The `alignas` specifier in C++ is used to set the alignment requirement of a variable or type, ensuring it is aligned to a specified boundary for performance optimization or compatibility reasons.
#include <iostream>
#include <cstddef>
alignas(16) int alignedVariable; // This variable is aligned to a 16-byte boundary
int main() {
std::cout << "Alignment of alignedVariable: " << alignof(alignedVariable) << " bytes." << std::endl;
return 0;
}
What is Memory Alignment?
Memory alignment refers to arranging data in memory according to certain rules concerning the boundaries on which data types can be stored. Memory alignment is critical in C++ because it directly affects the performance of your application. Misalignment can lead to inefficient memory access patterns and potential crashes due to hardware exceptions on some architectures.
When data is aligned correctly, the CPU can access it more efficiently, leading to improved performance. Well-aligned data can significantly reduce memory access time, minimizing the risk of runtime errors and ensuring smoother operation of your application.

Overview of `alignas`
What is `alignas`?
`alignas` is a specifier introduced in C++11 that allows you to explicitly control the alignment of variables, structures, and classes. By using `alignas`, you can specify the alignment requirement of a variable or user-defined type in bytes.
The syntax is straightforward:
alignas(alignment) type variableName;
Using `alignas` is important in modern C++ as it provides precise control over memory layout and optimization, which can be crucial for performance-critical applications.
Where to Use `alignas`
You might want to use `alignas` whenever you're working with specific data structures, especially in scenarios such as:
- High-Performance Computing (HPC), where data alignment can impact computational efficiency.
- Game Development, where optimized data layout can ensure faster rendering and processing times.
- Embedded Systems, where memory constraints require a meticulous approach to data structuring.
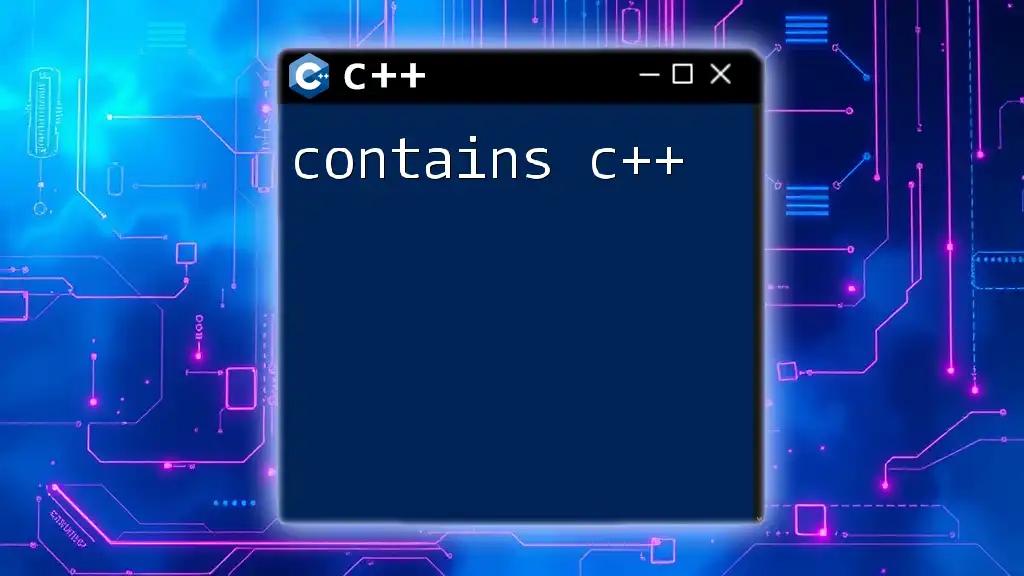
The Syntax of `alignas`
The syntax for `alignas` is very simple. Here’s an example to demonstrate its usage:
alignas(16) int myArray[4];
In this example, we declare an array of four integers (`myArray`) that is aligned on a 16-byte boundary. This means that the starting address of `myArray` will be a multiple of 16, which is particularly useful for SIMD (Single Instruction, Multiple Data) operations and other low-level programming tasks.
This alignment can lead to more efficient memory access. CPUs are designed to read data more efficiently when it is aligned to specific byte boundaries, thus reducing the number of read operations needed and speeding up program execution.

