The `rotate` function in C++ is used to rotate the elements in a range, moving the first `n` elements to the end of the range while shifting the rest to the front.
Here’s an example in code:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::rotate(vec.begin(), vec.begin() + 2, vec.end());
for (int i : vec) {
std::cout << i << " "; // Output: 3 4 5 1 2
}
return 0;
}
Understanding Rotation
Rotation is a fundamental concept in programming where elements in a data structure, such as arrays or vectors, are shifted circularly. This means that elements that go off one end of the structure re-enter from the other end. Understanding the mechanics of rotation can significantly enhance your algorithmic skill set, especially in contexts like sorting, searching, and game development.
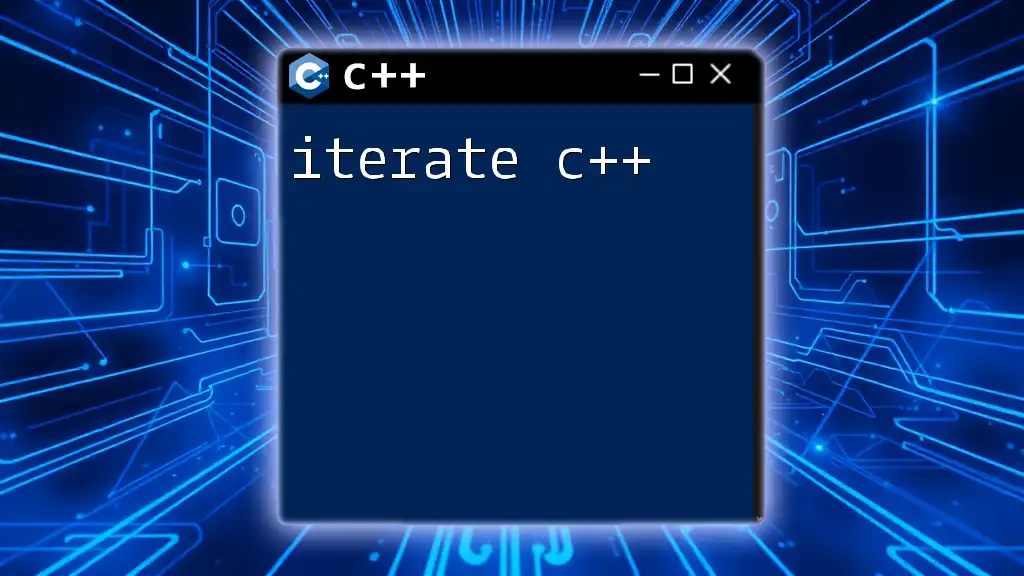
Key Concepts
In C++, when we refer to rotation, we typically mean the manipulation of arrays or vectors. Two primary types of rotation are utilized:
- Clockwise Rotation: Shifts elements to the right. For example, if we rotate the array `[1, 2, 3, 4]` clockwise by one position, it becomes `[4, 1, 2, 3]`.
- Counter-Clockwise Rotation: Shifts elements to the left. Following the previous example, rotating the array counter-clockwise by one position would change it to `[2, 3, 4, 1]`.
Understanding the difference between these two types of rotation is crucial for implementing the right algorithm in your code.
