"Hangman in C++ is a simple word-guessing game where the player tries to guess a hidden word one letter at a time, and here's a basic implementation:"
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string word = "hangman";
std::string guessed(word.size(), '_');
std::vector<char> wrongLetters;
int tries = 6;
while(tries > 0 && guessed != word) {
std::cout << "Current word: " << guessed << "\n";
std::cout << "Wrong letters: ";
for(char c : wrongLetters) std::cout << c << " ";
std::cout << "\nTries left: " << tries << "\n";
char guess;
std::cout << "Enter a letter: ";
std::cin >> guess;
if(word.find(guess) != std::string::npos) {
for(size_t i = 0; i < word.size(); i++) {
if(word[i] == guess) guessed[i] = guess;
}
} else {
wrongLetters.push_back(guess);
tries--;
}
}
if(guessed == word) std::cout << "Congratulations! You guessed the word: " << word << "\n";
else std::cout << "Game over! The word was: " << word << "\n";
return 0;
}
What is Hangman?
Hangman is a word-guessing game where players attempt to guess a hidden word within a limited number of incorrect attempts. The game starts by presenting dashes representing each letter in the word. Players then suggest letters one at a time. If the guessed letter is in the word, it is revealed in its correct position(s). If it is not, a part of the "hangman" figure is drawn. The objective is to correctly guess the word before the drawing of the hangman completes, typically featuring a noose, head, body, arms, and legs.
Learning C++ through the implementation of the Hangman game not only reinforces fundamental programming concepts but also transforms an abstract learning experience into something interactive and engaging. As you navigate through this tutorial, you'll tackle essential programming tasks such as using loops, conditionals, and user input.
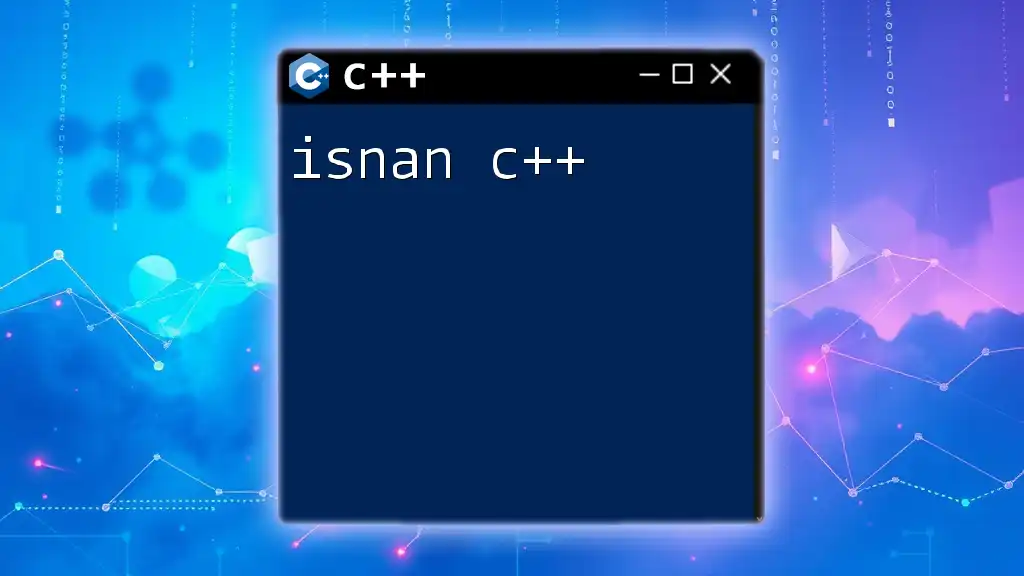
Setting Up Your C++ Environment
To start developing your game, you'll first need to set up a suitable C++ development environment. This involves selecting an Integrated Development Environment (IDE) and a compiler.
Required Software
For this task, consider using popular IDEs such as Visual Studio or Code::Blocks. These tools provide an excellent platform for C++ programming and debugging.
To install your chosen IDE:
- Download the installer from the official website.
- Run the installer and follow the prompts.
- Make sure to install the C++ extensions if prompted, as these are necessary for compiling and debugging your C++ code.
Creating Your First C++ Project
Once your development environment is ready, the next step is to create a new C++ project. This process may vary slightly but generally follows these steps:
- Open your IDE and select "New Project".
- Choose your project type (C++ project) and name your project based on your preference (e.g., Hangman).
- Set your project's location for easy access later.
A well-structured project enhances readability and maintenance, so it’s vital to organize code files properly.
