A queue implementation in C++ can be achieved using the Standard Template Library (STL) which provides a convenient way to manage a linear data structure that follows the First-In-First-Out (FIFO) principle.
Here's a simple code snippet to demonstrate how to implement a queue in C++:
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue; // Create a queue of integers
myQueue.push(10); // Add elements to the queue
myQueue.push(20);
myQueue.push(30);
std::cout << "Front element: " << myQueue.front() << std::endl; // Access the front element
myQueue.pop(); // Remove the front element
std::cout << "Front element after pop: " << myQueue.front() << std::endl; // Access the new front element
return 0;
}
Understanding Queues
What is a Queue?
A queue is a type of data structure that follows the FIFO (First In, First Out) principle. This means that the first element added to the queue will be the first one to be removed. Imagine a line at a grocery store: the person who arrives first is the first to be served.
Characteristics of a Queue:
- Enqueue: This operation adds an element to the rear of the queue.
- Dequeue: This operation removes an element from the front of the queue.
- Peek: This allows you to view the front element without removing it.
- isEmpty: This checks whether the queue is empty.
When you compare queues with other data structures, you find key differences. For example, stacks operate on a LIFO (Last In, First Out) basis, which means the last element added is the first one to be removed, making them suitable for different use cases than queues.
Types of Queues
- Linear Queue: A straightforward queue where addition and removal happen at opposite ends.
- Circular Queue: In a circular queue, the last position connects back to the first, allowing efficient use of space.
- Priority Queue: This queue allows elements to be dequeued based on priority rather than order, so higher priority items are processed first.
- Double-Ended Queue (Deque): A deque allows insertion and deletion of elements from both ends.

Implementing a Queue in C++
Why Use C++ for Queue Implementation?
C++ is particularly suited for implementing queues due to its efficiency and control over system resources. You can manage both static and dynamic memory allocation, offering flexibility for performance-sensitive applications. Additionally, C++ supports the Standard Template Library (STL), which provides built-in queue functionality.
Setting Up the Environment
To begin your queue implementation, make sure you have the right environment set up:
- Use any IDE that you are comfortable with (such as Visual Studio, Code::Blocks, or even an online compiler).
- Create a new C++ project and ensure you have the necessary libraries included.
Basic C++ Queue Implementation
Using Arrays
Let’s explore a basic queue implementation using arrays. This method implements static memory allocation, meaning the size of the queue must be defined at compile-time.
Here’s how you might implement it:
class Queue {
private:
int front, rear, size;
unsigned capacity;
int* array;
public:
Queue(unsigned capacity) {
this->capacity = capacity;
front = this->size = 0; // Initialize front and size
rear = capacity - 1; // Rear points to the last position
array = new int[this->capacity]; // Create the array
}
void enqueue(int item) {
if (isFull()) {
std::cout << "Queue is full\n";
return;
}
rear = (rear + 1) % capacity; // Circular increment
array[rear] = item; // Add item
size++;
std::cout << item << " enqueued to queue\n";
}
int dequeue() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return -1; // Indicate queue is empty
}
int item = array[front];
front = (front + 1) % capacity; // Circular increment
size--;
return item;
}
int peek() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return -1; // Indicate queue is empty
}
return array[front];
}
bool isEmpty() {
return size == 0;
}
bool isFull() {
return size == capacity;
}
};
The above code features an `enqueue` function to add items and a `dequeue` function to remove them, alongside essential functions for checking the status of the queue.
Using Linked Lists
For a more dynamic approach, you can implement a queue using linked lists, allowing flexible memory usage.
Here's a basic implementation using a linked list:
class Node {
public:
int data;
Node* next;
Node(int data) : data(data), next(nullptr) {}
};
class Queue {
private:
Node* front;
Node* rear;
public:
Queue() {
front = nullptr;
rear = nullptr;
}
void enqueue(int item) {
Node* newNode = new Node(item);
if (isEmpty()) {
front = rear = newNode; // Both front and rear point to the new node
return;
}
rear->next = newNode; // Attach new node to the end
rear = newNode; // Update rear to new node
}
int dequeue() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return -1; // Indicate queue is empty
}
int item = front->data; // Get data from front
Node* temp = front;
front = front->next; // Move front to next node
delete temp; // Free memory
if (front == nullptr) // If queue is empty, set rear to nullptr
rear = nullptr;
return item;
}
int peek() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return -1; // Indicate queue is empty
}
return front->data; // Return front node data
}
bool isEmpty() {
return front == nullptr; // Queue is empty if front is null
}
};
C++ Queue Implementation using the Standard Template Library (STL)
Using `std::queue`
The C++ Standard Template Library (STL) offers a built-in `queue` class, making it simple to implement queues without needing to handle the underlying mechanics manually.
Here’s an example of how to use `std::queue`:
#include <iostream>
#include <queue>
int main() {
std::queue<int> q;
q.push(10); // Enqueue element
q.push(20); // Enqueue another element
std::cout << "Front element: " << q.front() << std::endl; // Outputs 10
q.pop(); // Dequeue element
std::cout << "Front element after pop: " << q.front() << std::endl; // Outputs 20
return 0;
}
Using `std::queue`, you avoid the potential pitfalls of manual implementations and can focus on the functionality of your program.

