The C++ decrement operator (`--`) decreases the value of its operand by one and can be used either in prefix or postfix form.
Here’s a code snippet demonstrating both forms:
#include <iostream>
int main() {
int x = 5;
// Postfix decrement
std::cout << "Postfix: " << x-- << std::endl; // Outputs 5, then x becomes 4
std::cout << "Current value of x: " << x << std::endl; // Outputs 4
// Prefix decrement
std::cout << "Prefix: " << --x << std::endl; // x becomes 3, then outputs 3
return 0;
}
What is the Decrement Operator in C++?
In C++, the decrement operator (--) is an essential arithmetic operator that reduces the value of a variable by one. Understanding this operator is crucial for efficient programming, as it frequently appears in loops and various control structures. The decrement operator comes in two types: prefix and postfix.
Types of Decrement Operators
Prefix Decrement Operator
The prefix decrement operator decreases the value of a variable before it is used in an expression. This means that when the prefix operator is applied, the variable is first decremented, and then its new value is used.
How it works:
- In a line like `int b = --a;`, `a` is decremented first, and then `b` receives the decremented value.
- This is useful in scenarios where you want the updated value immediately for further calculations.
Example of Prefix Decrement in C++:
int a = 5;
int b = --a; // a is decremented to 4, then b is set to 4
After executing this code, both `a` and `b` will contain the value 4.
Postfix Decrement Operator
The postfix decrement operator operates differently. It decreases the value of a variable after it has been used in an expression. This means that the original value is used in calculations, and only then is the variable decremented.
How it works:
- In a statement like `int b = a--;`, `b` gets the current value of `a`, and then `a` is decremented.
- This is particularly useful when you want to use the current value in a condition while still planning to reduce it afterward.
Example of Postfix Decrement in C++:
int a = 5;
int b = a--; // b is set to 5, then a is decremented to 4
After running this snippet, `b` will hold the value 5 while `a` will be 4.
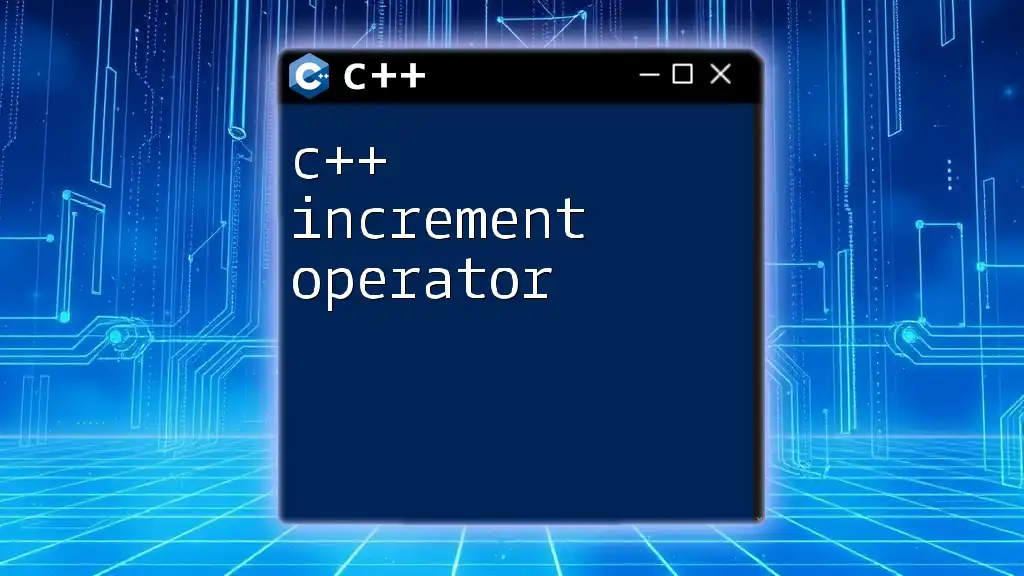
Practical Uses of the Decrement Operator
The decrement operator is commonly used in loops, such as for and while loops, where a counter needs to be decreased in each iteration.
