The `std::max_element` function in C++ is used to find the largest element in a range defined by two iterators, returning an iterator to the maximum element found.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 5, 3, 9, 2};
auto maxElement = std::max_element(numbers.begin(), numbers.end());
std::cout << "Maximum element: " << *maxElement << std::endl; // Output: Maximum element: 9
return 0;
}
What is `max_element`?
`max_element` is a function in the C++ Standard Library that finds the largest element within a range of elements. It is a powerful tool primarily used in situations where you need to identify the maximum value quickly and efficiently. Its efficiency makes it a go-to feature in data manipulation tasks, whether in developing algorithms, analyzing datasets, or implementing game logic.
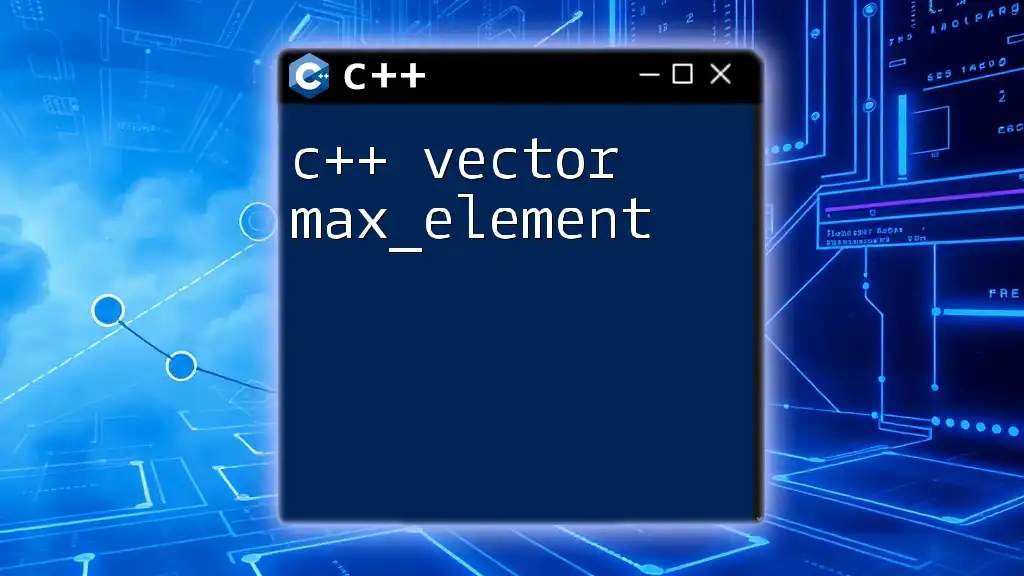
When to Use `max_element`?
You should consider using `max_element` in the following scenarios:
- Data Analysis: Quickly finding the maximum in large datasets can provide significant insights.
- Games and Simulations: Identifying the highest score or value can be essential for game mechanics.
- Algorithm Development: Many algorithms require the determination of maximum values as part of their logic.
Using `max_element` has several advantages over manual searching—the most notable being time efficiency and readability of code. Implementing `max_element` allows you to utilize built-in optimizations instead of writing your search logic.
