In C++, `nan` (Not-a-Number) is a special floating-point value defined in the `<cmath>` header that represents an undefined or unrepresentable value, often resulting from invalid operations like 0 divided by 0.
#include <iostream>
#include <cmath>
int main() {
double result = std::nan("1"); // creates a NaN value
if (std::isnan(result)) {
std::cout << "Result is NaN" << std::endl;
}
return 0;
}
What is NaN in C++?
NaN, short for "Not a Number," is a special floating-point value that represents an undefined or unrepresentable numerical result in computing. It plays a critical role in scientific and mathematical computations, particularly when dealing with invalid operations like dividing zero by zero or taking the square root of a negative number.
In C++, NaN is explicitly defined in the IEEE 754 standard for floating-point arithmetic. This standard ensures that NaN maintains consistency across different computing platforms, making it an integral part of numerical computations.
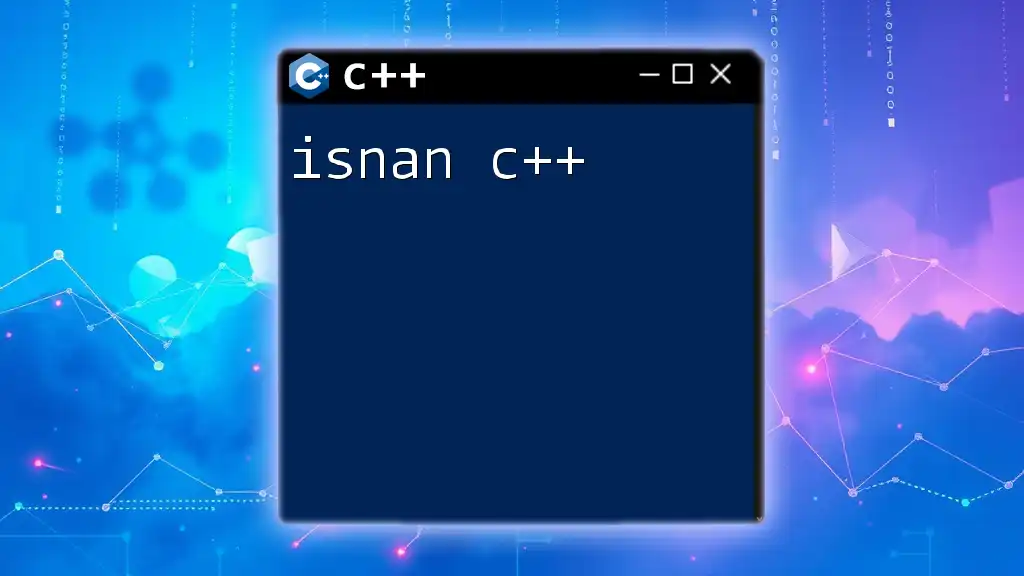
Using NaN in C++
Creating NaN Values in C++
To create NaN values in C++, you can use the `<cmath>` library, which provides various mathematical functions, including a native way to generate NaN.
Here's how you can create a NaN value:
#include <iostream>
#include <cmath>
int main() {
double nanValue = std::nan("1");
std::cout << "NaN Value: " << nanValue << std::endl; // Output: NaN Value: nan
return 0;
}
In the code snippet above, `std::nan("1")` generates a NaN value. The argument "1" is a string used to label or tag this NaN value for debugging purposes but doesn’t affect its functionality.
Identifying NaN in C++
Detecting whether a value is NaN can be done using the `std::isnan()` function from the `<cmath>` library. This function returns a boolean value indicating whether the specified value is NaN.
Here's an example:
#include <iostream>
#include <cmath>
int main() {
double nanValue = std::nan("1");
if (std::isnan(nanValue)) {
std::cout << "The value is NaN." << std::endl; // Output: The value is NaN.
}
return 0;
}
In this example, the `std::isnan()` function checks if `nanValue` is indeed NaN. If true, it outputs a corresponding message.
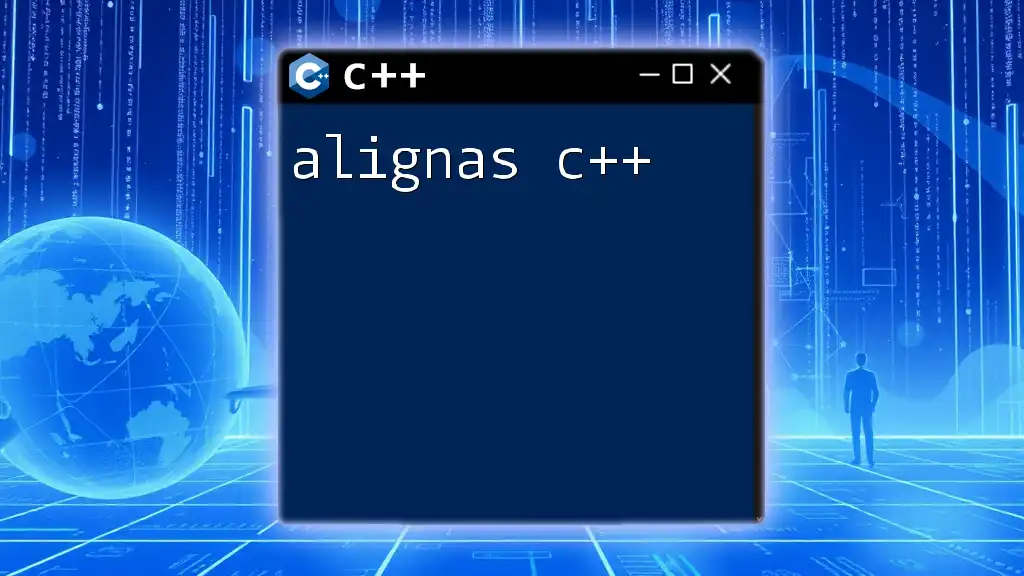
Common Operations with NaN
NaN and Mathematical Operations
One essential characteristic of NaN is that it propagates through mathematical operations. This means that any operation involving NaN will also yield NaN. This behavior is crucial for error detection in computations.
