In C++, the `auto` keyword allows for automatic type deduction, making it easier to declare iterators without explicitly specifying their types.
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (auto it = nums.begin(); it != nums.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Understanding Iterators in C++
What is an Iterator?
An iterator is an object that enables traversal through the contents of a container, such as arrays, vectors, lists, and more, without exposing the underlying representation of the data. Iterators act like pointers, allowing you to easily access elements within a collection through methods like increment (to move forward) and dereference (to access the element).
In C++, iterators are categorized into various types:
- Input Iterators: Allow read-only access to container elements.
- Output Iterators: Allow write-only access to container elements.
- Forward Iterators: Permit read/write operations and can only move in one direction.
- Bidirectional Iterators: Extend forward iterators by allowing moves in both directions.
- Random Access Iterators: Provide arbitrary access and full capabilities of pointer arithmetic.
Why Use Iterators?
Using iterators in your C++ code brings several advantages:
- Abstraction: Iterators provide a uniform interface for various containers, allowing you to iterate without needing to know the underlying data structure.
- Readability: Using iterators often leads to cleaner and more maintainable code compared to traditional loops. They allow you to focus on the logic rather than implementation details.
Example: Traditional loop vs. iterator usage.
// Traditional loop
for (int i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
// Iterator usage
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
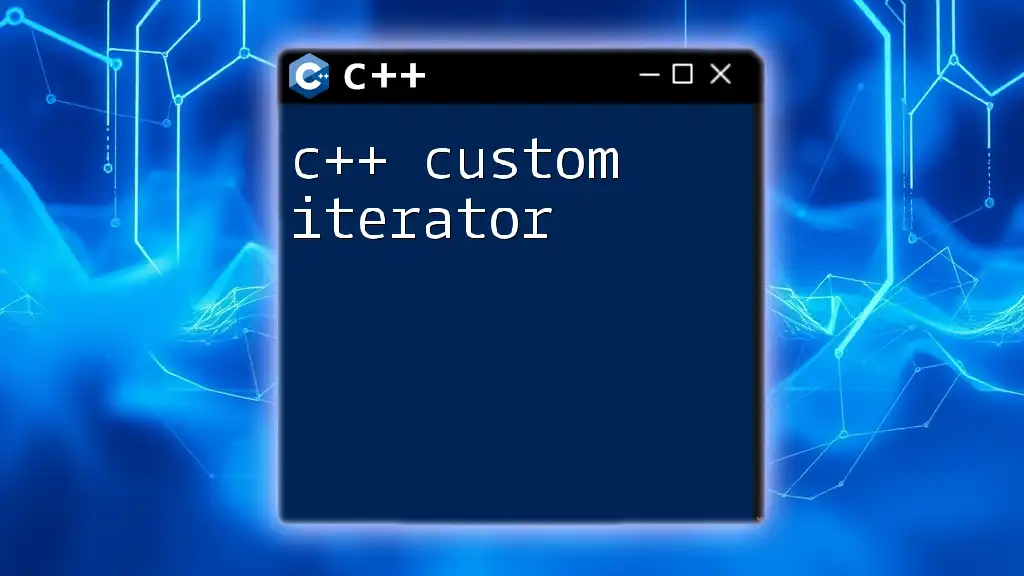
Introduction to the Auto Keyword
What is `auto` in C++?
The `auto` keyword in C++ serves to enable automatic type deduction. When you declare a variable using `auto`, the compiler determines its type based on the initializer provided. This can make your code shorter and eliminate type-related errors, especially when dealing with complex data types.
The Role of `auto` in Iterators
Combining `auto` with iterators simplifies the syntax. When you declare iterators, the type can frequently be lengthy and complicated (e.g., `std::vector<int>::iterator`). By using `auto`, you can make the code more concise and easier to understand, allowing you to focus on the logic.