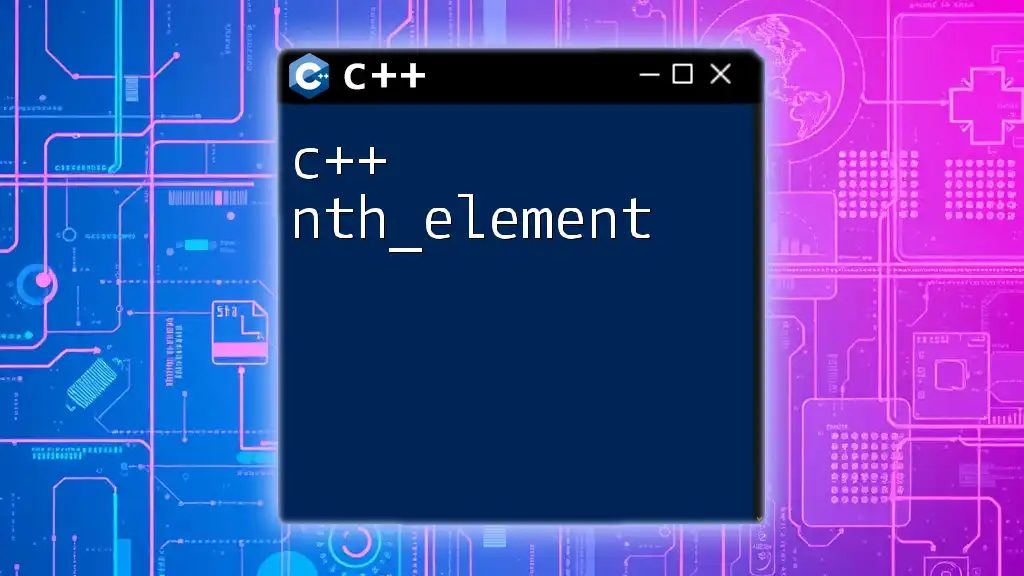
Understanding the Syntax of `max_element`
The basic syntax of `max_element` is as follows:
Iterator max_element(Iterator first, Iterator last);
Iterator max_element(Iterator first, Iterator last, Compare comp);
Parameters Explained
- `first`: An iterator that points to the beginning of the range from which you want to find the maximum element.
- `last`: An iterator that points to one past the end of the range (exclusive).
- `comp`: An optional binary predicate that defines how two elements are compared. If omitted, the function will use the default comparison operator.
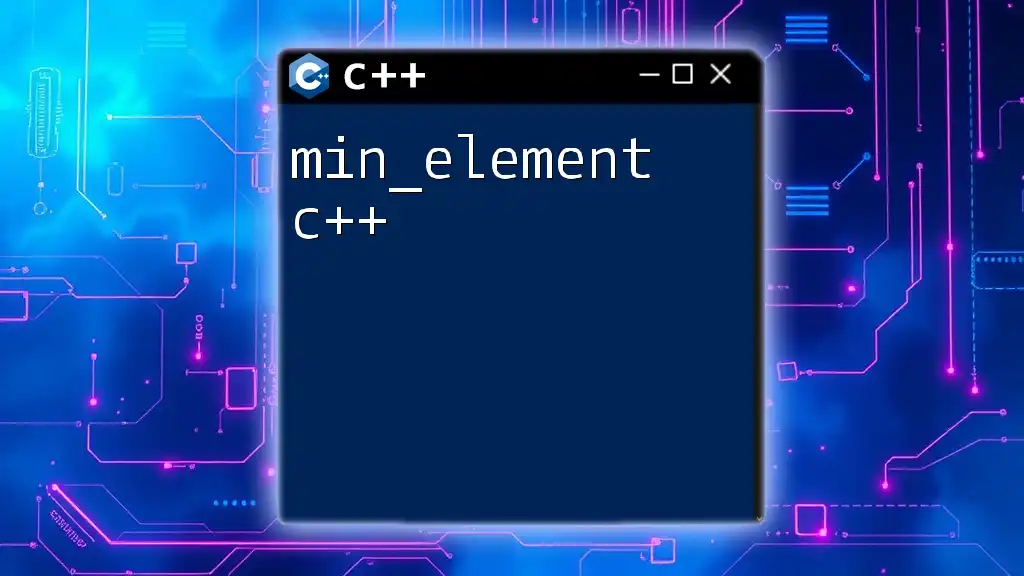
Including Required Headers
To use `max_element`, you need to include the algorithm header from the Standard Library:
#include <algorithm>
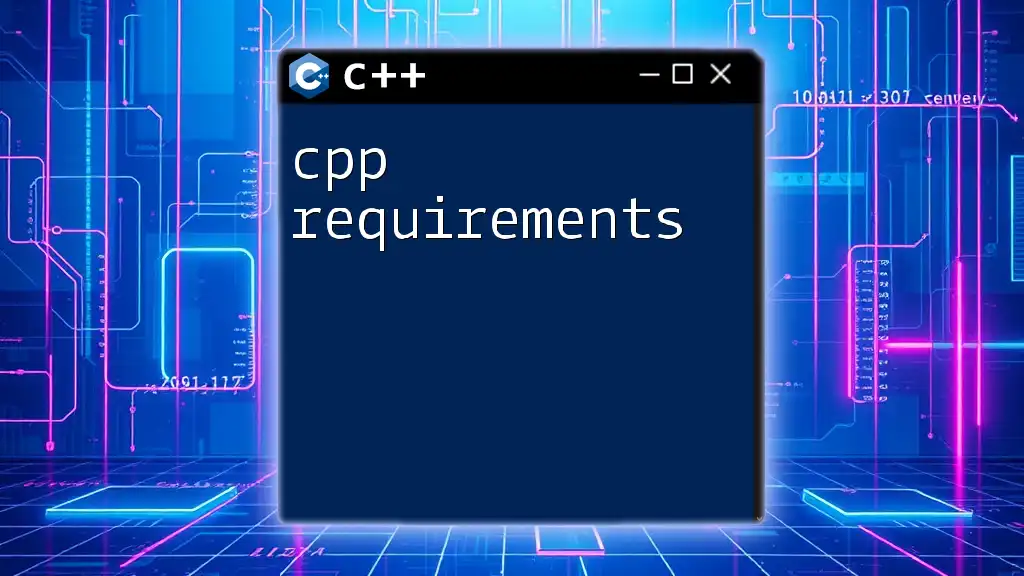
Using `max_element` with Built-in Types
Example with Integer Array
Let’s explore how to use `max_element` with a simple integer array. Here’s a step-by-step guide:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {1, 3, 5, 7, 9};