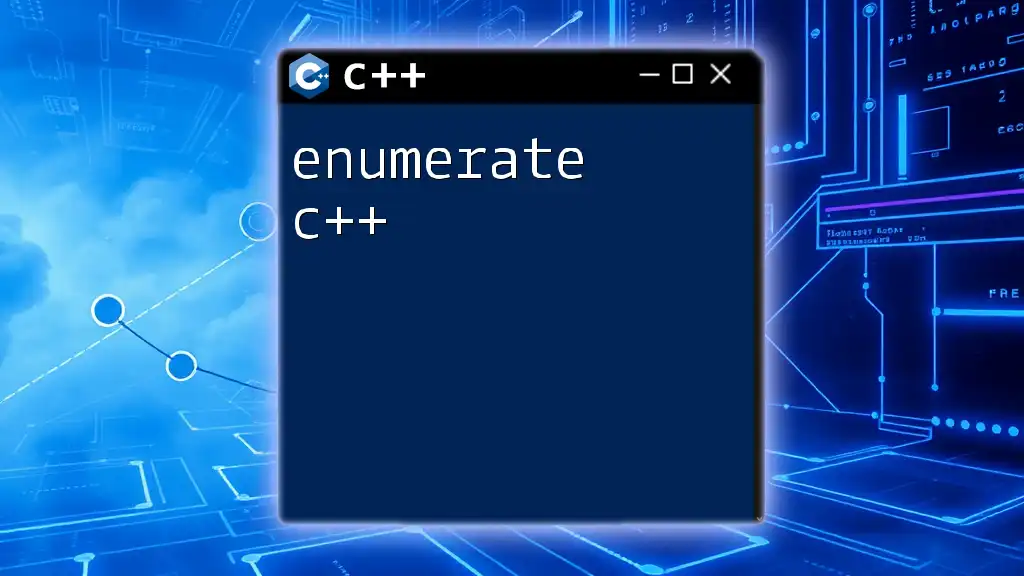
Using Built-in C++ Functions for Rotation
C++ STL and Rotate Function
The Standard Template Library (STL) in C++ provides a powerful function called `std::rotate()`, which simplifies the process of rotating a range of elements.
Syntax of `std::rotate`
std::rotate(first, middle, last);
- first: Iterator pointing to the beginning of the range to rotate.
- middle: Iterator pointing to the new starting point of the rotated range.
- last: Iterator pointing to the end of the range.
Example of using `std::rotate`
To illustrate the effectiveness of `std::rotate`, here’s a simple code snippet:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> arr = {1, 2, 3, 4, 5};
std::rotate(arr.begin(), arr.begin() + 2, arr.end());
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
Explanation: In this example, the vector is rotated to the left by 2 positions. The output will be `3 4 5 1 2`. Here, `arr.begin() + 2` determines the new starting point after the rotation.
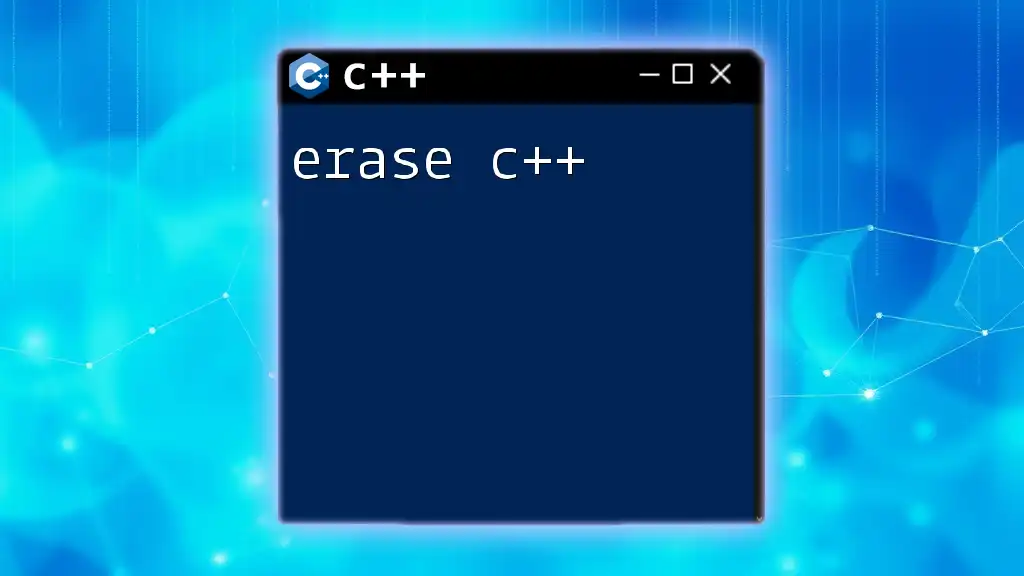
How to Rotate Arrays Manually
Implementing Manual Rotation Techniques
While `std::rotate` is efficient, manually implementing rotation techniques can provide deeper insight into algorithms.
