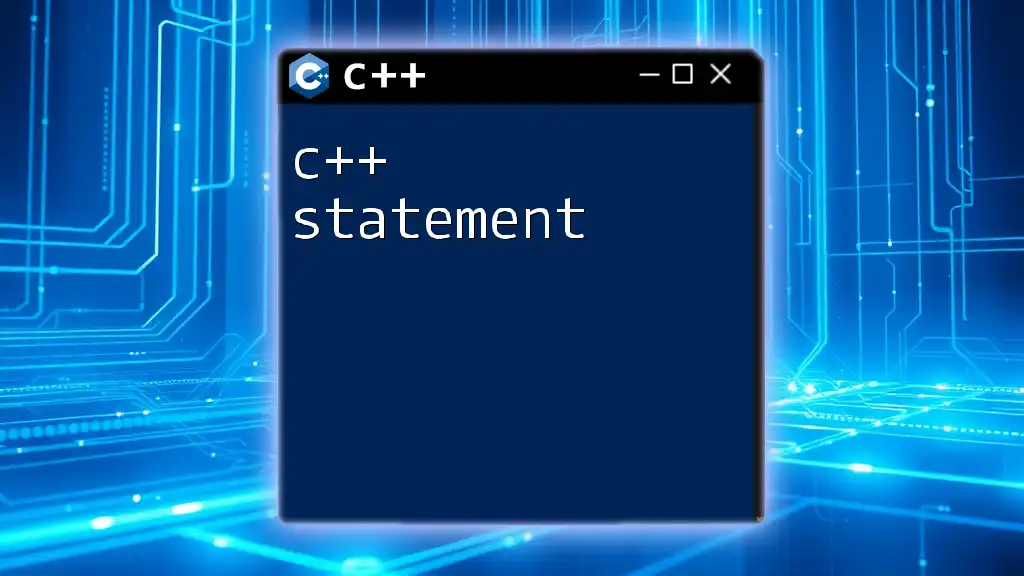
Understanding Memory Management
Basics of Memory Allocation
Memory in C++ is primarily managed through two different techniques: dynamic and static allocation.
- Dynamic Memory Allocation: Managed on the heap, allows for allocation and deallocation at runtime. Constructs objects using the regular new operator.
- Static Memory Allocation: Managed on the stack, automatically allocated and deallocated based on scope.
Traditional New in C++
The regular new operator allocates memory for an object on the heap and invokes its constructor. While this handles most of the memory management seamlessly, it comes with drawbacks like potential memory fragmentation and overhead due to dynamic memory allocation.
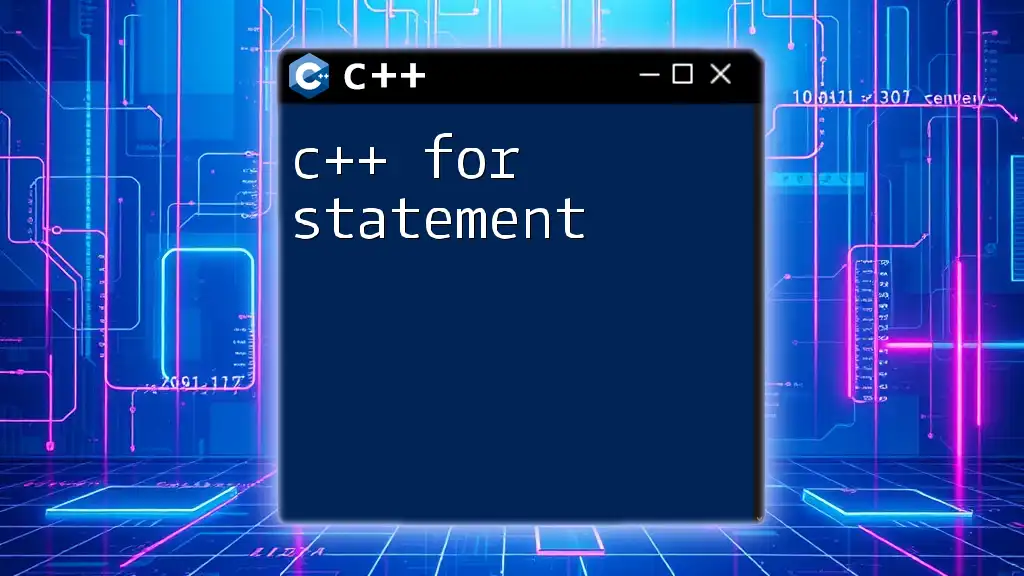
How Placement New Works
Syntax of Placement New
The syntax for placement new involves using the placement new operator in conjunction with a pointer to an appropriately sized buffer. Here’s how it looks:
#include <new> // Required header
void* buffer = operator new(sizeof(MyClass)); // Allocate raw memory
MyClass* obj = new (buffer) MyClass(); // Placement new
In this example, `operator new` is used to allocate raw memory, and placement new constructs an object of type `MyClass` at the specified memory location.
