C++ objects are instances of classes that encapsulate data and behavior, allowing for organized and modular programming.
Here's a simple example of a C++ class and object:
class Car {
public:
string model;
int year;
void displayInfo() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
int main() {
Car myCar;
myCar.model = "Toyota";
myCar.year = 2022;
myCar.displayInfo();
return 0;
}
What is an Object in C++?
In C++, an object is an instance of a class. It encapsulates both data (attributes) and functions (methods) that operate on the data, serving as a self-contained entity. This concept is central to Object-Oriented Programming (OOP), which promotes reusable and modular code. In practical terms, every time you define a class, you can create multiple objects of that class, each with its own set of properties and behavior.
The importance of objects in C++ cannot be overstated; they allow programmers to model real-world entities more effectively by grouping related data and functionalities. Objects make managing complex systems easier, enhancing both readability and maintainability.
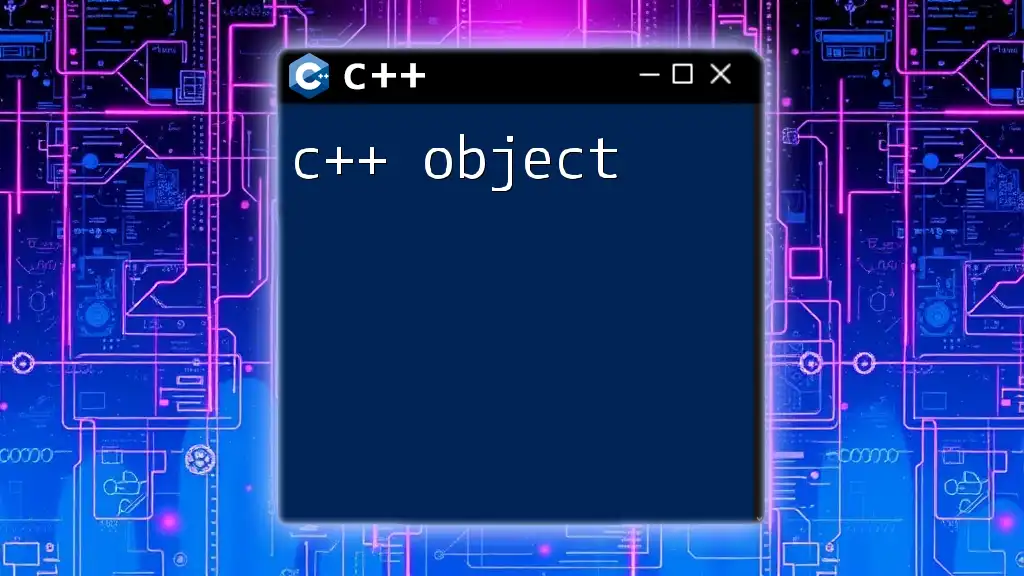
C++ Objects: The Building Blocks of OOP
C++ encourages a paradigm that revolves around the use of classes and objects. The class serves as a blueprint for creating objects, defining the structure and behavior that the objects will exhibit. When you create an object from a class, you are essentially instantiating that blueprint into a usable entity.
Characteristics of Objects:
- Encapsulation: Objects bundle data and methods together, restricting direct access to some components.
- Inheritance: Objects can inherit properties from other objects, promoting reuse and creating a hierarchy.
- Polymorphism: Objects can be treated as instances of their parent class, allowing for flexibility in code.
Understanding the core elements of object-oriented programming is crucial for efficient software design and development.