Example of Manual Left Rotation
A simple approach to rotating an array to the left by one position can be implemented as follows:
#include <iostream>
#include <vector>
void leftRotate(std::vector<int>& arr) {
int first = arr[0];
for (size_t i = 1; i < arr.size(); i++) {
arr[i - 1] = arr[i];
}
arr[arr.size() - 1] = first;
}
int main() {
std::vector<int> arr = {1, 2, 3, 4, 5};
leftRotate(arr);
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
Explanation: Here, the first element is stored and then each subsequent element is shifted left. Finally, the stored first element is placed at the end. The output will be `2 3 4 5 1`.
Example of Manual Right Rotation
For rotating an array to the right by one position, the implementation would look like this:
#include <iostream>
#include <vector>
void rightRotate(std::vector<int>& arr) {
int last = arr[arr.size() - 1];
for (int i = arr.size() - 1; i > 0; i--) {
arr[i] = arr[i - 1];
}
arr[0] = last;
}
int main() {
std::vector<int> arr = {1, 2, 3, 4, 5};
rightRotate(arr);
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
Detailed Explanation: This code snippet follows a similar logic, where the last element is stored, the rest of the elements are shifted right, and the last element is set at the first position, giving an output of `5 1 2 3 4`.
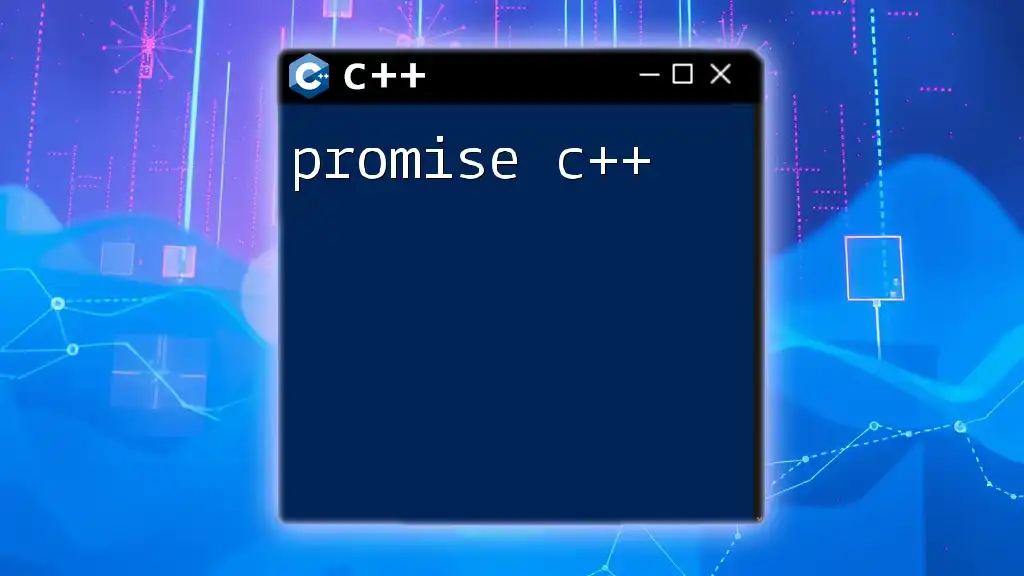
Advanced Rotation Techniques
Optimizing Rotation Algorithms
When working with larger datasets, optimizing your rotation algorithms becomes necessary.
Understanding Rotation with Reversal Method
One advanced technique is to use the reversal method, which involves three steps to achieve the desired rotation with better efficiency. This method operates in O(n) time complexity.
- Reverse the entire array.
- Reverse the first k elements (for left rotation).
- Reverse the remaining n-k elements.
