C++ bit operations allow you to manipulate individual bits within an integer, enabling efficient low-level programming and optimization.
Here's a code snippet illustrating some basic bit operations in C++:
#include <iostream>
int main() {
unsigned int a = 5; // 0101 in binary
unsigned int b = 3; // 0011 in binary
// Bitwise AND
unsigned int and_result = a & b; // 0001 (1 in decimal)
// Bitwise OR
unsigned int or_result = a | b; // 0111 (7 in decimal)
// Bitwise XOR
unsigned int xor_result = a ^ b; // 0110 (6 in decimal)
// Bitwise NOT
unsigned int not_result = ~a; // 1010...1010 (depends on the bit length)
std::cout << "AND: " << and_result << "\n"
<< "OR: " << or_result << "\n"
<< "XOR: " << xor_result << "\n"
<< "NOT: " << not_result << std::endl;
return 0;
}
Understanding C++ Bitwise Operators
C++ bit operations utilize a special set of operators that manipulate data at the bit level, directly affecting the binary representation of numbers.
What are C++ Bitwise Operators?
C++ provides several bitwise operators that perform various operations on bits and can significantly enhance the performance of certain algorithms. The primary bitwise operators are:
- Bitwise AND (`&`)
- Bitwise OR (`|`)
- Bitwise NOT (`~`)
- Bitwise XOR (`^`)
- Left Shift (`<<`)
- Right Shift (`>>`)
Bitwise AND Operator
The Bitwise AND operator compares each bit of two integers and returns a new integer formed by setting each bit to `1` if both corresponding bits are `1`. Otherwise, it sets the bit to `0`.
Syntax:
int result = a & b;
Example:
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a & b; // Result: 0001 (Decimal: 1)
Explanation: In this example, the result `1` corresponds to the binary representation where only the rightmost bits of both `a` and `b` are `1`.
Bitwise OR Operator
The Bitwise OR operator sets each bit to `1` if at least one of the corresponding bits of the operands is `1`.
Syntax:
int result = a | b;
Example:
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a | b; // Result: 0111 (Decimal: 7)
Explanation: The resulting binary `0111` indicates that any position where either `a` or `b` has a `1` contributes a `1` to the outcome.
Bitwise NOT Operator
The Bitwise NOT operator inverts the bits of its operand, turning `0`s into `1`s and vice versa.
Syntax:
int result = ~a;
Example:
int a = 5; // Binary: 0101
int result = ~a; // Result: 1010 (Decimal: -6 as a signed integer)
Explanation: The result `1010` is the two's complement representation of `-6` in a typical 4-bit environment.
Bitwise XOR Operator (Exclusive OR)
The Bitwise XOR operator compares corresponding bits of two operands, setting each bit to `1` if the bits are different.
Syntax:
int result = a ^ b;
Example:
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a ^ b; // Result: 0110 (Decimal: 6)
Explanation: In this case, `result` has `1`s in positions where `a` and `b` differ.
Left Shift Operator
The Left Shift operator shifts the bits of the left operand to the left by the number of positions specified by the right operand. Each shift to the left essentially multiplies the original number by `2`.
Syntax:
int result = a << n; // Shifts `a` left by `n` bits
Example:
int a = 4; // Binary: 0100
int result = a << 1; // Result: 1000 (Decimal: 8)
Explanation: Shifting `4` one position to the left results in `8` (double the original value).
Right Shift Operator
The Right Shift operator shifts the bits of the left operand to the right. Each right shift divides the original number by `2`.
Syntax:
int result = a >> n; // Shifts `a` right by `n` bits
Example:
int a = 8; // Binary: 1000
int result = a >> 1; // Result: 0100 (Decimal: 4)
Explanation: Shifting `8` one position to the right gives `4`.