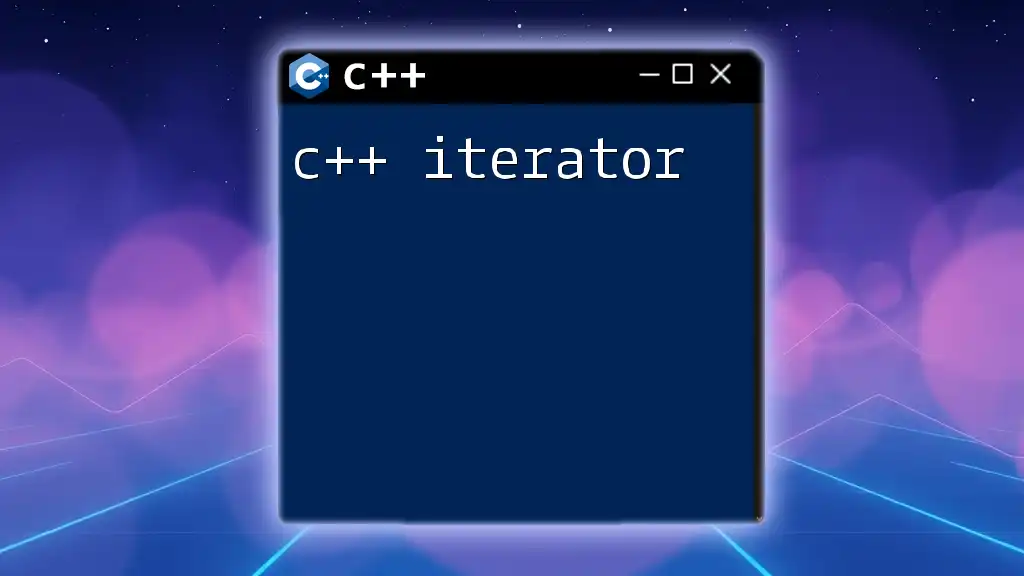
C++ Auto Iterators: A Deep Dive
Creating Auto Iterators
Creating auto iterators in C++ is straightforward. Here's how to declare and use an auto iterator with a standard container like `std::vector`.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
In this example, the `auto` keyword automatically deduces the type of `it` to be `std::vector<int>::iterator`, allowing clean and clear code.
Using `auto` with Different Containers
You can utilize `auto` with various standard library containers. Here are a few examples demonstrating how to iterate over different types of containers using `auto`:
Vectors
std::vector<std::string> fruits = {"Apple", "Banana", "Cherry"};
for (auto it = fruits.begin(); it != fruits.end(); ++it) {
std::cout << *it << " ";
}
Lists
std::list<char> letters = {'A', 'B', 'C'};
for (auto it = letters.begin(); it != letters.end(); ++it) {
std::cout << *it << " ";
}
Maps
std::map<int, std::string> studentGrades = {{1, "A"}, {2, "B"}, {3, "C"}};
for (auto it = studentGrades.begin(); it != studentGrades.end(); ++it) {
std::cout << it->first << ": " << it->second << " ";
}
Range-based for Loops with Auto
The advent of range-based for loops in C++11 makes it even easier to work with containers. By using `auto`, you can succinctly iterate over the elements in a collection.
for (auto fruit : fruits) {
std::cout << fruit << " ";
}
This approach allows you to iterate without manually handling iterators, promoting cleaner and more readable code.
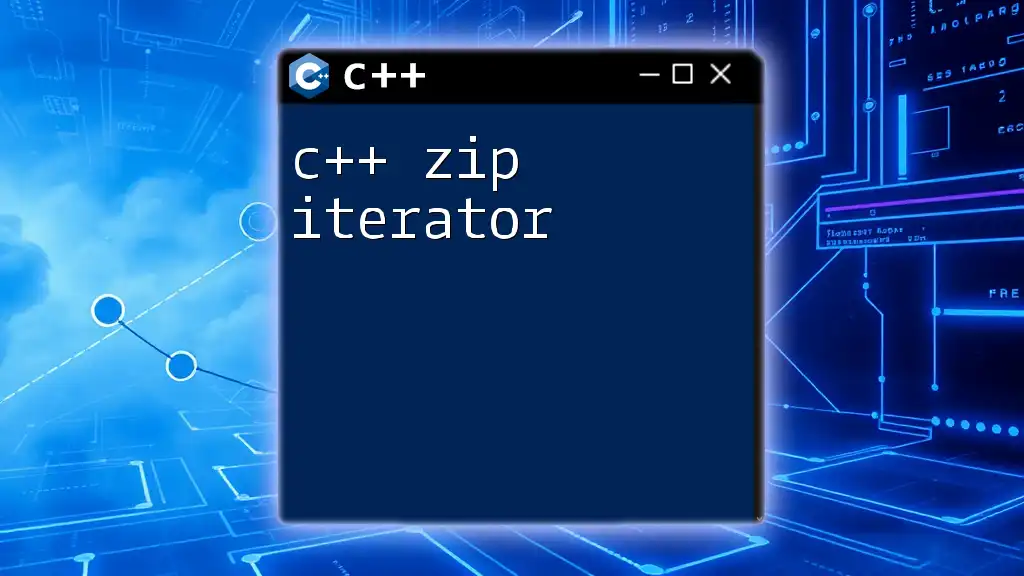
Best Practices Using Auto Iterators
When to Use `auto`
While `auto` can significantly simplify code, there are guidelines to consider:
- Prefer `auto` when the type is obvious from the context or when dealing with complex types.
