The C++ pipe operator (`|`) is primarily used in the context of bitwise operations, enabling you to perform bitwise OR operations between two integral types.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a | b; // Bitwise OR: 0111 in binary (7 in decimal)
std::cout << "The result of " << a << " | " << b << " is " << result << std::endl; // Output: 7
return 0;
}
What is the Pipe Operator in C++?
The C++ pipe operator, represented as `|`, serves multiple roles within the language, primarily functioning as a bitwise operator but also as a tool for stream manipulations. Understanding the versatility of this operator is crucial for both beginners and experienced developers looking to harness the full power of C++ in their programs.

The Bitwise OR Operator
Understanding the Basics
In C++, the pipe operator acts as a bitwise OR. This operator compares each bit of two integers and returns a new integer with bits set to `1` whenever at least one of the corresponding bits of either operand is `1`.
Bitwise OR Logic is grounded in binary representation. To illustrate, consider the following truth table:
a | b | a | b |
---|---|---|---|
0 | 0 | 0 | |
0 | 1 | 1 | |
1 | 0 | 1 | |
1 | 1 | 1 |
Code Example: Bitwise OR
#include <iostream>
using namespace std;
int main() {
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int result = a | b; // result will be 7 (0111 in binary)
cout << result << endl; // Output: 7
return 0;
}
In this code snippet, we initialize two integers, `a` and `b`. When we apply the pipe operator, we obtain the result `7`, which corresponds to binary `0111`, showcasing how the bitwise OR combines the binary representations of both integers.

Usage of Pipe Operator in Streams
Introduction to Streams
C++ uses I/O streams to handle input and output operations. These streams facilitate data flow between the program and external resources like consoles or files. The pipe operator's role in stream manipulation contributes to writing clean, efficient code.
The `|` Operator with Streams
The pipe operator can be used to concatenate multiple data streams, enhancing clarity and simplifying code. Instead of cluttering code with multiple output operations, combining outputs using the pipe operator yields both concise and readable syntax.
Code Example: Stream Manipulation
#include <iostream>
#include <sstream>
using namespace std;
int main() {
stringstream ss;
ss << "Hello, " << "world!" << endl; // Using pipe operator for streaming
cout << ss.str(); // Output: Hello, world!
return 0;
}
In this example, we use `stringstream` for assembling a string. By employing the pipe operator, we elegantly combine multiple strings into one visible output, rendering our code clearer and more maintainable.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/_next/image?url=%2Fimages%2Fposts%2Fc%2Fcpp-operator.webp&w=1080&q=75)
Custom Pipe Operator Overloading
What is Operator Overloading?
Operator overloading allows developers to redefine the behavior of standard operators when applied to user-defined types, enhancing their functionality and ease of use. It simplifies complex operations and aligns with the needs of specific classes.
Creating a Custom Pipe Operator
Overloading the pipe operator follows a straightforward syntax. To utilize a custom class, we define the operator function which specifies how the operator interacts with objects of that class.
Code Example: Custom Class
#include <iostream>
using namespace std;
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
MyClass operator|(const MyClass& other) {
return MyClass(this->value | other.value); // Bitwise OR implementation
}
};
int main() {
MyClass a(5); // 0101
MyClass b(3); // 0011
MyClass result = a | b; // result.value will be 7
cout << result.value << endl; // Output: 7
return 0;
}
In this code, we define a class `MyClass` that stores an integer value. By overloading the pipe operator, we permit objects of `MyClass` to interact using the bitwise OR operation, ultimately allowing for concise expressions that showcase how this operator can enrich user-defined types.