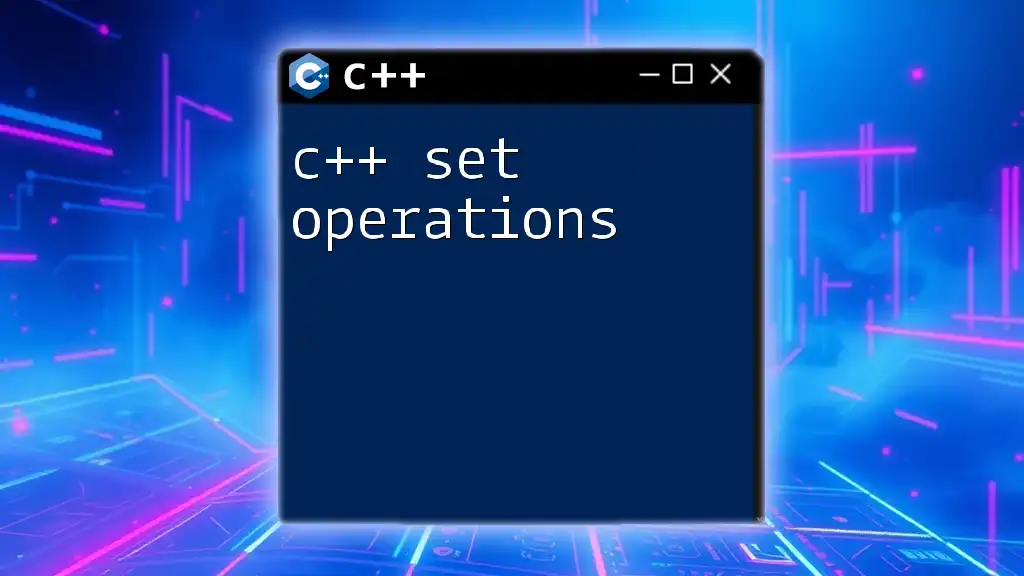
Practical Applications of C++ Bitwise Operations
Optimization and Performance Enhancement
C++ bit operations are often significantly faster than their arithmetic counterparts due to their direct manipulation of binary data. They can be used to optimize performance-sensitive applications, such as embedded systems and game engines. For instance, using bitwise operations for arithmetic can reduce the time complexity of algorithms.
Manipulating Flags using Bitwise AND and OR
Bitwise operations are particularly useful for manipulating flags in settings or configurations. This technique allows programmers to toggle certain options without needing separate boolean variables.
Example: Using Bitwise Operations for Setting Flags
int flags = 0; // 0000
flags |= (1 << 0); // Set first flag: 0001
flags |= (1 << 2); // Set third flag: 0101
Example: Using Bitwise Operations for Clearing Flags
flags &= ~(1 << 0); // Clear first flag: 0100
Efficiently Representing Data through Bit Manipulation
Bitwise operations enable efficient data representation, especially when memory usage is critical. For example, you can pack several boolean values into a single byte, with each bit representing a different value.
Example: Packing Data into a Single Integer
unsigned char packedData = 0;
packedData |= (1 << 0); // true
packedData |= (1 << 2); // true
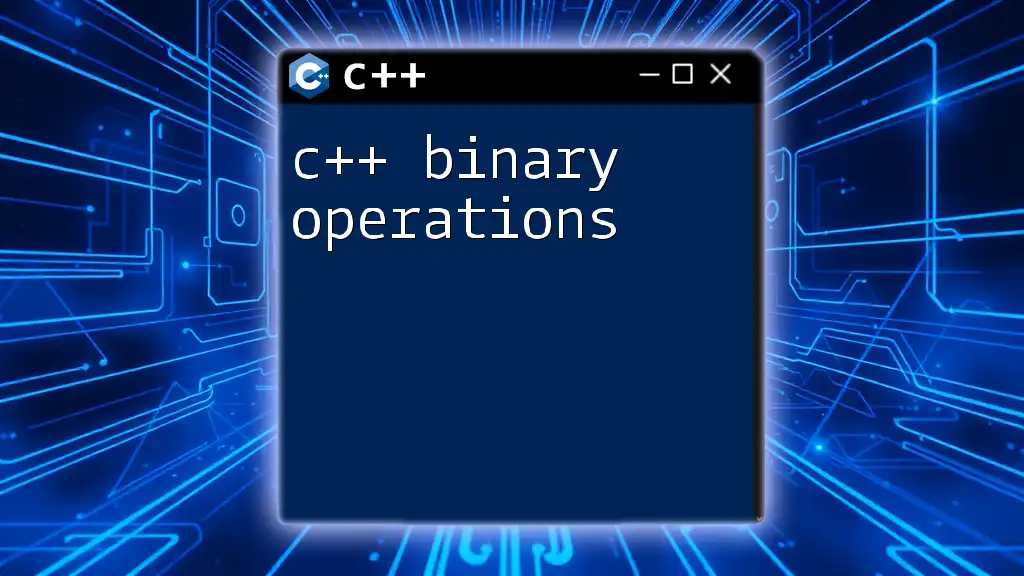
Combining Bitwise Operations
Chaining Bitwise Operations
You can combine multiple bitwise operations in a single expression, taking advantage of operator precedence in C++. An expression such as `a & b | c` first evaluates the AND operation, then the OR operation.
