The `<cctype>` header in C++ provides functions for character classification and conversion, allowing you to easily check and manipulate characters in text.
#include <iostream>
#include <cctype>
int main() {
char ch = 'a';
if (std::isalpha(ch)) {
std::cout << ch << " is an alphabet character." << std::endl;
}
char upper = std::toupper(ch);
std::cout << "The uppercase of " << ch << " is " << upper << "." << std::endl;
return 0;
}
Understanding the Ctype Library
What is Ctype?
The ctype library in C++ is a collection of functions that allows you to classify and manipulate character data. Primarily used to check character types and convert them between cases, ctype plays a crucial role in effective character handling, especially when dealing with string data. Understanding how ctype works can significantly enhance your ability to validate inputs and manipulate text data within your applications.
Ctype Header File
C++ provides the `<cctype>` header file, which contains declarations for all ctype functions. This is the C++ version of the traditional C library `<ctype.h>`. The primary difference between the two is that the former is part of the C++ Standard Library and adapts some of the functions to work with `char` and `wchar_t` types while being safer and cleaner in its use.
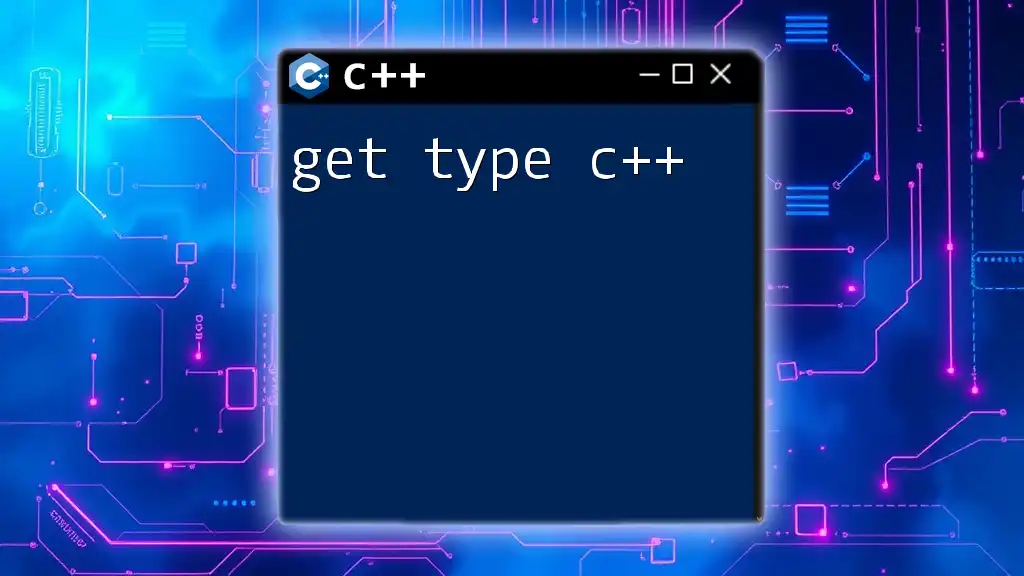
Basic Functions of Ctype
Character Classification
Character classification functions allow you to determine the nature of a character (e.g., whether it is a letter, digit, or whitespace). Let’s explore some of the key classification functions provided by ctype:
isalpha()
The `isalpha()` function checks if a character is an alphabetic letter (either uppercase or lowercase). It returns a non-zero value (true) if the character is an alphabet, or zero (false) otherwise. This can be particularly useful in input validation.
#include <iostream>
#include <cctype>
int main() {
char inputChar = 'A';
if (isalpha(inputChar)) {
std::cout << inputChar << " is an alphabet." << std::endl;
} else {
std::cout << inputChar << " is not an alphabet." << std::endl;
}
return 0;
}
isdigit()
The `isdigit()` function evaluates whether a character is a digit (0-9). It's widely used in scenarios where numeric input is expected.
#include <iostream>
#include <cctype>
int main() {
char inputChar = '5';
if (isdigit(inputChar)) {
std::cout << inputChar << " is a digit." << std::endl;
} else {
std::cout << inputChar << " is not a digit." << std::endl;
}
return 0;
}
isspace()
The `isspace()` function detects whitespace characters such as space, tab, and newline. This function is useful when processing text data to handle formatting and separation.
