The C++ spaceship operator (`<=>`) is a three-way comparison operator introduced in C++20 that simplifies the process of implementing comparison operators by allowing you to define a single operator for all relational operations.
#include <iostream>
struct Example {
int value;
// Define the spaceship operator
auto operator<=>(const Example& other) const = default;
};
int main() {
Example a{5};
Example b{10};
if (a < b) {
std::cout << "a is less than b" << std::endl;
}
return 0;
}
What is the Spaceship Operator?
The C++ spaceship operator, denoted by `<=>`, is a newly introduced feature in C++20 designed to facilitate a simpler and more efficient way of comparing objects. This operator is also known as the "three-way comparison operator," as it returns an indication of whether one object is less than, equal to, or greater than another.
Why Use the Spaceship Operator?
When developing C++ applications, comparison operations are frequently necessary, especially in classes. Traditionally, programmers often found themselves implementing multiple comparison operators (like `<`, `<=`, `>`, `>=`, `==`, and `!=`) for classes. The spaceship operator makes this cumbersome task substantially easier by allowing the automatic generation of these comparison operators, reducing boilerplate code.
By utilizing the spaceship operator, your code not only becomes easier to read, but also enhances its performance. This is particularly important in large applications where effective resource management and maintenance of clean code can significantly impact overall performance.
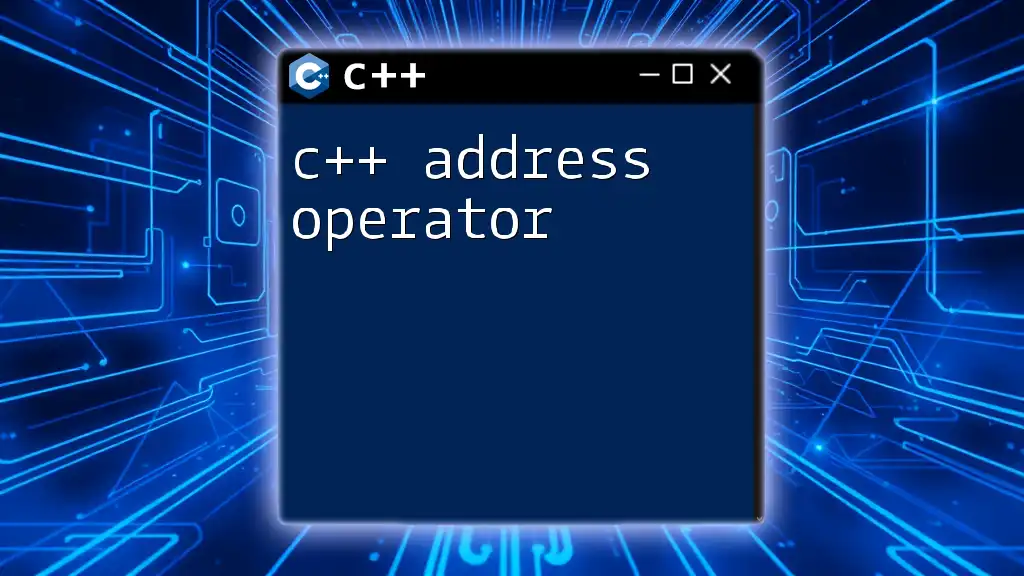
Understanding Operator Overloading
Overview of Operator Overloading
Operator overloading in C++ allows developers to define custom behavior for operators when they are used with user-defined types (classes). This is crucial for enhancing the expressiveness and usability of custom types.
How the Spaceship Operator Fits In
The spaceship operator stands apart from other operators as it consolidates multiple comparison operations into a single operator. This means that by implementing just this one operator, you can indirectly define the behavior of all six comparison operators for your class, streamlining your code significantly.
