C++ high performance refers to the language's capability to deliver fast execution speeds and efficient memory usage, making it ideal for system-level programming and resource-intensive applications.
Here’s a simple example demonstrating efficient memory management with dynamic arrays in C++:
#include <iostream>
int main() {
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
// Dynamic memory allocation
int* dynamicArray = new int[size];
for (int i = 0; i < size; ++i) {
dynamicArray[i] = i * 2; // Example operation
}
// Displaying the array
for (int i = 0; i < size; ++i) {
std::cout << dynamicArray[i] << " ";
}
std::cout << std::endl;
// Freeing allocated memory
delete[] dynamicArray;
return 0;
}
Understanding Performance in C++
What is Performance in Programming?
Performance in programming typically refers to how efficiently a program runs. High performance is characterized by several metrics, with speed, memory usage, and resource utilization being the most critical. In C++, achieving high performance means executing tasks quickly and efficiently while minimizing the use of memory and other system resources.
Why Choose C++ for High-Performance Applications?
C++ is renowned for its ability to deliver high performance due to its close-to-hardware nature, offering developers control over system resources. Here are a few reasons why you might choose C++:
- Efficiency: C++ compiles to machine code, allowing for optimized performance.
- Direct Memory Access: Low-level memory manipulation enables performance optimizations that higher-level languages may not support.
- Fine-Grained Control: C++ allows control over data structures, storage, and processes, enabling more informed performance decisions.
C++ is particularly effective in domains where performance is crucial, such as gaming, financial systems, and scientific computing, where split-second decisions can significantly impact outcomes.

Key Concepts for High-Performance C++ Programming
Memory Management
C++ offers mechanisms for manual memory management, which can lead to optimized performance if used correctly. Understanding pointers and references is crucial as they facilitate direct memory access and manipulation, a significant advantage in high-performance applications.
Consider the following examples illustrating different memory allocation techniques:
// Dynamic allocation using new
int* arr_dynamic = new int[100];
// Stack allocation
int arr_stack[100];
Using stack allocation, where feasible, is often faster and incurs less overhead than dynamic allocation, as the compiler can optimize stack use better.
Data Structures and Algorithms
Choosing the right data structure can dramatically affect performance. C++'s Standard Template Library (STL) provides various containers like `vector`, `list`, and `map`, each with its performance characteristics:
- Vector: Fast random access but costly insertions and deletions.
- List: Efficient for insertions/deletions but slower on random access.
- Map: Ordered key-value storage but has overhead in terms of performance compared to other containers.
Here’s an example comparing the time taken to sort a vector versus a list:
#include <vector>
#include <list>
#include <algorithm>
#include <chrono>
void benchmark_sort() {
std::vector<int> vec(1000000), list_data(1000000);
// Fill vector and list with data
std::generate(vec.begin(), vec.end(), rand);
std::copy(vec.begin(), vec.end(), list_data.begin());
auto start = std::chrono::high_resolution_clock::now();
std::sort(vec.begin(), vec.end());
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Vector sort time: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << "ms\n";
start = std::chrono::high_resolution_clock::now();
list_data.sort();
end = std::chrono::high_resolution_clock::now();
std::cout << "List sort time: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << "ms\n";
}
This comparison illustrates how data structures affect performance based on the task.
Optimizing Loops and Iterations
Optimizing loops is essential for performance. Techniques like loop unrolling and cache optimization can yield significant speed-ups by reducing the overhead of loop control.
For example, unrolling a loop can reduce the number of iterations and, with the right compiler optimizations, yield better CPU performance:
for (int i = 0; i < 8; i += 2) {
// Original loop
sum += arr[i];
sum += arr[i + 1]; // Loop unrolling
}
This approach can minimize branch operations and improve cache locality.

Advanced Techniques for Performance Optimization
Compiler Optimizations
Compiler optimizations play a vital role in performance enhancements. Utilizing the correct compiler flags can make a significant difference in your code's execution speed. For instance, using `-O2` or `-O3` in GCC enables various optimizations that can speed up execution:
g++ -O2 your_program.cpp -o your_program
You may observe an impressive performance increase in numerically intensive workloads.