Advanced Queue Implementations
Circular Queue Implementation
A circular queue improves the efficiency of a linear queue by connecting the end of the queue back to the front. This setup prevents wasted spaces that occur in a linear queue due to the static structure.
Advantages of Circular Queues
- Improved Space Utilization: Circular queues allow you to reuse space occupied by dequeued elements.
- Reduced Overhead: It eliminates the need to shift elements after a `dequeue` operation.
Priority Queue Implementation
A priority queue operates such that elements can be removed according to priority rather than the order they were added. For example, important tasks may be processed before less important ones.
You can implement a priority queue using the STL:
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int> pq;
pq.push(10);
pq.push(20);
pq.push(5);
std::cout << "Top priority element: " << pq.top() << std::endl; // Outputs 20
pq.pop();
std::cout << "Next top priority element: " << pq.top() << std::endl; // Outputs 10
return 0;
}
In the example above, elements are arranged in descending order automatically, so you always get the highest priority item when calling `top()`.

Common Challenges and Troubleshooting
Debugging Common Issues in C++ Queue Implementation
When implementing queues, certain issues may arise. One common problem is memory leaks in linked list implementations if nodes are not properly deleted. Always ensure that dynamically allocated memory is freed after use.
Another issue to consider is overflow and underflow conditions, especially in static array implementations. The `isFull()` and `isEmpty()` functions help prevent accessing elements that do not exist.
Performance Considerations
- Time Complexity of Queue Operations:
- Enqueue and dequeue operations typically take O(1) time with both array and linked list implementations.
- Space Complexity Considerations:
- Space complexity is O(n) for both implementations, where n is the number of elements in the queue.
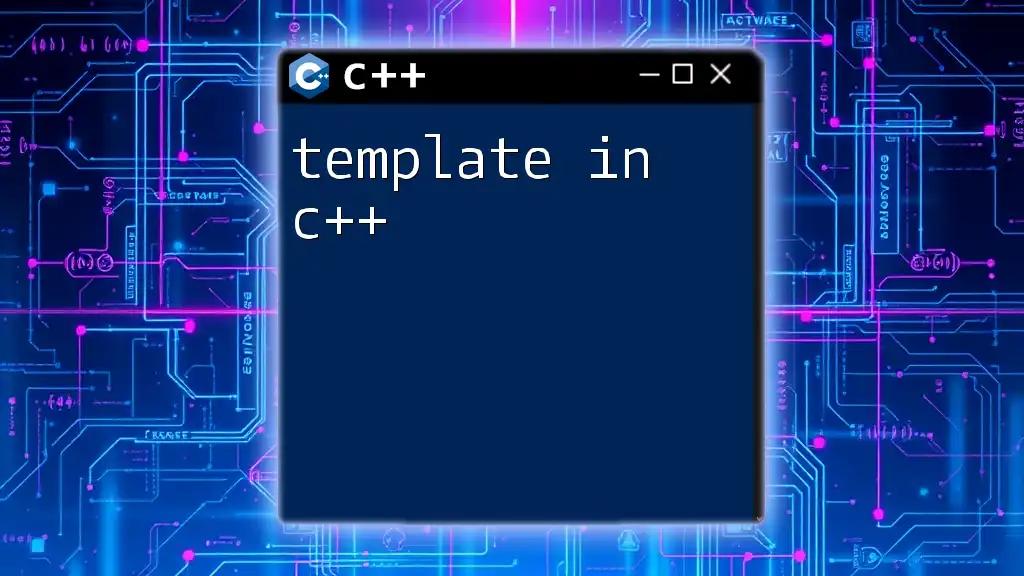
Conclusion
This comprehensive guide to queue implementation in C++ has provided you with the definitions, operations, and various implementation methods of queues. You should now be equipped with the foundational knowledge to leverage queues effectively in your programming endeavors.
As you continue your learning journey, consider experimenting with more advanced concepts and applications of queues to deepen your understanding. Happy coding!

Call to Action
If you're eager to put your knowledge to the test, try implementing different queue types based on the concepts discussed. Explore various projects that involve queue operations and enhance your C++ skills!