In C++, an object is an instance of a class that encapsulates data and functions, allowing for modular programming and data abstraction.
Here's a simple example of creating a class and an object in C++:
class Car {
public:
void honk() {
std::cout << "Beep! Beep!" << std::endl;
}
};
int main() {
Car myCar; // Creating an object of the Car class
myCar.honk(); // Calling the honk method on the object
return 0;
}
Understanding C++ Objects
What is an Object in C++?
A C++ object is an instance of a class, which is a blueprint or template for creating objects. Objects encapsulate data and the functions that operate on that data, allowing for a more organized and modular approach to programming. Think of an object as a real-world entity; for instance, if you have a class `Car`, then an object like `myCar` is a specific car with particular attributes such as color and speed.
Components of an Object
An object comprises two main components: attributes and methods.
- Attributes (also known as data members) hold the state of the object. In our `Car` class example, attributes might include `color` and `speed`.
- Methods (or member functions) define the behavior of the object. For example, a method like `accelerate()` can increase the speed attribute.
Here’s a simple example to illustrate this concept:
class Car {
public:
string color;
int speed;
void accelerate() {
speed += 10;
}
};
In this snippet, `color` and `speed` are attributes, while `accelerate()` is a method that modifies the object's state.
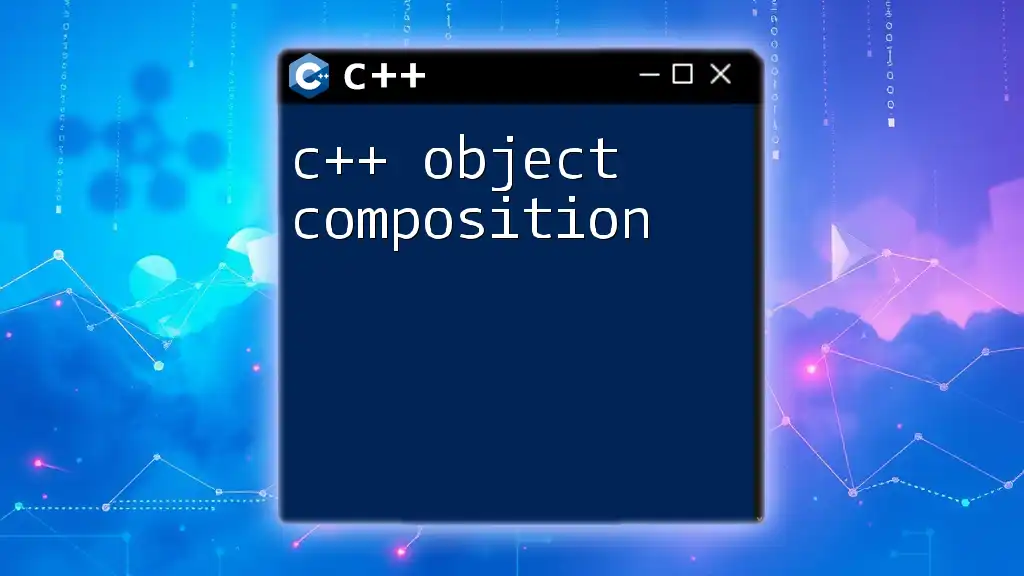
The Fundamentals of C++ Objects
C++ Classes vs Objects
A class serves as the blueprint for creating objects. A class defines what attributes and methods its objects will have. In our earlier example, we defined a `Car` class to describe the properties and behaviors of a car.
To create an object from a class, you must instantiate it. Here’s how to define the object using the class:
Car myCar;
Creating an Object in C++
When you create an object, you allocate memory for it automatically. The syntax for creating an object involves specifying the class name followed by the object name. In the previous example, `myCar` is an object of the `Car` class created on the stack, meaning it will be destroyed automatically when it goes out of scope.
Accessing Object Members
To access an object's attributes and methods, you use the dot operator (`.`).
For instance, to set the color of the `myCar` object and call the `accelerate()` method, the code would look like this:
myCar.color = "Red"; // Accessing attribute
myCar.accelerate(); // Calling method
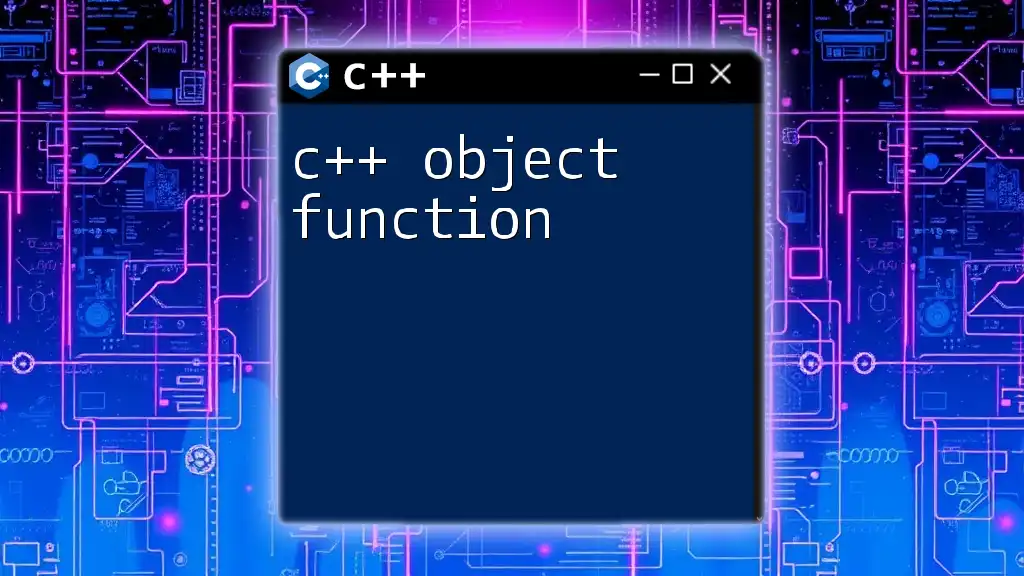
Types of Objects in C++
Static and Dynamic Objects
C++ allows for both static and dynamic objects.
