C++ generally offers superior performance due to its closer-to-hardware capabilities and more extensive optimization options, while Go provides faster development speeds and built-in concurrency features, making it a great choice for networked applications.
#include <iostream>
#include <vector>
#include <chrono>
void cpp_example() {
std::vector<int> numbers(1000000);
auto start = std::chrono::high_resolution_clock::now();
for(int i = 0; i < numbers.size(); ++i) {
numbers[i] = i * 2;
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "C++ execution time: "
<< std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count()
<< "ms" << std::endl;
}
Why Performance Matters in Programming
Performance is critical in programming, affecting how quickly and efficiently applications run. When evaluating performance, we consider several key metrics: speed, memory usage, and concurrency. For instance, in real-time applications such as video games or financial systems, milliseconds can mean the difference between a successful or failed operation. Understanding the nuances of performance helps developers choose the right language and optimize their code accordingly.

Overview of C++
C++ is a powerful, high-performance programming language widely used for system/software development and game programming.
Key Features of C++
C++ combines features of both high-level and low-level languages, providing robust tools for developers. It allows low-level memory manipulation, enabling developers to write very efficient code. C++ is known for its object-oriented and generic programming capabilities. Here's a simple C++ "Hello World" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl;
return 0;
}
This straightforward program illustrates C++ syntax while setting a foundation for more complex features.
Performance Advantages of C++
C++ is a compiled language, leading to faster execution times compared to interpreted languages. This language offers unparalleled control over system resources, allowing developers to fine-tune their applications. High-performance applications, such as gaming engines and real-time simulations, often harness the power of C++ due to its capabilities for optimization and direct hardware manipulation.