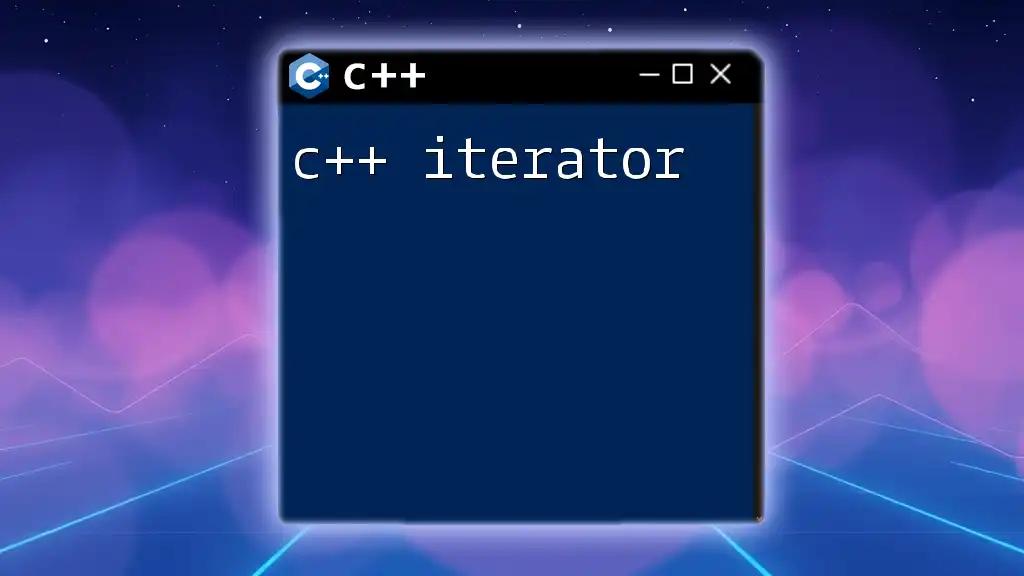
Advantages of Using the Pipe Operator
Readability and Clarity
The primary advantage of using the pipe operator in C++ is its ability to enhance the readability of code. By utilizing this operator, developers can write more expressive and cleaner code compared to traditional methods. Streamlined operations foster a clearer understanding of the logic and flow of the code.
Simplifying Complex Operations
Instead of constructing verbose code for multiple I/O operations or complex calculations, the pipe operator allows for a compact syntax. Consider the following traditional output approach versus one utilizing the pipe operator:
Traditional method:
cout << "The result is: ";
cout << result << endl;
With pipe operator:
cout << "The result is: " << result << endl;
The latter is not only shorter but also emphasizes the relation between the output and the displayed result, illustrating the C++ pipe operator's efficacy in simplifying the thought process of code creation.
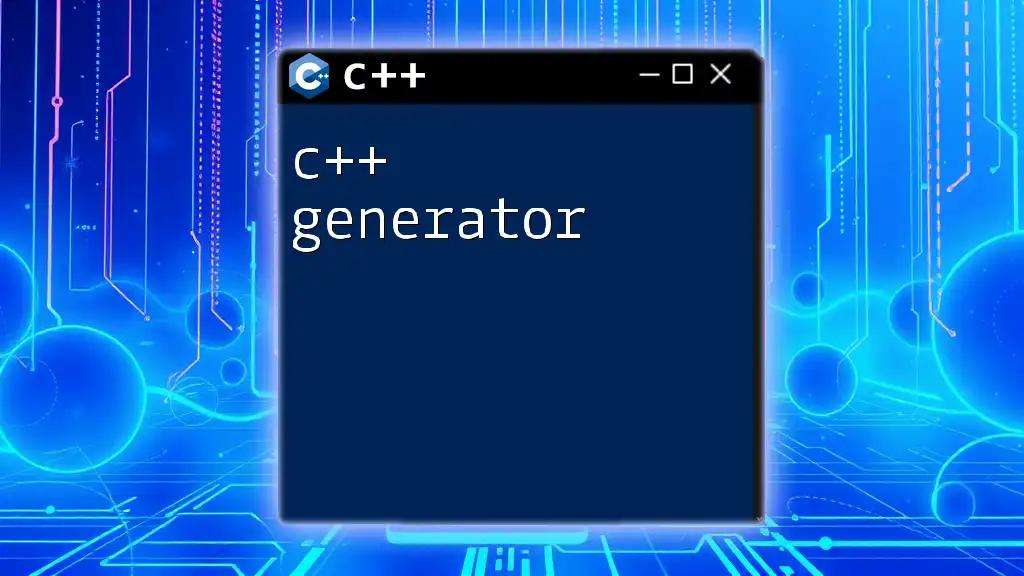
Common Mistakes and Pitfalls
Misunderstanding Bitwise vs. Logical Operators
A frequent error among beginners is confusing the pipe operator (`|`) with the logical OR operator (`||`). While the former operates on individual bits, the latter evaluates boolean expressions. It's essential to distinguish these operators to avoid logical errors in your programs.
Errors in Overloading
When overloading the pipe operator, developers may encounter issues such as:
- Returning non-object types: Ensure your function returns a valid object of the class type.
- Const issues: Be mindful of constant references and whether your operation modifies the current object.
To troubleshoot, always perform a thorough code review and leverage debugging tools to pinpoint the source of errors.
