"CPP performance online" refers to the efficiency and speed of executing C++ commands in web-based environments, where optimizing your code for quick execution is crucial.
Here's a simple code snippet demonstrating a common C++ command for calculating the sum of two numbers:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 10;
int sum = a + b;
cout << "Sum: " << sum << endl;
return 0;
}
Understanding C++ Performance
What is Performance in C++?
Performance in C++ refers to how efficiently a program executes, particularly in terms of speed and resource consumption. The significance of performance cannot be overstated—it directly influences user experience and system resource utilization. Some key performance indicators (KPIs) that developers often look at include:
- Execution Time: How long a program takes to run from start to finish. This metric is critical for applications where users expect quick responses.
- Memory Usage: Measures how much memory a program consumes during its execution. Efficient memory management can lead to better performance and lower costs in cloud environments.
- CPU Efficiency: Relates to how well a program utilizes CPU resources. Highly efficient code minimizes the load on the CPU.
Why C++ Performance Matters
C++ performance is crucial, especially in high-stakes environments such as gaming and finance. Studies have shown that performance can significantly affect profitability, user retention, and overall satisfaction. For example, in gaming, even a minor lag can lead to poor user experience, while in finance, milliseconds may inversely affect trading outcomes. Overall, optimizing for performance is not just about efficiency; it is about aligning with business objectives and user expectations.
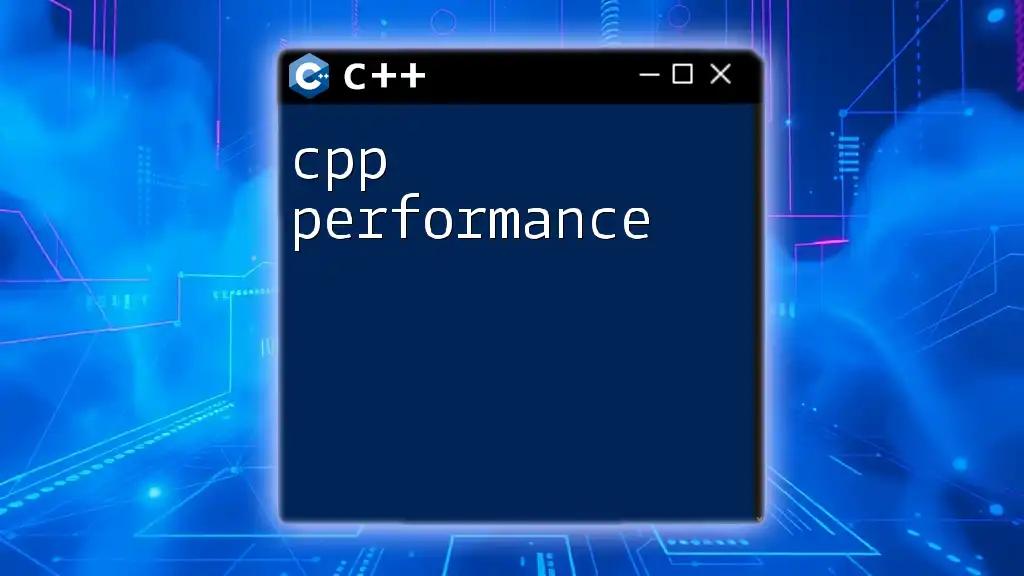
Key Factors Affecting C++ Performance
Compiler Optimization
Compiler optimization techniques can dramatically enhance the performance of C++ programs. Modern compilers offer several optimization flags that can be used to modify how a program is compiled, thereby improving runtime performance. Some common techniques include:
- Inlining Functions: This replaces a function call with the body of the function itself, which can save the overhead of function calls. However, excessive inlining can also lead to increased binary size.
- Loop Unrolling: This reduces the overhead of loop control by expanding the loop body, resulting in faster execution at the cost of increased binary size.
- Dead Code Elimination: Compilers can eliminate code that is never executed, thereby reducing the final binary size and potential load times.
