In C++, a type alias allows you to create a new name for an existing type, enhancing code readability and maintainability.
Here’s an example:
using Integer = int;
Integer num = 10;
Understanding C++ Type Aliases
Definition of Type Alias
A type alias in C++ serves as a synonym for an existing type, enhancing readability and making complex declarations simpler. By using type aliases, developers can create more comprehensible code, particularly when working with intricate types. Understanding the difference between type aliases and traditional data types is essential; while a type alias does not create a new type, it serves as an alternative name for an existing one.
Syntax of Creating Type Aliases
Type aliases can be defined using either the `typedef` keyword or the `using` directive. Both methods achieve the same goal but have different syntaxes.
Using `typedef`, the syntax looks like this:
typedef int Integer;
Here, `Integer` becomes an alias for `int`.
The `using` directive is a more modern feature introduced in C++11 and provides a clearer syntax:
using IntegerAlias = int;
Both examples create an alias, but many developers prefer `using` for its readability.
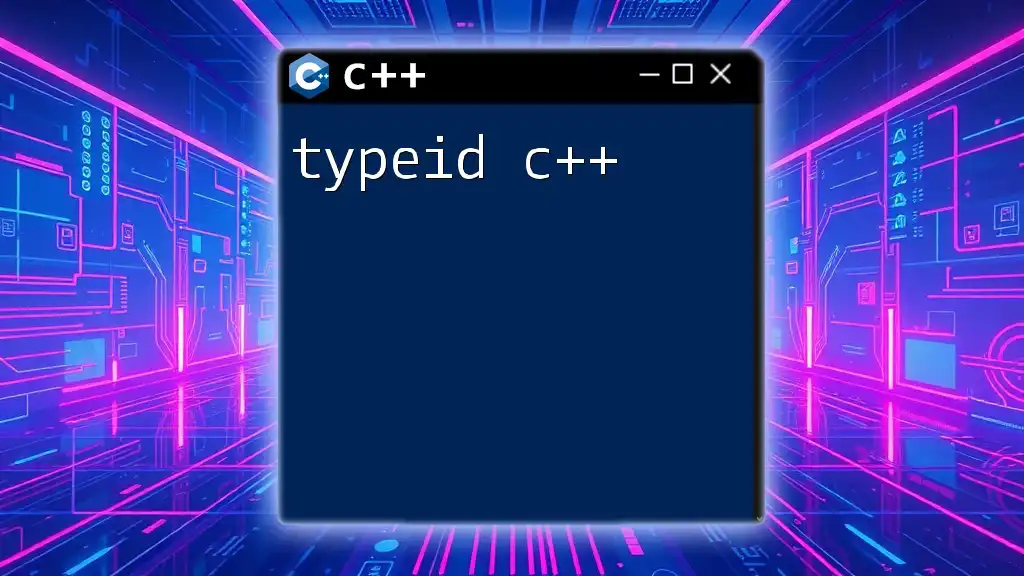
Why Use Type Aliases?
Enhancing Code Readability
Using type aliases can significantly improve the readability of your code. Instead of using long or complex types, you can define a type alias that conveys meaning clearly. For example, if you often use `std::vector<int>` in your code, you can create an alias:
using VectorInt = std::vector<int>;
By using `VectorInt`, your code communicates more effectively, giving readers a better understanding of its purpose at a glance.
Simplifying Complex Type Definitions
Type aliases shine when defining complex types that can hinder code readability. For instance, if you have a function that utilizes a pointer to a function type, using a type alias can clarify intentions:
using CallbackType = void(*)(int, double);
This declaration can be daunting when first encountered in code. However, by encapsulating it in an alias, you give developers a clearer concept of the expected function signature.
Promoting Type Safety
Type aliases also contribute to type safety. They help minimize the potential for errors related to type mismatches. For example, if you frequently pass around a custom type like `MyCustomType`, you can create an alias to ensure consistency:
using CustomTypeAlias = MyCustomType;
This way, you have a clear indication of what type is being used at various places in your code, reducing the chances of incorrect type assignments.