Here's how you can implement this:
#include <iostream>
#include <vector>
#include <algorithm>
void reverse(std::vector<int>& arr, int start, int end) {
while (start < end) {
std::swap(arr[start], arr[end]);
start++;
end--;
}
}
void rotateUsingReversal(std::vector<int>& arr, int k) {
int n = arr.size();
k = k % n; // To handle k greater than n
reverse(arr, 0, n - 1);
reverse(arr, 0, n - k - 1);
reverse(arr, n - k, n - 1);
}
int main() {
std::vector<int> arr = {1, 2, 3, 4, 5};
rotateUsingReversal(arr, 2);
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
Explanation: This method rotates the array either left or right by k positions in a systematic way, drastically improving efficiency over naive methods, especially as the size of the dataset increases.
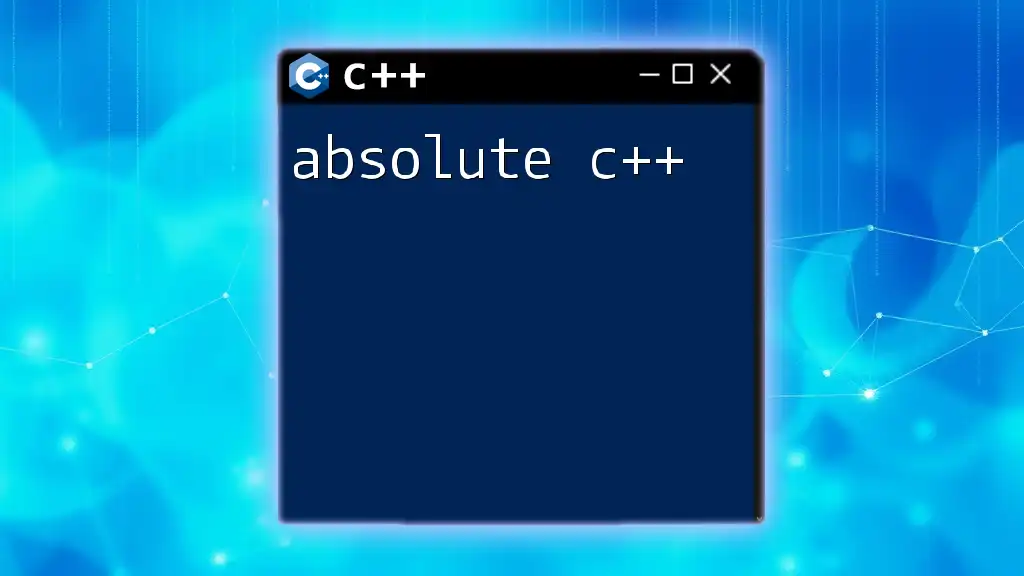
Handling Edge Cases in Rotations
When implementing rotation functions, it is crucial to handle potential issues:
- Rotating Empty Arrays: When the array has no elements, any rotation should simply return the array as is.
- Over-rotating: If k exceeds the size of the array, using `k = k % n` will ensure that the extra rotations do not affect the outcome.