Allocating Raw Memory
Using `operator new`, you can allocate raw memory without invoking any constructors immediately. This decision allows you to control when, and if, you instantiate an object within that memory block.
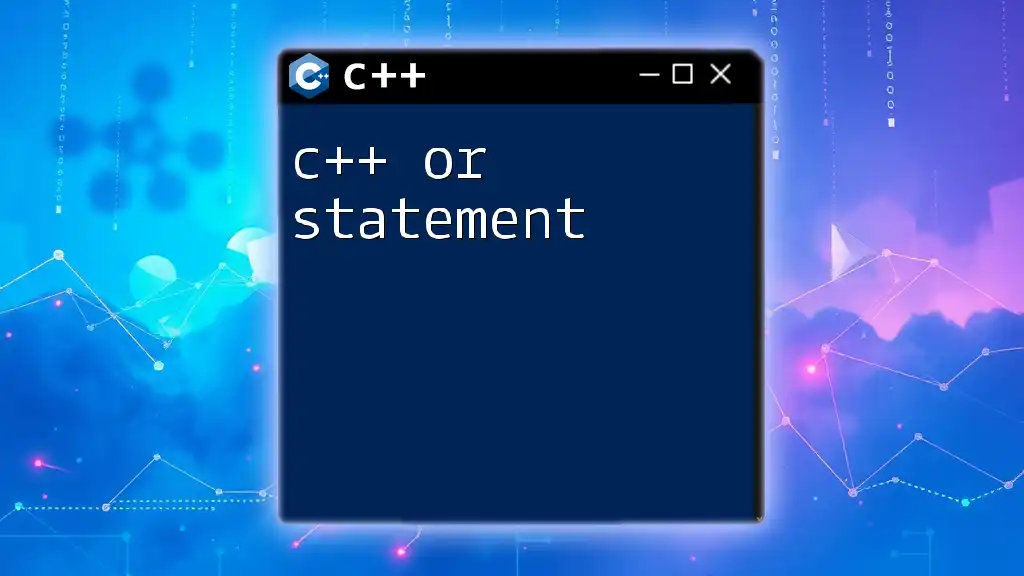
Using Placement New in C++
Example of Placement New
Let’s look at a detailed example to solidify understanding:
#include <iostream>
#include <new>
class MyClass {
public:
MyClass() { std::cout << "Constructor called." << std::endl; }
~MyClass() { std::cout << "Destructor called." << std::endl; }
};
int main() {
char buffer[sizeof(MyClass)]; // Step 1: Allocate raw memory
MyClass* obj = new (buffer) MyClass(); // Step 2: Construct object using placement new
obj->~MyClass(); // Step 3: Manually call destructor
return 0;
}
In this example, we first allocate a block of memory large enough to hold an instance of `MyClass` within a char array named `buffer`. Next, we use placement new to construct our object within this memory space. Finally, we must call the destructor manually since placement new does not automatically handle this when the object goes out of scope.
Common Use Cases
Placement new is ideal for scenarios where you're working with pre-allocated memory. Common use cases include creating objects in a specific memory buffer or within complex data structures, such as custom allocators or memory pools, which can enhance both performance and memory efficiency.