Example: A Complex Bitwise Operation
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int c = 2; // Binary: 0010
int result = (a & b) | c; // Result: 0011 (Decimal: 3)
Real-World Scenarios for Combining Operations
Combining operations is commonly found in low-level programming scenarios, like device drivers or network packet analysis where you must quickly manipulate bits for performance reasons.
Example: Implementing a Simple Encryption Algorithm using Bitwise Operations
char encryptChar(char input) {
return input ^ 0xFF; // Simple XOR-based encryption
}
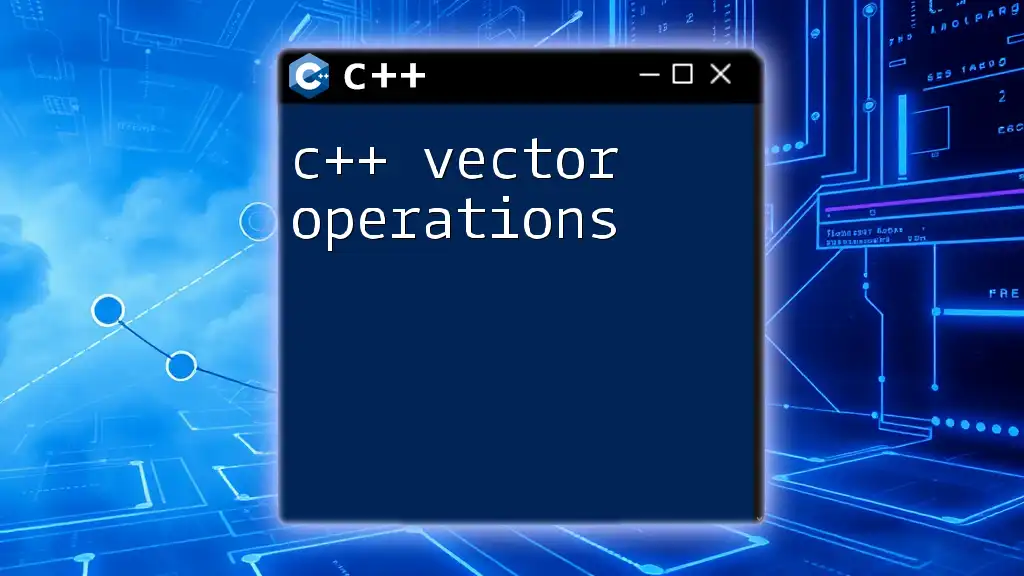
Bitwise Operations with Different Data Types
Applying Bitwise Operations on Integers
Most commonly, bitwise operations are applied to integers. Positive and negative integers yield different results, especially with NOT and shift operations.
Using Bitwise Operations on Characters
Bitwise operations can also be effectively applied to characters since they are stored as integers in C++ (using ASCII).
Example:
char ch = 'A'; // ASCII: 65
char result = ch | 0x20; // Lowercase: 97 ('a')
Working with Unsigned Integers
Using unsigned integers with bitwise operations can avoid negative numbers, which is often beneficial for clear operations on binary values.