Consider the following code snippet:
#include <iostream>
#include <cmath>
int main() {
double nanValue = std::nan("1");
double result = nanValue + 10.0; // result is also NaN
std::cout << "Result of NaN + 10: " << result << std::endl; // Output: Result of NaN + 10: nan
return 0;
}
Here, adding any number to a NaN value results in NaN, which preserves the integrity of error signaling in computations.
Comparisons Involving NaN
When it comes to comparisons, NaN is unique in that it is not equal to itself. This behavior can lead to unexpected results if not properly anticipated.
Here’s how you might check for equality involving NaN:
#include <iostream>
#include <cmath>
int main() {
double nanValue = std::nan("1");
if (nanValue == nanValue) {
std::cout << "They are equal." << std::endl;
} else {
std::cout << "They are not equal." << std::endl; // Output: They are not equal.
}
return 0;
}
In this example, the equality check involving `nanValue` returns false because, by definition, NaN is never equal to any value, including itself.
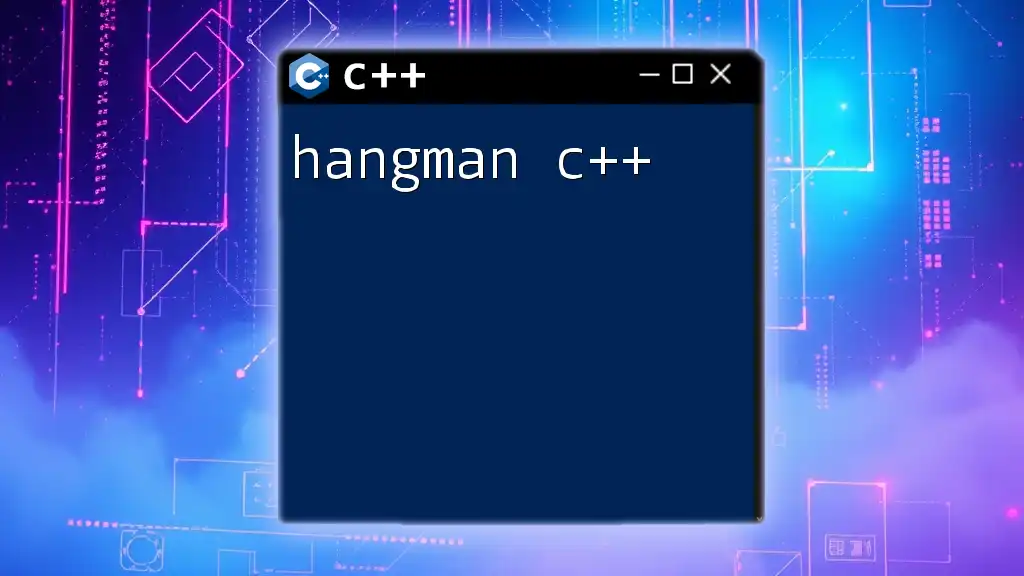
Handling NaN in C++
Best Practices for Dealing with NaN Values
Working with NaN values can be tricky, so adopting best practices is essential. Here are some strategies to consider:
-
Error Checking: Make it a routine practice to check for NaN values before performing calculations. This approach helps prevent the propagation of undefined results.
-
Consistent Data Validation: Use input validation on datasets to identify and handle NaN values early in your data processing pipeline.
Strategies to Manage NaN in Algorithms
When dealing with datasets, it is crucial to filter out NaN values. This ensures that further analysis or computations are not skewed by undefined values.
Here’s a practical example of filtering NaN values from a vector:
#include <iostream>
#include <vector>
#include <cmath>
std::vector<double> filterNaN(const std::vector<double>& data) {
std::vector<double> result;
for (double value : data) {
if (!std::isnan(value)) {
result.push_back(value);
}
}
return result;
}
int main() {
std::vector<double> data = {1.0, std::nan("1"), 3.0, 4.0, std::nan("1")};
std::vector<double> cleanData = filterNaN(data);
for (double value : cleanData) {
std::cout << value << " "; // Output: 1.0 3.0 4.0
}
return 0;
}
In this example, the function `filterNaN` checks each value in the input vector and adds it to the result only if it is not NaN.