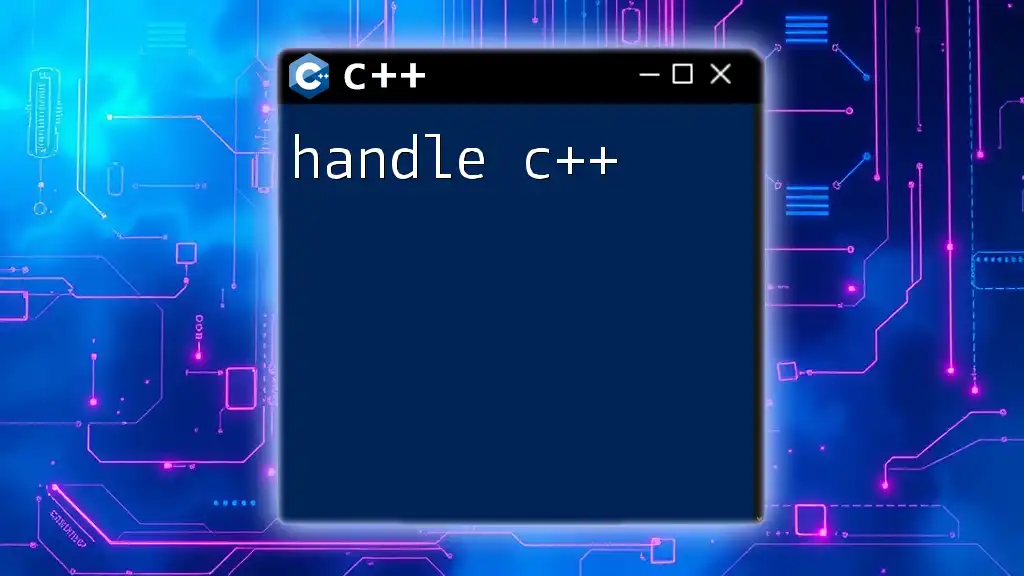
Building the Hangman Game in C++
Planning Your Game Structure
Before diving into the code, it’s beneficial to plan out the key components of your Hangman game. Identify essential elements such as:
- Displaying the word's progress
- Tracking the number of wrong guesses
- Allowing the player to make guesses
- End-game conditions for both wins and losses
Starting with the Main Function
The foundation of any C++ application is the main function. It acts as the entry point of your program, where the execution begins. Here’s a basic structure of the main function:
#include <iostream>
int main() {
// Game Logic will go here
return 0;
}
This code initializes your program and concludes with a return statement, which indicates successful program completion.
Creating Word List
Choosing Words for the Game
Choosing engaging words is crucial for maintaining player interest. You can either curate your own list, find public domain word lists online, or utilize existing libraries.
A simple sample word list might include:
- programming
- hangman
- cplusplus
Implementing the Word List in Code
To store these words in C++, you can use a `vector` or an `array`. Here's a code snippet demonstrating how to include the word list as a `vector`:
#include <vector>
#include <string>
std::vector<std::string> words = {"programming", "hangman", "cplusplus"};
This snippet sets up a vector that can be dynamically adjusted and accessed when needed.
Displaying the Hangman Board
Drawing the Hangman
To add an engaging visual component, you'll want to represent the hangman at various stages. This involves defining a function that will display different parts of the hangman based on the number of incorrect guesses. Here’s how you might define such a function:
void displayHangman(int wrongGuesses) {
switch (wrongGuesses) {
case 0: std::cout << " | \n"; break; // No parts drawn
case 1: std::cout << " O \n"; break; // Head
case 2: std::cout << " O \n | \n"; break; // Head and body
// Add more cases for arms and legs
}
}
Showing Progress to the Player
A key aspect of keeping the player engaged is showing their progress. You can create a string that represents the current state of the hidden word. Utilize a loop to replace dashes with correctly guessed letters:
std::string currentProgress = hiddenWord;
for (char letter : guessedLetters) {
size_t index = hiddenWord.find(letter);
while (index != std::string::npos) {
currentProgress[index] = letter;
index = hiddenWord.find(letter, index + 1);
}
}
This code helps to update the displayed state of the word dynamically based on player guesses.
Handling Player Input
Taking User Guesses
Capturing user input is critical for gameplay. You can employ a simple method to receive guesses while ensuring that inputs are valid:
char guess;
std::cin >> guess;
// Validate input TODO: Ensure it's only one letter and hasn't been guessed before.
Add logic here for input validation to prevent errors.