Consider the following example of using compiler optimization flags:
g++ -O2 my_program.cpp -o my_program
Data Structures and Algorithms
Choosing the right data structures can yield significant performance gains. For instance, when considering the differences between Standard Template Library (STL) containers:
- std::vector is often more efficient for random access as it provides contiguity in memory storage. However, inserting or deleting elements can be costly.
- std::list, on the other hand, allows for quick insertions and deletions but lacks efficient random access.
The choice of algorithms also plays a crucial role. Understanding algorithm complexity—best, average, and worst-case scenarios—allows developers to write code that performs optimally under various conditions. For example, using quicksort versus bubblesort can lead to vastly different performance results on large datasets.
Memory Management
Memory management directly influences the performance of C++ applications. Knowing when to use stack versus heap memory is fundamental. Stack allocations are generally faster and automatically cleaned up, while heap allocations offer more flexibility but can lead to fragmentation and memory leaks if not managed correctly.
Utilizing smart pointers—like `unique_ptr` and `shared_ptr`—can improve memory management and reduce bugs associated with raw pointer usage. For example:
#include <memory>
void example() {
std::unique_ptr<int> ptr = std::make_unique<int>(5);
// Automatically deallocated when ptr goes out of scope
}

Profiling C++ Applications
Performance Profiling Tools
Profiling tools are essential for understanding how an application utilizes resources. Popular C++ profiling tools include:
- gprof: A simple profiling tool integrated with GCC that helps identify performance bottlenecks.
- Valgrind: Not only identifies memory leaks but also aids in performance profiling.
- Visual Studio Profiler: Provides detailed insights into application performance and resource consumption.
To utilize `gprof`, for example, you can compile your application with profiling options enabled:
g++ -pg my_program.cpp -o my_program
./my_program
gprof ./my_program gmon.out > analysis.txt
Interpreting Profiling Results
Analyzing profiling results involves understanding where time is being spent within your code. Look for functions that consume excessive resources and investigate potential inefficiencies. For instance, if a specific function is repeatedly mucking through large data structures, consider optimizing or redesigning that function to facilitate quicker access.

Best Practices for Enhancing C++ Performance
Writing Efficient Code
Code efficiency can often be enhanced through clear, concise practices. Guidelines for improving code efficiency include:
- Use const and references whenever possible to avoid unnecessary copying.
- Minimize the scope of variables, especially in large loops, to enhance optimization.
- Eliminate redundant calculations. Store results when calculations are reused.
Leveraging Built-in Functions and Libraries
C++ offers multiple built-in functions and standard algorithms within the STL that are highly optimized. Leveraging these functions can save time and effort while enhancing performance. For example, using `std::sort` instead of writing a custom sorting function can be a significant improvement because it utilizes highly optimized algorithms already implemented in the standard library.
Concurrency and Parallelism
C++ provides tools for leveraging concurrency, allowing programs to perform multiple operations simultaneously. Using threads and asynchronous programming can effectively utilize multi-core architectures.
Here’s a basic example of using threads in C++:
#include <thread>
void threadFunction() {
// Perform operations simultaneously
}
int main() {
std::thread t1(threadFunction);
// Use join to wait for the thread to finish
t1.join();
return 0;
}
With proper synchronization, multi-threading can improve application responsiveness and execution speed.

Real-world Applications of C++ Performance Optimization
Case Study 1: Game Development
In game development, performance optimization is paramount. Developers often employ several tactics including reducing the complexity of rendering techniques and utilizing efficient data structures like quad-trees for collision querying. These optimizations lead to a smoother gaming experience and reliable performance across varying hardware configurations.
Case Study 2: Financial Systems
Financial systems rely on fast and accurate calculations. Given the competitive nature of trading, milliseconds can mean significant profit or loss. Techniques such as multi-threading, efficient algorithms, and optimized data accesses enable systems to handle vast quantities of trades while maintaining reliability.
