The C++ equal operator (`=`) is used to assign the value on its right to the variable on its left.
int x = 10; // Assigns the value 10 to the variable x
Understanding the C++ Equal Operator
The C++ equal operator is a fundamental part of the language, used primarily for comparison purposes. The syntax for the equality operator is straightforward: `a == b`, where it checks if the values of `a` and `b` are equal.
What Does It Compare?
The equality operator can compare various types, including primitive data types like integers, floats, and characters, as well as user-defined types such as classes and structs.
Basic Syntax
To use the operator, you simply write it as follows:
int a = 5;
int b = 5;
if (a == b) {
// Code to execute if a is equal to b
}
In this example, the condition evaluates to true, allowing the associated block of code to execute.
Conditions for Equality
Understanding how equality works is crucial, especially when it comes to different data types. Not all types behave similarly under comparison. When comparing two variables, they must be of compatible types. If they are not, implicit type coercion occurs, leading to potentially unexpected results.
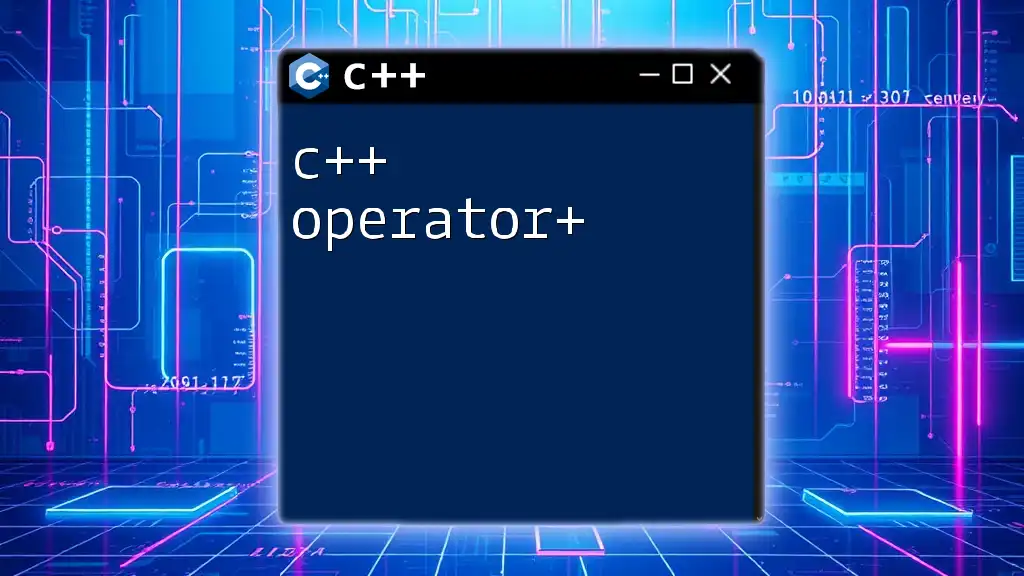
Types of Comparisons with the Equality Operator
Comparing Primitive Data Types
The equality operator can easily compare primitive data types such as integers, characters, and floating-point numbers.
Example:
char a = 'A';
char b = 'A';
if (a == b) {
// This condition is true
}
In this example, since both characters are 'A', the condition evaluates to true. When working with floating-point numbers, one must be cautious due to precision issues that can arise.
Explanation of Results
The equality operator checks for exact matches. If two integer or character values are the same, the result is true; if they differ, the result is false.
Comparing User-Defined Types
C++ allows you to create complex data types, such as classes and structs. However, these types require special attention if you want to compare their values using the equality operator.
Example of Comparison:
To enable the use of the equality operator, you need to overload it within the class:
class Point {
public:
int x, y;
bool operator==(const Point& other) const {
return (x == other.x && y == other.y);
}
};
In this code snippet, the `operator==` function checks if two `Point` objects are equal by comparing their `x` and `y` coordinates.
Why Overload?
Overloading the equality operator enables developers to define specific behavior that reflects the nature of the type being compared. This way, you maintain logical comparisons based on the attributes that are significant to the object.