- Avoid using `auto` if it introduces ambiguity or makes it harder to understand the code — especially in cases of overloaded operators.
Performance Considerations
Using `auto` with iterators can have several benefits regarding performance. It reduces the possibility of errors due to mismatched types and enables the compiler to optimize operations better. However, while `auto` aids in readability and prevents type mismatches, it is crucial to understand the underlying types for context-specific optimizations in performance-critical applications.
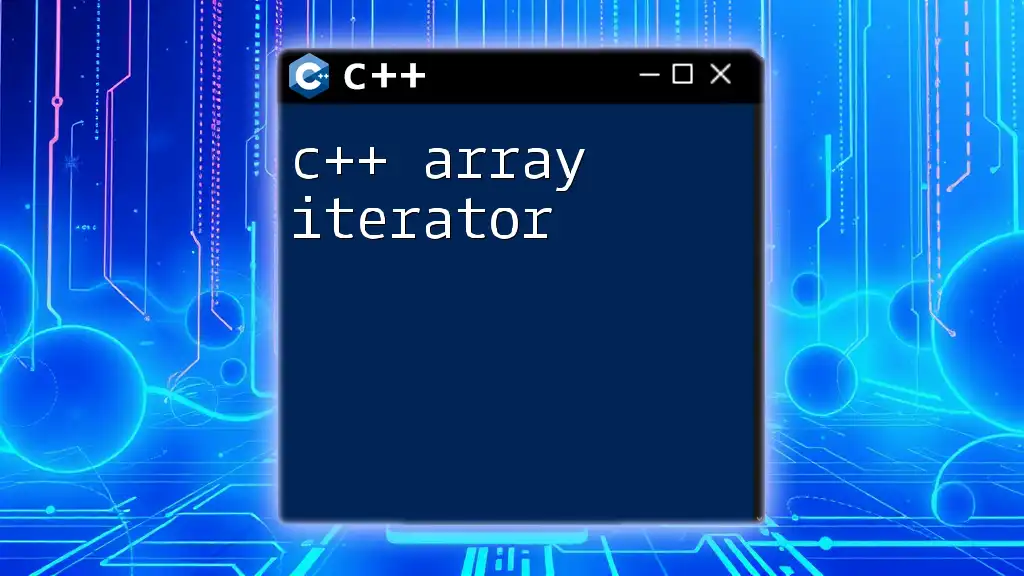
Common Mistakes with Auto Iterators
Misusing `auto` with Iterators
One of the common pitfalls of using `auto` is misinterpreting the deduced type. For example, if you attempt to mix different iterator types or containers, it can lead to unexpected behavior.
Debugging Issues Related to Auto Iterators
When it comes to debugging, the use of `auto` can sometimes make it difficult to track down type-related problems. Utilize static analysis tools or compile-time checks to ensure that types are as expected, especially when dealing with complex collections.