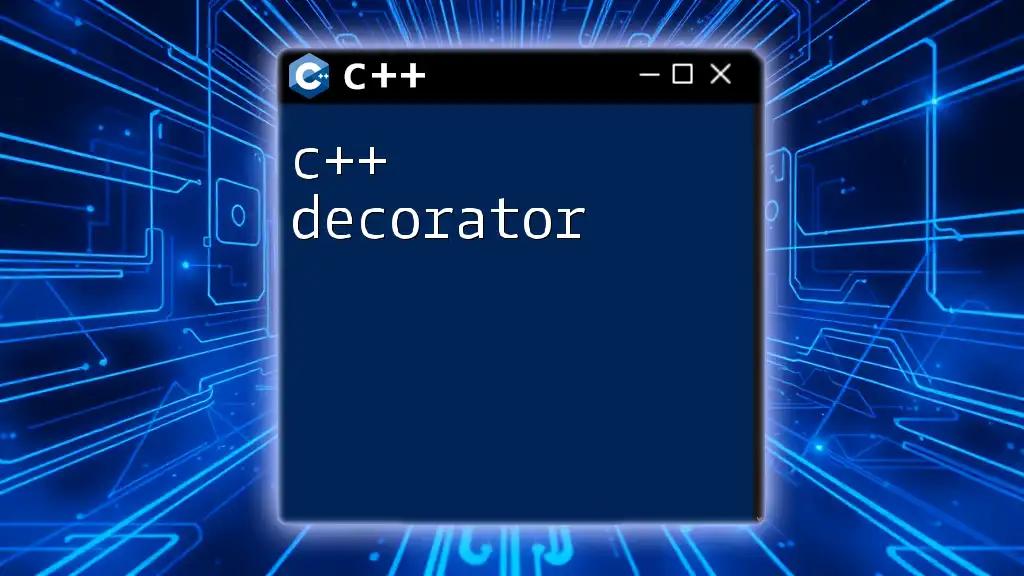
Conclusion
The C++ pipe operator is a powerful tool in the programming arsenal that simplifies and clarifies operations. Whether you're engaging in bitwise operations or stream manipulations, the pipe operator enhances readability and enables concise code. Don’t hesitate to explore the functionality of this operator in your code, and appreciate the efficiency it brings to your programming practices.
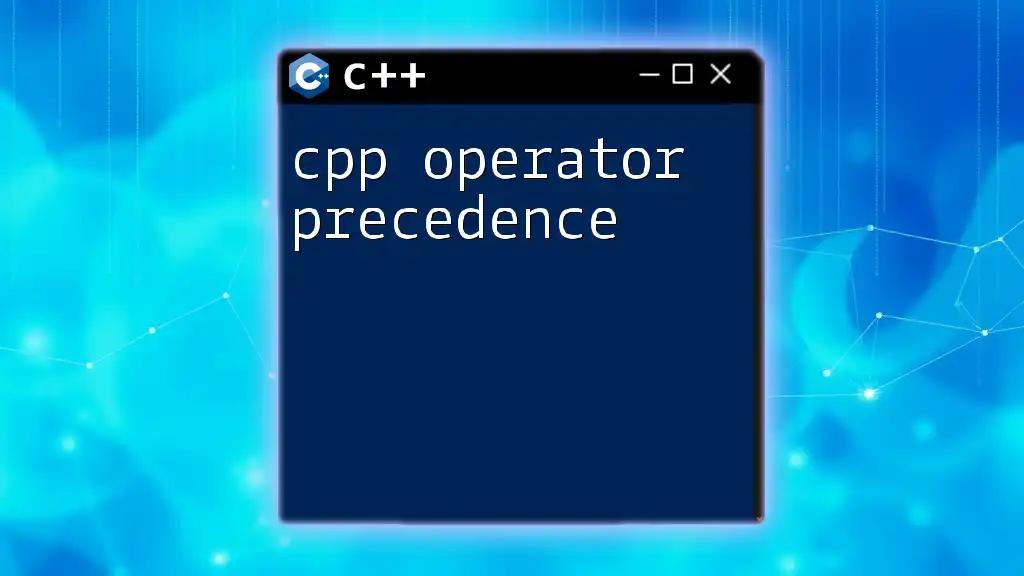
Call to Action
Now that you have a grasp of the C++ pipe operator, practice implementing it in your projects. Try out the examples provided or modify them to experiment with new functionalities. Deepening your understanding of operators is a crucial step toward mastering C++.

Additional Resources
For further exploration, consider delving into reputable C++ documentation and resources, as well as engaging with online courses that offer comprehensive learning experiences regarding C++ operators and coding best practices.