Understanding Static Objects
Static objects are allocated on the stack and automatically cleaned up after they go out of scope. Here’s how you declare a static object:
void exampleFunction() {
Car myCar; // Static object
}
Once `exampleFunction` exits, `myCar` is destroyed.
Understanding Dynamic Objects
Dynamic objects are allocated on the heap using the `new` keyword and must be manually managed. Here's how you can create a dynamic object:
Car* myDynamicCar = new Car(); // Dynamic object
To avoid memory leaks, it's crucial to release the allocated memory using `delete` once the object is no longer needed:
delete myDynamicCar; // Deleting dynamic object
Global and Local Objects
Objects can also be categorized based on their scope.
Global Objects
A global object is accessible from anywhere in the code. If you declare an object outside of any function, it is considered global:
Car myGlobalCar; // Global object
Local Objects
Local objects exist only within the scope of a function. If declared inside a function, these objects are destroyed when the function exits:
void function() {
Car myLocalCar; // Local object
} // myLocalCar is destroyed here
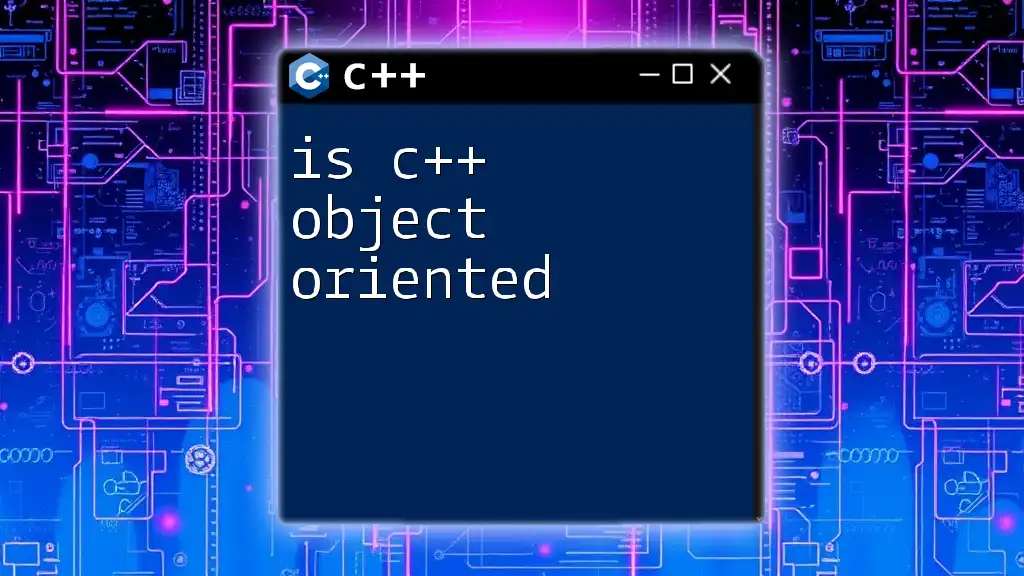
Advanced Concepts in C++ Objects
Copy Constructors and Assignment Operators
Understanding how to copy objects correctly is crucial for managing their state and memory.
Understanding Copy Constructors
A copy constructor is called when an object is initialized using another object of the same class. It makes a copy of the object’s attributes. Here’s an example:
class Car {
public:
string color;
int speed;
Car(const Car &other) { // Copy constructor
color = other.color;
speed = other.speed;
}
};
Operator Overloading for Assignment
You can define how the assignment operator works for your class, which is useful when assigning one object to another. Here's how to overload the assignment operator:
Car& operator=(const Car &other) {
if (this != &other) {
color = other.color;
speed = other.speed;
}
return *this;
}
Dynamic Memory Management for Objects
Dynamic memory management is crucial when using dynamic objects. Allocate memory with `new` and free it with `delete` to prevent memory leaks.
Ensure you are careful with ownership: if multiple objects share the same allocated memory, avoid double deletion by properly managing copy constructors and assignment operators.
