Aggregation in C++ is a relationship where a class can contain references or pointers to objects of another class, representing a "has-a" relationship, typically used to model complex entities composed of simpler ones.
Here's a simple example of aggregation in C++:
#include <iostream>
#include <string>
class Engine {
public:
Engine(std::string type) : type(type) {}
void displayType() { std::cout << "Engine type: " << type << std::endl; }
private:
std::string type;
};
class Car {
public:
Car(std::string model, Engine* engine) : model(model), engine(engine) {}
void display() {
std::cout << "Car model: " << model << std::endl;
engine->displayType();
}
private:
std::string model;
Engine* engine; // Aggregation relationship
};
int main() {
Engine engine("V8");
Car car("Mustang", &engine);
car.display();
return 0;
}
Understanding Aggregation
What is Aggregation?
Aggregation in C++ is a fundamental concept in object-oriented programming that defines a specific type of relationship between classes. It is characterized by a "has-a" relationship, where one class (the container) contains references or objects of another class (the contained) but does not control its lifecycle. This is different from composition, where the lifecycle of the contained objects is strictly tied to the container.
For example, if a `School` class contains multiple `Student` objects, we say that the school aggregates its students. However, if a student leaves the school, the `Student` object can still exist elsewhere in the program, demonstrating the independent lifespan of aggregated objects.
Why Use Aggregation?
The benefits of aggregation are numerous:
- Code Organization: It allows for better structuring of your codebase by encapsulating related objects.
- Reusability: Classes that serve as aggregates can be reused in different contexts without modification.
- Separation of Concerns: Each class can focus on its functionality, promoting cleaner designs and easier testing.
In real-world applications, aggregation is often evident in scenarios such as schools containing students, libraries holding books, or even teams having players.
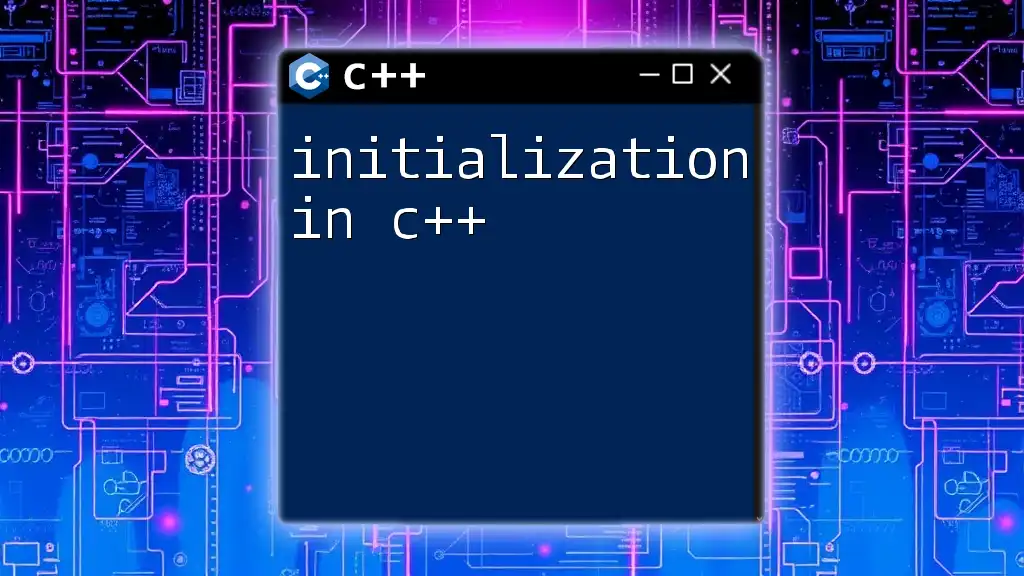
Implementing Aggregation in C++
Creating Classes with Aggregation
To illustrate how to implement aggregation in C++, let's consider a simple example of a `School` class that aggregates `Student` objects. Below is how we can define these classes:
class Student {
public:
std::string name;
int age;
Student(std::string n, int a) : name(n), age(a) {}
};
class School {
private:
std::vector<Student> students; // Aggregation relationship
public:
void addStudent(const Student& student) {
students.push_back(student);
}
};
In this example, the `School` class contains a vector of `Student` objects, demonstrating aggregation. The `addStudent` member function allows the addition of `Student` instances into the `students` vector.
Accessing Aggregated Objects
Once we have our aggregated objects in place, it's crucial to understand how to access and manipulate them. Let's consider a function to print the details of all students in the school:
void printStudents(const School& school) {
for (const auto& student : school.students) {
std::cout << "Name: " << student.name << ", Age: " << student.age << std::endl;
}
}
This function takes a `School` object as a parameter and iterates over the `students` vector, printing each student's name and age.