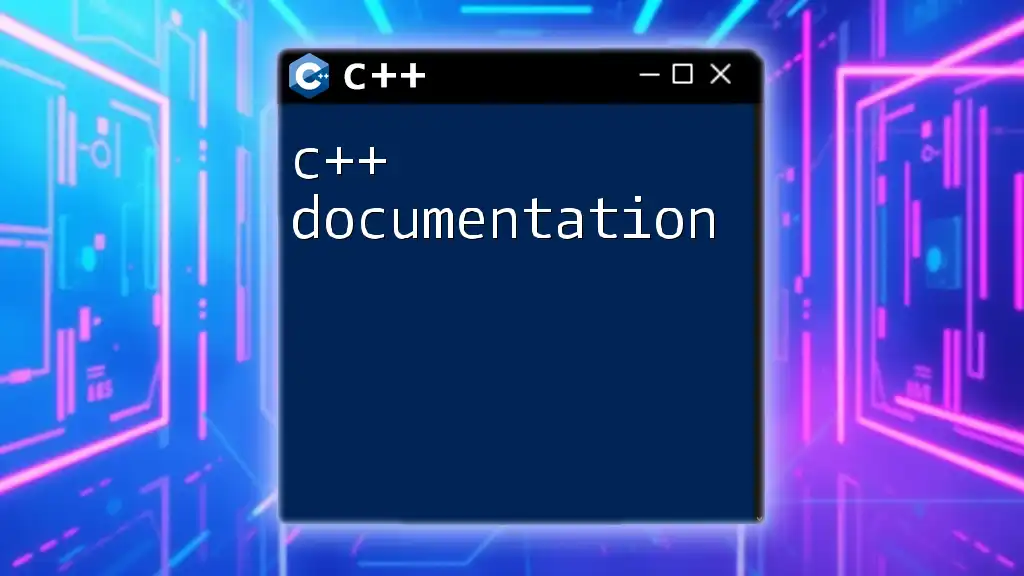
Advantages of Placement New
Efficiency in Resource Management
Placement new drastically reduces memory fragmentation by utilizing pre-allocated memory regions. Unlike regular new which may scatter objects across the heap, placement new allows for objects to be stacked neatly in a designated area.
Flexibility in Object Creation
With placement new, you gain the flexibility to create multiple objects in the same buffer. You can use specialized constructors as needed without having to manage separate memory allocations for each object, which streamlines resource handling.
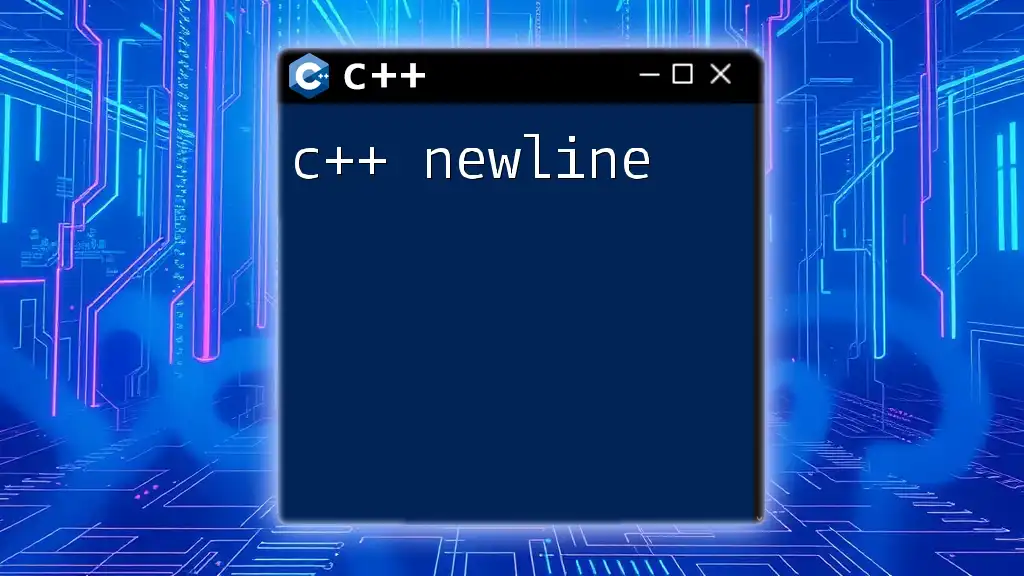
Potential Pitfalls and Best Practices
Common Mistakes
One of the most common mistakes when using placement new involves forgetting to call the destructor manually. Since objects created with placement new do not have their destructors called automatically, this oversight can lead to resource leaks. Additionally, misusing placement new within memory regions that will be overwritten can corrupt data.
Best Practices
To ensure proper memory management when using placement new:
- Always confirm that you'll call the destructor manually after you are done with the object.
- Utilize smart pointers and RAII (Resource Acquisition Is Initialization) principles where possible to automate resource handling.
- Use placement new judiciously, only in scenarios where the performance gains outweigh added complexity.