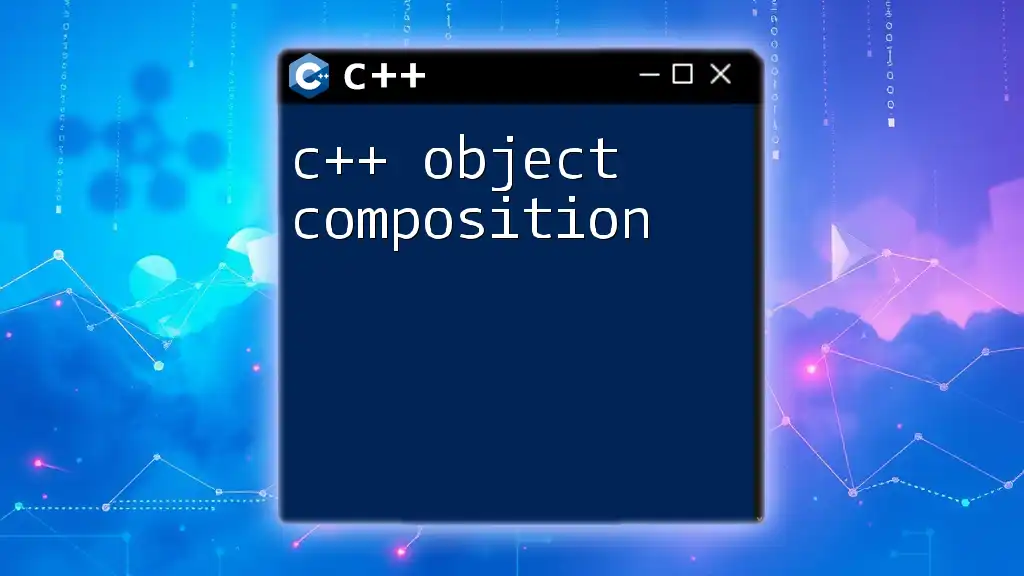
Defining a Class in C++
To create C++ objects, you first need to define a class that outlines the properties and behaviors. The syntax for defining a class is straightforward:
class Vehicle {
public:
string brand;
int year;
void display() {
cout << brand << " was manufactured in " << year << endl;
}
};
In this example, the `Vehicle` class has two public attributes (`brand` and `year`) and one method (`display()`) that outputs these values when called. Having this class available enables the creation of various vehicle objects.
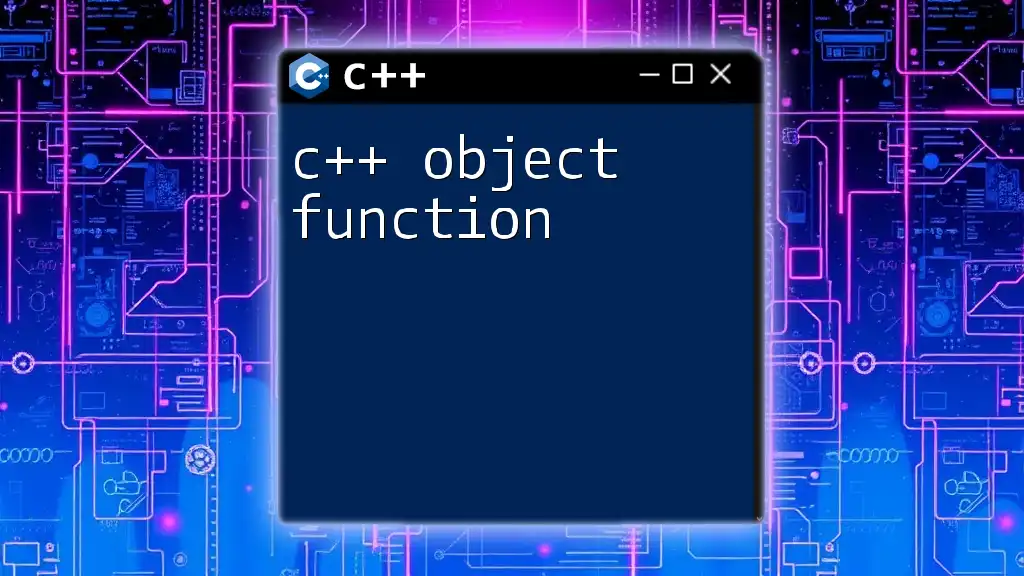
Instantiating Objects in C++
Once a class is defined, you can create objects based on that class. This process is called instantiation.
For example, consider the following code that illustrates how to create an object and access its properties:
Vehicle car;
car.brand = "Toyota";
car.year = 2020;
car.display(); // Outputs: Toyota was manufactured in 2020
Here, `car` is an object of the `Vehicle` class, allowing you to set its attributes and call its methods directly. Each object can store different data, making them unique instances of the class.