Default and Custom Alignment
Default Alignment in C++
In C++, the compiler provides default alignment requirements for different data types. For example, typically, an `int` will have a default alignment of 4 bytes, while a `double` might have a default alignment of 8 bytes.
The alignment requirements can vary depending on the platform and architecture, so it is crucial to be aware of these defaults when designing your application’s data structures.
Custom Alignment with `alignas`
With `alignas`, you can define custom alignment requirements. Here’s a more complex example:
struct alignas(32) MyStruct {
int x;
double y;
};
In this case, the `MyStruct` structure is explicitly aligned on a 32-byte boundary. This is particularly useful when you intend to use it in performance-critical applications, such as graphics programming or high-frequency trading systems, where misalignment can lead to performance penalties.
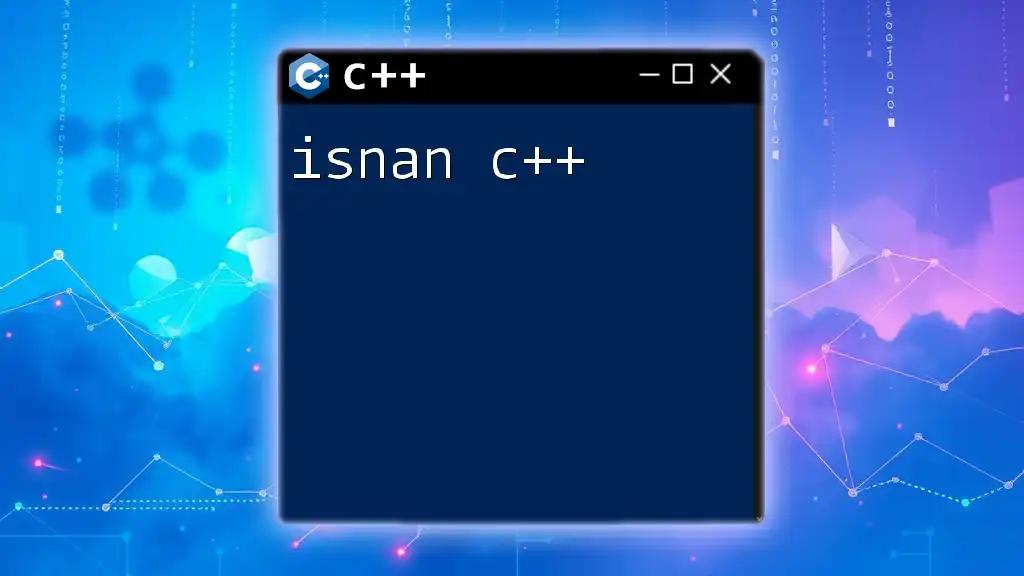
Compatibility and Portability
Using `alignas` contributes to the compatibility and portability of your code across different platforms. However, keep in mind that when specifying alignments, not all platforms might support the same alignment values. This could lead to discrepancies in behavior, especially on architectures with different alignment rules.
Potential Issues with Misalignment:
- Some CPUs may require data to be aligned in specific ways, and accessing misaligned data could raise exceptions.
- Testing alignment thoroughly is crucial when working on cross-platform applications, as each platform might interpret alignment requirements differently.

Best Practices When Using `alignas`
Guidelines for Alignment
-
Use alignment values that are appropriate for your data types. For instance, use 4 bytes for `int`, 8 bytes for `double`, and 16 or 32 bytes for structures heavily used in performance critical paths.
-
Avoid extreme alignment values unless necessary, as this can lead to wasted memory.
Understanding the Trade-offs
While `alignas` can optimize performance, note that excessive alignment can lead to wasted memory. Whenever you specify an alignment, consider the impact on memory usage. Testing with different alignment requirements and observing performance can help identify the most effective alignment levels for your particular use case.
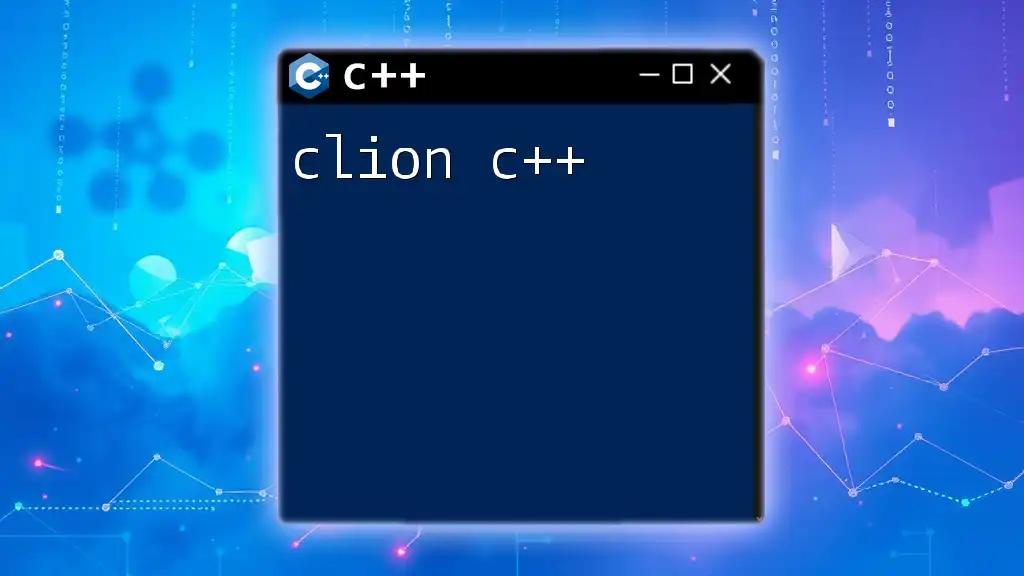
Examples of Using `alignas` in Real Applications
Performance Optimization in High-Performance Computing
Consider a scientific computation application where large arrays of floating-point numbers are processed. By utilizing `alignas` to align these arrays on cache lines, you can improve cache performance. For example:
alignas(64) float dataArray[1024];
In this scenario, aligning `dataArray` on a 64-byte boundary can maximize cache usage, leading to noticeably faster computation times.
Game Development Applications
In game engines, structuring character data with `alignas` can speed up rendering processes. For instance:
struct alignas(16) Character {
float position[3];
float health;
// Other attributes...
};
This ensures that the `Character` structure benefits from alignment optimized for vector processing, which games frequently utilize for physics calculations and rendering.