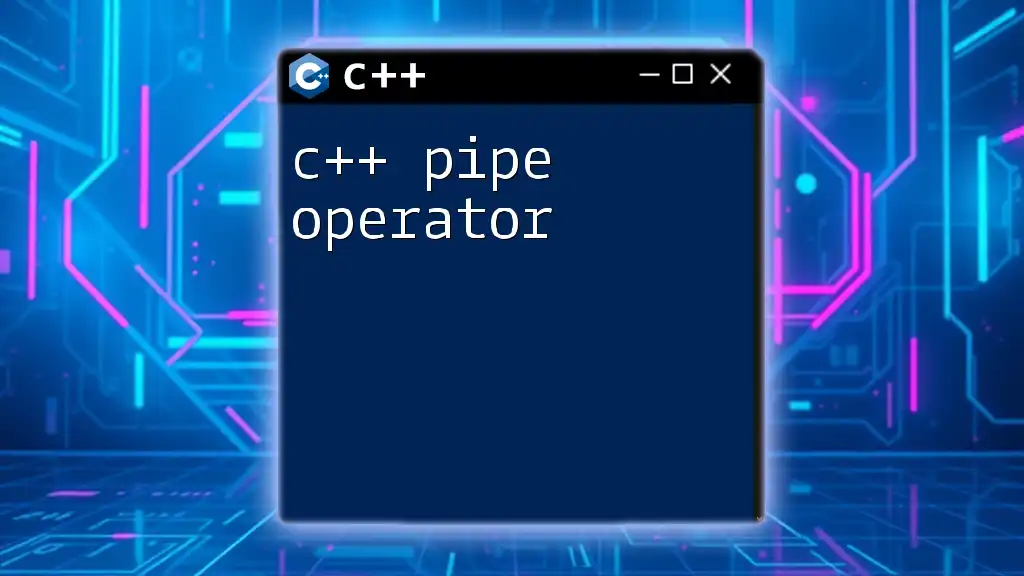
Practical Implementation of the Spaceship Operator
Syntax of the Spaceship Operator
The basic structure for implementing the spaceship operator is as follows:
auto operator<=>(const T& lhs, const T& rhs);
The return type can be essential, as it indicates the nature of the comparison. The operator can return one of three possible results: `std::strong_ordering`, `std::weak_ordering`, or `std::partial_ordering`, depending on how you choose to implement comparisons.
Step-by-Step Implementation Example
To illustrate how to implement the spaceship operator, let’s create a simple class called `Point` that represents a point in a 2D space.
class Point {
public:
int x;
int y;
// Spaceship operator
auto operator<=>(const Point& other) const = default;
};
In the example above, the `default` keyword allows the compiler to automatically implement the comparisons based on the members of the class. This reduces the workload for the programmer, as manual implementation isn't required unless custom behavior is desired.
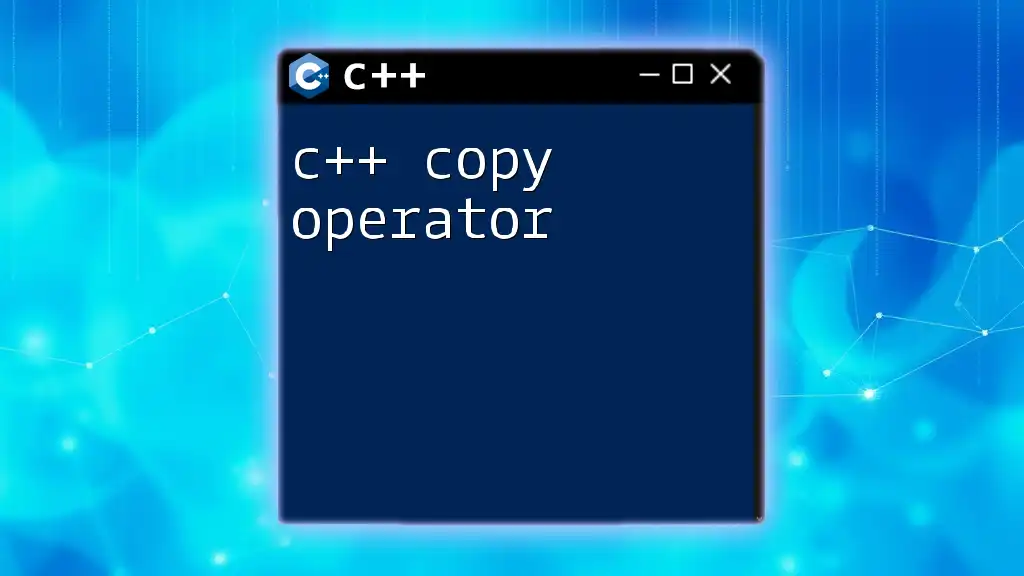
Detailed Comparison Modes
Strong vs. Weak vs. Partial Ordering
The spaceship operator supports various types of comparisons:
-
Strong Ordering: Determines a strict ordering based on all members of a class. For example, if one object is less than another, it will not be considered equal in any circumstances.