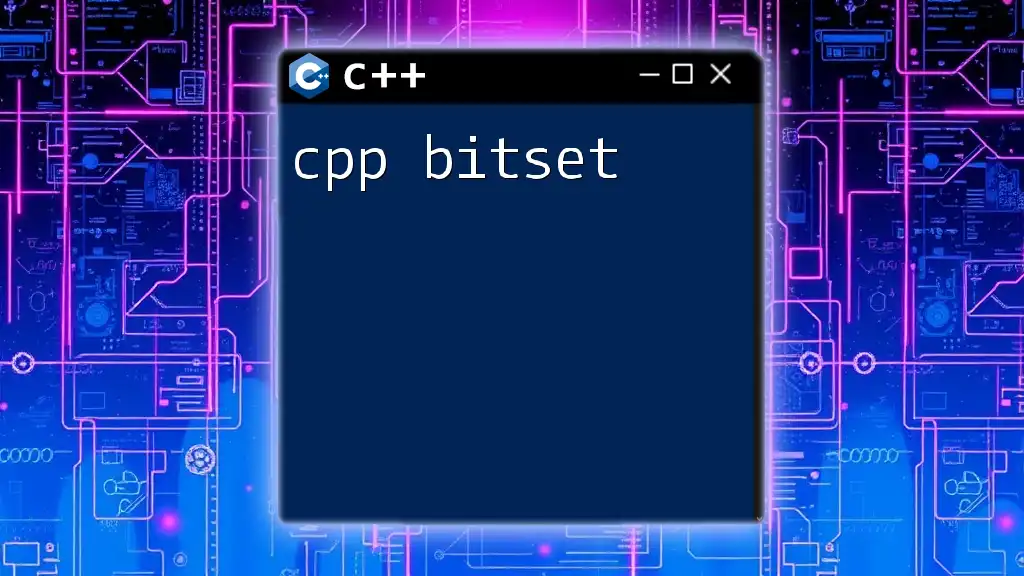
Accessing Object Members
To interact with an object's attributes and methods, you utilize the dot operator (`.`).
It's also essential to understand access specifiers—such as public, private, and protected—that dictate how attributes and methods can be accessed. Public members can be accessed from outside the class, while private members can only be accessed within the class itself.
Example of Accessing Object Members
class Vehicle {
private:
string brand;
public:
void setBrand(string b) {
brand = b;
}
string getBrand() {
return brand;
}
};
Vehicle myVehicle;
myVehicle.setBrand("Honda");
cout << myVehicle.getBrand(); // Outputs: Honda
In this example, the `brand` attribute is private, emphasizing the importance of data encapsulation in C++. The methods `setBrand()` and `getBrand()` provide controlled access to the private member.
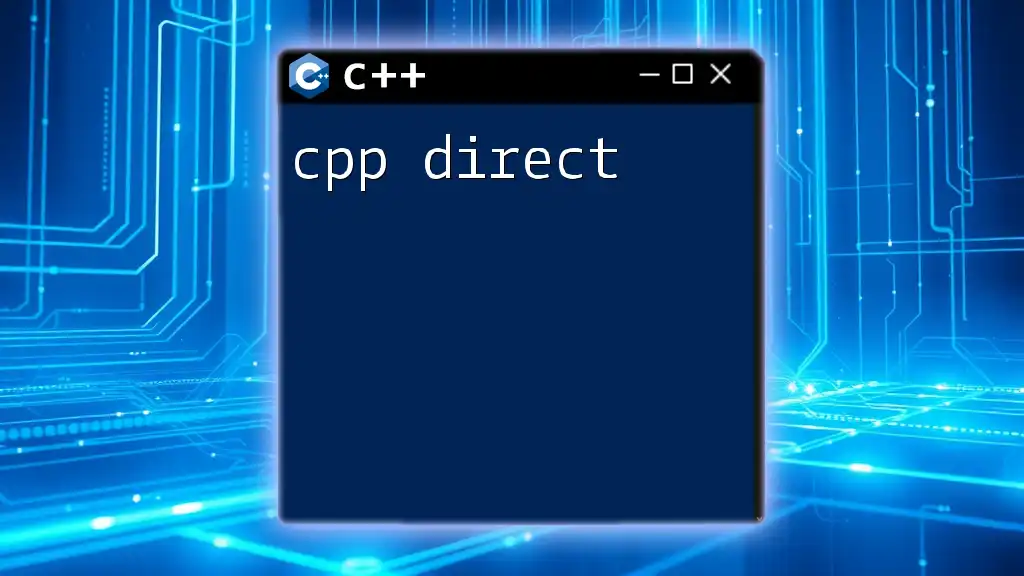
Object Lifecycle in C++
Understanding the lifecycle of an object from creation to destruction is critical in managing resources effectively.
Object Creation
Objects can be created on the stack or the heap.
- Stack allocation is faster and automatically handles resource deallocation when the object goes out of scope.