auto max_iter = std::max_element(arr, arr + 5);
std::cout << "The maximum element is: " << *max_iter << std::endl;
return 0;
}
In this example, `arr` holds a collection of integers. By calling `std::max_element`, you obtain an iterator pointing to the maximum value. Important: You must dereference `max_iter` to retrieve the actual maximum value.
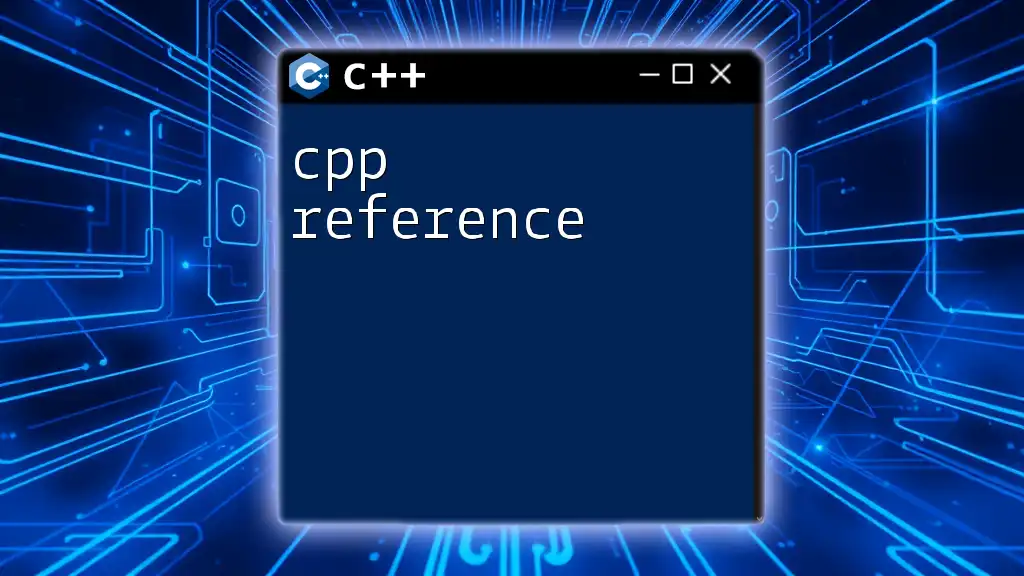
Using `max_element` with Containers
Example with C++ STL Vector
`max_element` is versatile and works seamlessly with various C++ containers, such as `std::vector`. Here's an illustrative example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {2, 4, 6, 8, 10};
auto max_iter = std::max_element(vec.begin(), vec.end());
std::cout << "The maximum element in the vector is: " << *max_iter << std::endl;
return 0;
}
In this case, we use `vec.begin()` and `vec.end()` to define the range. This highlights the adaptability of `max_element`, indicating that it can work with iterators rather than array indices, which enhances code flexibility and readability.