-
Weak Ordering: Allows for equivalence, meaning that two different objects can be considered equal based on some criteria, while still retaining order based on others.
-
Partial Ordering: Applies when not all objects are comparable, introducing flexibility in how comparisons are handled.
How to Choose the Right Comparison for Your Class
When deciding which type of ordering to utilize, consider the context of your class. Ensure that the chosen logic aligns with the intended purpose of the objects you are defining. It’s critical that comparisons reflect the meaningful distinctions between the objects.
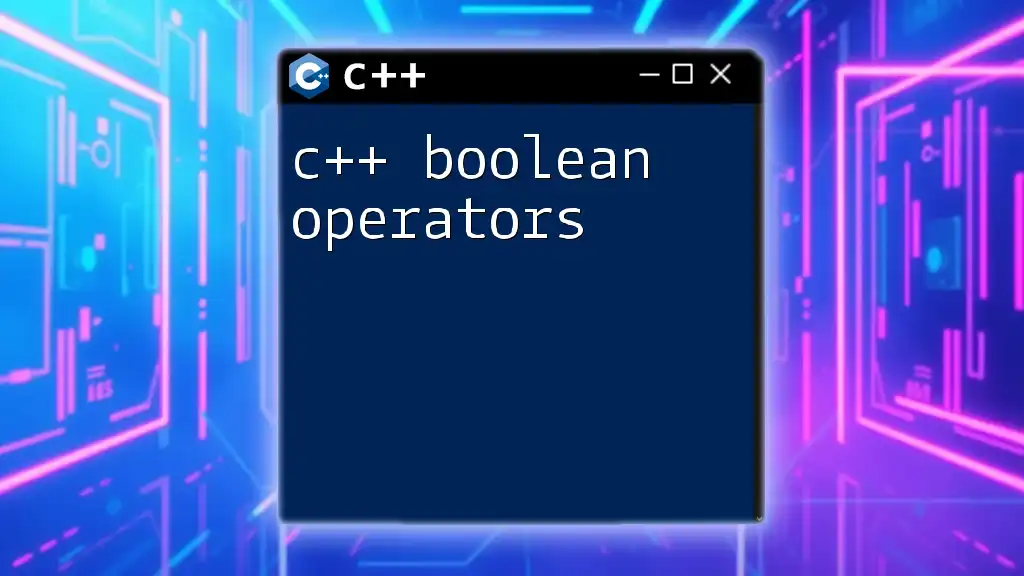
Practical Examples with Code Snippets
Example 1: Using the Spaceship Operator with Primitive Types
The spaceship operator can also apply to primitive types or standard classes. Here’s a simple example using integers:
#include <compare>
int main() {
int a = 5, b = 10;
auto result = (a <=> b);
// result will indicate which is greater
}
In this case, `result` will yield a value that signifies whether `a` is less than, equal to, or greater than `b`. This simple outcome conveys a clear comparison without manually defining operators.
Example 2: Custom Class with Multiple Members
Consider a more complex class, `Rectangle`, which features length and width:
class Rectangle {
public:
int length;
int width;
auto operator<=>(const Rectangle& other) const = default;
};
// Usage
Rectangle rect1{3, 4};
Rectangle rect2{4, 3};
bool isEqual = (rect1 <=> rect2) == 0;
In this example, the comparison will take both dimensions into account, providing a straightforward way to assess equivalency and order.
Example 3: Combining Different Comparison Types
Creating a class with important real-world implications, let's consider an `Employee` class defined as follows:
#include <compare>
class Employee {
public:
std::string name;
int id;
auto operator<=>(const Employee& other) const = default;
};
This implementation allows for seamless comparisons of `Employee` objects based on `name` and `id`, facilitating operations like sorting and searching.