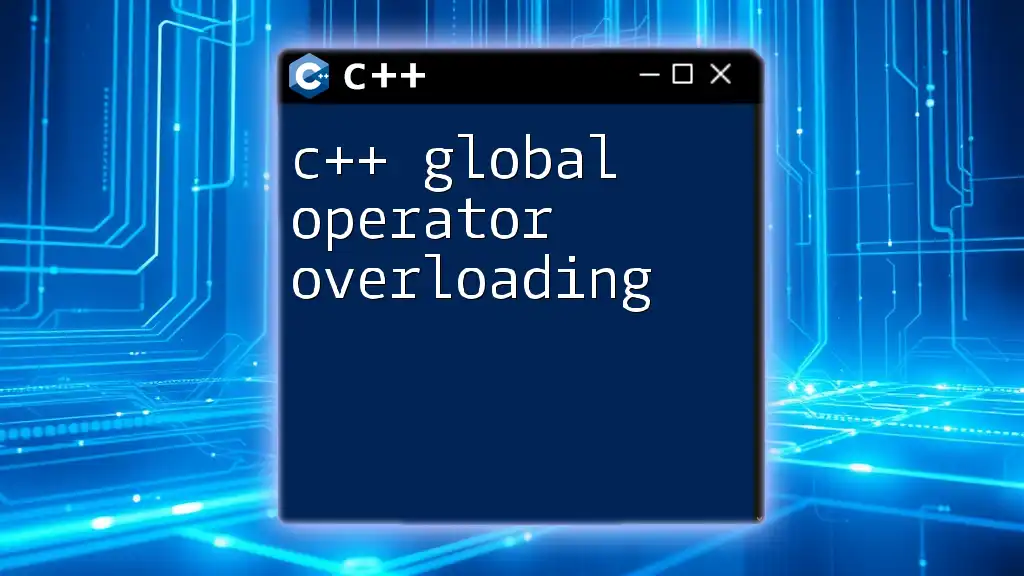
The Importance of Type Safety
Type safety is a key aspect of using the C++ equal operator. When comparing variables of different types, C++ attempts to perform implicit type conversion. This can lead to unexpected results, particularly with floating-point numbers or character types.
Type Coercion
While C++ handles type coercion automatically, it is essential to be aware of it. Implicit conversions can sometimes yield misleading outcomes in conditions. For instance, comparing an integer to a floating-point number may not behave as expected, depending on the values involved.
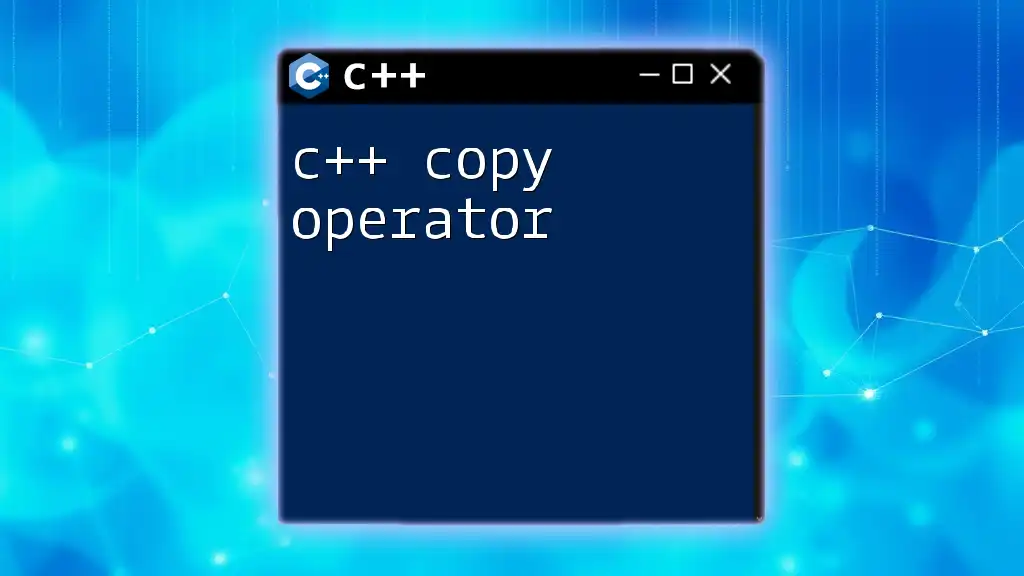
Common Pitfalls with C++ Equality Operator
Floating-Point Comparisons
Floating-point numbers introduce an additional layer of complexity due to precision errors. The expression `0.1 + 0.2 == 0.3` may not always yield true due to floating-point representation inaccuracies. Instead, it's advisable to check if the values are "close enough" within a small threshold value.