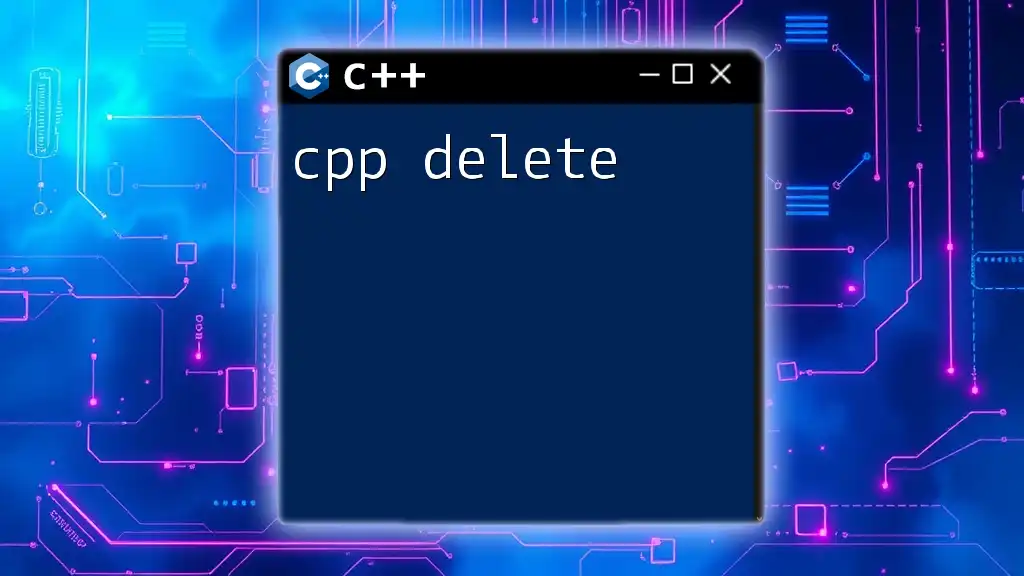
Custom Comparison Function
Using a Custom Comparator
In scenarios where a custom comparison is required, you can define your own comparator. Here’s an example using a structure with price information:
#include <iostream>
#include <vector>
#include <algorithm>
struct Product {
std::string name;
double price;
};
bool comparePrice(const Product &a, const Product &b) {
return a.price < b.price;
}
int main() {
std::vector<Product> products = {{"Apple", 1.2}, {"Banana", 0.8}, {"Cherry", 2.0}};
auto max_iter = std::max_element(products.begin(), products.end(), comparePrice);
std::cout << "The most expensive product is: " << max_iter->name << " at $" << max_iter->price << std::endl;
return 0;
}
In this example, we create a custom comparator, `comparePrice`, which helps determine the product with the highest price. This showcases the versatility of `max_element`, enabling tailored comparisons according to specific criteria.