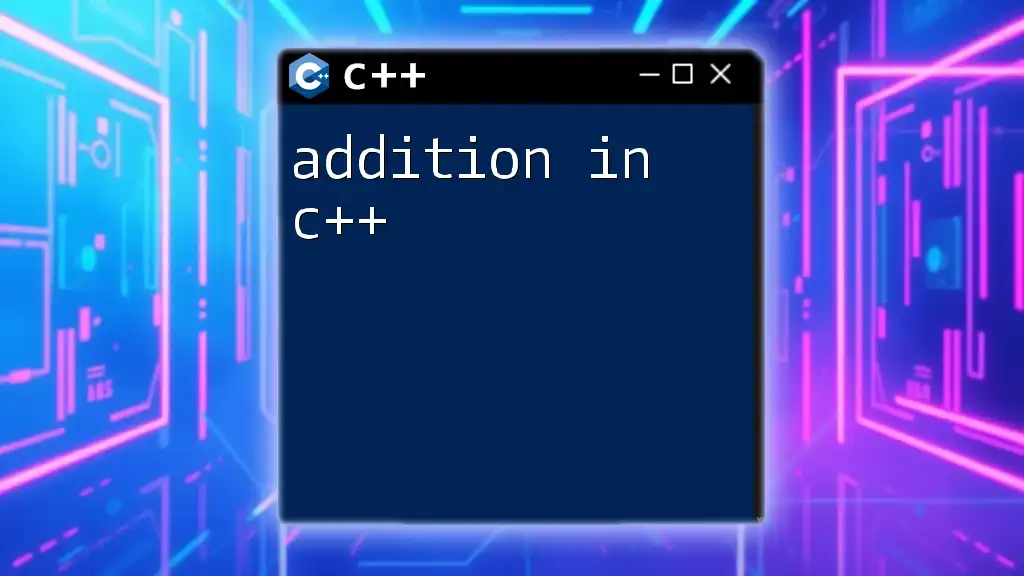
Key Characteristics of Aggregation
Lifespan of Aggregated Objects
One of the key characteristics of aggregation is how it affects the lifespan of objects. In aggregation, the lifecycle of the aggregated objects is independent of the container. This means that even if the `School` class is destroyed, the `Student` objects can continue to exist elsewhere in the program if they are maintained by other entities.
Flexibility and Reusability
Aggregation promotes greater flexibility and reusability in code design. For instance, you could easily modify the `Student` class without needing to alter the `School` class. Additionally, if you develop another class that needs a collection of `Student` objects, you can reuse the existing `Student` class, simplifying development and saving time.
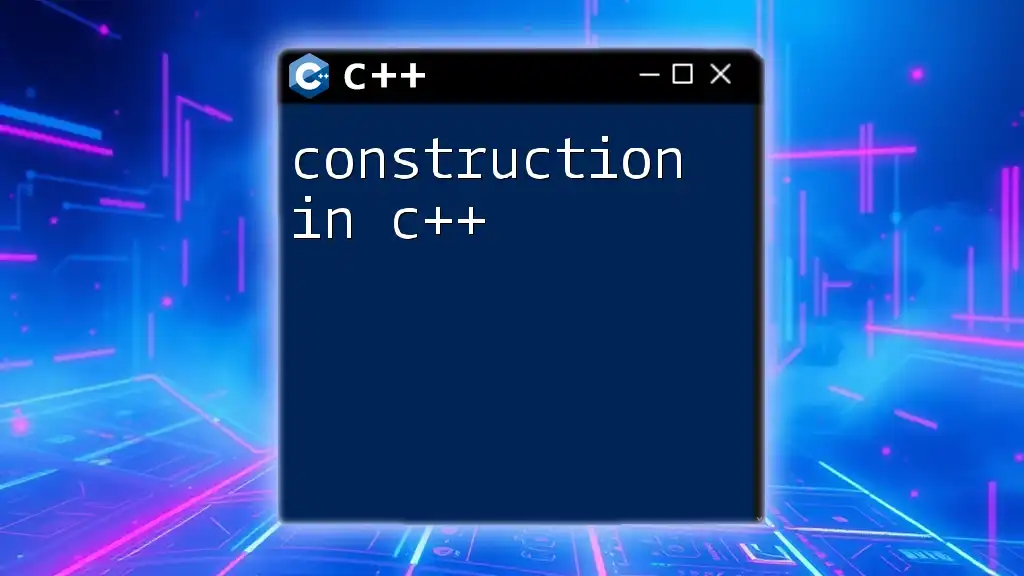
Common Use Cases of Aggregation
Real-World Examples
To further understand aggregation in C++, let's consider another real-world scenario with a `Library` class, which aggregates `Book` objects:
class Book {
public:
std::string title;
std::string author;
Book(std::string t, std::string a) : title(t), author(a) {}
};
class Library {
private:
std::vector<Book> books; // Aggregation relationship
public:
void addBook(const Book& book) {
books.push_back(book);
}
};
In this example, the `Library` contains multiple `Book` objects. This demonstrates how aggregation is utilized to represent real-world relationships effectively.