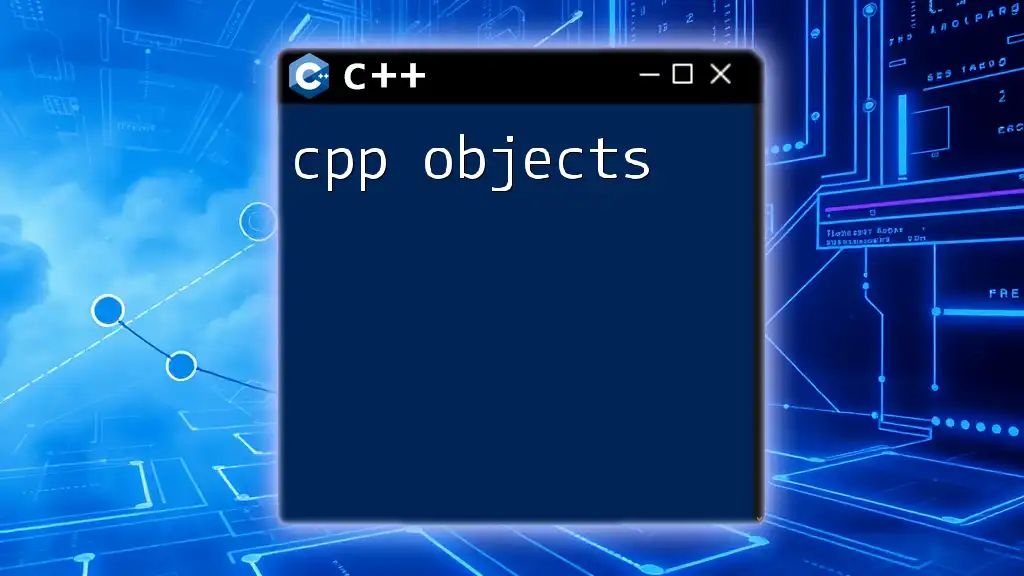
Common Misconceptions About C++ Objects
Objects vs Structures
A common misconception is that objects and structures are identical in C++. While both can have attributes and methods, structures are primarily used for data aggregation without encapsulation, whereas classes emphasize encapsulation and contain methods.
Here’s a simple comparison:
struct CarStruct {
string color;
int speed;
};
class CarClass {
public:
string color;
int speed;
void accelerate() {
speed += 10;
}
};
Mistakes to Avoid with C++ Objects
There are a few common pitfalls programmers face when working with C++ objects:
- Improper access: Always ensure you access object members using the correct syntax.
- Memory leaks: Always free dynamically allocated memory.
- Not implementing copy constructors: Avoid shallow copies which can lead to unintended side effects.
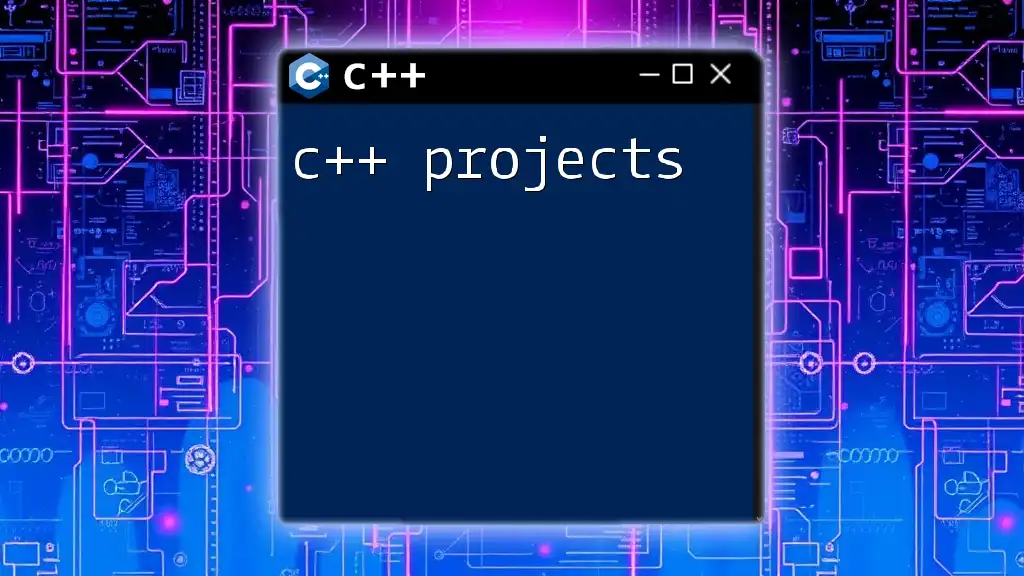
Conclusion
Wrapping Up C++ Objects
Understanding C++ objects is fundamental for leveraging the object-oriented paradigm effectively. By encapsulating attributes and methods, you can develop organized and maintainable code. This knowledge is critical for efficient programming and software design.
Call to Action
By practicing with C++ objects, you will enhance your programming skills. Experiment by creating your classes and manipulating objects to reinforce your understanding of this vital concept in C++.