A C++ zip iterator allows you to iterate over multiple containers simultaneously, combining their elements into pairs for easy processing.
Here's a simple code snippet demonstrating a zip iterator using C++ ranges:
#include <iostream>
#include <vector>
#include <ranges>
template<typename... Containers>
class zip_iterator {
public:
// Implementation details for the zip iterator
// This is just a placeholder for illustration
};
int main() {
std::vector<int> vec1 {1, 2, 3};
std::vector<char> vec2 {'a', 'b', 'c'};
// Example usage of zip_iterator
for (auto [num, ch] : zip_iterator(vec1, vec2)) {
std::cout << num << ", " << ch << std::endl;
}
return 0;
}
(Note: The actual implementation of `zip_iterator` is not shown here as it would require significant coding, but this serves as a conceptual placeholder.)
What is a Zip Iterator?
A zip iterator in C++ is a powerful iteration tool that allows developers to traverse multiple containers in parallel. This abstraction helps in scenarios where you need to combine elements from two or more data structures, enabling a concise and readable approach to such operations. The core idea behind it is to "zip" together elements from different sequences, allowing for simultaneous access to each container's items.
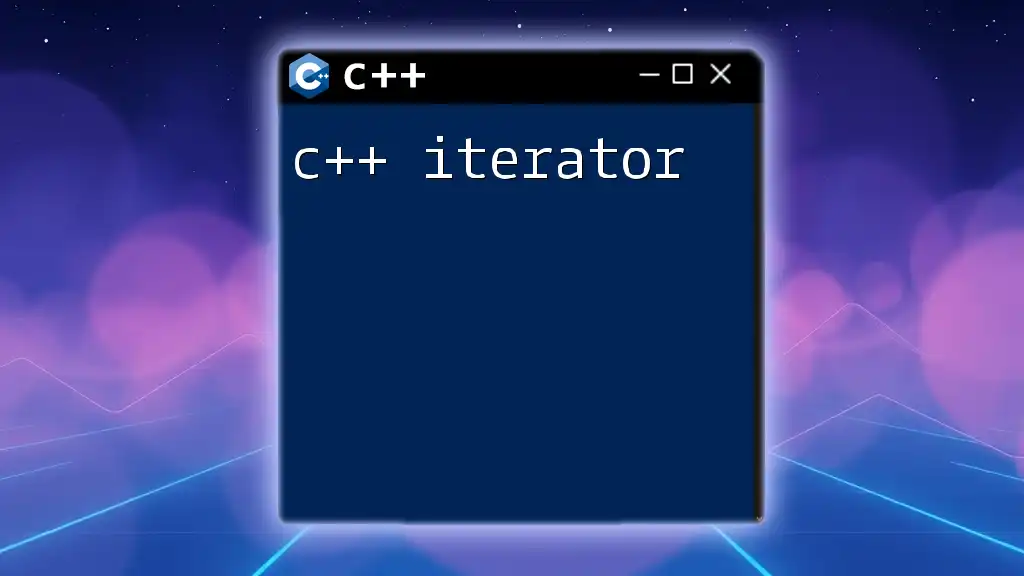
Why Use Zip Iterators?
Zip iterators provide several advantages compared to traditional iteration techniques:
- Simplified Syntax: They reduce the boilerplate code needed for accessing multiple containers, which leads to cleaner and more maintainable code.
- Increased Readability: When combined with appropriate algorithms, zip iterators make your intentions clearer, enhancing the readability of your code.
- Proliferation in Functional Programming: With the rise of functional programming paradigms in C++, zip iterators fit naturally into this style, often used in conjunction with lambda functions and STL algorithms.