- Heap allocation provides more flexibility but requires manual management, typically using `new` and `delete`.
Vehicle *ptrCar = new Vehicle(); // Heap Allocation
Vehicle myCar; // Stack Allocation
In this snippet, `ptrCar` is allocated on the heap, while `myCar` exists on the stack.
Object Destruction
A class can also define a destructor, which is invoked when an object goes out of scope. The purpose of a destructor is to clean up resources that the object may have acquired during its lifetime.
class Vehicle {
public:
~Vehicle() {
cout << "Vehicle destroyed." << endl;
}
};
The destructor `~Vehicle()` outputs a message upon object destruction, which can be useful for debugging memory management issues.
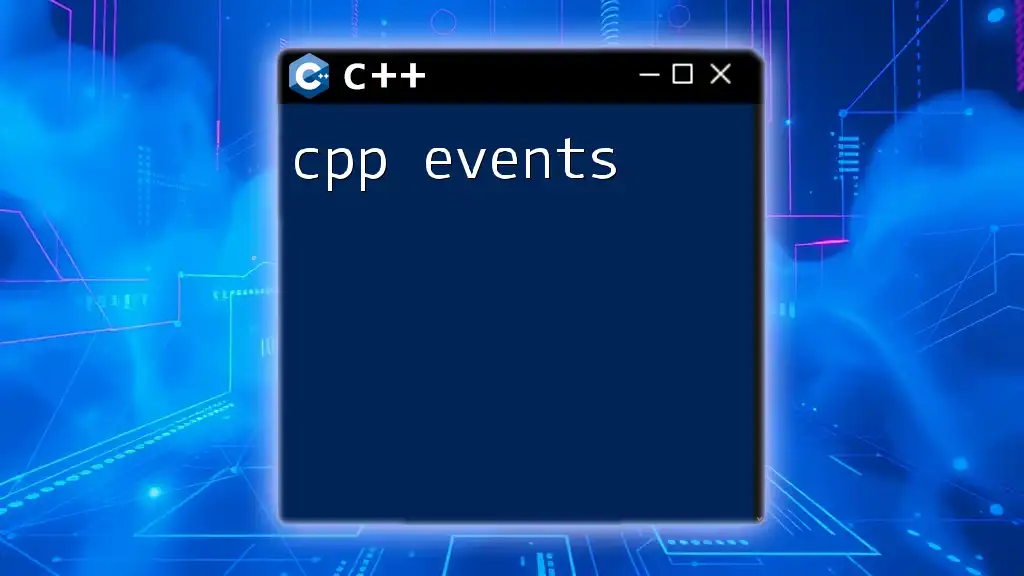
Object Copying and Assignment
When working with objects, you may need to copy or assign them to other objects. This requires comprehension of copy constructors and assignment operators.
Copy Constructor
A copy constructor allows for creating a new object as a copy of an existing object.
class Vehicle {
public:
string brand;
// Copy Constructor
Vehicle(const Vehicle &v) {
brand = v.brand;
}
};
Vehicle car1;
car1.brand = "BMW";
Vehicle car2 = car1; // Calls the copy constructor
In this example, `car2` is a new object initialized by copying the data from `car1`.
Deep vs. Shallow Copying
Understanding the distinction between deep and shallow copying is vital when dealing with objects that manage dynamic resources. A shallow copy duplicates the pointer values, leading to potential issues if both objects manipulate the shared resources. A deep copy duplicates the actual content, ensuring that each object manages its own resources independently.
