C++ vectors are dynamic arrays that can grow and shrink in size, allowing for efficient data management and access through simple commands.
Here's a quick implementation example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adds 6 to the end of the vector
for (int i : numbers) {
std::cout << i << " "; // Outputs: 1 2 3 4 5 6
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can grow and shrink in size as needed. Unlike traditional arrays, which have a fixed size, vectors provide a flexible way to manage collections of data. They are part of the Standard Template Library (STL), which means they come with a variety of built-in functionalities that simplify data manipulation.
Advantages of Using Vectors
Vectors offer several benefits that make them an appealing choice for developers:
-
Dynamic Size Management: Vectors can increase or decrease their size dynamically. When you add or remove elements, the vector automatically reallocates memory, making it easier to handle varying amounts of data.
-
Automatic Memory Management: Vectors manage memory automatically. You don't need to manually allocate and deallocate memory like you do with raw arrays, which helps prevent memory leaks.
-
Ease of Use and Flexibility: With built-in methods for common operations such as adding, removing, and accessing elements, vectors allow for more straightforward coding.
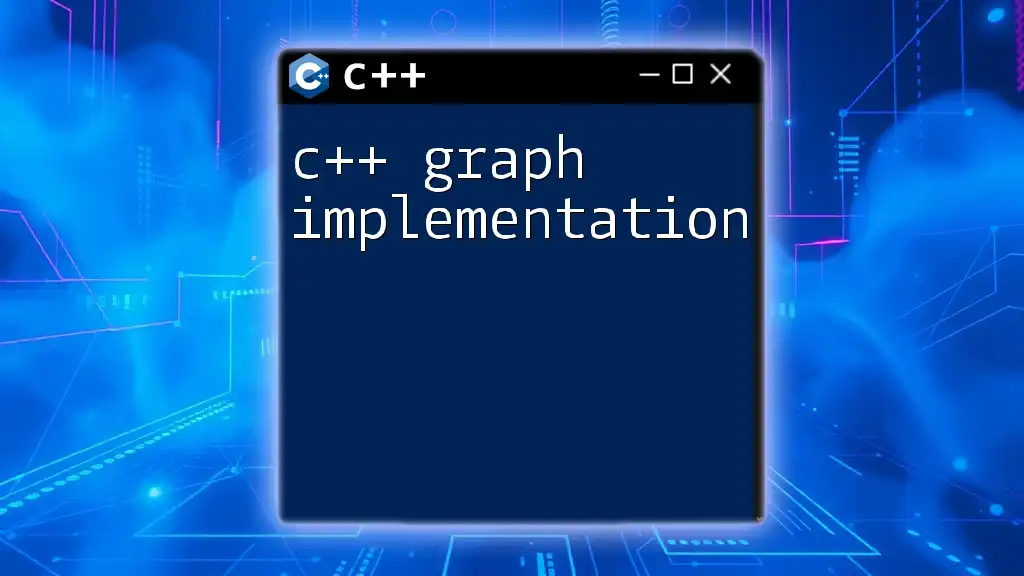
Getting Started with Vectors
Including the Relevant Header
Before you can use vectors, you need to include the vector library at the start of your program:
#include <vector>
This inclusion gives you access to all the functionalities related to vectors.
Declaring a Vector
Declaring a vector in C++ is simple. The basic syntax is:
std::vector<data_type> vector_name;
For example, you can declare vectors of different data types like so:
std::vector<int> intVector; // Vector of integers
std::vector<std::string> stringVector; // Vector of strings
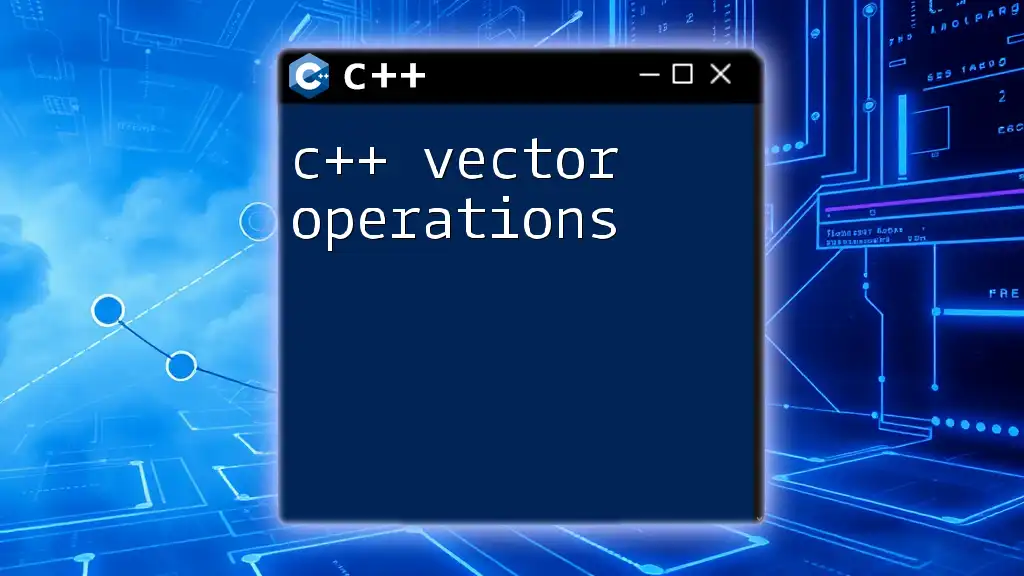
Basic Operations on Vectors
Adding Elements to a Vector
To add elements to a vector, you can use the `push_back()` method. This method appends an element to the end of the vector, expanding its size automatically. Here’s how you can do it:
intVector.push_back(10);
intVector.push_back(20);
After these operations, `intVector` will contain two elements: 10 and 20.