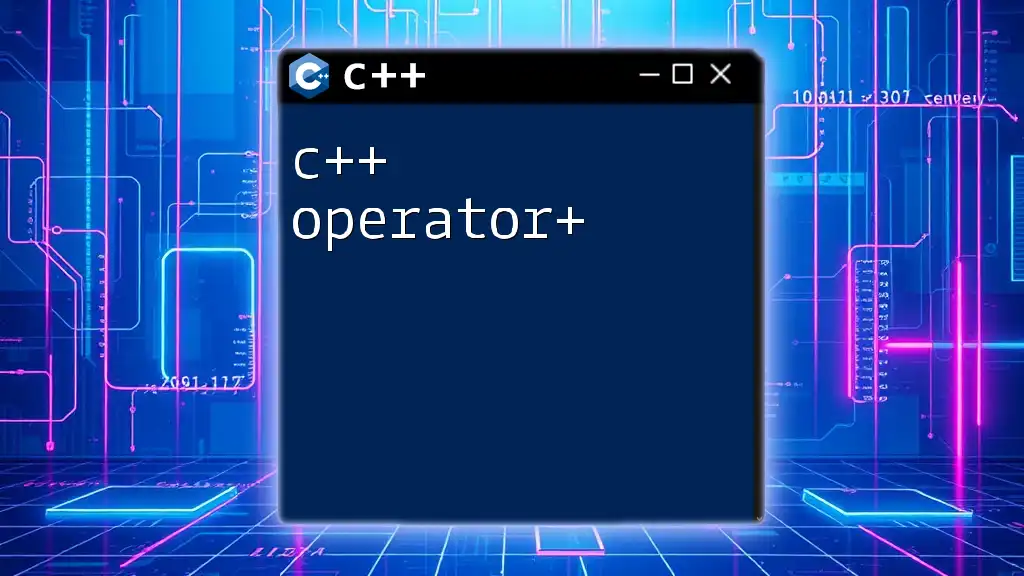
Understanding the Basics of Iterators
What are Iterators in C++?
In C++, an iterator is an object that enables a programmer to traverse a container, like a vector or list, without exposing the underlying structure. They serve multiple important roles:
- Generalization of Pointers: Iterators act like pointers, directing to elements within algorithms.
- Abstraction: They provide a unified interface to access different data types seamlessly.
There are various types of iterators in C++, including input, output, forward, bidirectional, and random access. Each has its specific use cases and operational characteristics.
How Zip Iterators Fit In
Zip iterators extend the concept of traditional iterators by allowing simultaneous traversal of multiple sequences. Unlike standard iterators that focus on a single container, zip iterators leverage a tuple to hold iterators from several containers, iterating through them together. This enables developers to easily compare, combine, or process data across collections in a tight and efficient manner.
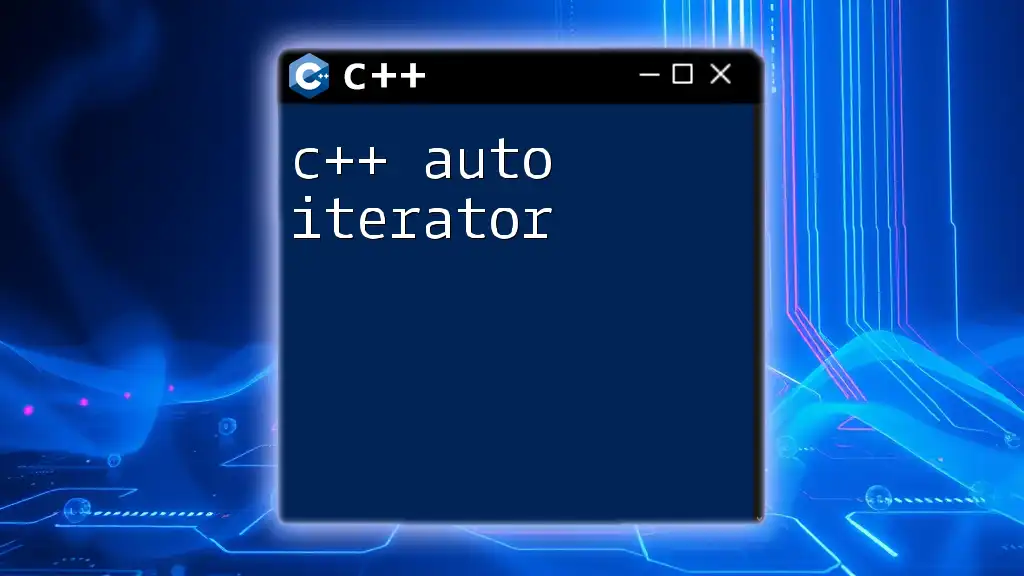
The Mechanics of Creating a Zip Iterator
Building Your Own Zip Iterator
Creating a zip iterator involves designing a class that accepts multiple iterators and allows combined access to their elements.
Essential Components
- Constructor: Initializes the iterators.
- Operator Overloads: Overload essential operators, such as `++` for incrementing the iterator and `*` for dereferencing it.
Code Example: Basic Zip Iterator Implementation
Here is a basic implementation of a zip iterator:
#include <tuple>
#include <iterator>
template<typename... Iterators>
class zip_iterator {
std::tuple<Iterators...> iterators;
public:
zip_iterator(Iterators... its) : iterators(its...) {}
zip_iterator& operator++() {
std::apply([](auto&... it) { ((++it), ...); }, iterators);
return *this;
}
auto operator*() {
return std::apply([](auto&... it) { return std::make_tuple(*it...); }, iterators);
}
bool operator!=(const zip_iterator& other) const {
return iterators != other.iterators;
}
};
Understanding Variadic Templates
Variadic templates in C++ allow for the creation of functions or classes that cater to a varying number of arguments, making them perfect for our zip iterator. This feature enables the zip iterator to handle any number of containers seamlessly, providing immense flexibility when combining diverse data structures.