#include <iostream>
#include <cctype>
int main() {
char inputChar = ' ';
if (isspace(inputChar)) {
std::cout << "Whitespace detected." << std::endl;
} else {
std::cout << "No whitespace." << std::endl;
}
return 0;
}
Character Conversion
Ctype also provides functions for character conversion, allowing you to transform characters to their respective cases.
tolower()
The `tolower()` function converts a character to lowercase. It is particularly handy when standardizing input data for comparison.
#include <iostream>
#include <cctype>
int main() {
char inputChar = 'G';
char lowerChar = tolower(inputChar);
std::cout << "Lowercase: " << lowerChar << std::endl;
return 0;
}
toupper()
In contrast, the `toupper()` function converts a character to uppercase. This is useful for normalizing string data before processing.
#include <iostream>
#include <cctype>
int main() {
char inputChar = 'h';
char upperChar = toupper(inputChar);
std::cout << "Uppercase: " << upperChar << std::endl;
return 0;
}
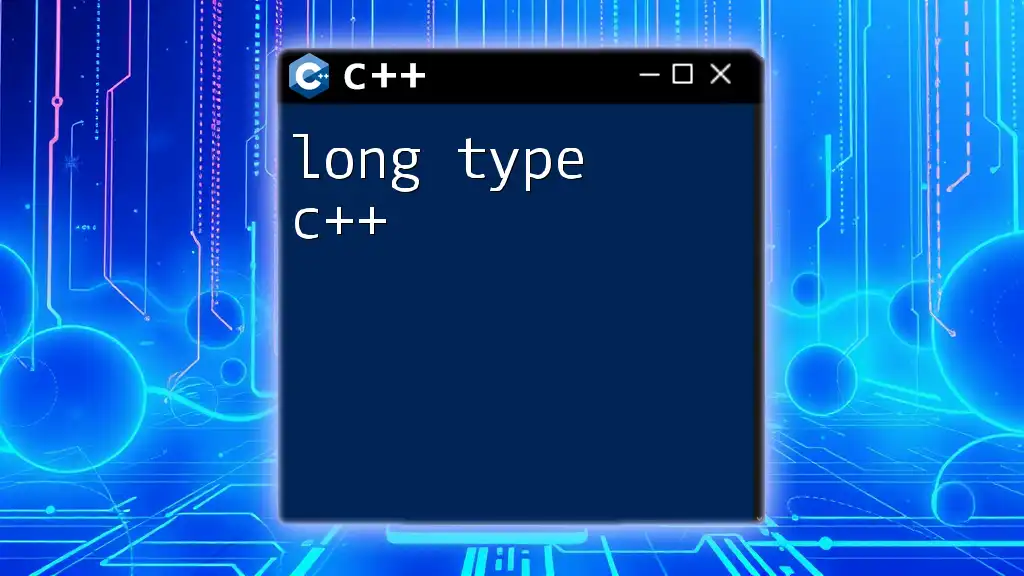
Advanced Uses of Ctype
Combining Classification and Conversion
Utilizing classification and conversion functions together can streamline many text processing tasks. For instance, consider creating a function that converts a character to lowercase only if it is an alphabet.
#include <iostream>
#include <cctype>
void convertToLower(char c) {
if (isalpha(c)) {
std::cout << "Lowercase: " << static_cast<char>(tolower(c)) << std::endl;
} else {
std::cout << c << " is not an alphabet." << std::endl;
}
}
int main() {
convertToLower('A'); // Lowercase: a
convertToLower('1'); // 1 is not an alphabet.
return 0;
}
Custom Character Classifiers
You also have the option to create custom character classification functions that fit your specific needs. This flexibility can be particularly valuable when dealing with specialized input formats or validation rules.