Accessing Vector Elements
You can access the elements of a vector using the `[]` operator or the `at()` method. The `at()` method is safer as it performs bounds checking, preventing out-of-bounds errors.
Here's how you can access vector elements:
int firstElement = intVector[0]; // Accessing with []
int secondElement = intVector.at(1); // Accessing with at()
Removing Elements
To remove elements from a vector, you can use `pop_back()` to remove the last element or `erase()` to remove an element at a specific position. Here are code snippets demonstrating both methods:
intVector.pop_back(); // Removes the last element
intVector.erase(intVector.begin()); // Removes the first element
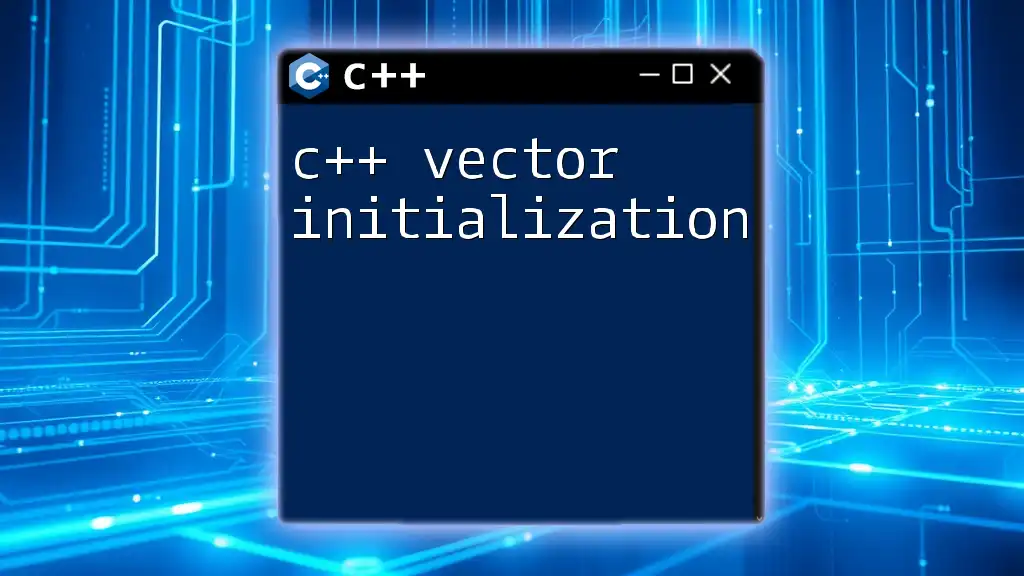
Advanced Vector Usage
Resizing and Reserving Space
Vectors can be resized to change their size dynamically. The `resize()` method changes the size, adding default values if increasing the size. The `reserve()` method, on the other hand, allocates memory in advance without changing the size, optimizing memory allocation when you know you’ll add a certain number of elements.
