In C++, the size of a vector can be obtained using the `size()` member function, which returns the number of elements currently stored in the vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Size of vector: " << vec.size() << std::endl; // Output: Size of vector: 5
return 0;
}
Understanding the Size of a Vector
What is the size of a vector? In the context of C++, the size of a vector refers to the number of elements currently contained within it. This is fundamental when managing collections of data, allowing you to keep track of how many items you are working with in real-time. Understanding the size of a vector is essential for effective memory management and ensuring optimal performance in your applications.
The `size()` Method
To retrieve the size of a vector, you can use the built-in `size()` method. This method returns a value of type `size_t`, which is an unsigned integer type specifically designed to represent sizes.
The syntax for the `size()` method is straightforward:
vector<Type> myVector;
size_t vectorSize = myVector.size();
Here's an example illustrating how to use the `size()` method effectively:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Size of numbers vector: " << numbers.size() << std::endl;
return 0;
}
In this code, we create a vector called `numbers` and initialize it with five integers. When we call `numbers.size()`, it outputs `5`, showcasing the current number of elements in the vector.
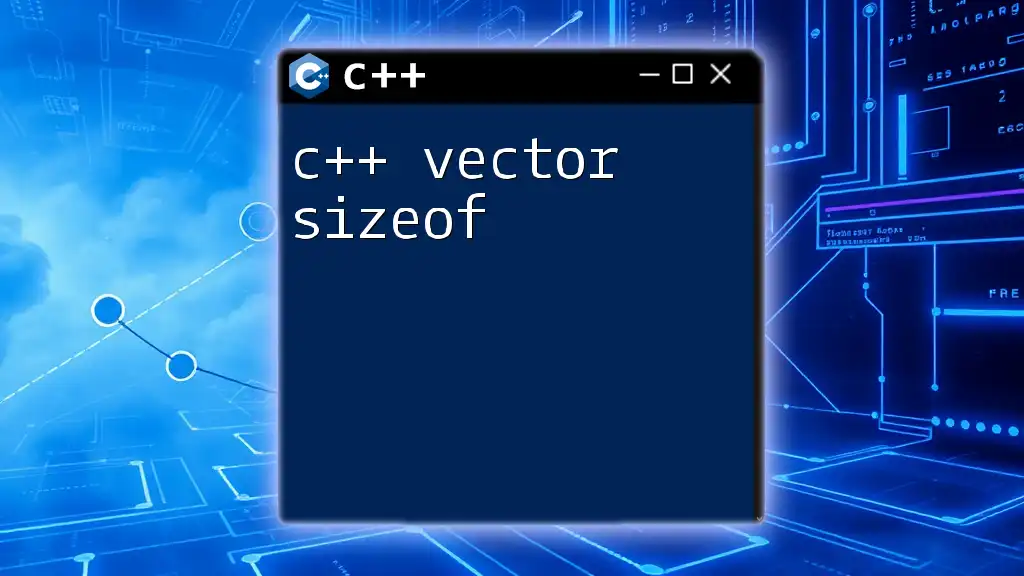
Manipulating Vector Size
Adding Elements to a Vector
When you need to increase the size of a vector, you can easily add elements using the `push_back()` function. This approach appends a new element to the end of the vector, automatically increasing its size. For instance:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6);
std::cout << "New size after adding an element: " << numbers.size() << std::endl;
return 0;
}
After adding the number `6`, the output confirms that the size has increased to `6`.
Removing Elements from a Vector
To decrease the size of a vector, you can remove elements using either the `pop_back()` method, which removes the last element, or the `erase()` method, which removes an element at a specified position.
Here’s an example using `pop_back()`:
numbers.pop_back();
std::cout << "Size after popping an element: " << numbers.size() << std::endl;
After calling `pop_back()`, the output would now indicate the vector size is `5` again.
For more specific removals, such as removing an element at a desired index, use `erase()`:
numbers.erase(numbers.begin());
std::cout << "Size after erasing the first element: " << numbers.size() << std::endl;
If the first element is removed, the vector’s size will decrease by one, reflecting that change.