Debugging Alignment Issues
Common alignment errors might manifest as runtime crashes or erratic program behavior, particularly on architectures that strictly enforce alignment. To debug alignment issues:
- Use compiler flags: Many compilers offer options to warn about alignment mismatches.
- Check the alignment of your data types using the `alignof` operator introduced in C++11, which can assist in understanding how types are aligned.
For example, you can check the alignment of a type like this:
#include <iostream>
#include <type_traits>
struct Unaligned {
char c;
int i;
};
int main() {
std::cout << "Alignment of Unaligned: " << alignof(Unaligned) << std::endl;
}
This will give you the required alignment of `Unaligned`, allowing you to confirm if it meets the expectations laid out in your design.
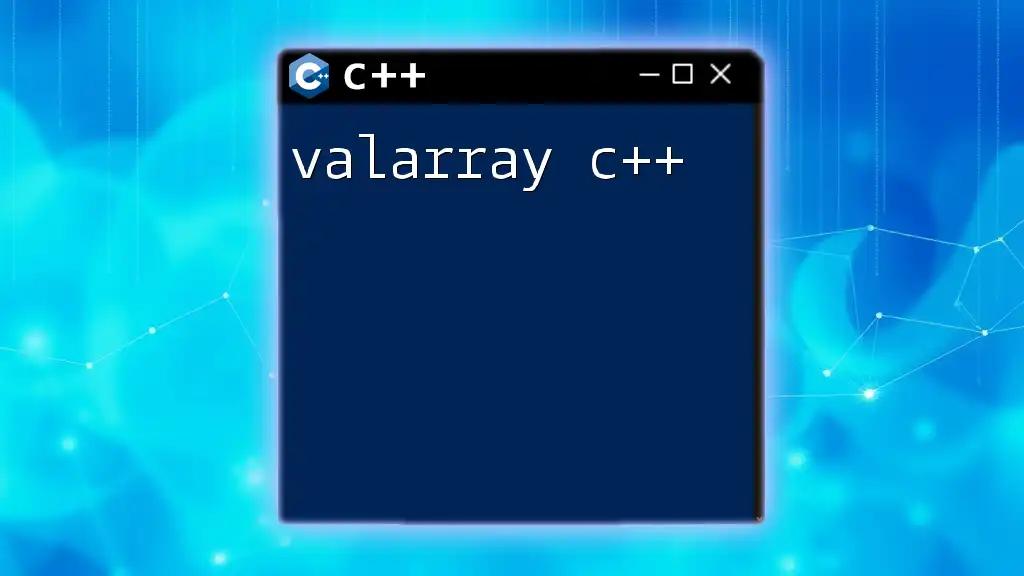
Conclusion
The `alignas` keyword in C++ is a powerful tool that provides explicit control over the alignment of data types. By understanding the benefits and proper usage of `alignas`, you can significantly enhance the performance of your applications, particularly in areas where memory alignment is critical. Remember, alignment plays a crucial role in memory efficiency and program performance, so leveraging `alignas` thoughtfully can lead to considerably better applications.

Call to Action
Experiment with `alignas` in your projects to see its effects firsthand. Alongside this, subscribe to our updates for more C++ tips and tutorials that will elevate your programming skills.