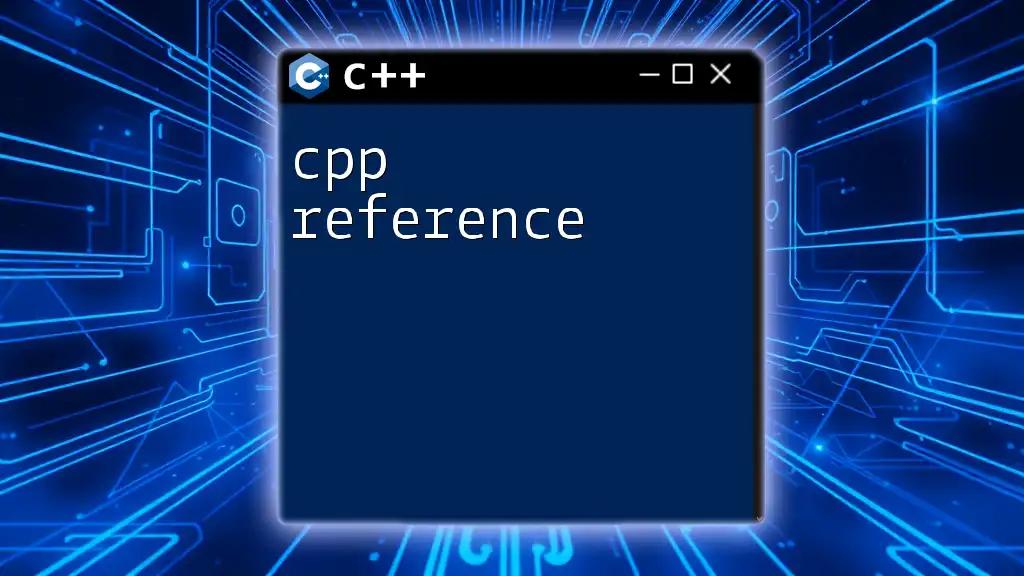
Conclusion
Performance is a critical aspect of C++ programming, affecting everything from user experience to overall application viability. By understanding compiler optimizations, choosing the right data structures and algorithms, managing memory effectively, and utilizing profiling tools, developers can greatly enhance the performance of their C++ applications. Striving for continuous improvement in C++ performance not only benefits individual projects but also contributes to greater industry standards.
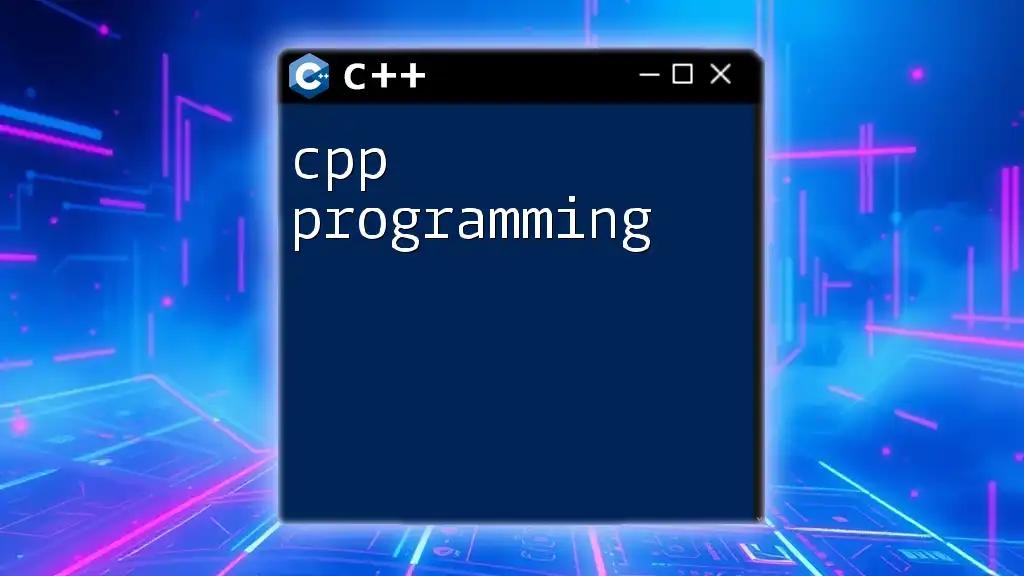
Additional Resources
For those seeking to deepen their understanding of C++ performance, consider exploring additional literature, online courses, and forums focused on best practices, advanced techniques, and the latest developments in the world of C++.