#include <iostream>
#include <cctype>
bool isSpecialCharacter(char c) {
return !isalpha(c) && !isdigit(c) && !isspace(c);
}
int main() {
char testChar = '#';
if (isSpecialCharacter(testChar)) {
std::cout << testChar << " is a special character." << std::endl;
} else {
std::cout << testChar << " is not a special character." << std::endl;
}
return 0;
}
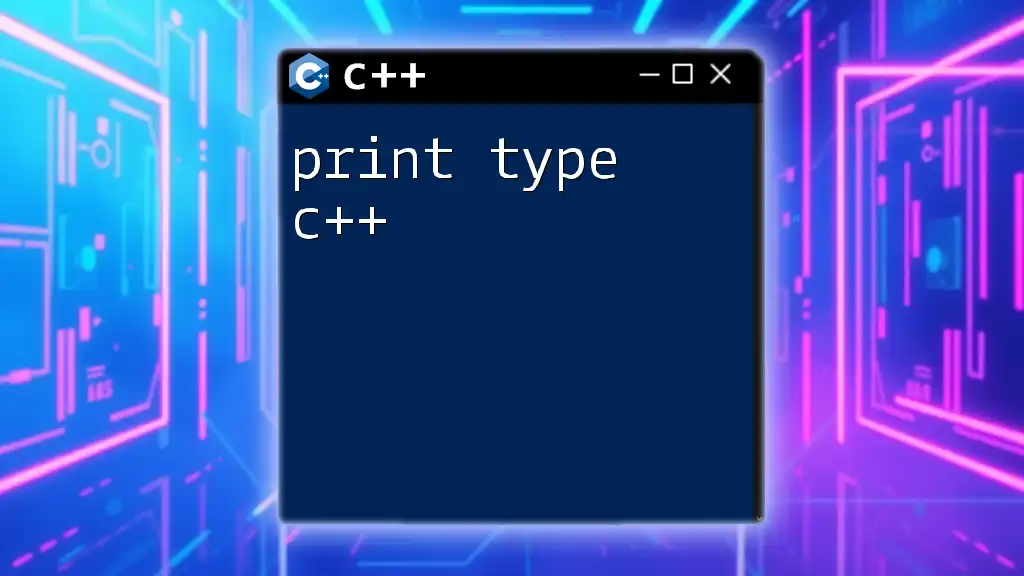
Performance Considerations
Efficiency of Ctype in C++
When it comes to performance, using ctype functions is generally more efficient than implementing manual checks for character types and transformations. The ctype functions are highly optimized, making them ideal for applications that require processing large strings or repeated character-check operations. Benchmarks have shown that ctype functions outperform equivalent manual checks in cases with significant input sizes.
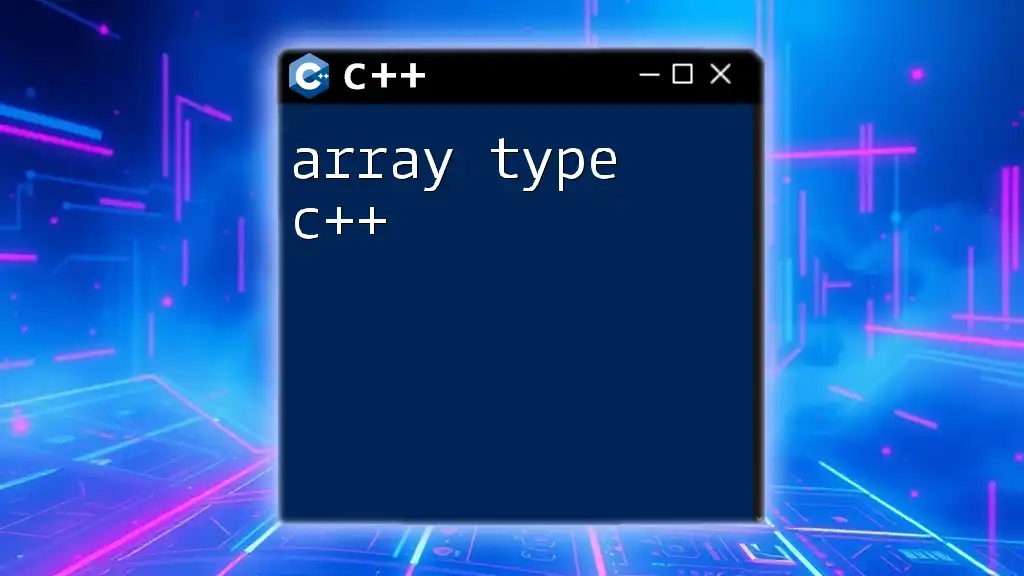
Common Mistakes to Avoid
While working with ctype functions, keep the following common pitfalls in mind:
- Using the wrong character type: Ensure you’re passing a `char` or `unsigned char` to the ctype functions. Passing values outside of these types can lead to undefined behavior.