Overview of Go
Go, also known as Golang, is designed for simplicity and efficiency, emerging as a strong contender for modern backend systems.
Key Features of Go
Go's design philosophy emphasizes ease of use and productivity, with built-in features for concurrency. The language is statically typed and compiled, with a garbage collection system that manages memory automatically. Here's a simple Go "Hello World" program:
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
Go’s syntax is clean and straightforward, making it accessible for developers of all skill levels.
Performance Benefits of Go
One of Go's most significant advantages is its fast compilation times, producing efficient binaries with low latency. The built-in garbage collector improves memory management, allowing developers to focus on functionality rather than manual memory handling. Furthermore, Go promotes memory safety, which can lead to more stable applications, particularly in web services and microservices architectures.
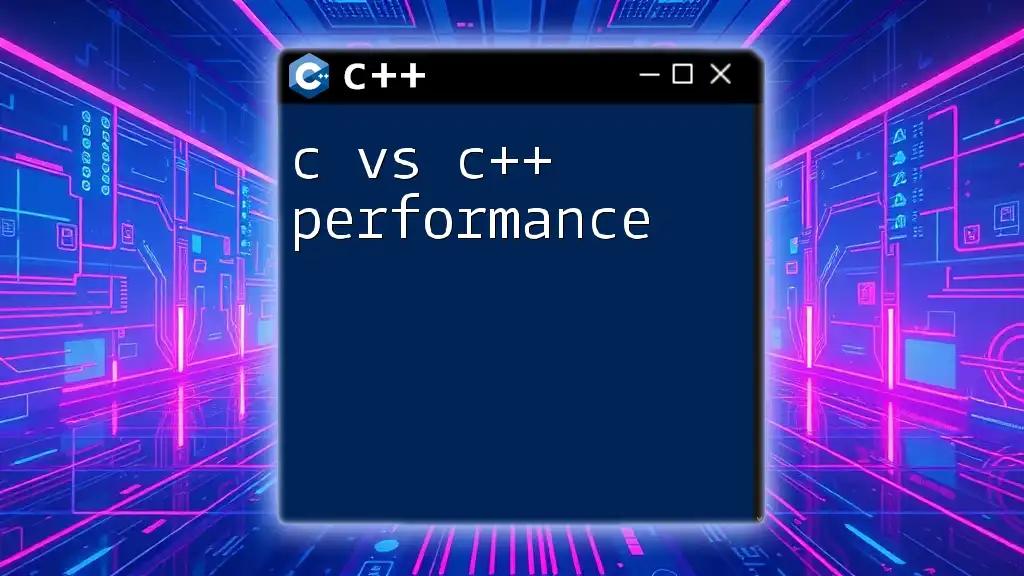
C++ vs Go Performance: A Side-by-Side Comparison
Compilation and Execution Times
In the debate of C++ vs Go performance, compile-time is a crucial factor. C++ typically has a longer compile time due to extensive optimizations, while Go is designed for rapid compilation, making it ideal for iterative development processes. Benchmark tests reveal that C++ often outperforms Go in raw execution speed, but Go’s fast compile times facilitate quicker feedback loops during development.
Memory Management
C++ Memory Management
C++ provides manual memory management, empowering developers to allocate and deallocate memory as needed. This level of control allows for optimized memory usage, but it also increases the risk of memory leaks if not handled correctly. Below is an example of memory management in C++:
int* arr = new int[10]; // memory allocation
delete[] arr; // memory deallocation
Go Memory Management
In contrast, Go employs automatic garbage collection, which takes the burden of memory management off the programmer’s shoulders. This feature speeds up development and minimizes risks associated with improper memory handling, allowing developers to focus on crafting efficient algorithms rather than worrying about memory leaks.
Speed of Execution
When looking at execution speed, C++ commonly shines in CPU-bound applications, mainly due to its low-level capabilities. For example, in computational heavy applications, C++ typically exhibits lower latencies and faster execution times than Go. Benchmarks often demonstrate that while Go provides satisfactory performance, C++ leads in speed, particularly in scenarios requiring intensive data processing.
Concurrency and Parallelism
Concurrency in C++
Concurrency in C++ can be achieved using the threading library, allowing multiple threads to run simultaneously. This provides a way to improve resource utilization, but managing threads can be complex. Below is an example of threading in C++:
#include <thread>
void function_1() {
// Code to be executed in a thread
}
std::thread t1(function_1);
t1.join(); // Wait for t1 to finish
Concurrency in Go
Go simplifies concurrency through goroutines and channels. Goroutines are lightweight and can be created with minimal overhead. Channels facilitate communication between goroutines, offering a clean way to handle concurrent tasks. An example of concurrency in Go is shown below:
go func() {
// perform task
}()
This simplicity allows developers to leverage concurrency without the nuanced complexities found in C++.
Scalability
Scalability is another pivotal factor in choosing between C++ and Go. C++ applications can be highly scalable due to their performance; however, as the complexity of concurrent tasks increases, managing this can become cumbersome. Go’s design includes a built-in model for handling multiple tasks concurrently, making it inherently better suited for scalability, especially in cloud-native environments.
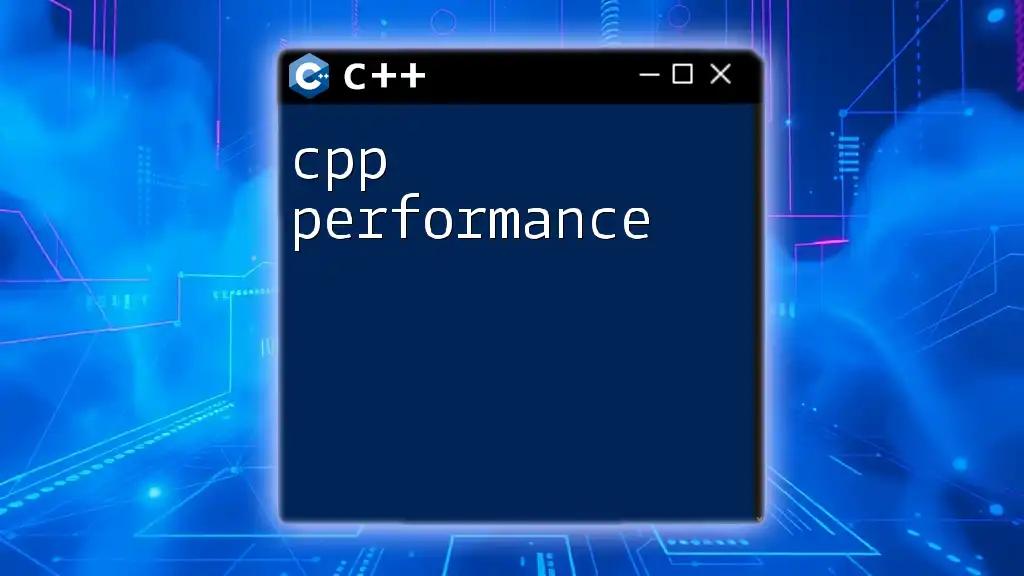
Use Cases: When to Choose C++ or Go
Ideal Scenarios for C++
C++ is the go-to choice for projects where performance and resource control are paramount. Industries such as game development, real-time systems, and system programming frequently utilize C++ to leverage its efficiency and control. For instance, game engines that demand high-performance graphics and rapid execution often rely on C++ capabilities.
Ideal Scenarios for Go
Go shines in modern applications like cloud services, network servers, and microservices. Its strengths lie in ease of development, built-in concurrency support, and performance when dealing with concurrent tasks. The language is particularly effective in projects that require quick deployment and straightforward maintenance, making it a popular choice in fast-paced software development environments.

Conclusion
In the ongoing discussion of C++ vs Go performance, both languages present unique advantages and trade-offs. C++ excels in performance-intensive applications, offering unparalleled control, while Go provides an easier and more efficient path to concurrent programming and web development. Developers must consider their project needs and performance metrics before choosing between these potent languages. Exploring both C++ and Go can lead to a well-rounded skill set for tackling a variety of programming challenges.

Additional Resources
To enhance your understanding of both C++ and Go, consider exploring their respective documentation and performance benchmarks. Engaging with online communities and forums designed for C++ and Go enthusiasts can provide valuable insights and support as you embark on your programming journey. Happy coding!