In C++, you can calculate the tangent of an angle in radians using the `tan()` function from the `<cmath>` library.
#include <iostream>
#include <cmath>
int main() {
double angleInRadians = 1.0; // Example angle
double tangentValue = tan(angleInRadians);
std::cout << "The tangent of the angle is: " << tangentValue << std::endl;
return 0;
}
Understanding Tangent in Mathematics
Tangent is a fundamental concept in trigonometry, defined as the ratio of the length of the opposite side to the length of the adjacent side in a right triangle. In mathematical terms, if we have an angle \( \theta \), then:
\[ \tan(\theta) = \frac{\text{opposite}}{\text{adjacent}} \]
The tangent function plays a critical role in various fields, including physics and engineering, making it essential for programmers to understand how to work with it in their applications.
Tangent Function Properties
Key properties of the tangent function include its periodic nature with a period of \( \pi \) radians (or 180 degrees) and a range of all real numbers. Moreover, the function is undefined at odd multiples of \( \frac{\pi}{2} \) (90 degrees), creating vertical asymptotes in its graph.
Understanding these properties enables developers to utilize the tangent function in practical applications, from physics simulations to computer graphics.

Utilizing the Tangent Function in C++
To work with the tangent function in C++, we'll primarily use the `<cmath>` library, which provides a multitude of mathematical functions, including tangent.
Introduction to the `<cmath>` Library
The `<cmath>` library is essential for mathematical operations in C++. By including this library, you can access various mathematical functions such as sine, cosine, and, of course, tangent.
Here's how you include it in your program:
#include <cmath>
Calculating Tangent in C++
The syntax for calculating tangent using the `<cmath>` library is straightforward:
double tan(double angle);
Note: The input angle must be in radians. To convert degrees to radians, remember the conversion formula:
\[ \text{radians} = \text{degrees} \times \frac{\pi}{180} \]
Example: Calculating Tangent of 45 Degrees
The tangent of 45 degrees is known to be 1. Let's see how to calculate it in C++:
#include <iostream>
#include <cmath>
int main() {
double angleDegrees = 45.0;
double angleRadians = angleDegrees * M_PI / 180.0; // Convert to radians
double tangentValue = tan(angleRadians);
std::cout << "Tangent of " << angleDegrees << " degrees is: " << tangentValue << std::endl;
return 0;
}
Output:
Tangent of 45 degrees is: 1

Converting Degrees to Radians
Why Convert?
Radians are the standard unit of angular measure used in mathematics. Understanding how to convert degrees to radians is critical when using C++ for mathematical calculations.
Code Example for Conversion
A simple function can be created to handle this conversion:
double degreesToRadians(double degrees) {
return degrees * M_PI / 180.0;
}
Comprehensive Example Using Conversion Function
Here’s an overarching example that showcases the conversion and calculates the tangent:
#include <iostream>
#include <cmath>
double degreesToRadians(double degrees) {
return degrees * M_PI / 180.0;
}
int main() {
double angleDegrees = 30.0;
double tangentValue = tan(degreesToRadians(angleDegrees));
std::cout << "Tangent of " << angleDegrees << " degrees is: " << tangentValue << std::endl;
return 0;
}
Output:
Tangent of 30 degrees is: 0.57735
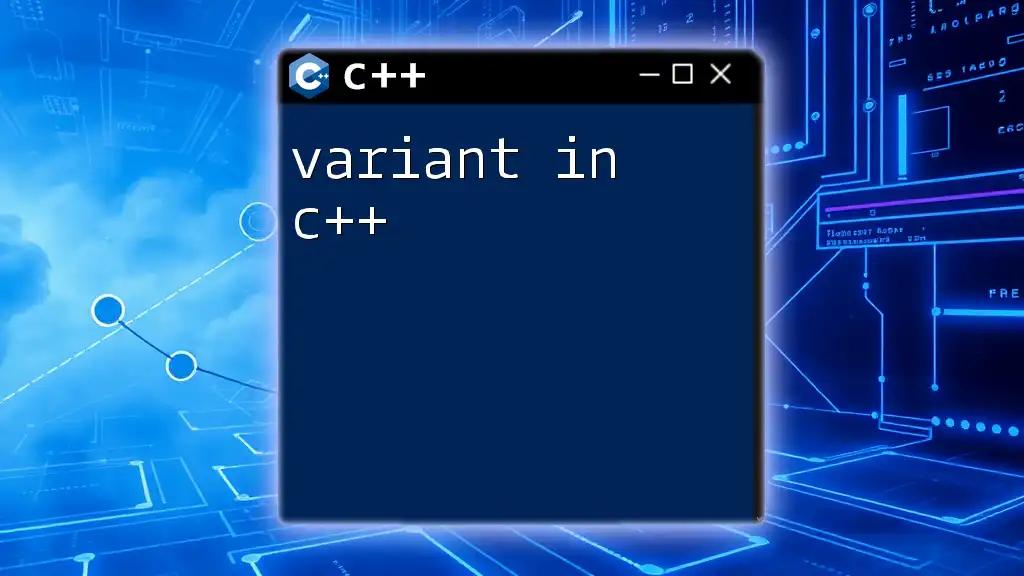
Handling Special Cases
Limits of the Tangent Function
The tangent function is undefined for angles where the cosine is zero; specifically, this occurs at odd multiples of \( \frac{\pi}{2} \) (90 degrees, 270 degrees, etc.). When programming, it’s crucial to anticipate these edge cases to avoid runtime errors.
Error Handling in C++
Here's how you can ensure that your program gracefully handles invalid inputs:
double safeTangent(double angle) {
double radians = degreesToRadians(angle);
if (tan(radians) == std::numeric_limits<double>::infinity()) {
throw std::domain_error("Tangent is undefined for this angle.");
}
return tan(radians);
}
This `safeTangent` function checks if the tangent calculation would result in an undefined value and throws an exception accordingly.

Practical Application of Tangent in C++
Example: Building a Simple Calculator
One practical application of the tangent function is developing a basic calculator. Below is an example of how to incorporate tangent functionality into a simple console application:
#include <iostream>
#include <cmath>
#include <string>
void showMenu() {
std::cout << "Choose an operation:\n";
std::cout << "1. Calculate Tangent\n";
std::cout << "2. Exit\n";
}
int main() {
int choice;
double angle;
while (true) {
showMenu();
std::cin >> choice;
if (choice == 2) break;
std::cout << "Enter angle in degrees: ";
std::cin >> angle;
try {
double result = safeTangent(angle);
std::cout << "Tangent of " << angle << " is " << result << "\n";
} catch (const std::domain_error &e) {
std::cerr << e.what() << "\n";
}
}
return 0;
}
This program will allow users to compute the tangent of different angles, handling errors for undefined cases effectively.

Conclusion
Understanding how to calculate and use the tangent in C++ is vital for any programmer engaging with mathematical computations. From converting angles to managing edge cases, this guide has covered essential components necessary for accurate tangent calculations. Be sure to practice implementing tangent in your own projects and continue exploring other trigonometric functions to enhance your programming skills further.

Further Resources
Documentation and References
To deepen your understanding, refer to:
- The official C++ reference documentation for the `<cmath>` library.
- Recommended C++ programming books that cover mathematical functions.
- Online coding platforms that provide practice problems on trigonometric functions.
By leveraging these resources, you can continue to build upon your knowledge of using the tangent function in C++.