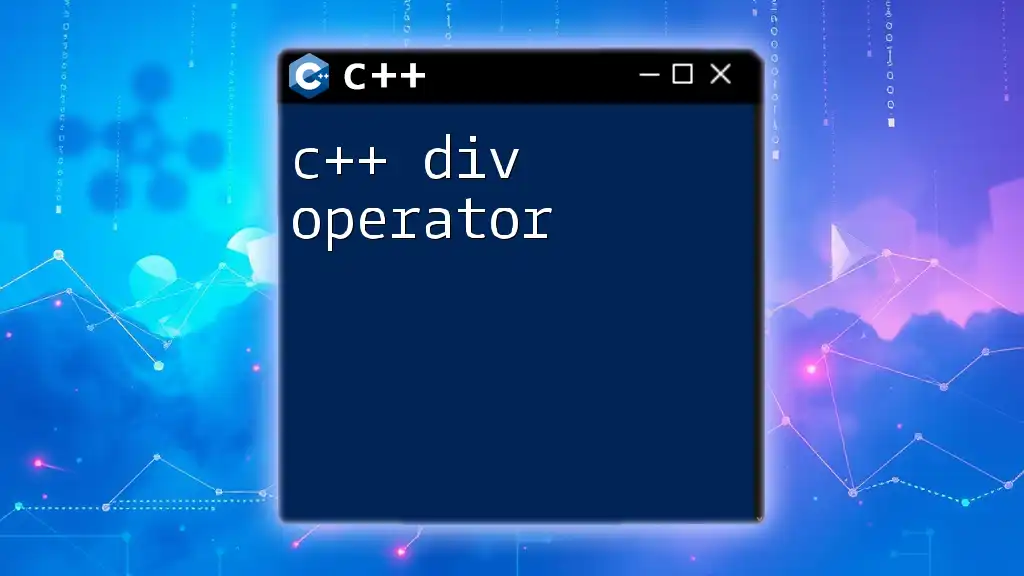
How to Use a Zip Iterator in Practice
Basic Usage Example
Using a zip iterator to traverse two vectors can simplify code when wanting to interrelate elements.
#include <iostream>
#include <vector>
template <typename T1, typename T2>
void zip_and_print(const std::vector<T1>& v1, const std::vector<T2>& v2) {
auto begin = zip_iterator(v1.begin(), v2.begin());
auto end = zip_iterator(v1.end(), v2.end());
for (auto it = begin; it != end; ++it) {
auto [a, b] = *it; // Tuple unpacking
std::cout << a << ", " << b << std::endl;
}
}
In this example, zip_and_print demonstrates how elements from two containers can be accessed and printed in tandem. The use of tuple unpacking is especially noteworthy here, as it keeps the code concise and clear.
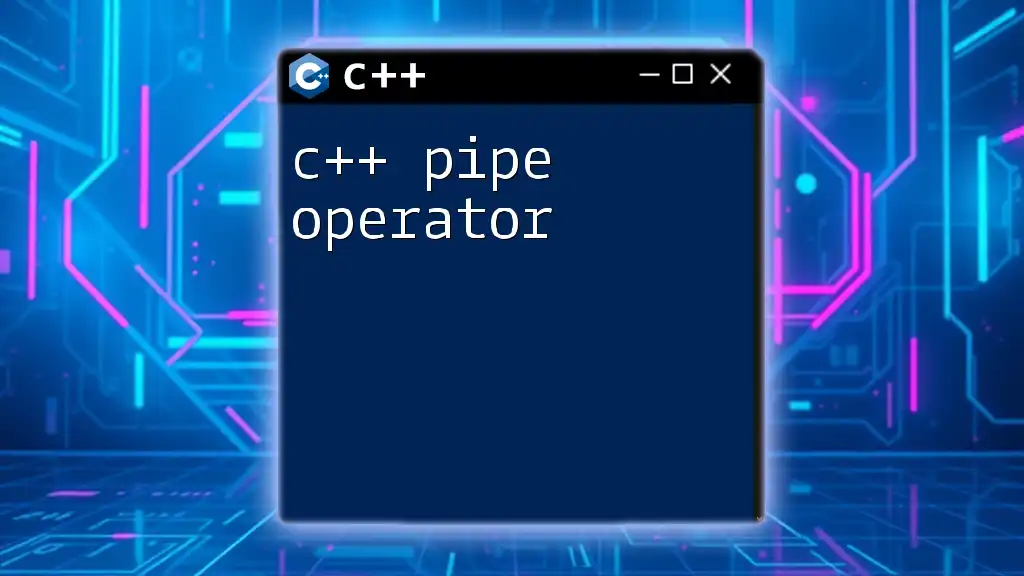
Advanced Topics in Zip Iterators
Customizing Zip Iterators for Different Containers
When designing zip iterators, it’s essential to consider the types and sizes of the containers involved. Zip iterators can be robust by implementing logic to handle cases where the containers have unequal lengths, preventing out-of-bounds errors.