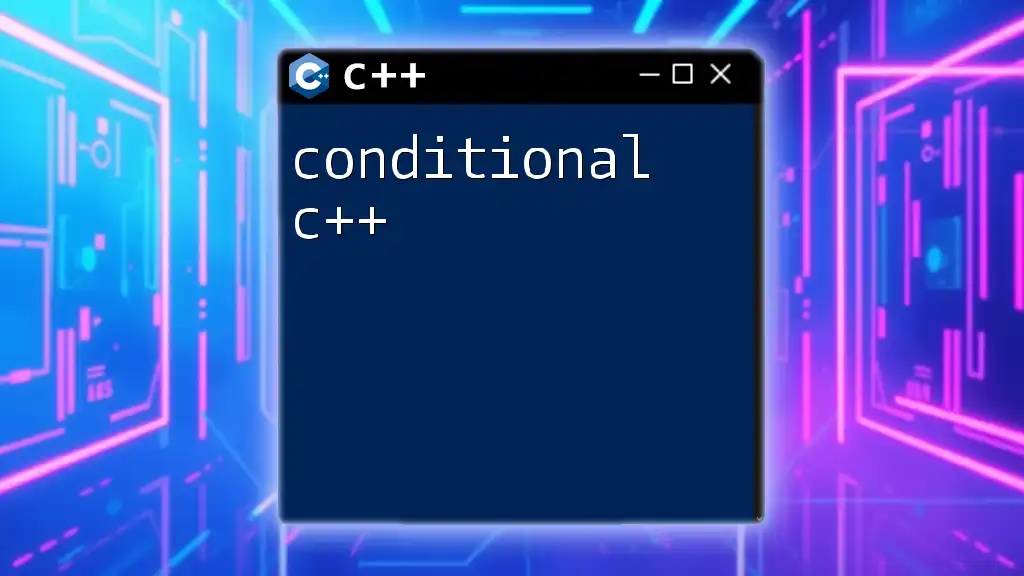
Real-World Applications of NaN Handling
Scientific Computing
In scientific computing, NaN values often indicate measurement errors or invalid calculations. For instance, the result of a mathematical function may yield NaN if inputs are out of a given domain. Therefore, detecting NaN is vital for researchers who rely on accurate results for analysis.
Machine Learning
Handling NaN values properly is essential in machine learning. Datasets that contain NaN can lead to incorrect model training. As such, techniques for preprocessing and cleaning data must include procedures to identify and appropriately manage NaN values to ensure model accuracy and robustness.
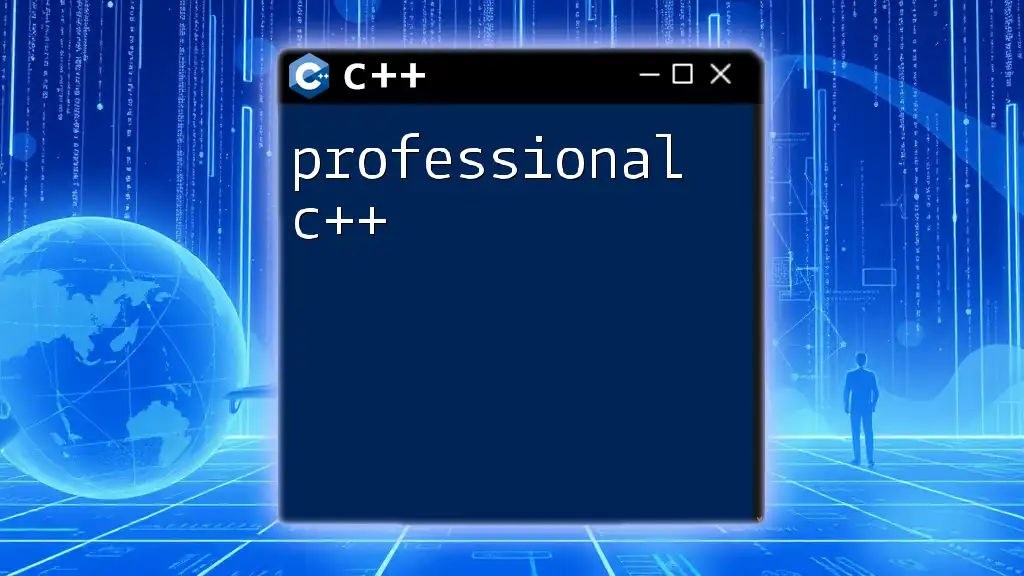
Conclusion
Understanding and effectively managing NaN in C++ is crucial for anyone working in fields that require numerical computations, such as data science, finance, or engineering. By utilizing the tools and techniques outlined in this guide, you can improve the robustness of your C++ applications and ensure that you handle exceptional cases, like "Not a Number," with confidence.
Additional Resources
For further exploration of these concepts, refer to the C++ Standard Library Documentation, which offers in-depth descriptions of the mathematical functions available, including those used for handling NaN values.