Memory Pooling and Object Recycling
Memory pooling is a technique to mitigate fragmentation and unnecessary allocations, improving performance by reusing memory chunks. Implementing a simple memory pool can look like this:
class MemoryPool {
public:
MemoryPool(size_t size) : pool(new char[size]), next(pool) {}
~MemoryPool() { delete[] pool; }
void* allocate(size_t size) {
if(next + size > pool + pool_size) return nullptr; // OOM
void* block = next;
next += size;
return block;
}
private:
char* pool;
char* next;
size_t pool_size;
};
This pattern reduces the overhead associated with frequent dynamic allocations.
Concurrency and Parallelism
C++11 introduced robust threading capabilities, empowering developers to leverage multi-threading to optimize performance. By distributing tasks across multiple threads, applications can achieve significant speed improvements.
Here’s a basic example using `std::async` for parallel computation:
#include <future>
#include <vector>
int parallel_sum(std::vector<int>& vec) {
return std::accumulate(vec.begin(), vec.end(), 0);
}
void example_parallel() {
std::vector<int> data(1000000, 1);
auto future = std::async(std::launch::async, parallel_sum, std::ref(data));
int result = future.get();
std::cout << "Sum: " << result << "\n";
}
Utilizing concurrency can maximize CPU usage and minimize runtime for large computations.
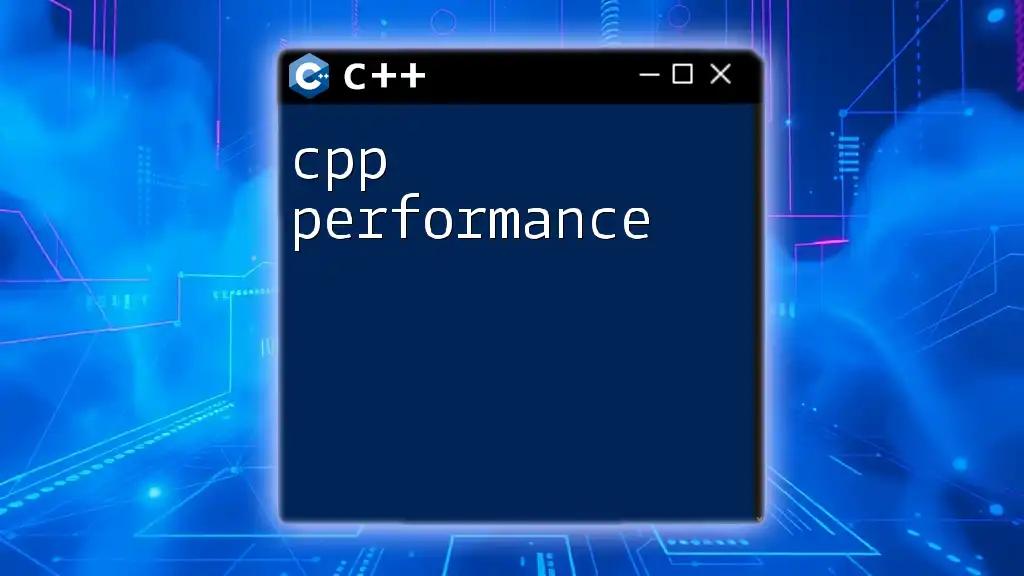
Profiling and Benchmarking C++ Code
Understanding Profiling in C++
Profiling is essential for identifying performance bottlenecks within your code. Tools like `gprof` and `Valgrind` help analyze which parts of your code consume the most time and resources, guiding optimization efforts effectively.
How to Benchmark Your Code
To achieve an accurate measurement of your code's performance, benchmarking is critical. Implementing robust testing methodologies, such as executing multiple iterations and averaging results, helps obtain reliable benchmarks while avoiding common pitfalls, like measuring only a single execution time.
Here is an example benchmark for a simple operation:
#include <chrono>
void benchmark() {
auto start = std::chrono::high_resolution_clock::now();
// Code to benchmark
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Duration: " << std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << "µs\n";
}
This method aids in tracking performance changes as optimizations are applied.