Example of Decrement in Loop
for (int i = 5; i > 0; --i) {
std::cout << i << " ";
}
This loop will print the numbers 5 through 1. Here, the use of the prefix decrement operator (--i) ensures that the value of `i` is decreased before the next iteration starts.
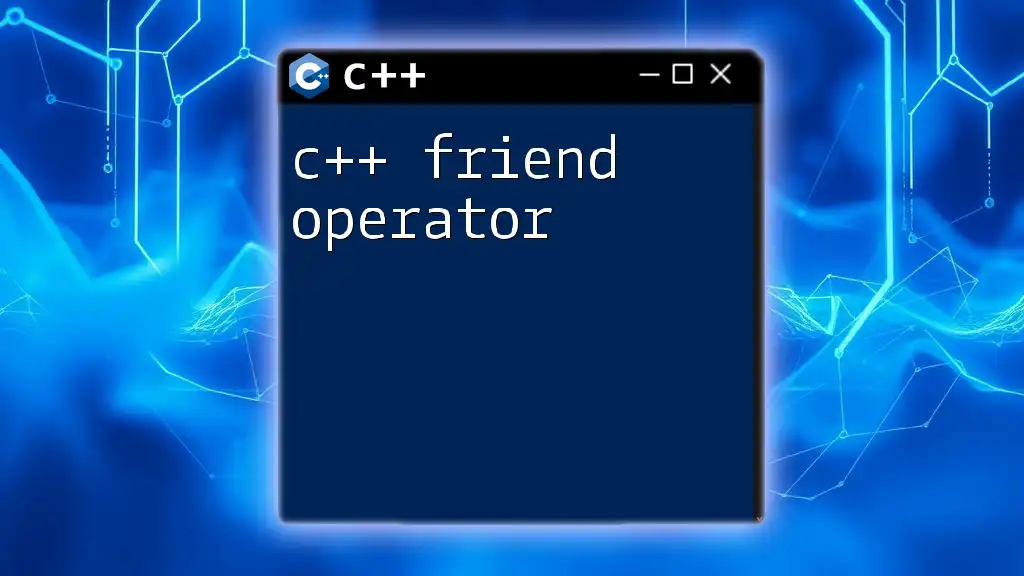
Understanding Decrement in Context
Difference Between Increment and Decrement
While both the increment (`++`) and decrement (`--`) operators serve the purpose of adjusting values by one, they are used in opposite directions. The increment operator increases the value of a variable, while the decrement operator reduces it.
Example Comparing Increment and Decrement:
int a = 5;
int b = ++a; // Increment (b = 6)
int c = --b; // Decrement (c = 5)
In this example, `b` first becomes 6 after the increment, and then `c` reverts back to 5 after decrementing `b`. Recognizing how these two operators interact is key to mastering your C++ coding practices.
Decrement Operator with Data Types
The decrement operator is versatile and can be used with various data types, including integers, floating points, and user-defined types. Understanding how it interacts with different data types can prevent bugs and ensure your program behaves as expected.
Code Snippet Example:
double num = 10.5;
num--; // num is now 9.5
In this case, the value of `num` decreases from 10.5 to 9.5, illustrating the decrement operator's behavior with floating-point numbers.