Here's how to use both:
intVector.resize(5); // Resizes the vector to contain 5 elements
intVector.reserve(10); // Reserves memory for 10 elements without resizing
Iterating Over a Vector
When working with vectors, iterating through the elements is vital. You can use traditional `for` loops or iterators. Here’s how:
Using a `for` loop:
for (size_t i = 0; i < intVector.size(); i++) {
std::cout << intVector[i] << " ";
}
Using iterators:
for (auto it = intVector.begin(); it != intVector.end(); ++it) {
std::cout << *it << " ";
}
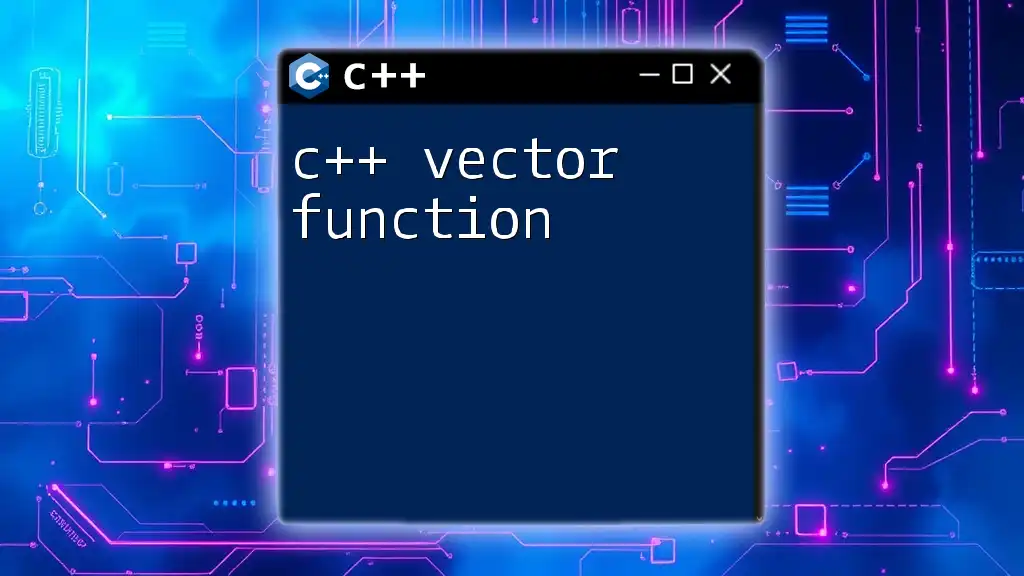
Vector Algorithms
Sorting Vectors
You can easily sort vectors using the `sort()` function from the `<algorithm>` header. Here's how to sort a vector in ascending order:
#include <algorithm>
std::sort(intVector.begin(), intVector.end());
After this operation, the elements in `intVector` will be sorted numerically.
Searching Within Vectors
To find elements within a vector, you can employ the `find()` function, which is also part of the `<algorithm>` header. This method returns an iterator to the element if found, or to the end of the vector if not found.
Here’s how to utilize it:
auto it = std::find(intVector.begin(), intVector.end(), 10);
if (it != intVector.end()) {
// Element found
}
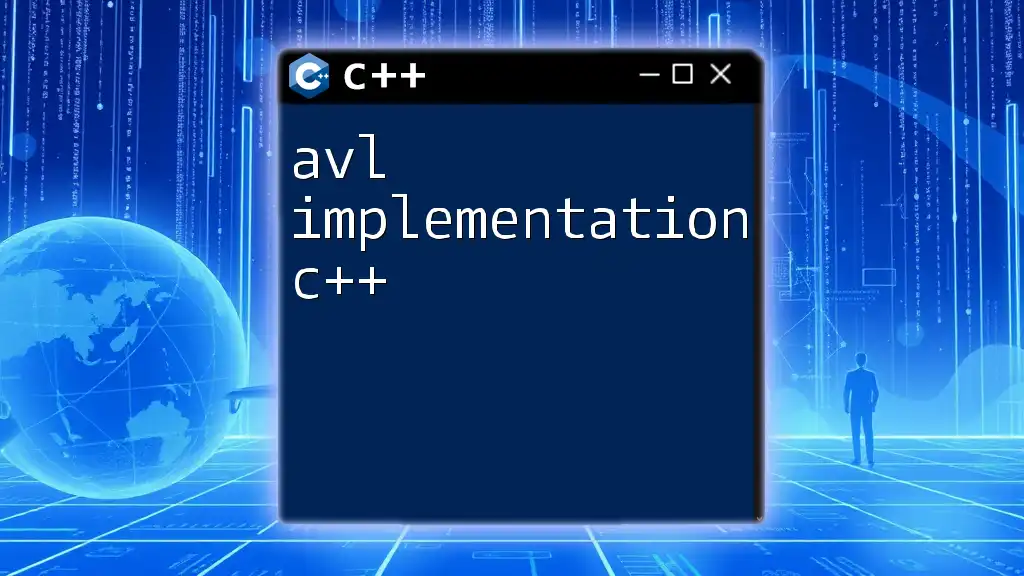
Common Pitfalls When Using Vectors
Out-of-Bounds Access
One of the most common pitfalls is accessing elements out of the vector's bounds. Using the `[]` operator for access does not check for bounds, potentially leading to undefined behavior. Always prefer using `at()` for safer access when you’re unsure about the bounds.