Checking Guesses Against the Word
Now that you have the player’s guess, check if it exists in the hidden word. Use the following snippet to determine if the guess is correct:
if (hiddenWord.find(guess) != std::string::npos) {
// Correct guess logic
}
This snippet allows you to seamlessly update gameplay based on the player’s input.
Game Loop Functionality
Implementing the Game Loop
Your game will operate inside a loop until either the player wins or exhausts all attempts. This can be done using a `while` loop:
bool gameIsActive = true;
while (gameIsActive) {
// Display hangman
displayHangman(wrongGuesses);
// Show progress
std::cout << currentProgress << std::endl;
// Take user input and check game status as needed
}
This loop keeps the game active, providing updates on the game status until a winning or losing condition occurs.
End of Game Conditions
Define clear conditions that dictate when the game ends, such as the player winning or exceeding the maximum number of wrong guesses. This logic should reside within your game loop to check the game status at each iteration:
if (currentProgress == hiddenWord) {
std::cout << "Congratulations! You've won!" << std::endl;
gameIsActive = false;
} else if (wrongGuesses >= MAX_WRONG_GUESSES) {
std::cout << "Sorry, you've lost! The word was: " << hiddenWord << std::endl;
gameIsActive = false;
}
This code snippet includes straightforward conditional checks that allow for smooth transitions between game states.
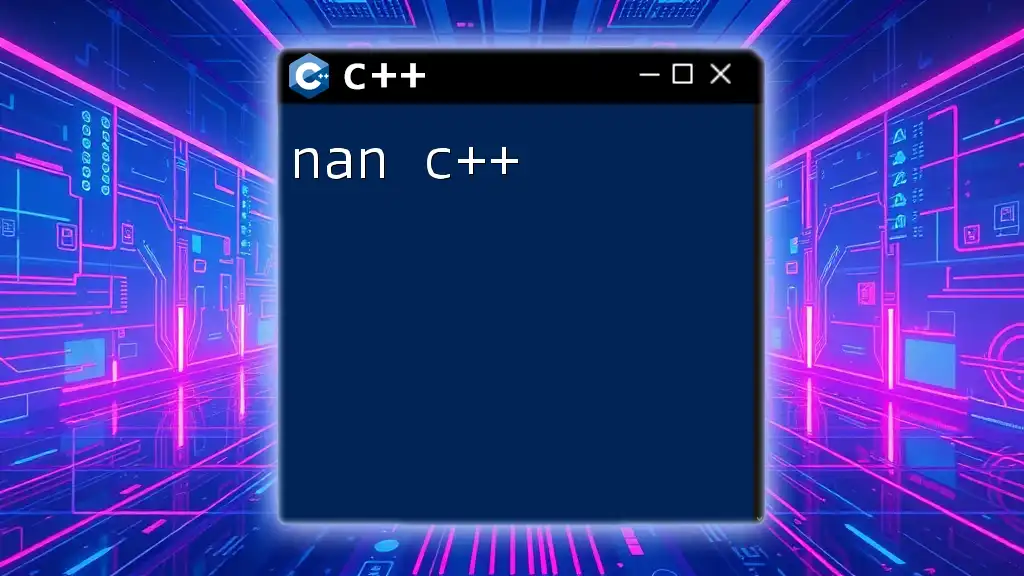
Enhancing the Game Experience
Adding Difficulty Levels
To keep players engaged, consider implementing varying difficulty levels. This could involve adjusting the number of wrong guesses allowed or the length of the words. For instance, you can prompt players to select a difficulty level at the start:
int maxGuesses;
std::cout << "Choose difficulty: 1 (Easy), 2 (Medium), 3 (Hard)\n";
std::cin >> difficultyLevel;
switch (difficultyLevel) {
case 1: maxGuesses = 10; break; // Easy
case 2: maxGuesses = 7; break; // Medium
case 3: maxGuesses = 5; break; // Hard
}
Such a feature increases replayability and personalizes the gaming experience according to the player’s skill level.
Implementing a Replay Feature
At the end of the game, prompt the player if they would like to play again and reset the game’s variables accordingly. This improves user engagement and encourages longer play sessions:
char playAgain;
std::cout << "Do you want to play again? (y/n): ";
std::cin >> playAgain;
if (playAgain == 'y' || playAgain == 'Y') {
// Reset game variables for a new game
}
This simple yet effective feature can enhance user satisfaction by offering a seamless transition between game sessions.