- Overlooking locale differences: Be aware that ctype functions may behave differently depending on the current locale settings, particularly in non-ASCII character handling.
- Confusing classification and conversion functions: Remember that classification functions return a boolean value indicating character properties, while conversion functions modify the character itself.
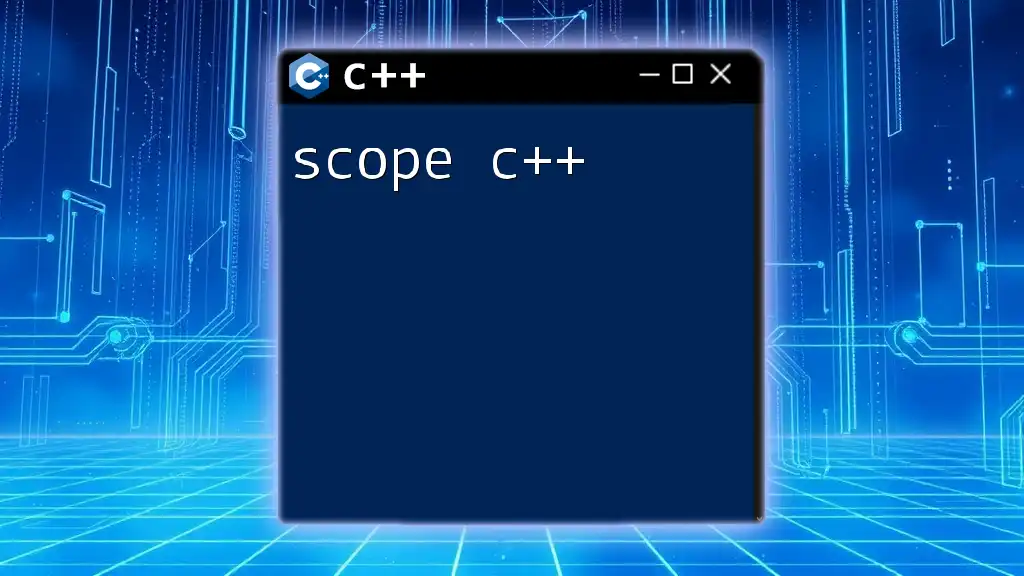
Real-World Applications of Ctype
Data Validation
Ctype functions are incredibly effective for input validation tasks. For example, when eliciting user input, you can leverage these functions to ensure only the desired types of characters are accepted.
#include <iostream>
#include <cctype>
#include <string>
bool validateInput(const std::string& input) {
for (char c : input) {
if (!isalpha(c) && !isdigit(c)) {
return false; // Invalid character found
}
}
return true; // All characters valid
}
int main() {
std::string userInput = "Hello123";
if (validateInput(userInput)) {
std::cout << "Input is valid." << std::endl;
} else {
std::cout << "Input is invalid." << std::endl;
}
return 0;
}
Text Processing
Ctype functions can enhance various text-processing tasks, such as parsing and formatting. For example, you could use these functions to sanitize inputs by removing unwanted characters or capitalizing the first letter of each word in a string.