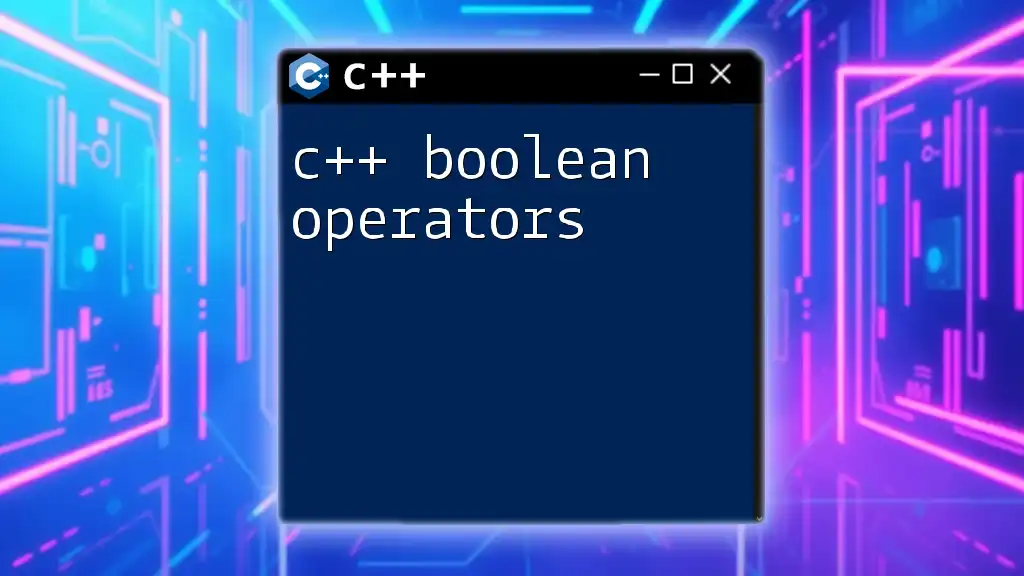
Best Practices When Using Decrement
To utilize the decrement operator effectively in C++, it is vital to steer clear of common pitfalls, like mistaking prefix for postfix or mismanaging the decrement in loops. These mistakes can lead to logical errors that are sometimes difficult to debug.
Tips for Efficient Code
- Avoid Confusion: Be conscious of when to use prefix vs. postfix to ensure that your logic remains consistent.
- Readability: In critical code segments, consider using comments to clarify your intention, especially if it's not immediately clear why a decrement is being used.
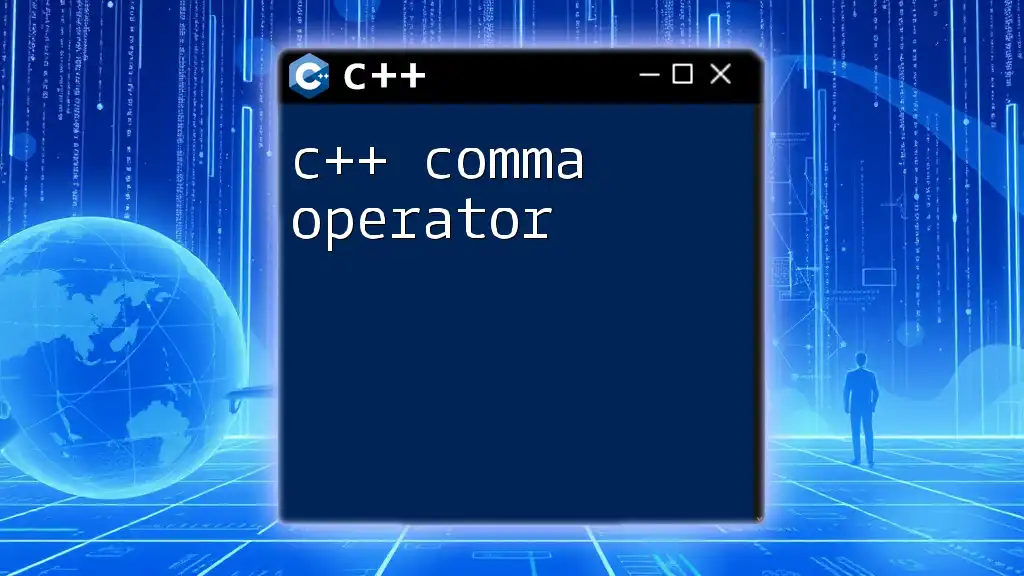
Conclusion
The C++ decrement operator is a powerful tool that plays a pivotal role in arithmetic operations and logic control structures. By mastering both the prefix and postfix variations, you can significantly enhance your programming efficiency and design. Practice implementing the decrement operator in your own C++ projects, and take a moment to reflect on how it interacts with increment operators for a deeper understanding of these fundamental concepts.
For those eager to dive deeper, exploring additional resources or engaging in practice problems related to the decrement operator can further solidify your skills in C++. Whether you're a complete beginner or an experienced programmer, understanding the nuances of the decrement operator is a stepping stone toward becoming proficient in C++.