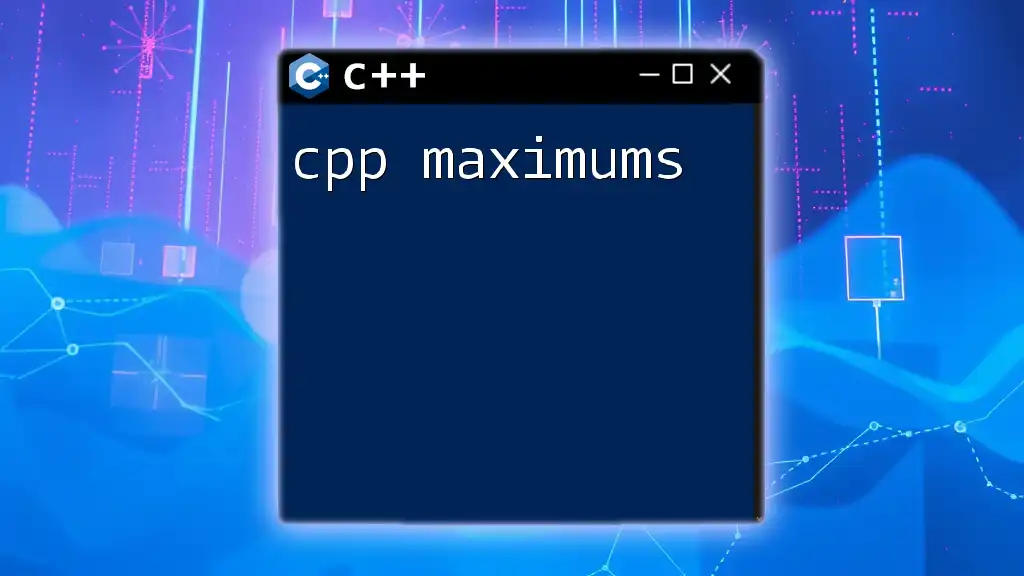
Handling Edge Cases
Empty Ranges
A common edge case arises when `max_element` is called on an empty range. The function's behavior is undefined, which can lead to issues if your code doesn’t account for this. To avoid this, ensure you check the range size before calling `max_element`.
Identical Elements
When all elements in the range are identical, `max_element` will return an iterator pointing to the first maximum value. This behavior can be useful to understand, especially when working with data sets that can contain duplicates.
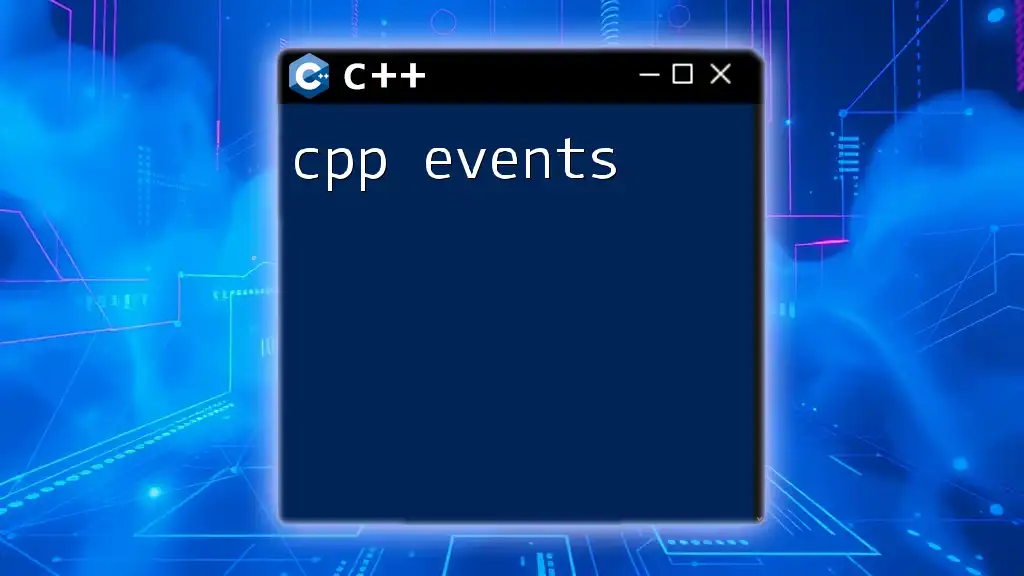
Performance Considerations
Time Complexity
The time complexity of `max_element` is O(n), where n is the number of elements in the range. Despite being linear time, `max_element` is highly efficient in practice due to its internal optimizations.
Best Practices
While `max_element` is generally performant, be cautious when working with exceedingly large datasets. If defining a custom comparison, ensure that it isn't computationally prohibitive, as this can significantly affect performance.