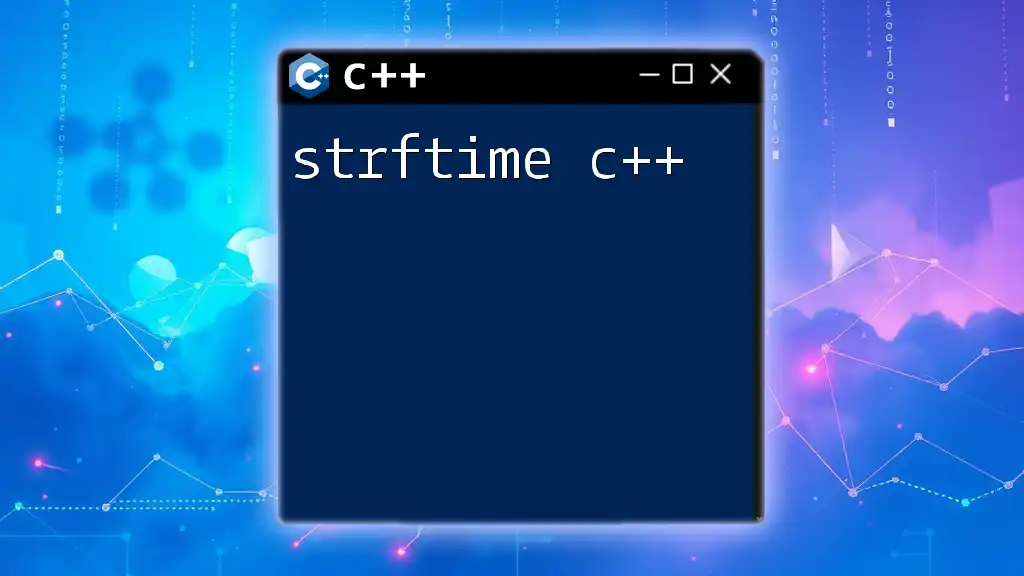
Practical Applications of Rotation in C++
Understanding and utilizing the concept of rotation opens doors to various applications, such as:
- Data Processing: Rotating data structures can optimize searching or sorting algorithms.
- Game Development: Rotating sprites or models in animation mechanics is essential.
- Algorithms: Many algorithmic challenges require the manipulation of data in a rotating manner, making this skill invaluable.
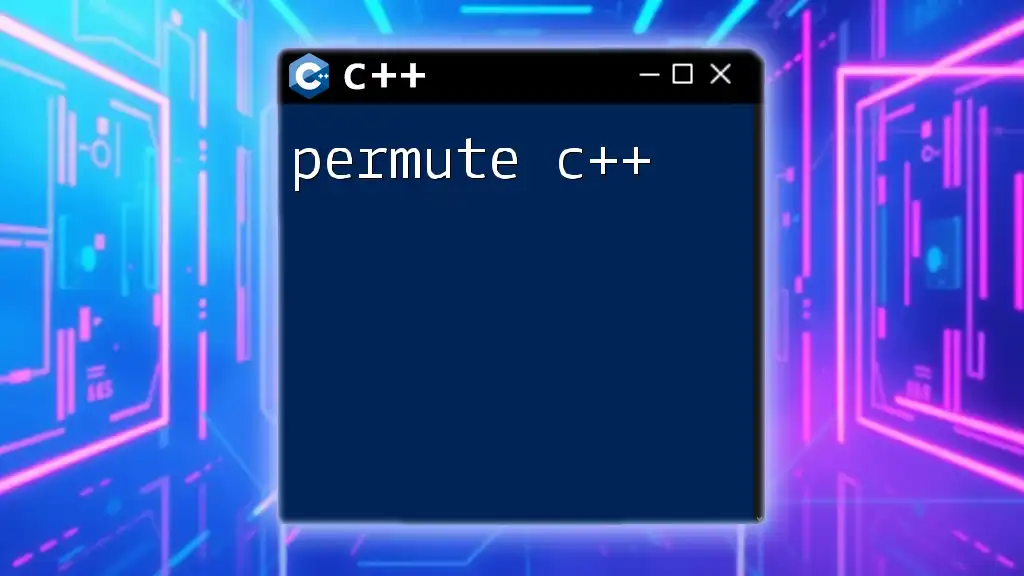
Recap of Key Points
This comprehensive understanding of rotate C++ not only highlights the utility of built-in functions like `std::rotate` but also encourages the exploration of manual methods. The practical applications discussed further showcase the significance of mastering rotation techniques in C++.