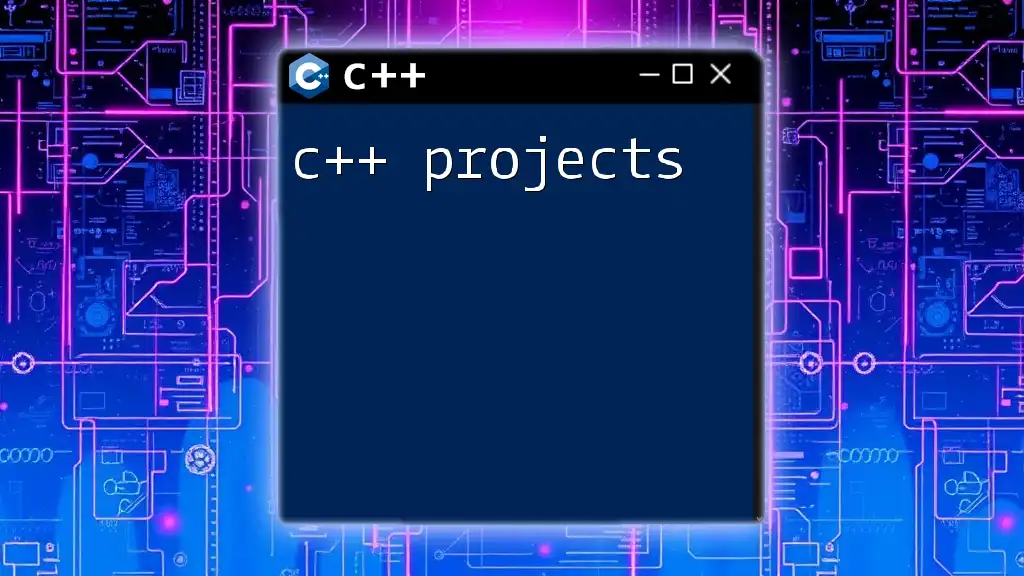
Object Composition and Aggregation
Composition and aggregation are design techniques in which one class contains references to other classes, thus forming complex entities.
- Composition indicates a strong relationship where the lifetime of the contained object depends on the container.
- Aggregation describes a weaker relationship where the contained object's lifecycle is independent of the container.
Example of Composition
class Engine {
public:
string type;
};
class Car {
private:
Engine engine; // Composition: Engine's lifecycle is tied to Car
public:
void setEngineType(string t) {
engine.type = t;
}
};
In this case, if a Car object is destroyed, its contained Engine object will also cease to exist.
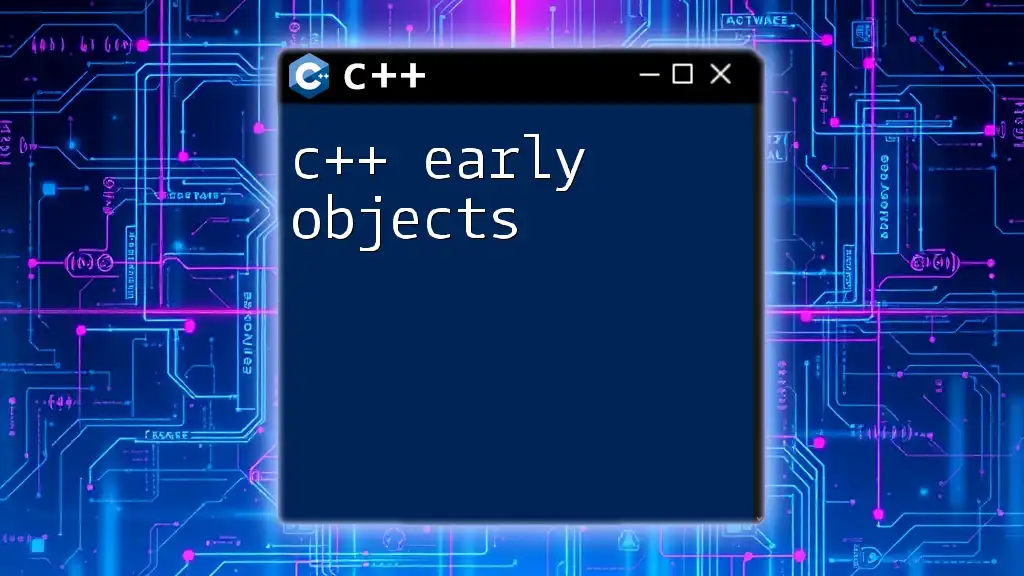
Real-World Examples of C++ Objects
Consider a banking system where you might define an `Account` class to model bank accounts:
class Account {
private:
double balance;
public:
Account() : balance(0) {}
void deposit(double amount) {
balance += amount;
}
double getBalance() {
return balance;
}
};
Similarly, in a library management system, you might have a `Book` class:
class Book {
private:
string title;
public:
Book(string t) : title(t) {}
string getTitle() {
return title;
}
};
In these scenarios, each account or book represents an object with its own identity and behaviors, reinforcing the utility of C++ objects.