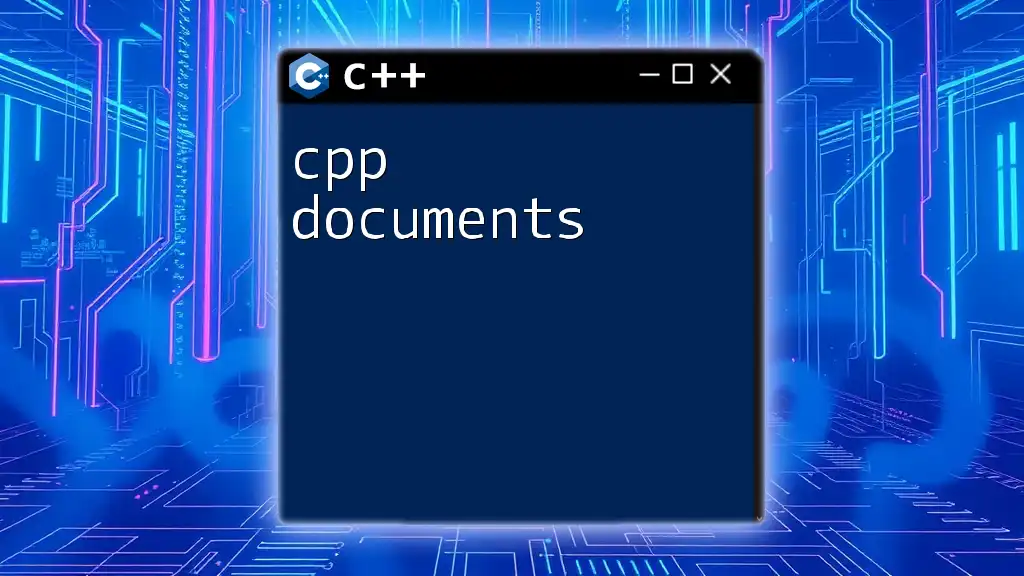
Conclusion
In summary, `cpp max_element` is an essential function that enhances your capability to work with collections in C++. Its efficiency, versatility with various data structures, and the ability to utilize custom comparison functions make it an invaluable tool in your programming toolkit. Don’t hesitate to integrate it into your coding practice and explore its potential across different scenarios.
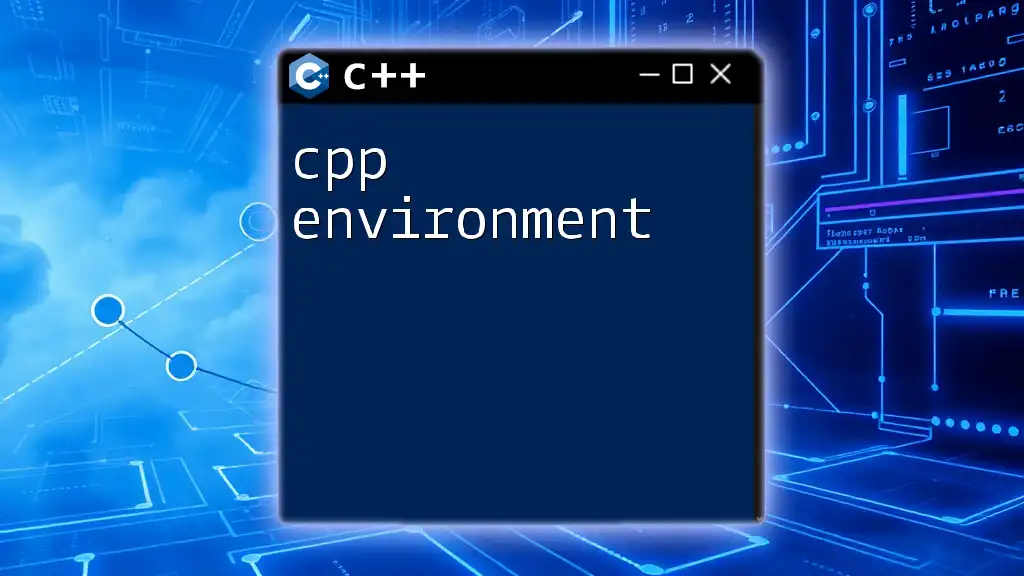
Additional Resources
To deepen your understanding and practices with `max_element`, consult the C++ Standard Library documentation. Engaging with additional tutorials can also provide further insights into C++ features and algorithms, enabling you to become proficient in the language.