#include <iostream>
#include <cctype>
#include <string>
std::string capitalizeFirstLetter(std::string input) {
if (!input.empty()) {
input[0] = toupper(input[0]);
}
return input;
}
int main() {
std::string text = "hello world";
std::cout << capitalizeFirstLetter(text) << std::endl; // Hello world
return 0;
}
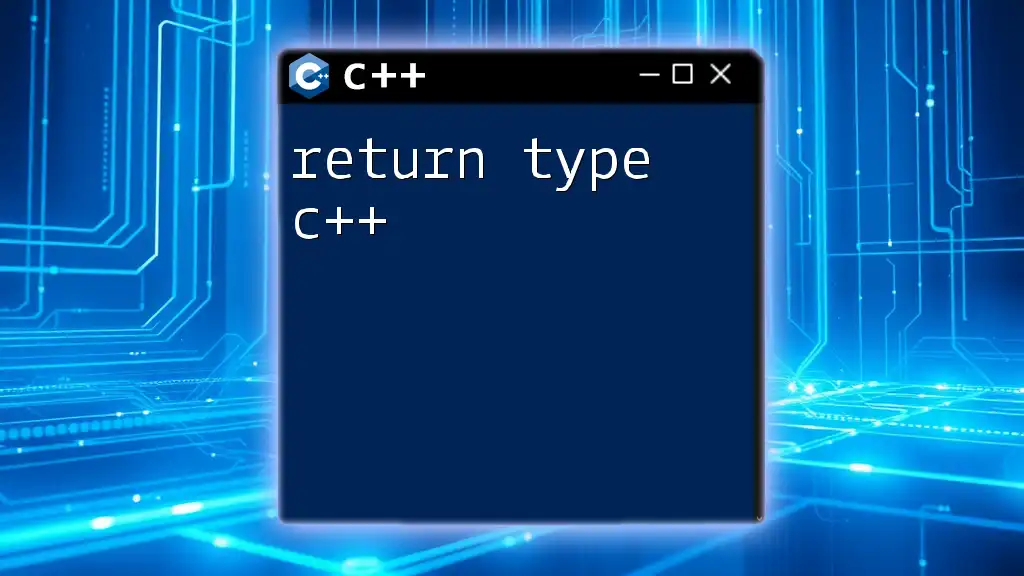
Conclusion
The ctype library is a powerful tool in C++ that offers various functions for character classification and conversion. By mastering ctype functions such as `isalpha()`, `isdigit()`, `tolower()`, and `toupper()`, you can efficiently manage and manipulate character data in your applications. These capabilities make it a fundamental component for tasks ranging from input validation to text processing. By integrating ctype effectively into your coding practices, you can enhance your programming efficiency and output quality, leading to better software solutions.
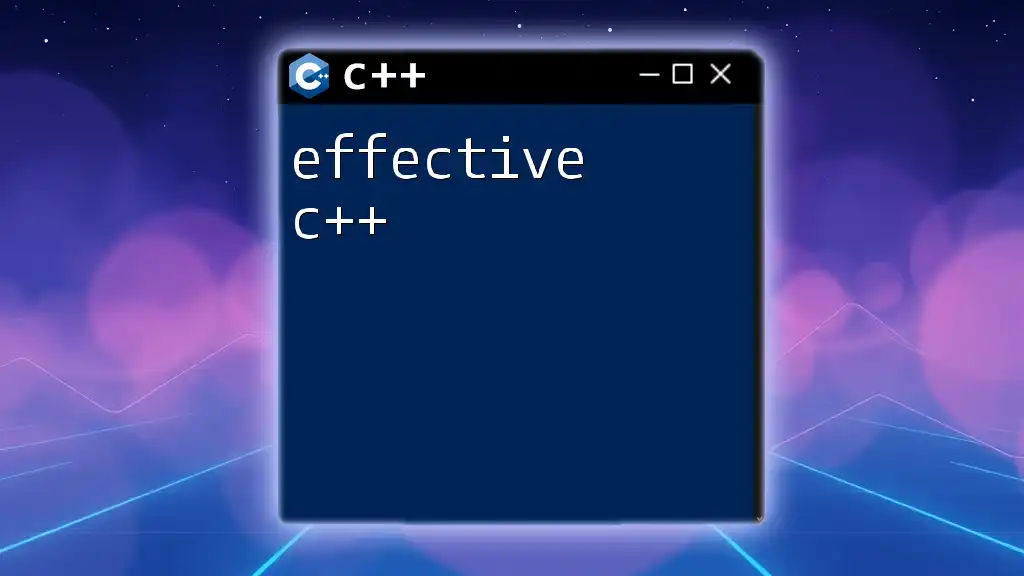
Additional Resources
For further exploration of C++ character handling, consider checking out the C++ Standard Library documentation, online coding tutorials, and community forums to connect with other developers. Understanding the full potential of the ctype library can open up new tools and techniques in your programming toolkit.