Memory Management
While vectors handle memory automatically, unnecessary copying of vectors can lead to performance issues. Use references or pointers when passing vectors to functions to avoid these pitfalls.
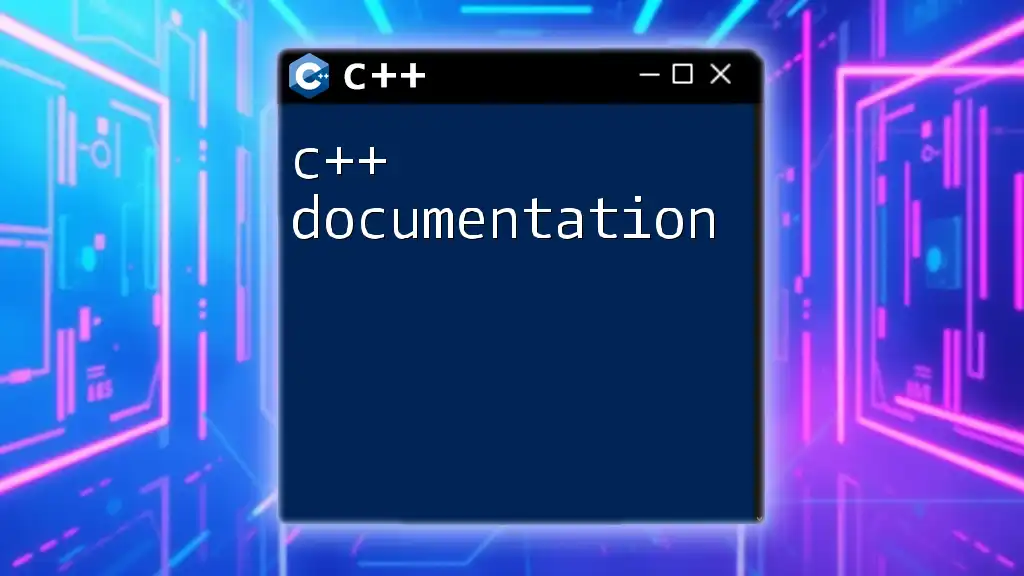
Conclusion
This comprehensive guide on C++ vector implementation has outlined the essential aspects of vectors, including their advantages, operations, advanced usage, and common pitfalls. Understanding and implementing vectors can significantly enhance your coding efficiency. Remember, practice makes perfect, so experiment with vectors in your own projects!
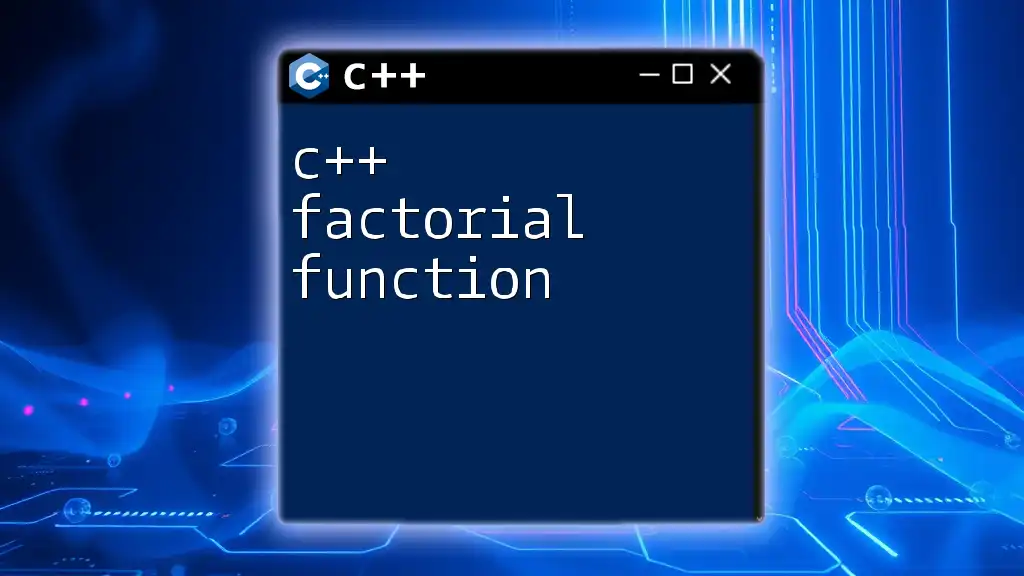
Additional Resources
- Check out the official C++ STL documentation for more in-depth knowledge of vectors and other STL components.
- Consider tackling some exercises that challenge your understanding of vector operations to reinforce your learning.