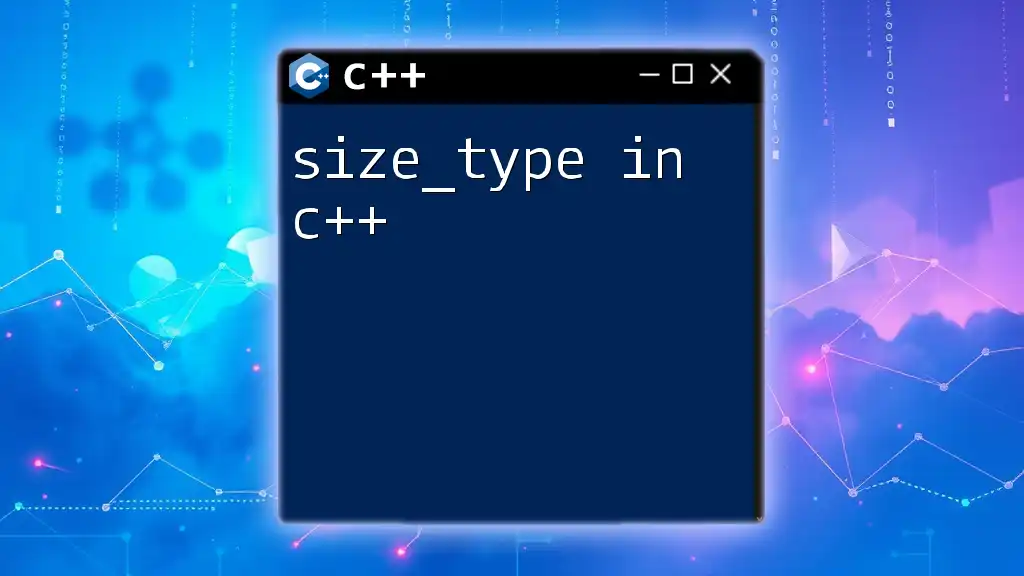
Best Practices for Using C++ Alias
Consistency and Naming Conventions
When utilizing type aliases, it is crucial to adopt consistent naming conventions. Clear and descriptive names should be used so that the purpose of the alias is immediately understandable. For example, using `UserList` instead of just `List` makes it obvious what the alias signifies.
Avoiding Ambiguity
While type aliases can enhance clarity, they run the risk of becoming ambiguous if not carefully managed. Always ensure that your aliases are distinct and communicate their purpose effectively. For instance, creating an alias like `Value` could lead to confusion if there are multiple types of values in your codebase. Instead, consider more descriptive aliases, such as `EmployeeID` or `InvoiceAmount`.
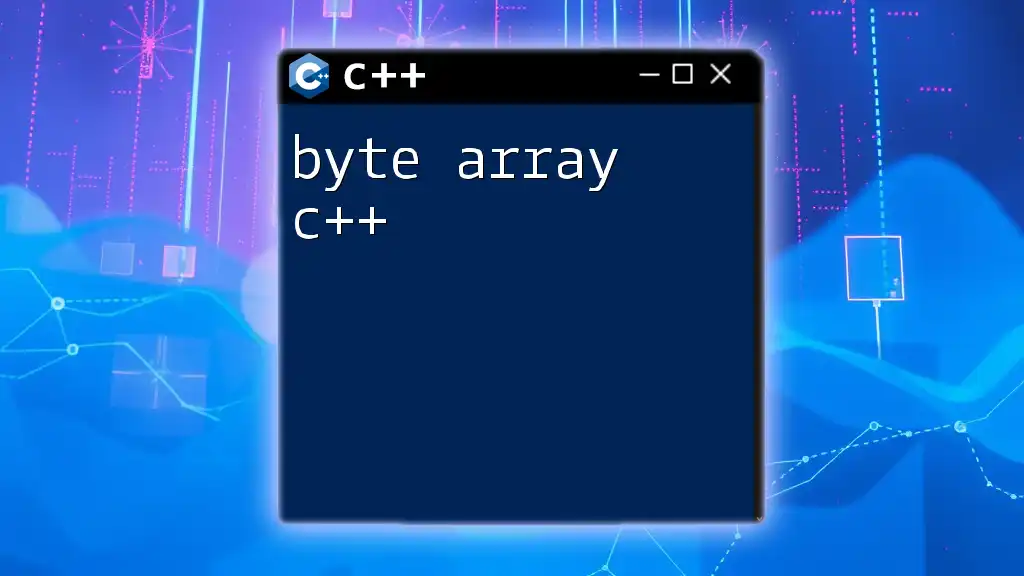
Advanced Usage of Type Aliases
Template Type Aliases
With C++11 onwards, you can create template type aliases, allowing for flexibility in type definitions. This feature can be especially useful when working with generic programming.
template<typename T>
using Ptr = T*; // This creates a alias for a pointer of type T
With this alias, `Ptr<int>` would be equivalent to `int*`, making your code cleaner and more succinct without losing safety.
Type Aliases with Standard Library
Several standard library types can be simplified with type aliases for improved clarity. For instance, when dealing with maps that use `std::string` as keys and `std::vector<std::string>` as values, you can introduce a type alias such as:
using StringMap = std::unordered_map<std::string, std::vector<std::string>>;
This not only reduces code clutter but also enhances the understanding of what data structure is being used, leading to cleaner and more maintainable code.