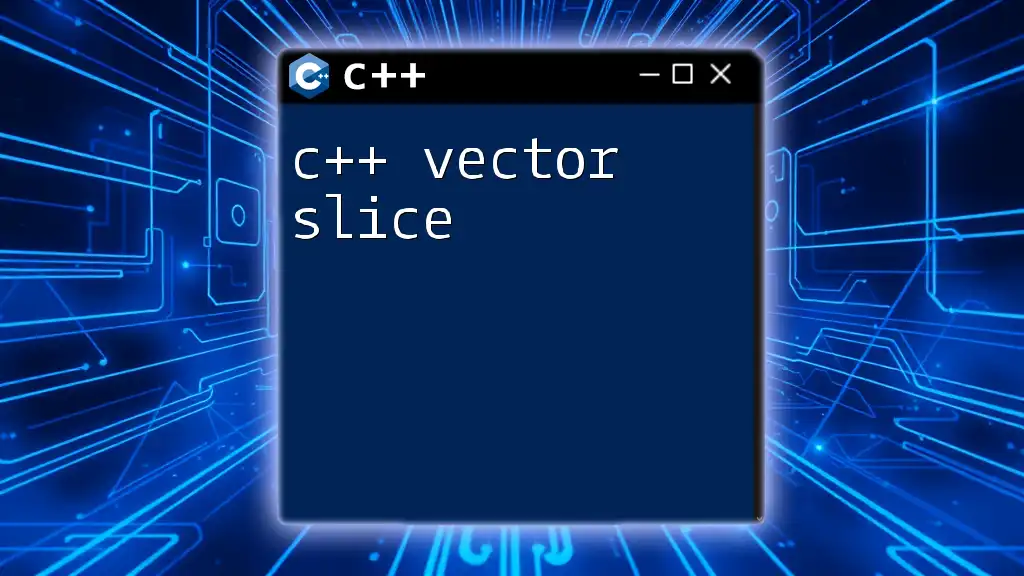
Capacity vs. Size
Understanding Capacity
It is crucial to understand that the capacity of a vector is not the same as its size. The size refers to the number of elements present, while the capacity denotes the total number of elements that the vector can hold before needing to resize.
Checking Capacity with `capacity()` Method
You can use the `capacity()` method to check the current capacity of the vector:
size_t vectorCapacity = myVector.capacity();
For example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Capacity of vector: " << numbers.capacity() << std::endl;
return 0;
}
This snippet prints out the capacity of the vector upon initialization. Initially, the capacity may be larger than the size, indicating that the vector can accommodate more elements without resizing.
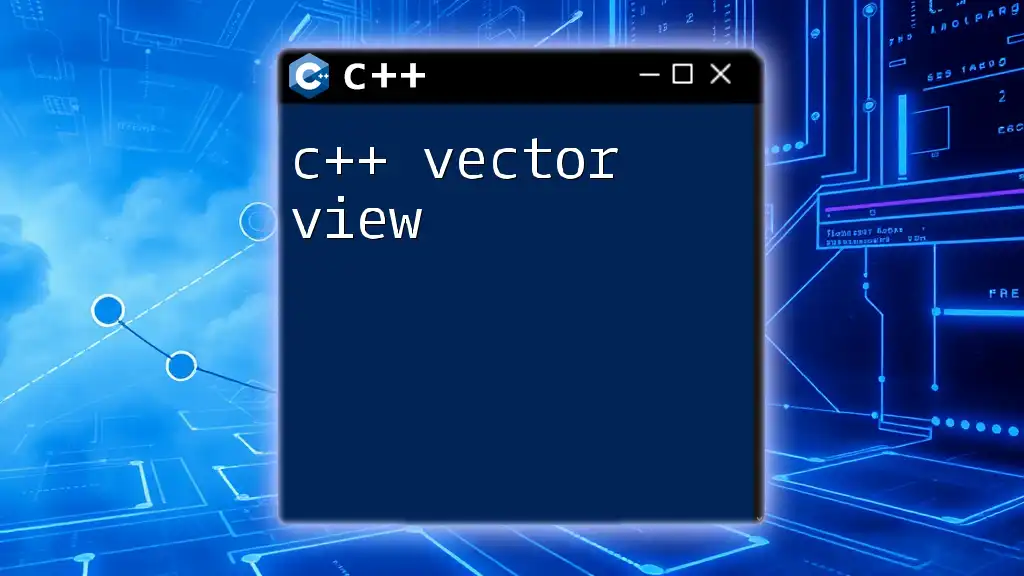
Vector Size and Memory Management
How Size Affects Memory Allocation
As you manipulate the vector by adding or removing elements, C++ handles memory allocation dynamically. When the size exceeds the capacity, C++ automatically allocates more memory to accommodate the new elements, which may involve allocating a new memory block and copying existing elements to it. This resizing process can lead to increased time complexity, primarily when done repeatedly.