Performance Considerations
Performance is always an integral aspect of software development. Zip iterators can provide efficiency improvements by minimizing the complexity of nested loops commonly used in multi-container iteration. Benchmarking performance vs. traditional methods shows that for certain operations, especially when combined with algorithms, zip iterators can significantly reduce execution time in C++ applications.
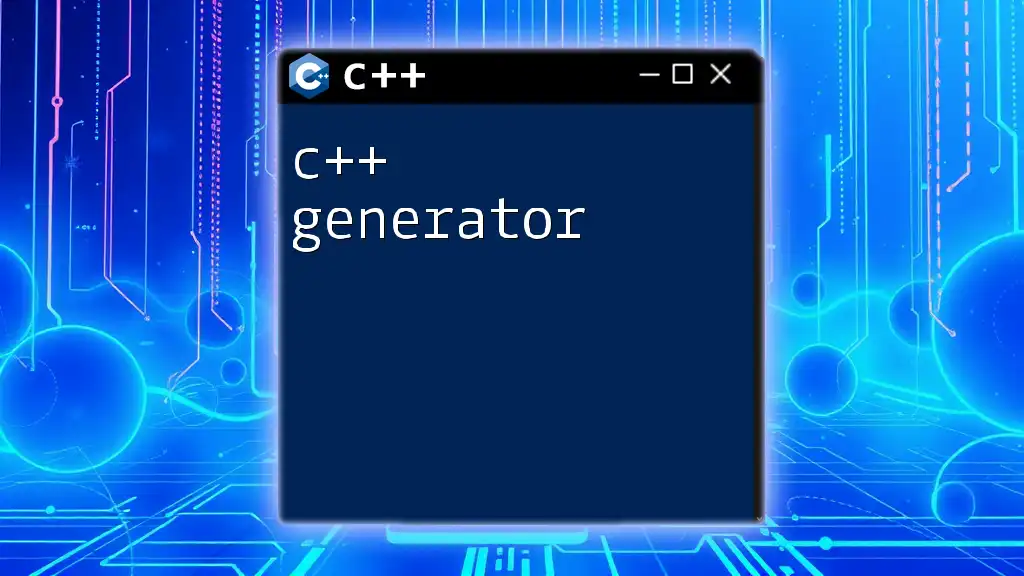
Best Practices and Common Pitfalls
Best Coding Practices
When implementing zip iterators, developers should adhere to the following best practices:
- Const Correctness: Make sure that const iterators are respected to avoid accidental modifications.
- Iterators Invalidation: Pay special attention to the life cycle of the iterators used to prevent access violations.
Common Mistakes to Avoid
- Mixing Container Types: Ensure that containers passed to the zip iterator are compatible in terms of size and type. This is crucial when processing data.
- Lifetime and Memory Management: Be cautious about the lifetimes of iterators within complex structures to avoid dangling references.