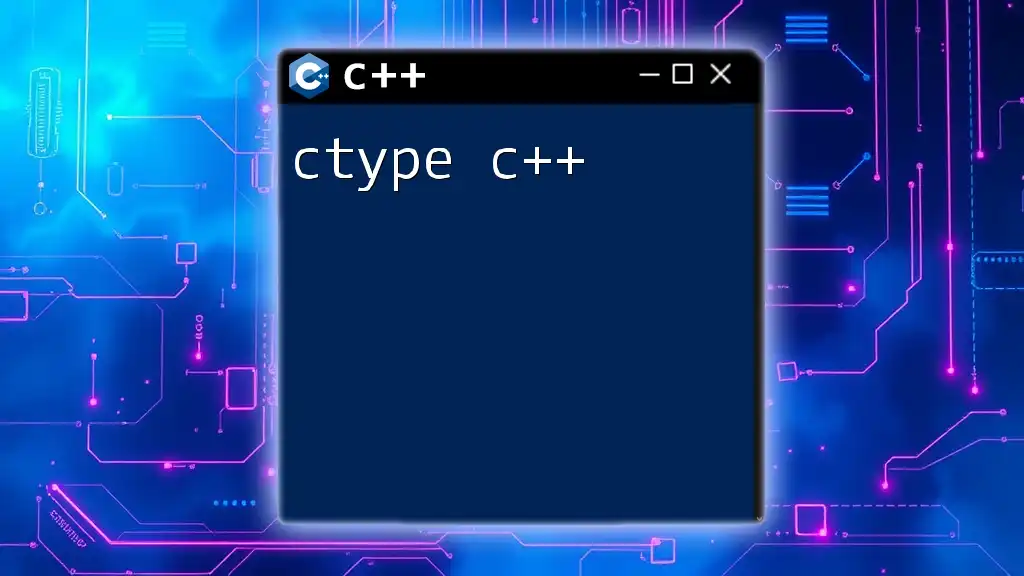
Common Pitfalls When Using Type Aliases
Overuse of Type Aliases
While type aliases can greatly improve the readability of your code, overusing them can lead to confusion. If every type in your code has an alias, developers may struggle to track down the underlying types. It is essential to strike a balance; use aliases when they enhance clarity but avoid clutter.
Lack of Documentation
An oft-overlooked aspect of type aliases is the necessity for documentation. Each alias should be documented to describe its intent and use, especially in larger codebases. A poorly documented alias can confuse developers, especially when it represents more complex or critical types. Always include comments or documentation to clarify your aliases.
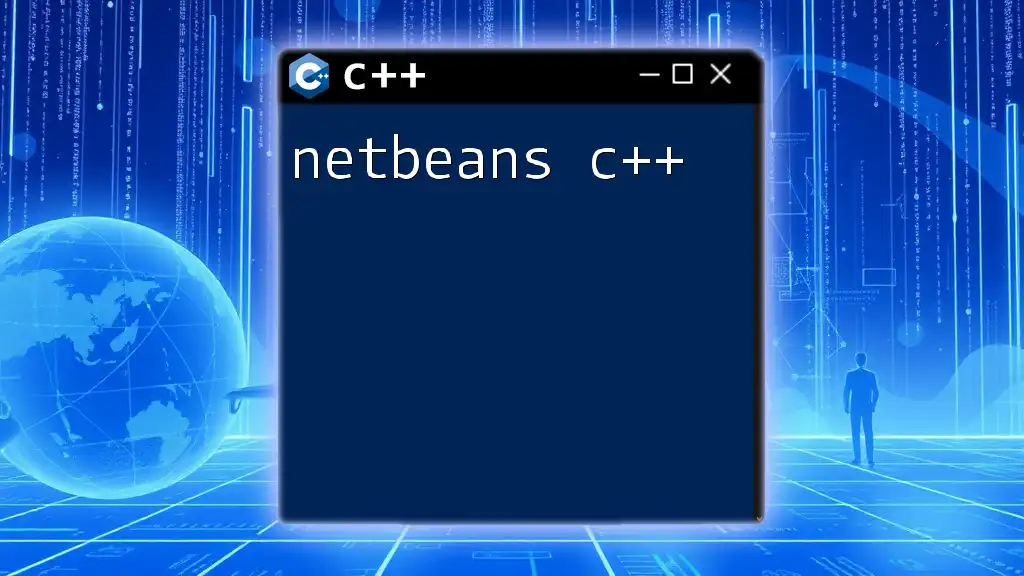
Conclusion
In summary, type aliases in C++ play an essential role in improving code clarity, reducing complexity, and promoting type safety. They allow developers to create more readable and maintainable code by encapsulating complex types and providing meaningful names. By adopting best practices such as consistent naming, avoiding ambiguity, and documenting aliases, you can greatly enhance the effectiveness and usability of your code. Practice using type aliases to see their full potential in your projects.