Example:
const double EPSILON = 1e-9;
double a = 0.1 + 0.2;
if (fabs(a - 0.3) < EPSILON) {
// Code for successful case...
}
Null Pointer Comparisons
Another common pitfall involves comparing pointers. When you compare pointers, particularly null pointers, it’s crucial to ensure that you're not attempting to dereference a null pointer, which could lead to undefined behavior.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
Best Practices When Using the C++ Equality Operator
Emphasize Readability
Always prioritize code readability. Using descriptive variable names and keeping comparisons clear will enhance maintainability. Avoid using complex expressions that make the intent less obvious.
Use Parentheses
In situations involving multiple comparisons, using parentheses can help clarify the order of operations and ensure that your comparisons are correctly evaluated.
Example:
if ((a == b) && (c == d)) {
// Clear and easy to understand
}
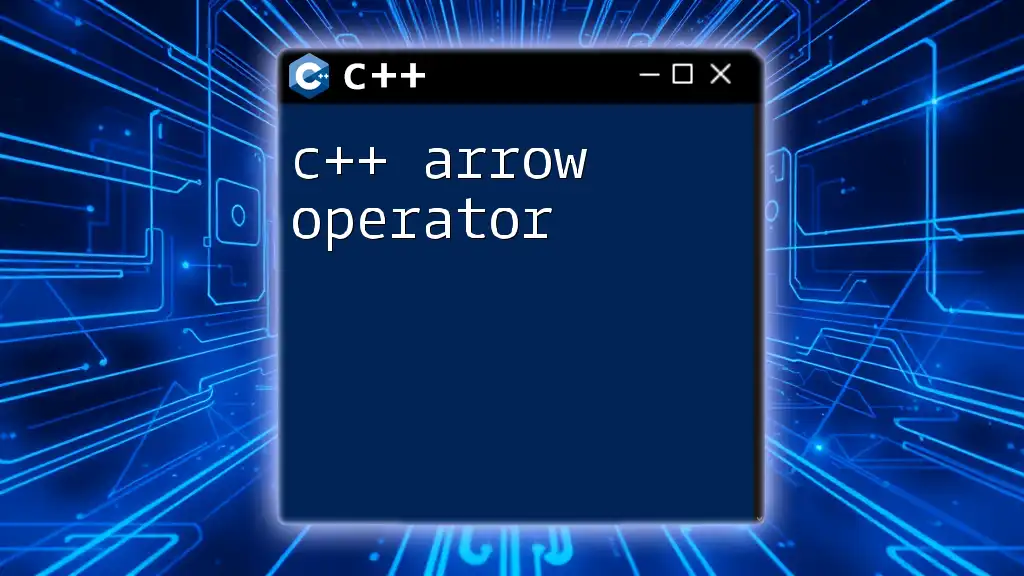
Real-World Applications of the C++ Equality Operator
The C++ equal operator is frequently used in conditional statements, making it essential in control flow. For instance, it can determine the path of execution in loops or if-else statements.
Examples:
- With `if` Statements:
if (userInput == expectedValue) {
// Code for successful case...
}
Here, the program checks if the user input matches an expected value, allowing you to handle user input effectively.