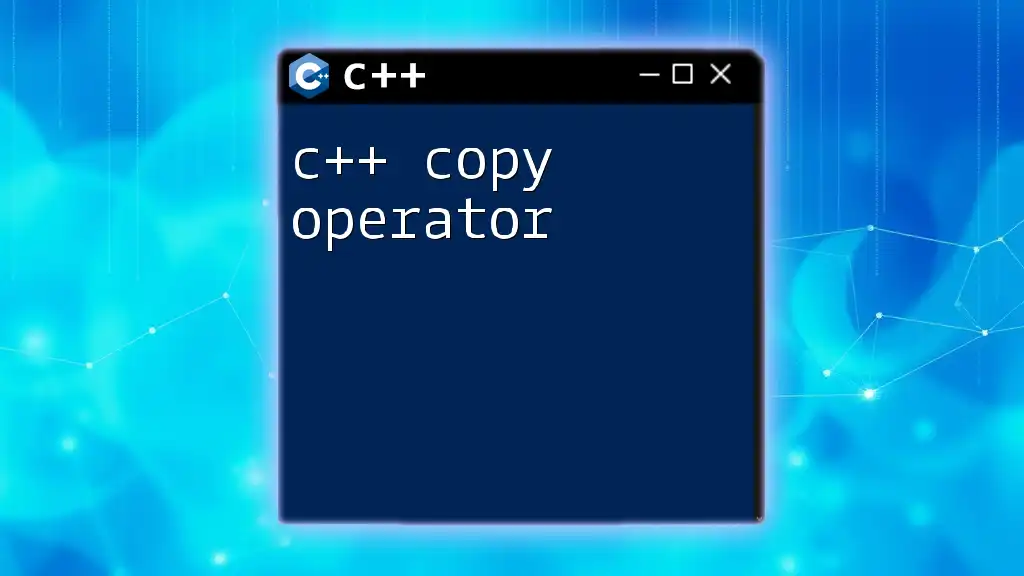
Real-World Applications of Zip Iterators
Use Cases in Data Processing
In real-world applications, zip iterators are particularly useful in data processing situations where numerous attributes need to be correlated. For example, they can be used to quickly compare values from multiple datasets in a statistical application.
Integration with Standard Algorithms
Zip iterators integrate seamlessly with STL algorithms, allowing complex operations to be applied with minimal fuss. For instance, combining `std::transform` with zip iterators can efficiently apply a function across corresponding elements from multiple containers.
Code Example: Using Zip Iterator with STL Algorithms
#include <algorithm>
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> result;
std::transform(zip_iterator(vec1.begin(), vec2.begin()),
zip_iterator(vec1.end(), vec2.end()),
std::back_inserter(result),
[](const auto& elems) {
return std::get<0>(elems) + std::get<1>(elems);
});
This example illustrates how zip iterators can be useful in crafting succinct and expressive data transformations.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/images/posts/c/cpp-operator.webp)
Conclusion
Recap of Key Points: Zip iterators enhance the ability to manage and traverse multiple containers in C++, streamlining your code and improving its clarity. The comprehensive capabilities of zip iterators are advantageous for a variety of applications, from data processing to integrating with STL algorithms.
Further Reading and Resources
For those interested in deepening their understanding of iterators and zip iterators in particular, consider exploring the C++ STL documentation, official C++ programming guidelines, or specialized programming books focusing on advanced C++ techniques.
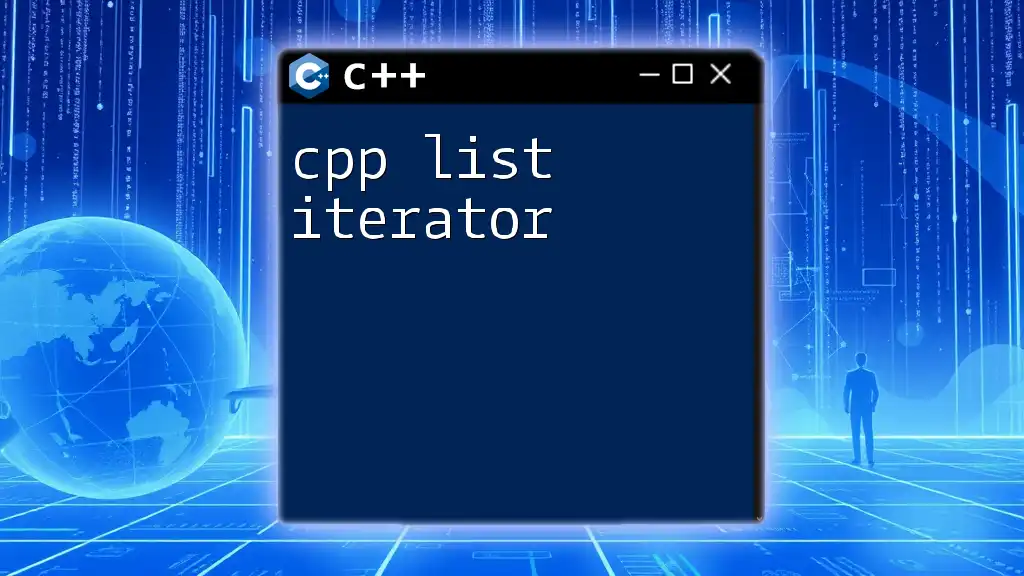
Call to Action
As you begin utilizing C++ zip iterators, measure their impact on your coding practices. Experiment with different types of containers and integrations within your projects. Join our community for ongoing tutorials, tips, and shared experiences as you refine your skills in C++.