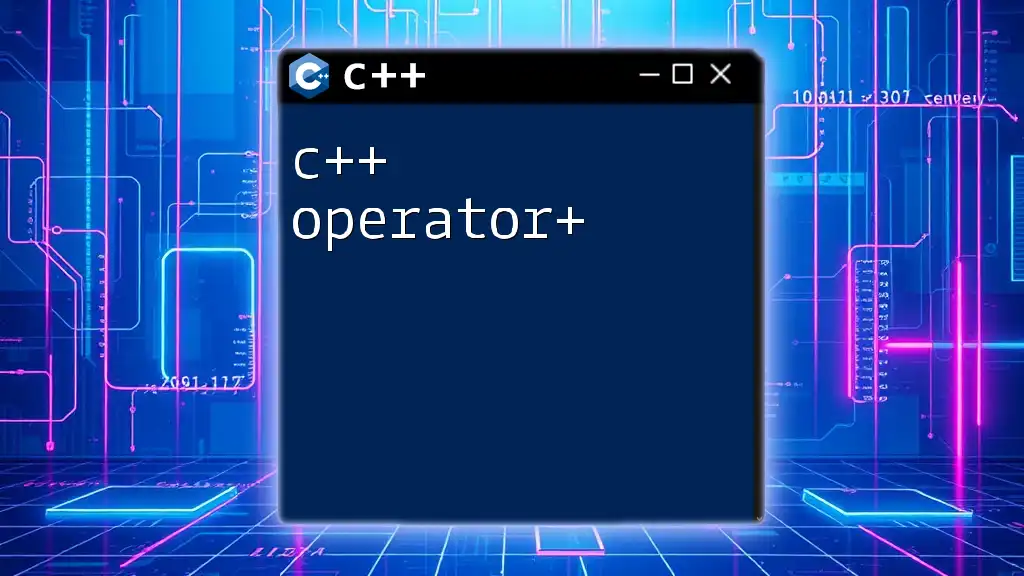
Conclusion
The C++ auto iterator is a powerful feature that combines the strengths of automatic type deduction with the flexibility of iterators. By understanding how to use it effectively, you can create more readable, maintainable, and efficient C++ code. The use of `auto` not only enhances your programming experience but also encourages a deeper familiarity with the containers and algorithms within the C++ standard library. I encourage you to experiment with auto iterators in your projects, as they can save you time and potential headaches down the road.
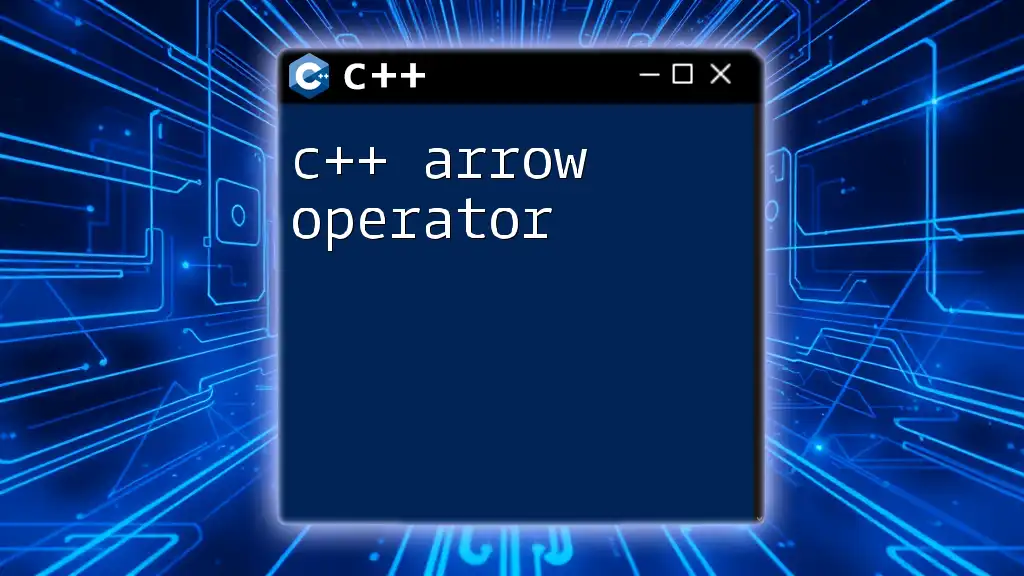
Additional Resources
Further Reading on C++ Iterators
For those looking to deepen their understanding of C++ iterators, consider exploring recommended books, online courses, and official documentation. Community forums also provide valuable insights and discussions that can help advance your knowledge.
Practice Problems
Engage with the community by tackling practical problems that involve implementing auto iterators in various scenarios. This proactive approach allows for learning through experience and peer feedback, further enhancing your proficiency in C++.