C++ documents are structured files that contain C++ source code, which can include declarations, definitions, and commands that are compiled to create applications.
Here's a simple example of a C++ 'Hello, World!' program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Document Types
Technical Documentation
Technical documentation serves as a crucial resource for understanding C++ applications. This type of documentation often includes API references and user manuals, both of which are essential for developers and end-users. It helps users comprehend how to interact with software and features, thus facilitating smoother integration and usage. Well-crafted technical documentation can significantly reduce user frustration and support queries, improving overall user experience.
Code Documentation
In-line Documentation plays a vital role in enhancing code readability. By using comments to explain complex logic and provide context, developers can help others (or themselves) understand the thought process behind their code at a later date. For example:
// This function calculates the factorial of a number
int factorial(int n) {
if (n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive call
}
In this code snippet, the comments clarify the purpose of both the function and the logic used within it. Such clarity is crucial, especially in collaborative environments where multiple developers are working on the same codebase.
Function Documentation is another important aspect, which usually takes the form of docstrings or comments above functions. This establishes what a function does, its parameters, and return values. Consider the following example:
/**
* Calculates the factorial of a given number.
*
* @param n The non-negative integer value to calculate the factorial for.
* @return The factorial of the input number.
*/
int factorial(int n);
This documentation snippet succinctly conveys vital information about the `factorial` function, ensuring that any user can understand its purpose and requirements without needing to dive into the implementation details.
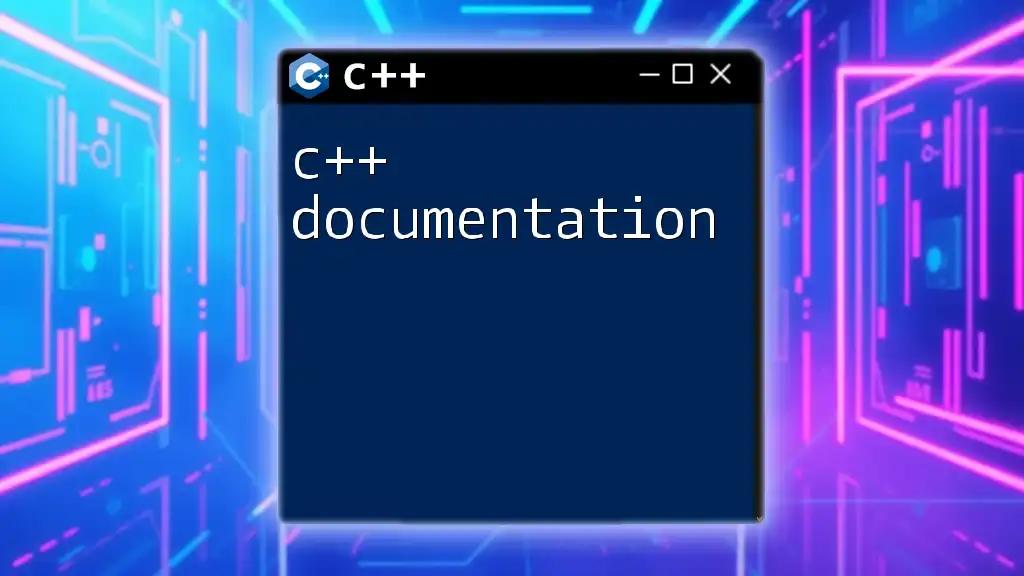
Best Practices for Writing C++ Documents
Use of Comments
Choosing the right type of comment is key to effective C++ documentation. Single-line comments are useful for brief clarifications, while multi-line comments can provide comprehensive explanations. It’s important to strike a balance—avoid excessive commenting that clutters the code, yet do not leave essential logic unexplained.
Consistent Naming Conventions
Adhering to consistent naming conventions is fundamental in enhancing code clarity. Variable and function names should be descriptive and follow a standard pattern. For example, instead of naming a variable with a vague `x`, use `employeeCount` to make its purpose clear. Poor naming conventions lead to confusion, requiring additional documentation to clarify intent, which is counterproductive.
Structure and Organization
The organization of code files is crucial, especially as projects grow larger. Logical segmentation—such as categorizing files into folders by functionality—makes it much easier for developers to find and understand components. Using a structured approach not only reduces the time spent searching for files but also enhances the overall maintainability of the project.