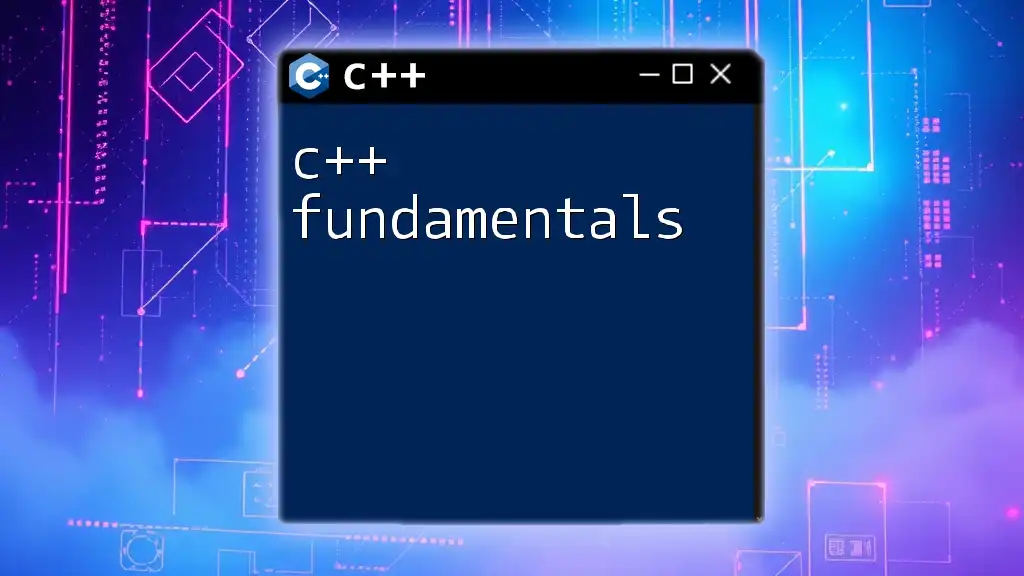
Conclusion
Placement new provides advanced C++ programmers with precise control over memory allocation and object lifetimes. Understanding its intricacies and applications not only enhances code performance but also allows for more manageable memory usage. By grasping the concepts surrounding placement new, you prepare yourself to tackle the challenges of modern C++ with confidence.
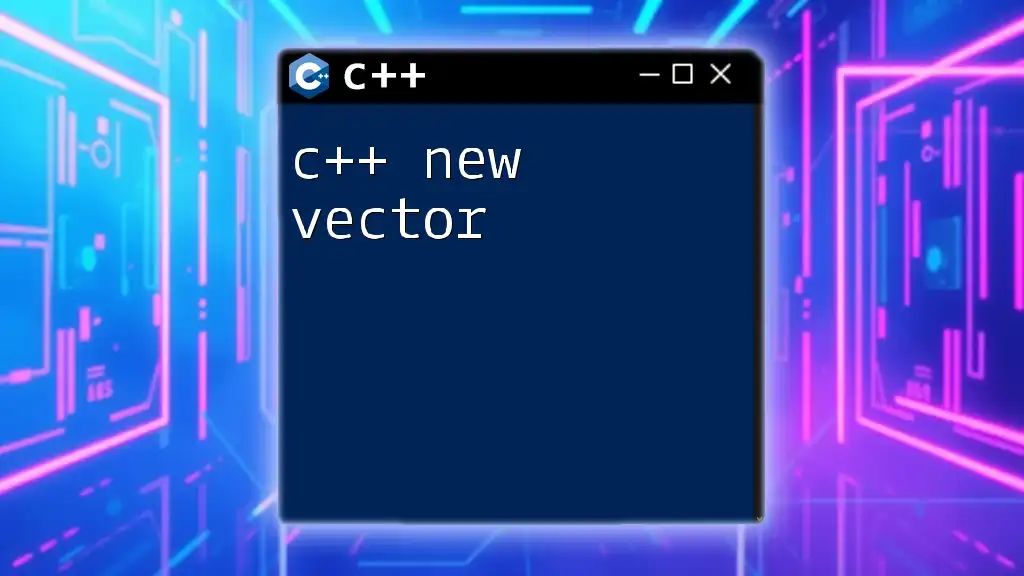
References and Further Reading
For those interested in delving deeper into the subject, consider exploring the following resources:
- The C++ Standard Library documentation
- "Effective C++" by Scott Meyers
- Online tutorials and forums focused on C++ memory management techniques.