Best Practices
When implementing aggregation in C++, consider the following best practices:
- Modular Design: Keep aggregated objects in separate files or modules to promote cleaner designs.
- Smart Pointers: Utilize smart pointers (e.g. `std::shared_ptr` or `std::unique_ptr`) for managing the memory of aggregated objects. This helps avoid memory leaks and ensures proper resource management.
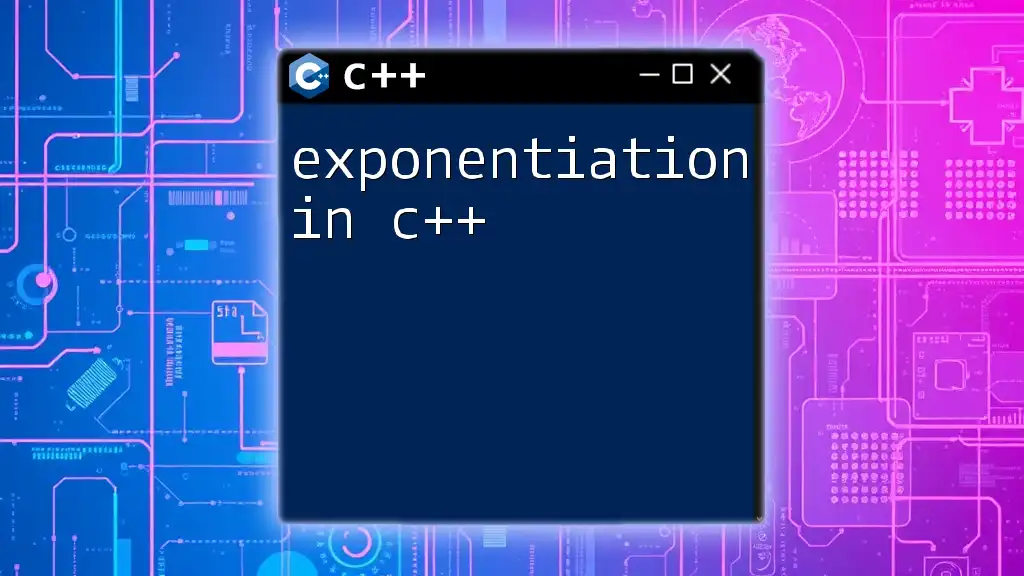
Challenges and Limitations of Aggregation
Common Pitfalls
When working with aggregation, developers might encounter certain common pitfalls:
- Unintended Lifespan Sharing: It's important to ensure that the objects being aggregated are managed correctly so that they do not unintentionally share lifespans if that is not the intention.
- Overcomplicating Models: Sometimes, developers use aggregation where composition would be more appropriate, leading to over-complicated class designs.
Performance Considerations
While using aggregation can be beneficial, it is essential to consider performance. Performance issues may arise, particularly with larger datasets, since aggregating objects often results in additional overhead when passing and managing object references. Comparing the memory consumption and processing time of aggregation versus composition can provide insights into which model better suits your application’s needs.