Using `reserve()` to Manage Capacity
To optimize performance and manage memory better, you can pre-allocate space by using the `reserve()` method. This allows the programmer to specify the desired capacity, reducing the likelihood of costly reallocation during vector operations.
myVector.reserve(new_capacity);
For instance:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.reserve(10);
std::cout << "Capacity after reserving 10 spaces: " << numbers.capacity() << std::endl;
return 0;
}
Here, reserving space for ten elements ensures that when you fill the vector, it doesn’t need to resize as often, thereby improving performance.
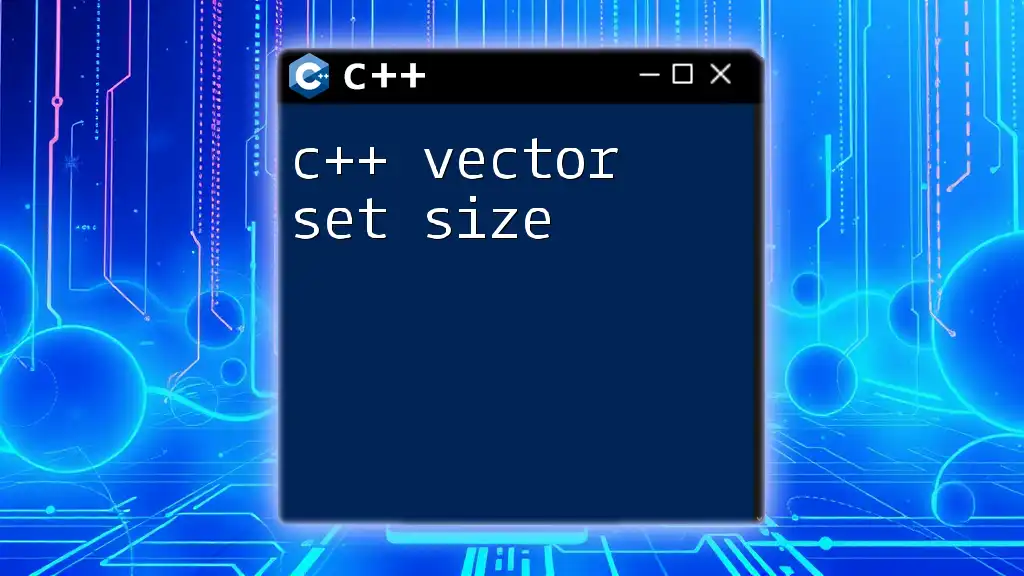
Real-World Applications
Understanding the concept of C++ vector size has practical implications in various scenarios. Whether you are developing complex algorithms, managing data efficiently, or ensuring real-time applications maintain performance, the effective use of vector size concepts can assist you in optimizing both memory usage and runtime efficiency.