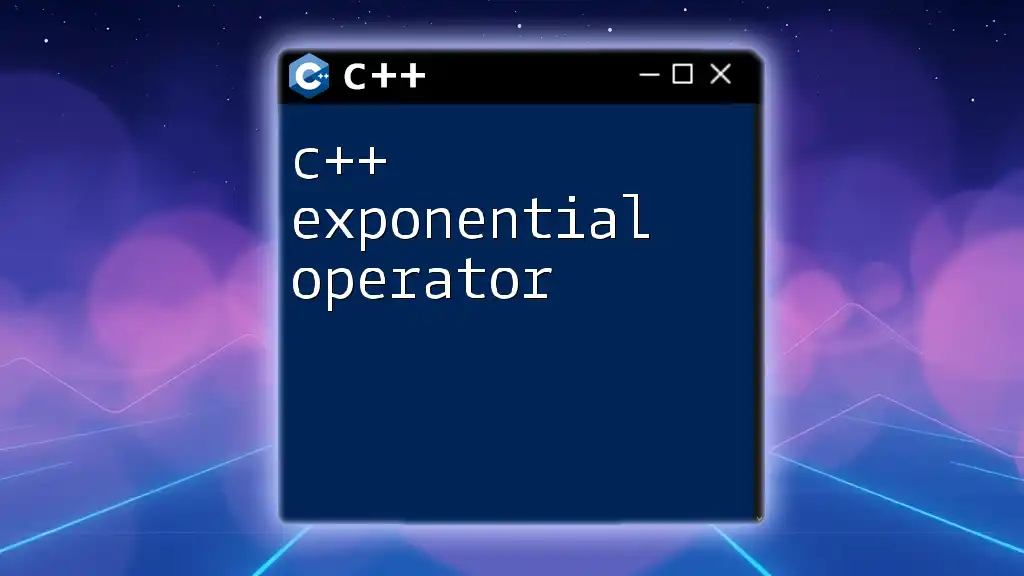
Best Practices for Using the Spaceship Operator
Clarity in Class Design
When designing classes, aim for clarity in their comparison logic. Classes should represent logical entities where comparisons make sense, helping to reduce confusion when reading or maintaining the code.
Testing Your Implementations
Testing is pivotal when using the spaceship operator. Ensure to create unit tests that assess the correctness of operator behavior. Here’s a common practice:
- Create instances of your class with known values.
- Compare them using the spaceship operator.
- Verify that the results align with your expectations.
By rigorously testing your custom operators, you safeguard against unexpected behavior in real-world applications.
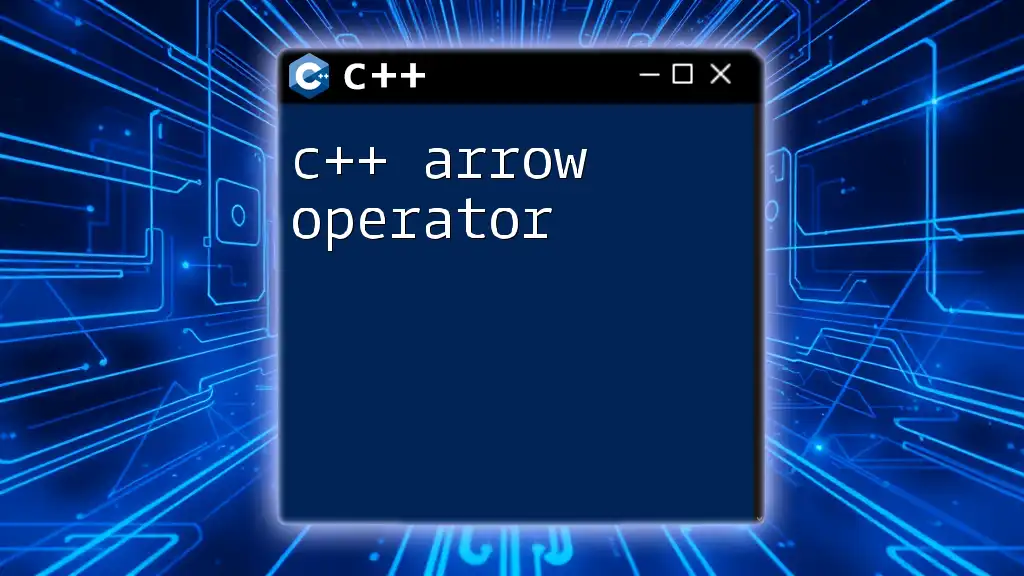
Conclusion
The C++ spaceship operator not only simplifies the implementation of comparison operators but also enhances the maintainability and readability of your code. By understanding its functionality and best practices, you can leverage this powerful feature to create effective and efficient C++ applications. As C++ continues to evolve, the spaceship operator remains a valuable tool for modern programming, making your code both elegant and performant.