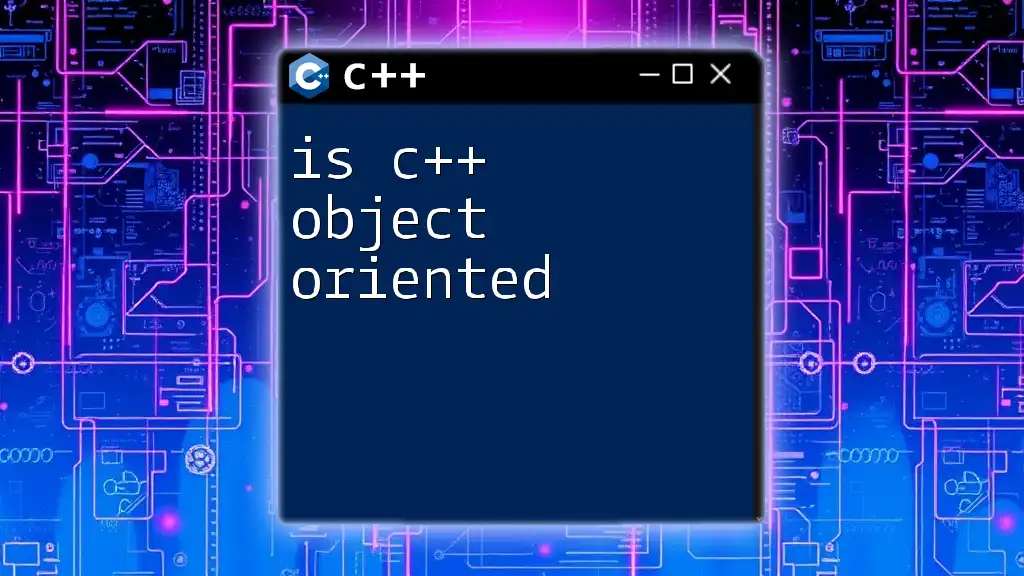
Conclusion
Understanding C++ objects is paramount for anyone aspiring to master C++. They form the backbone of object-oriented programming, allowing for better organization and modularity in code. The creation, manipulation, and management of objects enable developers to model complex systems effectively while adhering to robust programming principles.
To deepen your knowledge, engage in practical exercises by creating various C++ objects and exploring their interactions. Expanding your expertise in this area will significantly enhance your programming capabilities.
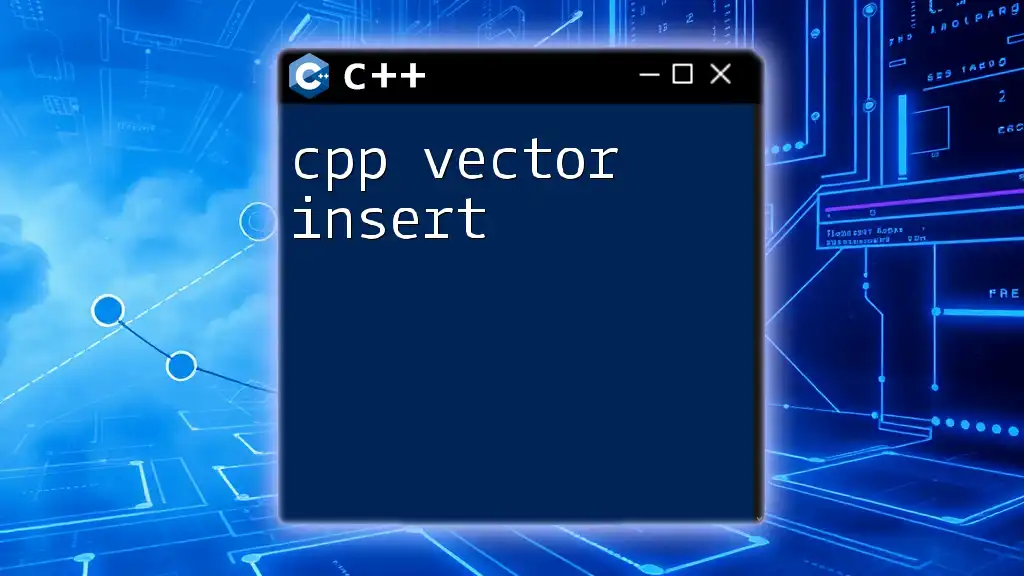
Further Learning Resources
If you're eager to learn more about C++ and object-oriented programming, consider diving into reputable books, online courses, or official documentation that can complement your understanding of these fundamental concepts.
Call to Action
Stay updated with the latest tutorials and insights by subscribing to our newsletter, where you'll discover tips and tricks for mastering C++ commands and object-oriented programming!