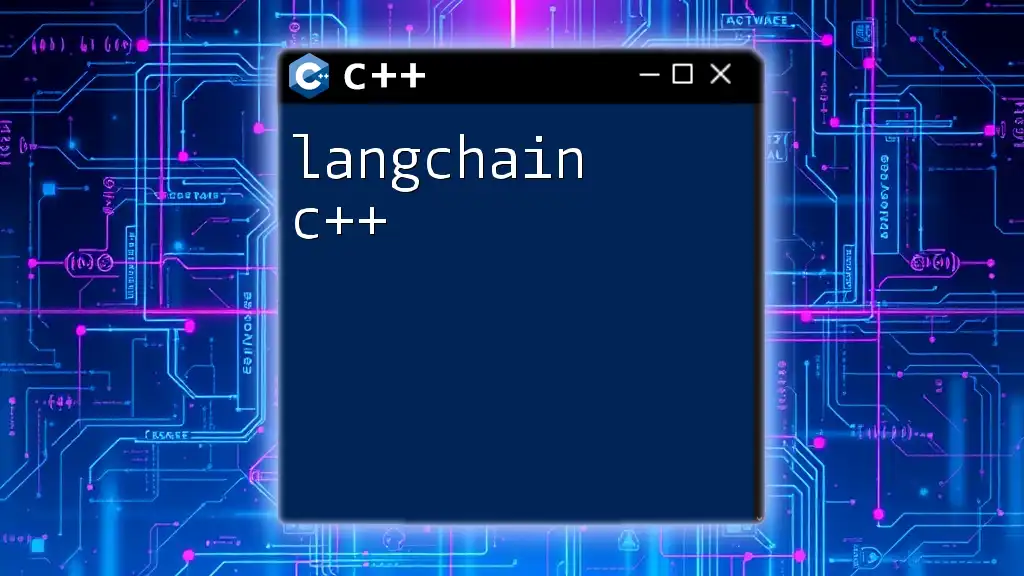
Conclusion
In this comprehensive guide to developing the Hangman game in C++, you’ve learned how to set up your environment, build the game structure, and implement various game mechanics. Through this process, you not only reinforced fundamental programming concepts but also gained hands-on experience in managing user inputs, end-game conditions, and dynamic game state transitions.
The implementation of Hangman in C++ can serve as a fantastic project for aspiring programmers. Feel free to modify and expand upon your game, adding new features and challenges as you continue to enhance your programming skill set. Don’t stop at just Hangman; use this foundational knowledge to explore more complex C++ projects!
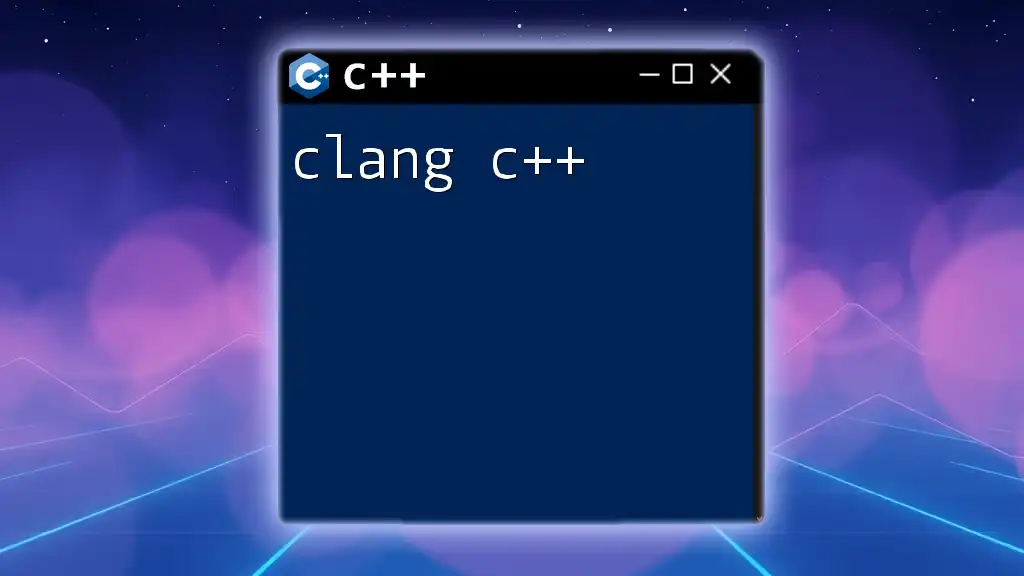
Further Resources
To deepen your understanding of C++ and game development, consider exploring additional literature and online courses tailored to C++. Engaging with communities can also provide invaluable insights and opportunities for collaboration. Happy coding!