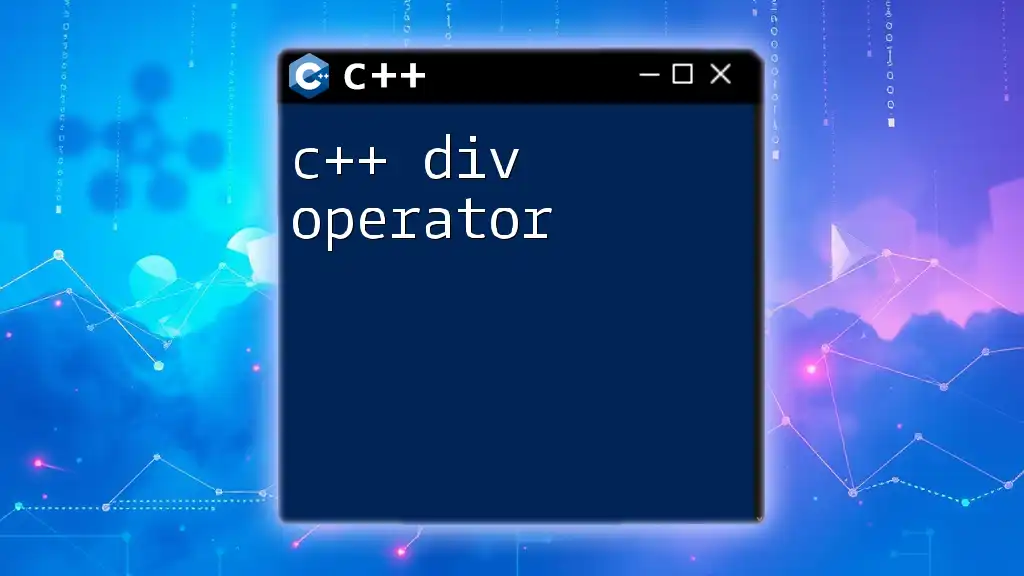
Debugging and Common Pitfalls in Bitwise Operations
Common Errors when Using Bitwise Operators
New programmers may misunderstand the operator precedence, leading to unexpected results. Confusion over signed versus unsigned integers also frequently causes issues in calculations.
Tips for Troubleshooting Bitwise Operations
To clarify behavior, always print intermediate results to understand how each operation affects the bits. Consistent formatting of your expressions can help track down logical errors.
Example of Common Mistakes
int a = 5; // 0101
int result = (a & 3) | 8; // Mistaken precedence can confuse the outcome.
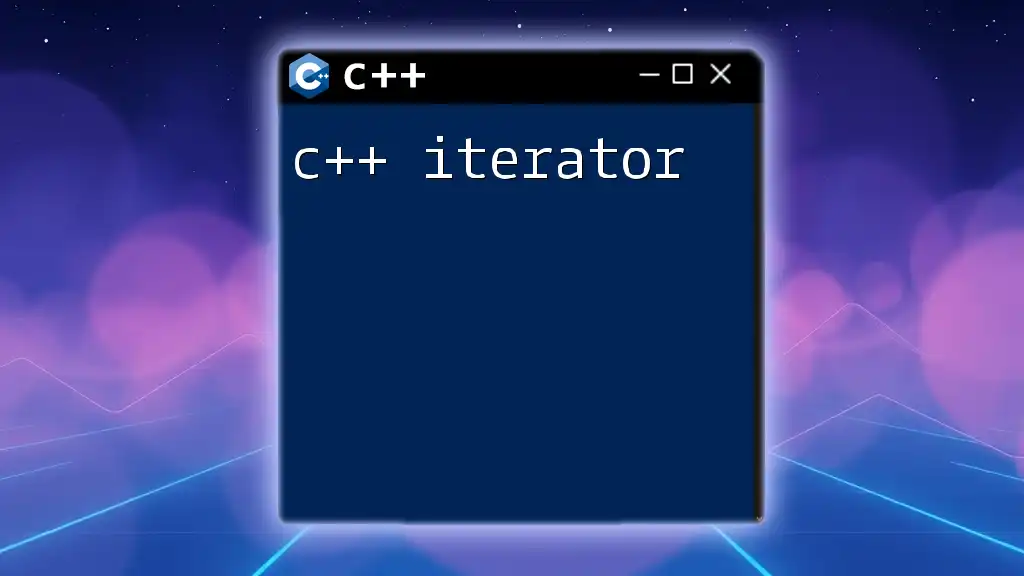
Conclusion
In summary, C++ bit operations are a powerful tool for programmers, offering speed and efficiency for managing binary data. Understanding and mastering these operators can greatly enhance your programming skills and allow for more optimized code in various applications.