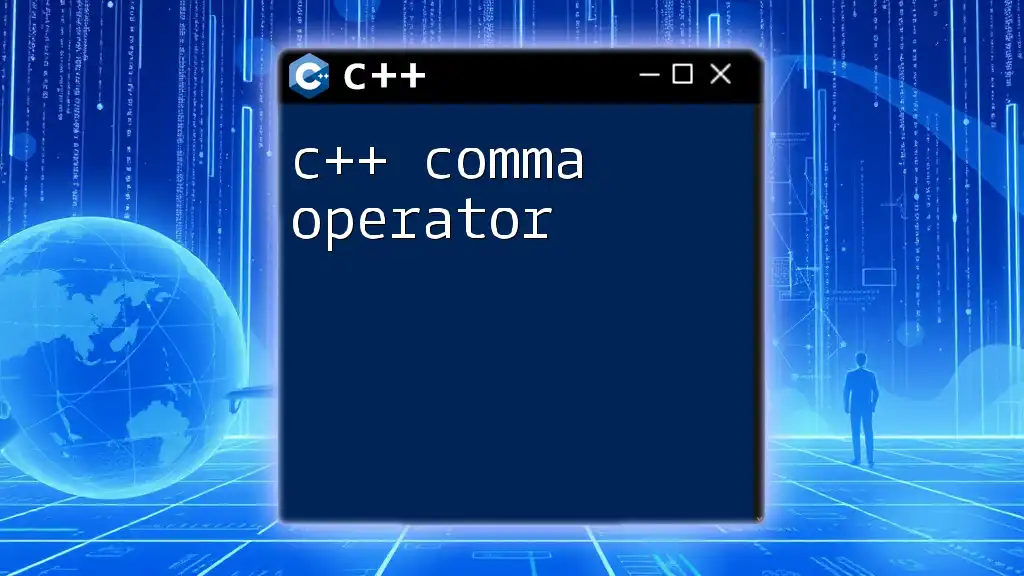
Additional Resources
To expand your knowledge further, refer to the C++20 Standard Library Documentation, explore online coding platforms for practice, and check out tutorials and courses that focus on C++ operator overloading. Engage with these resources to deepen your understanding and application of the C++ spaceship operator.
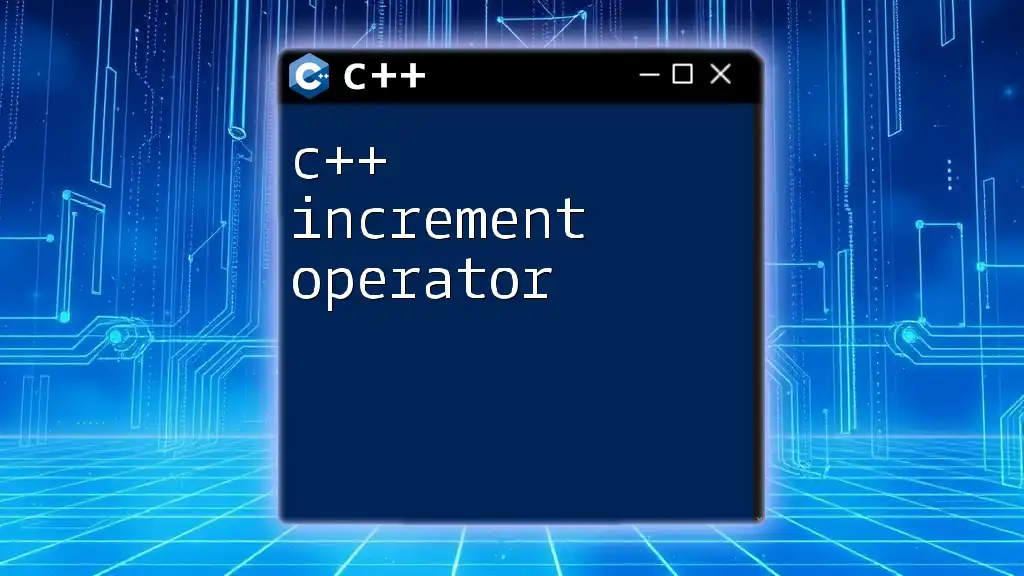
Call to Action
Try implementing the spaceship operator in your upcoming projects, and take advantage of its benefits for streamlined comparisons. Stay ahead in your C++ programming journey by subscribing for more concise tips and guides. Happy coding!