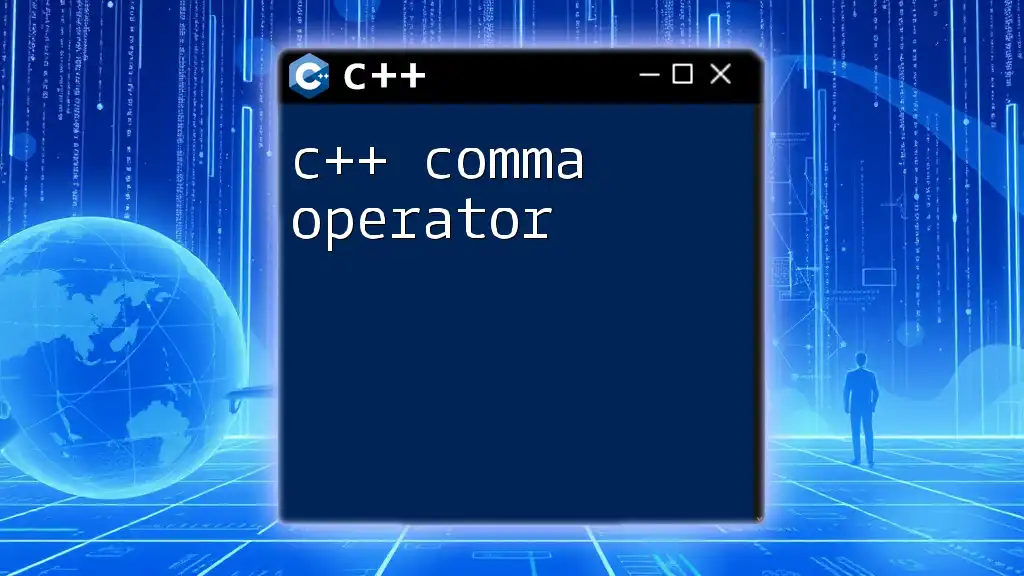
Alternative Operators
C++ Inequality Operator (`!=`)
Just as the equality operator is important, so is the inequality operator. The syntax is straightforward: `a != b`. This operator checks if two operands are not equal.
Example:
if (a != b) {
// Code here executes if a is not equal to b
}
The inequality operator is essential when defining conditions that rely on non-matching values, providing a simple solution for exclusion scenarios.
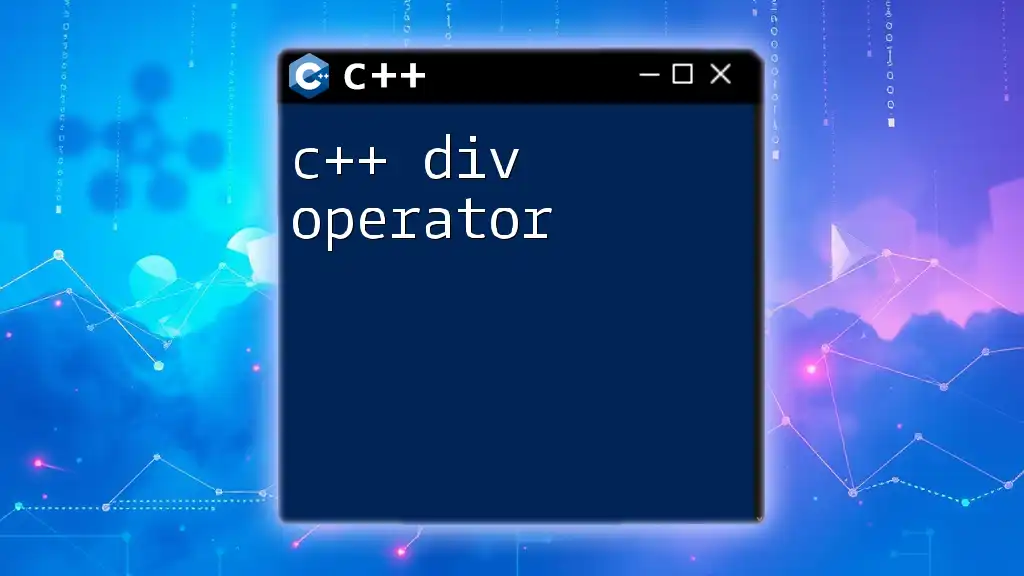
Conclusion
In summary, the C++ equal operator plays a vital role in the language, allowing for straightforward comparisons between various data types. Understanding its usage, pitfalls, and best practices will greatly enhance your ability to write efficient and effective C++ code. By practicing and applying the knowledge of equality in your projects, you can achieve better programming results.