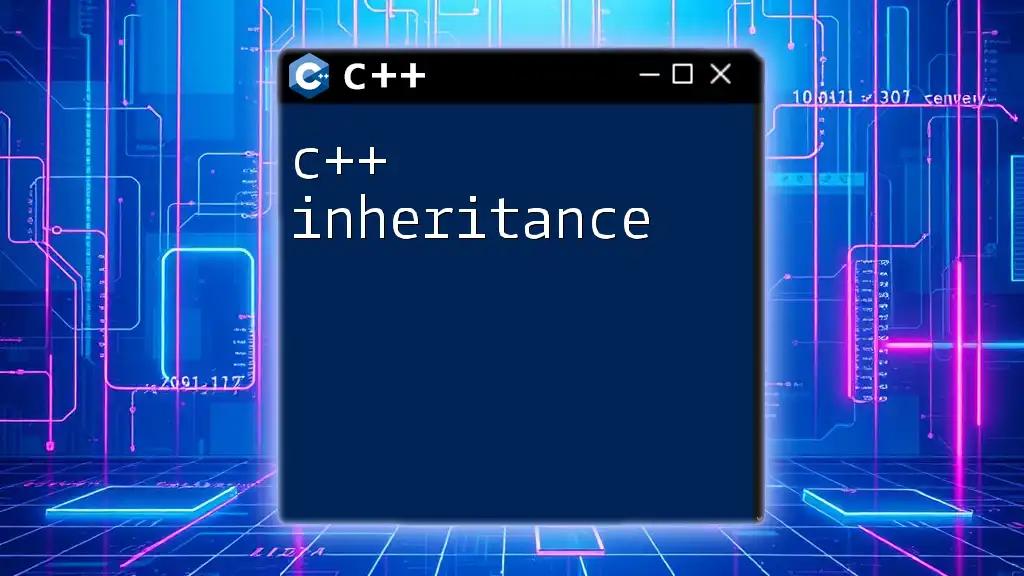
Performance Testing Best Practices
Writing Efficient Tests
To measure performance effectively, writing tests that consider various scenarios is crucial. Emphasize isolated tests that assess individual components to pinpoint inefficiencies without external influences.
Interpreting Results and Making Adjustments
Once profiling and benchmarking are complete, it is essential to analyze the results meticulously. Look for functions consuming excessive CPU time or using unnecessary memory. Iteratively refining your code in response to feedback from profiling data can lead to optimized performance.

Conclusion
High-performance C++ programming involves a comprehensive understanding of various concepts, from memory management to advanced optimization techniques. By implementing the best practices outlined in this guide and continuously profiling your code, you can ensure your applications are not only fast but also efficient, making C++ an unmatched choice for performance-critical applications.
Engage with the community; share your experiences, ask questions, and continue exploring the potential of C++ high performance!
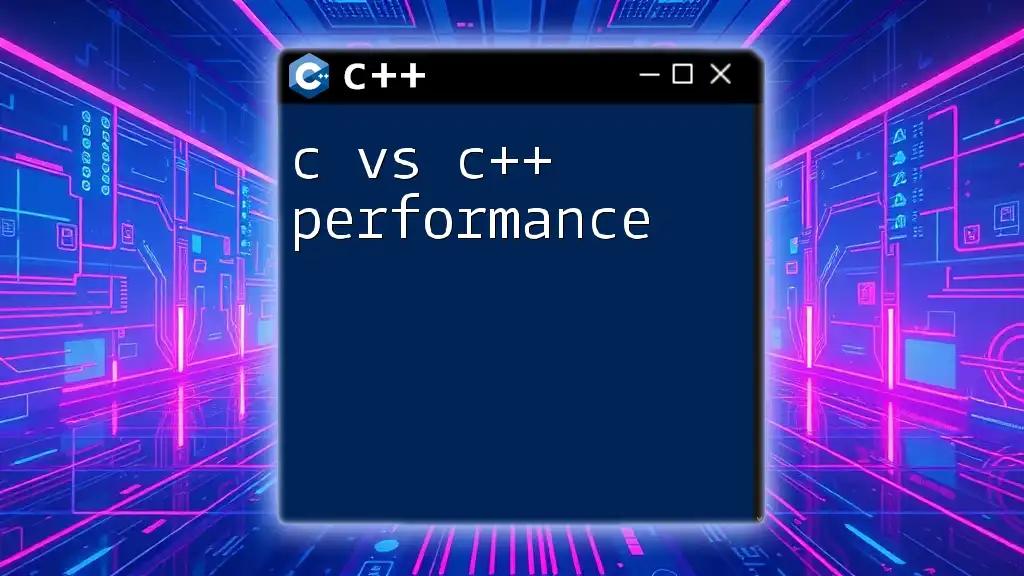
Additional Resources
For further exploration, consider delving into C++ performance books, online courses, and developer forums dedicated to high-performance programming in C++. Tools like `gprof`, `Valgrind`, and various performance profiling software can provide additional insights into optimizing C++ applications effectively.