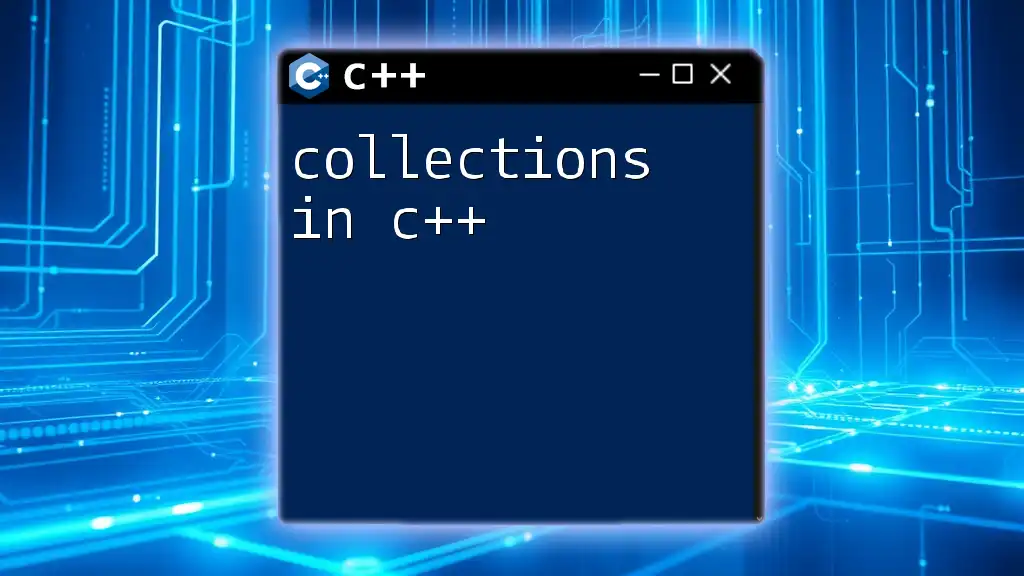
Conclusion
In summary, understanding aggregation in C++ is crucial for writing organized and reusable code. By mastering this concept, developers can create more flexible designs and encapsulate relationships between objects effectively. Whether implementing relationships in simple programs or complex systems, knowing when and how to use aggregation will enhance your programming capabilities and improve code quality significantly.
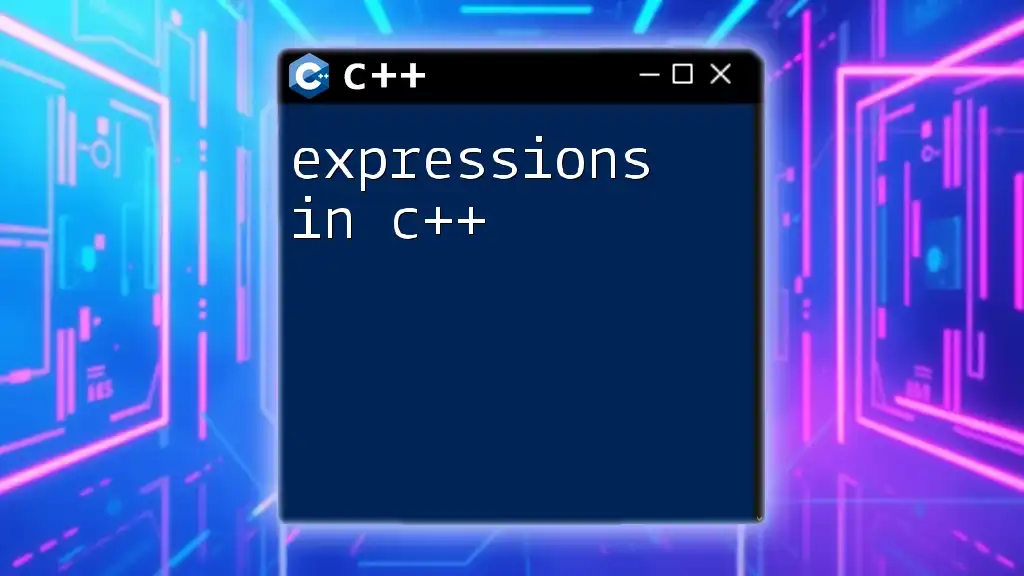
Frequently Asked Questions (FAQ)
What is the difference between aggregation and composition in C++?
The primary difference between aggregation and composition lies in the lifespan of the dependent objects. In aggregation, the contained objects can exist independently of the containing object. In contrast, in composition, the contained objects’ lifecycle is bound to the lifecycle of the containing object, meaning if the container is destroyed, so are the contained objects.
Can aggregation be implemented using pointers?
Yes, aggregation can utilize pointers. For example, a `School` class can maintain a collection of students via pointers, allowing for dynamic memory management. Here’s a simple illustration:
class School {
private:
std::vector<Student*> students; // Using pointers for aggregation
public:
void addStudent(Student* student) {
students.push_back(student);
}
};
This allows `Student` objects to exist independently and can be managed more flexibly.
When should I use aggregation over inheritance?
Aggregation should be used over inheritance when the relationship between classes is best described as "has-a" rather than "is-a." If you have classes that are more compositional in nature, where one class can contain multiple instances of another without establishing a strict subclass-superclass relationship, aggregation is the preferable choice.