Automated Documentation Tools
Doxygen is a powerful tool for generating documentation directly from annotated C++ code. This can save time and effort while ensuring that documentation is kept up to date. To set up Doxygen, follow these steps:
-
Generate a default configuration file:
doxygen -g
-
Run Doxygen with your configuration file:
doxygen Doxyfile
Doxygen integrates seamlessly with C++ and automatically creates user-friendly documentation in various formats, such as HTML and PDF.

Examples of Well-Documented C++ Projects
Open Source C++ Projects
Examining well-documented open-source projects can provide invaluable insights into effective documentation practices. Projects like Boost and OpenCV are renowned for their robust documentation. The clarity in their descriptions, combined with a structured format, sets a benchmark for aspiring developers on how to document their code properly.
Personal Project Case Study
Consider a hypothetical project implementing a simple library management system. In such a project, focusing on function-level documentation and comprehensive README files detailing installation procedures, usage, and examples will greatly enhance user understanding. Sharing snippets of the code along with well-placed comments will demonstrate best practices in action, reinforcing the importance of documentation.
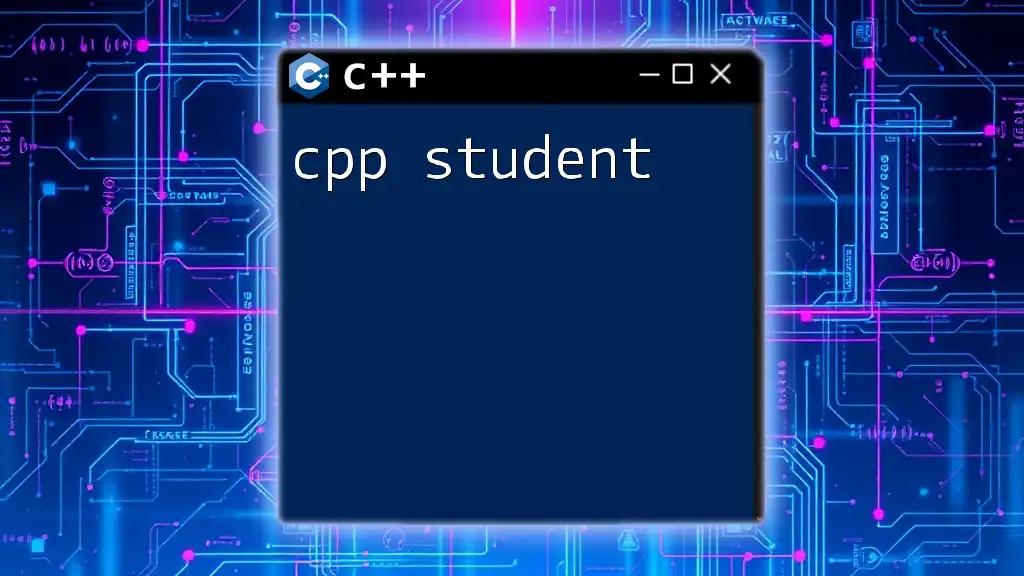
Common Pitfalls in C++ Documentation
Over-commenting vs. Under-commenting
One of the most prevalent challenges is finding the right balance between over-commenting and under-commenting. Over-commenting can lead to confusion and distraction, obscuring clarity by cluttering the code structure. Conversely, under-commenting can leave users without sufficient context. For optimal documentation, comments should be purposeful and help clarify the intent without overwhelming the reader.
Outdated Documentation
Keeping documentation current is essential for effective development. As code changes, documentation may often be neglected, leading to discrepancies between what the code does and what the documentation states. Regular reviews and updates of documentation alongside code changes will ensure that all contributors have access to accurate information, ultimately maintaining the quality of the project.