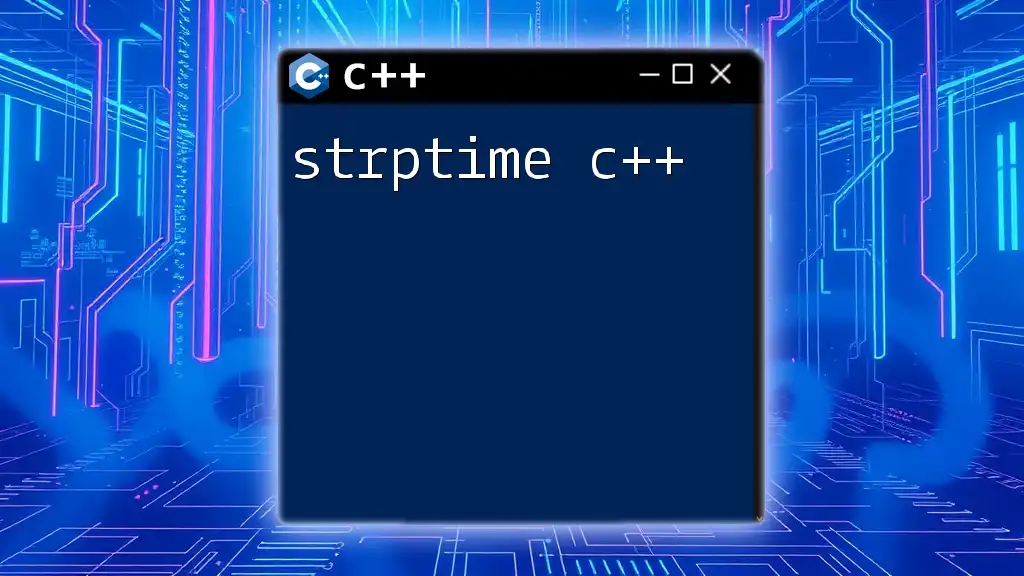
Encouragement to Practice
By practicing various rotation algorithms, you will solidify your understanding and become proficient in effectively manipulating data structures in C++. Start implementing these concepts in your projects and exercises to leverage their full potential!
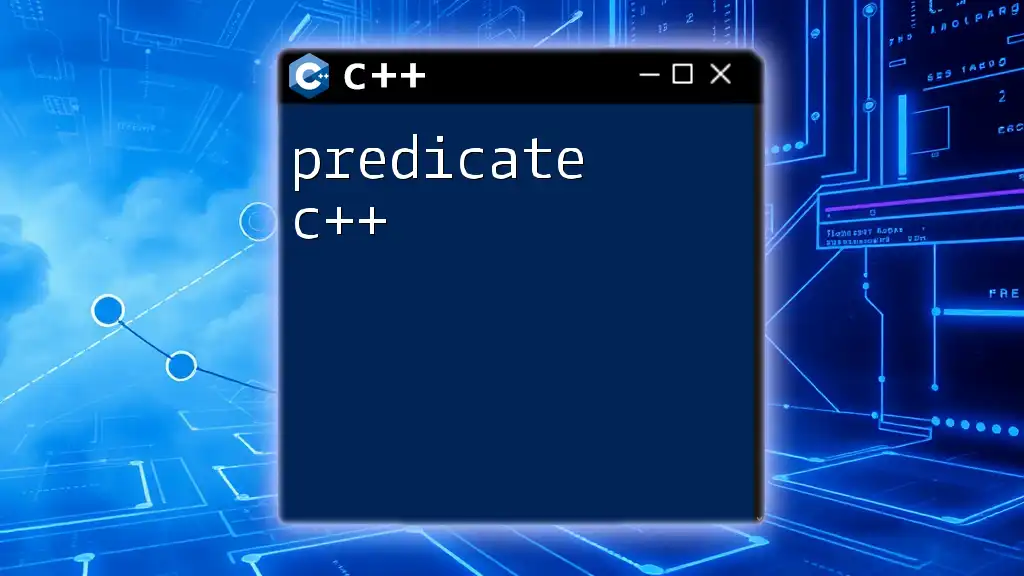
Additional Resources
For more extensive learning, consider referencing textbooks on algorithms, online coding platforms offering exercises, and other tutorials specifically focusing on C++ data structures and algorithms. These will complement the skills you've acquired here and assist in mastering rotation techniques.