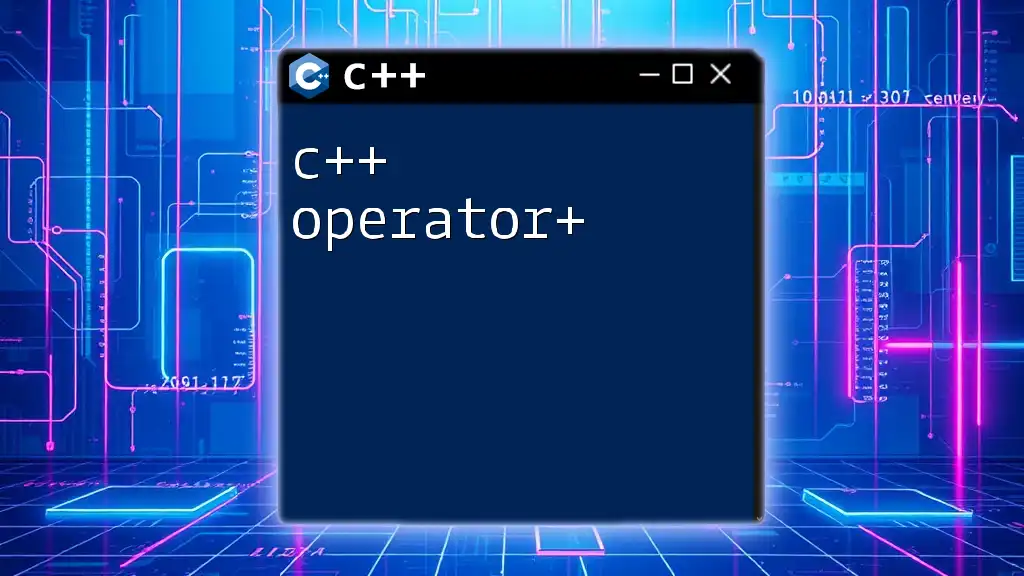
Additional Resources
For those wishing to delve deeper into C++ and bitwise operations, many books and online courses are available that can enhance your learning experience. Engaging with community forums can also provide valuable tips and tricks from experienced programmers.
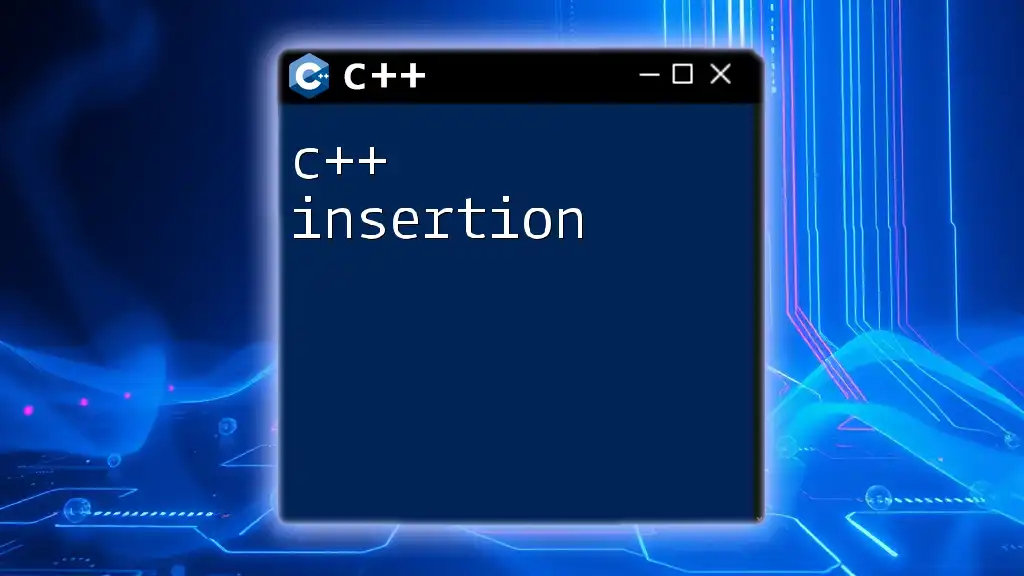
Call to Action
If you're drawn to learning more about C++, consider joining a dedicated course, and don't forget to download our useful cheat sheet for C++ bitwise operations for quick reference!