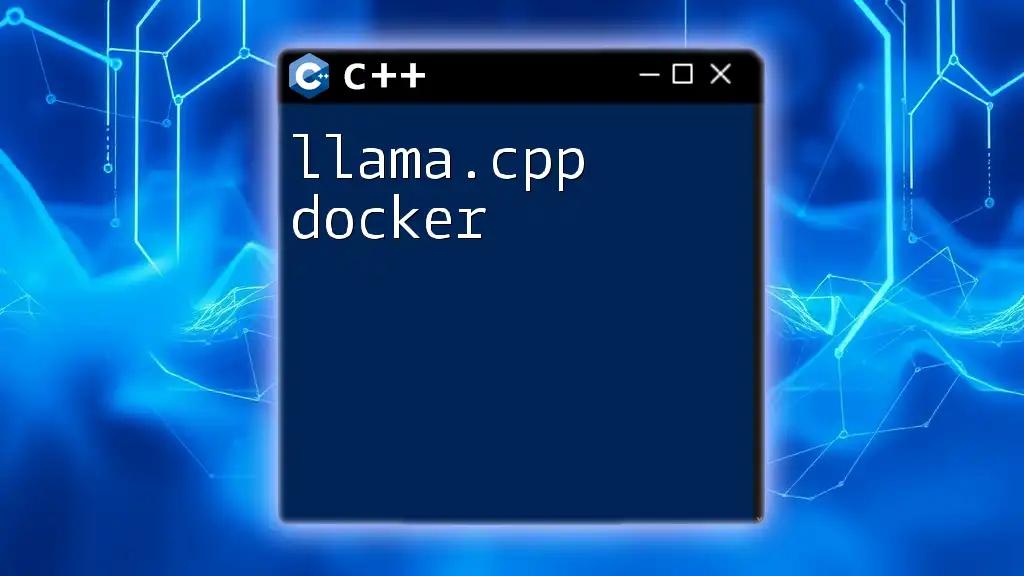
Conclusion
In summary, C++ documents are essential not just for the current execution of code but also for maintaining and enhancing it over time. Engaging in effective documentation practices can improve collaboration and knowledge sharing across teams. By adhering to the best practices discussed and examining real-world examples, developers can elevate their coding standards and contribute positively to the programming community.
Call to Action
Start documenting your code today! Take the initially daunting task of writing documentation and integrate it into your coding practice. Remember that good documentation benefits everyone involved, from developers to end-users.
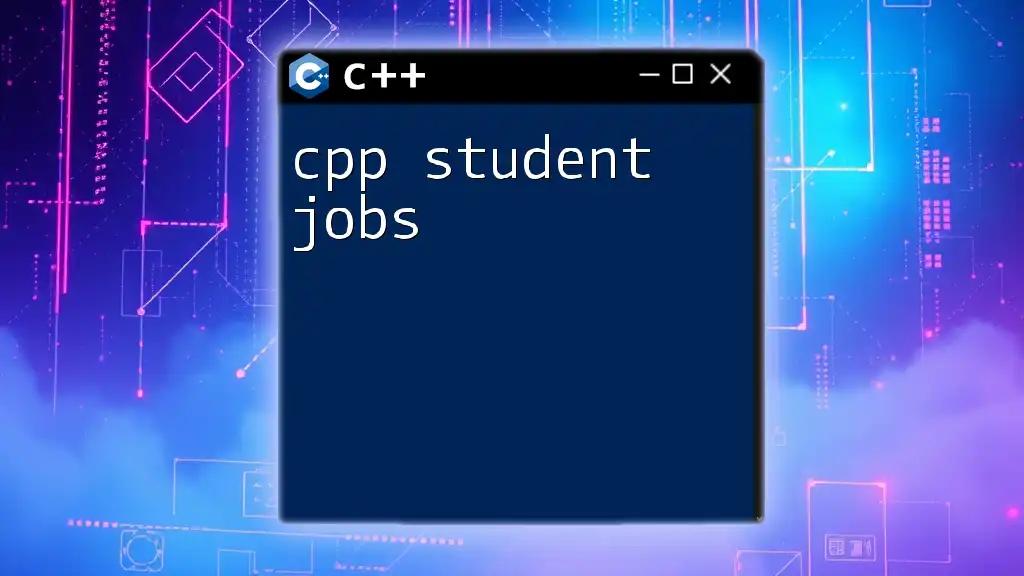
Resources for Further Learning
To continue your journey in mastering C++ documentation, consider exploring the following:
- Recommended Books: Look for resources that focus on C++ best practices and effective documentation strategies.
- Documentation Tools: Doxygen, Sphinx, and other documentation generators can significantly streamline your workflow.
- Online Resources: Engaging with communities, forums, and tutorials that concentrate on C++ can provide additional insights and support.
By investing time into understanding and applying effective documentation practices in your C++ projects, you set a solid foundation for your development work, ensuring clarity and sustainability for years to come.