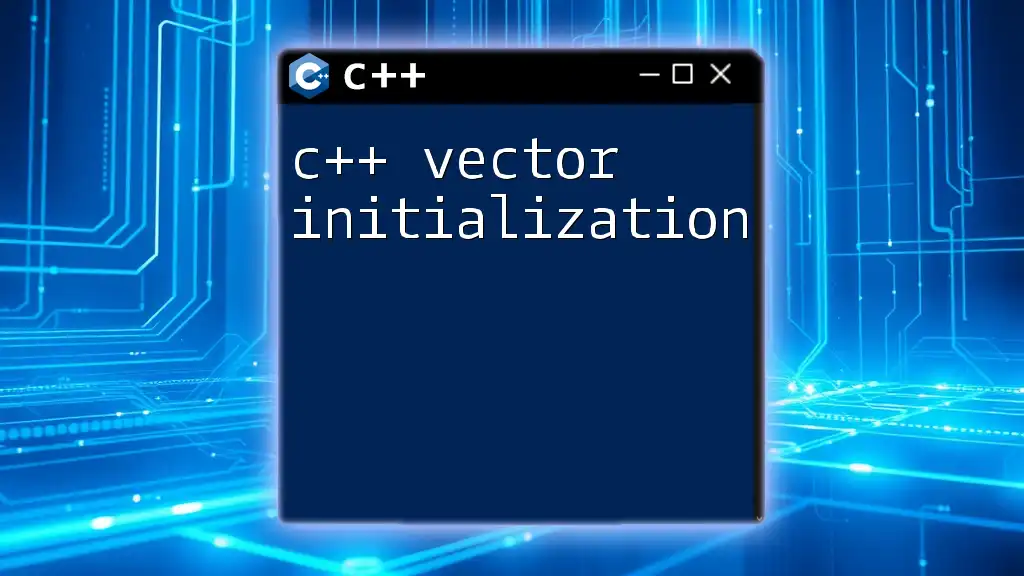
Best Practices for Working with Vector Size
To make the most of your vector implementations, consider these best practices:
- Pre-reserve Capacity: Always use `reserve()` when the number of elements is known in advance to prevent multiple reallocations.
- Use `size()` Wisely: Avoid unnecessary checks with `size()` in performance-critical code.
- Differentiate Size and Capacity: Be clear about the difference between size and capacity to avoid confusion in your code and to ensure effective memory handling.
Common Pitfalls
One common mistake is misunderstanding the relationship between size and capacity. Beginners often expect `size()` to modify a vector's size, but it merely returns the current number of elements. Additionally, forgetting to reserve capacity can lead to suboptimal performance due to multiple memory reallocations during vector resizing.
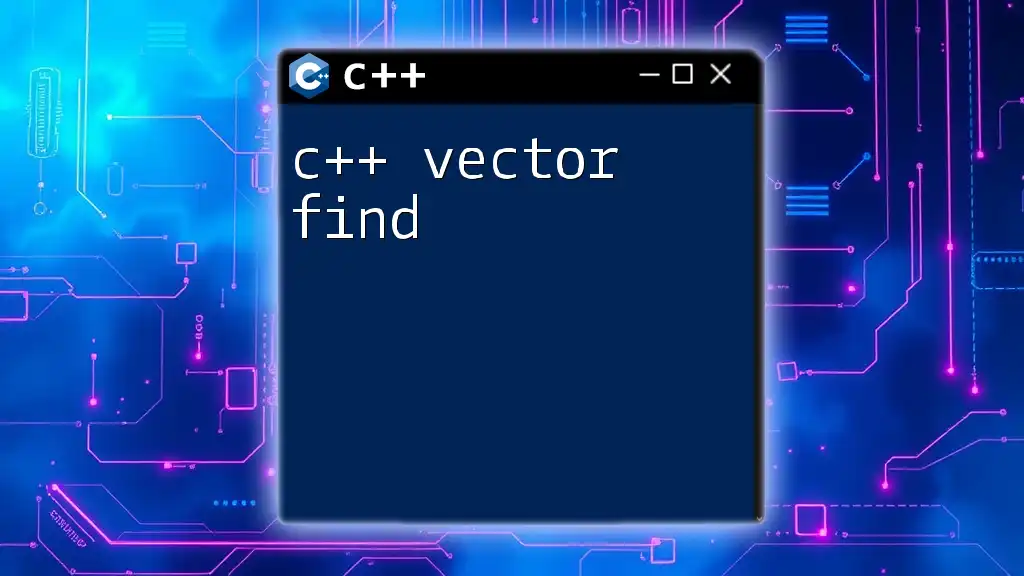
Conclusion
Understanding C++ vector size is crucial for anyone looking to effectively manage collections of data. By keeping track of the size, manipulating elements accurately, and recognizing the distinction between size and capacity, developers can write more efficient code that optimally utilizes memory resources while avoiding common pitfalls.
Remember, practice makes perfect. Apply these principles in your programming endeavors, and leverage C++ vectors for robust data management in your applications. For a deeper understanding, explore more features and tricks related to vectors, as they are an essential part of the C++ Standard Library that can greatly enhance your development skills. Happy coding!